首先创建后台用户表
php artisan make:migration CreateAdminUserTable
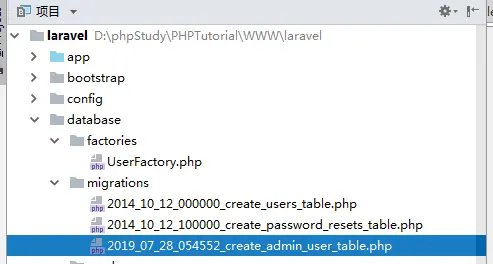
结果
打开设置表字段
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateAdminUserTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('admin_user', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('name',100)->comment('用户名');
$table->string('email',100)->comment('邮箱');
$table->string('password',100)->comment('密码');//密码字段必须用password
$table->timestamp('group')->comment('用户组')->nullable();//可以不为空
$table->timestamp('email_verified_at')->nullable();
$table->tinyInteger('status')->default(0)->comment('状态:0正常');//整型
$table->ipAddress('ip')->comment('ip地址');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('admin_user');
}
}
填充数据
php artisan make:seeder AdminUserTableSeeder
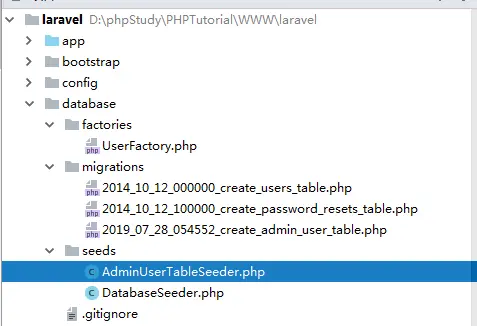
image.png
打开AdminUserTableSeeder.php
public function run()
{
$data = [
'name' => 'admin',
'email' => '1065628795@qq.com',
'password' => bcrypt('123456'),
'ip' => '127.0.0.1'
];
DB::table('admin_user')->insert($data);
}
在seeds/DatabaseSeeder.php注册一下
public function run()
{
// $this->call(UsersTableSeeder::class);
$this->call(AdminUserTableSeeder::class);
}
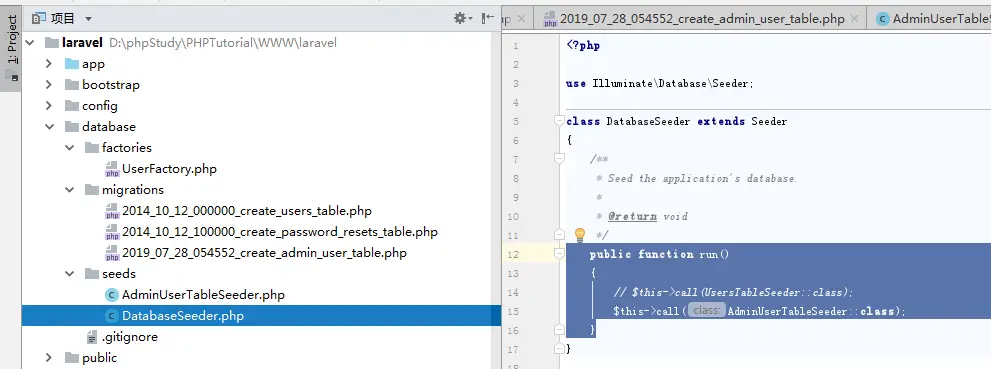
image.png
执行数据填充命令
php artisan db:seed
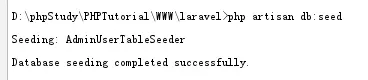
image.png

image.png
到这里数据库方面操作完成,接下来整理代码逻辑方面
路由
Route::get('/admin/login','Admin\LoginController@login');
Route::post('/admin/dologin','Admin\LoginController@dologin')->name('login.dologin');
登录代码
<form action="/admin/login/dologin" method="post">
{{ csrf_field() }}
<input type="text" placeholder="用户名" name="name"/>
<input type="password" placeholder="密码" name="password"/>
<button type="submit" >登录</button>
</form>
登录方法
<?php
namespace App\Http\Controllers\Admin;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Auth;
class LoginController extends Controller
{
//登录
public function login(){
return view('admin.login');
}
public function dologin(Request $request){
$res = Auth::attempt(['name' => $request->name,'password' => $request->password]); //自带验证,里面传验证字段
dd($res);die;
}
}
auth修改
'defaults' => [
// 'guard' => 'web',
'guard' => 'admin', //默认的改成admin
'passwords' => 'users',
],
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
/*新增*/
'admin' => [
'driver' => 'session',
'provider' => 'admin',
],
'api' => [
'driver' => 'token',
'provider' => 'users',
'hash' => false,
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\User::class,
],
// 新增
'admin' => [
'driver' => 'eloquent',
'model' => App\Admin::class,
],
// 'users' => [
// 'driver' => 'database',
// 'table' => 'users',
// ],
],
创建Admin模型
php artisan make:model Admin
修改文件
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use \Illuminate\Foundation\Auth\User;
class Admin extends User
{
//
public $table='admin_user';
}
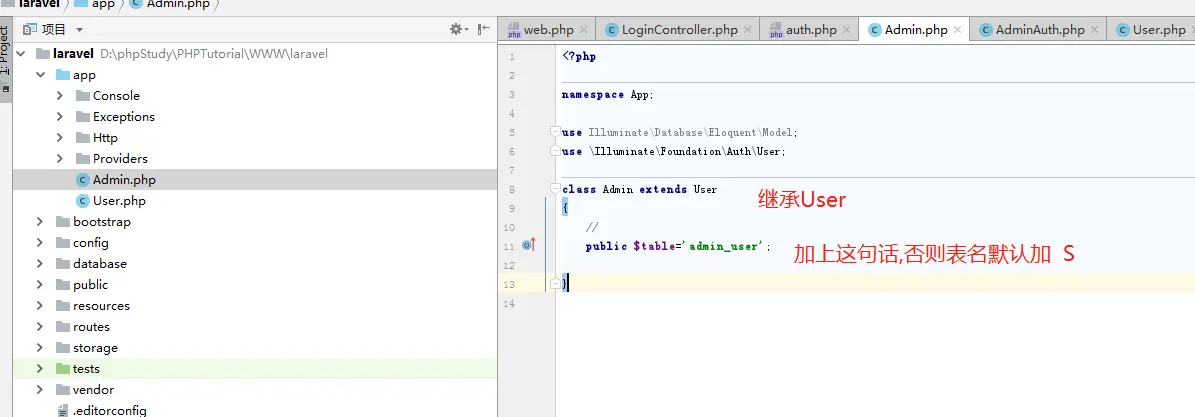
image.png