全局的
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>谷歌炸了</title>
</head>
<body>
<div id="app">
<div v-loading="isLoading">
<div>{{message}}</div>
<button @click="update">更新</button>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
Vue.directive('loading', {
update(el, binding, vnode) {
console.log(el, binding, vnode)
if (binding.value) {
const div = document.createElement('div');
div.innerText = "加载中...",
div.setAttribute('id', 'loading');
div.style.position = 'fixed';
div.style.left = 0;
div.style.right = 0;
div.style.width = '100%';
div.style.height = "100%";
div.style.display = 'flex';
div.style.justifyContent = 'center';
div.style.alignItems = "center";
div.style.color = "white";
div.style.background = "rgba(0,0,0,0.7)";
document.body.append(div);
} else {
document.body.removeChild(document.getElementById('loading'))
}
}
})
new Vue({
el: "#app",
data: {
isLoading: false,
message: ''
},
methods: {
update() {
this.isLoading = true;
setTimeout(() => {
this.message = "更新完成!";
this.isLoading = false;
}, 3000);
}
}
})
</script>
</body>
</html>
组件内部的
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<div v-loading="isLoading">
<div>{{message}}</div>
<button @click="update">更新</button>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const app = new Vue({
el: "#app",
data: {
isLoading: false,
message: ''
},
methods: {
update() {
this.isLoading = true;
setTimeout(() => {
this.message = "更新完成!";
this.isLoading = false;
}, 3000);
}
},
directives: {
loading: {
update(el, binding, vnode) {
console.log(el, binding, vnode)
if (binding.value) {
const div = document.createElement('div');
div.innerText = "加载中...",
div.setAttribute('id', 'loading');
div.style.position = 'fixed';
div.style.left = 0;
div.style.right = 0;
div.style.bottom = 0;
div.style.width = '100%';
div.style.height = "100%";
div.style.display = 'flex';
div.style.justifyContent = 'center';
div.style.alignItems = "center";
div.style.color = "white";
div.style.background = "rgba(0,0,0,0.7)";
document.body.append(div);
} else {
document.body.removeChild(document.getElementById('loading'))
}
}
}
}
})
</script>
</body>
</html>
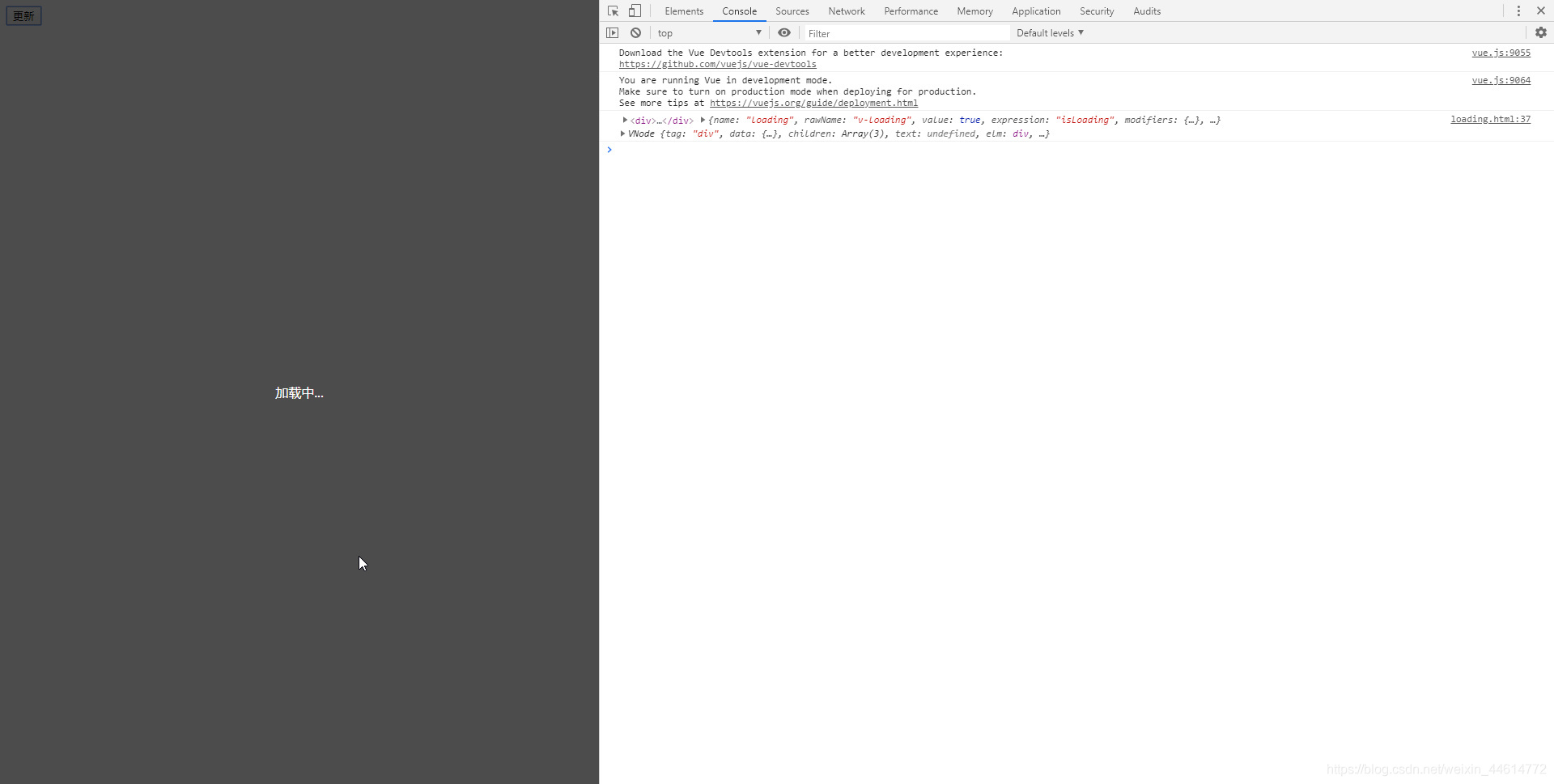
extend制作loading
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>谷歌炸了</title>
<style>
#loading-wrapper{
position: fixed;
top:0;
left:0;
right:0;
display: flex;
justify-content: center;
align-items: center;
width:100%;
height:100%;
background:rgba(0,0,0,0.7);
color:#fff;
}
</style>
</head>
<body>
<div id="app">
<button @click="showLoading">显示Loading</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const LoadingComponent = Vue.extend({
template: '<div id="loading-wrapper">{{msg}}</div>',
props: {
msg: {
type: String,
default: 'loading...'
}
}
}, 'LoadingComponent')
function loading(msg) {
console.log(msg)
const div = document.createElement('div');
div.setAttribute('id', 'loading-wrapper');
document.body.append(div);
new LoadingComponent({
props: {
msg: {
type: String,
default: msg
}
}
}).$mount('#loading-wrapper')
return ()=>{
document.body.removeChild(document.getElementById('loading-wrapper'))
}
}
Vue.prototype.$loading=loading
new Vue({
el: "#app",
data: {
isLoading: false,
message: ''
},
methods: {
showLoading() {
const hide = this.$loading('正在加载中...');
setTimeout(() => {
hide();
}, 100)
}
}
})
</script>
</body>
</html>
将上面的代码封装成插件
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>谷歌炸了</title>
<style>
#loading-wrapper {
position: fixed;
top: 0;
left: 0;
right: 0;
display: flex;
justify-content: center;
align-items: center;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.7);
color: #fff;
}
</style>
</head>
<body>
<div id="app">
<button @click="showLoading">显示Loading</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const loadingPlugin = {
install: function (vm) {
const LoadingComponent = Vue.extend({
template: '<div id="loading-wrapper">{{msg}}</div>',
props: {
msg: {
type: String,
default: 'loading...'
}
}
}, 'LoadingComponent')
function loading(msg) {
console.log(msg)
const div = document.createElement('div');
div.setAttribute('id', 'loading-wrapper');
document.body.append(div);
new LoadingComponent({
props: {
msg: {
type: String,
default: msg
}
}
}).$mount('#loading-wrapper')
return ()=>{
document.body.removeChild(document.getElementById('loading-wrapper'))
}
}
vm.prototype.$loading=loading
}
}
Vue.use(loadingPlugin)
new Vue({
el: "#app",
data: {
isLoading: false,
message: ''
},
methods: {
showLoading() {
const hide = this.$loading('正在加载中...');
setTimeout(() => {
hide();
}, 1000)
}
}
})
</script>
</body>
</html>