一:单向链表
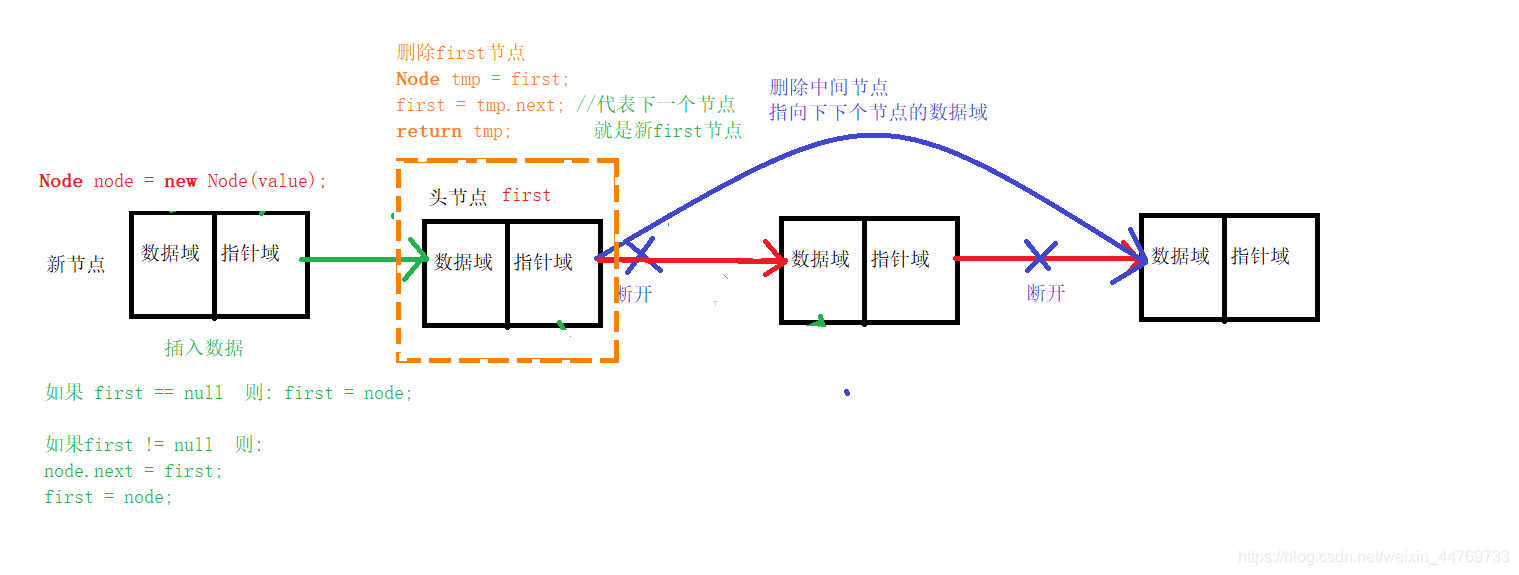
1.创建链表节点Node
public class Node {
long data;
Node next;
public Node(long value){
this.data = value;
}
public void display(){
System.out.println(data);
}
}
2.创建链表和操作链表
public class LinkList {
private Node first;
public LinkList(){
first = null;
}
public void insert(long value){
Node node = new Node(value);
if (first == null){
first = node;
}else{
node.next = first;
first = node;
}
}
public Node removeFirst(){
Node next = first.next;
Node tmp = first;
first = next;
return tmp;
}
public Node remove(){
Node tmp = first.next;
first.next = tmp.next;
return tmp;
}
public void show(){
Node current = first;
while (current != null){
current.display();
current = current.next;
}
}
public Node find(long value){
Node current = first;
while (current != null && current.data != value){
if (current.next == null){
return null;
}
current = current.next;
}
return current;
}
public Node delete(long value){
Node current = first;
Node pre = first;
while (current != null && current.data != value){
if (current.next == null){
return null;
}
pre = current;
current = current.next;
}
if (current == first){
first = first.next;
}else{
pre.next = current.next;
}
return current;
}
}
3.测试链表
public class Test {
public static void main(String[] args) {
LinkList linkList = new LinkList();
linkList.insert(1);
linkList.insert(6);
linkList.insert(8);
linkList.insert(0);
linkList.show();
Node first = linkList.removeFirst();
System.out.println("删除头节点: "+first.data);
linkList.show();
Node remove = linkList.remove();
System.out.println("删除中间节点: "+remove.data);
linkList.show();
Node node = linkList.find(0);
if (node != null){
System.out.println("找到指定节点");
node.display();
}else{
System.out.println("找不到节点:"+node);
}
Node delete = linkList.delete(0);
if (delete != null){
System.out.println("找到被删除节点");
delete.display();
}else{
System.out.println("找不到删除节点:"+delete);
}
linkList.show();
}
}