链表的增删
遍历打印链表:
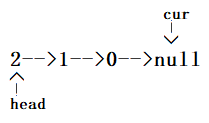
Node cur = head;
while(cur!=null){
System.out.println(cur.val);
cur=cur.next;
}
private static void print(Node head) {
System.out.println("打印链表:");
for (Node cur = head; cur != null; cur = cur.next) {
System.out.print(cur + " --> ");
}
System.out.println("null");
}
练习代码
class Node {
int val;
Node next;
Node(int val){
this.val=val;
this.next=null;
}
public String toString(){
return String.format("Node(%d)",val);
}
}
public class MyLinkedList {
public static void print(Node head){
System.out.println("打印链表:");
for (Node cur=head;cur!=null;cur=cur.next){
System.out.print(cur+"-->");
}
System.out.println("null");
}
private static Node pushFront(Node head,int val) {
Node node=new Node(val);
node.next=head;
return node;
}
private static Node pushBack(Node head, int val) {
Node node=new Node(val);
if(head==null){
return node;
}else{
Node last=head;
while(last.next != null){
last=last.next;
}
last.next=node;
return head;
}
}
private static void pushAfter(Node pos, int val) {
Node node=new Node(val);
node.next=pos.next;
pos.next=node;
}
private static Node popFront(Node head) {
if(head==null){
System.err.println("空链表无法删除");
return null;
}
return head.next;
}
private static Node popBack(Node head) {
if(head==null){
System.err.println("空链表无法删除");
return null;
}
if(head.next==null){
return null;
}else{
Node lastSecond=head;
while(lastSecond.next.next !=null){
lastSecond=lastSecond.next;
}
lastSecond.next=null;
return head;
}
}
private static void popAfter(Node pos) {
pos.next=pos.next.next;
}
public static void main(String[] args) {
Node head = null;
head = pushFront(head, 0);
head = pushFront(head, 1);
head = pushFront(head, 2);
print(head);
head = popFront(head);
print(head);
head = pushBack(head, 10);
head = pushBack(head, 20);
head = pushBack(head, 30);
print(head);
head = popBack(head);
head = popBack(head);
head = popBack(head);
head = popBack(head);
head = popBack(head);
head = popBack(head);
print(head);
head = pushBack(head, 100);
print(head);
}
}