一、
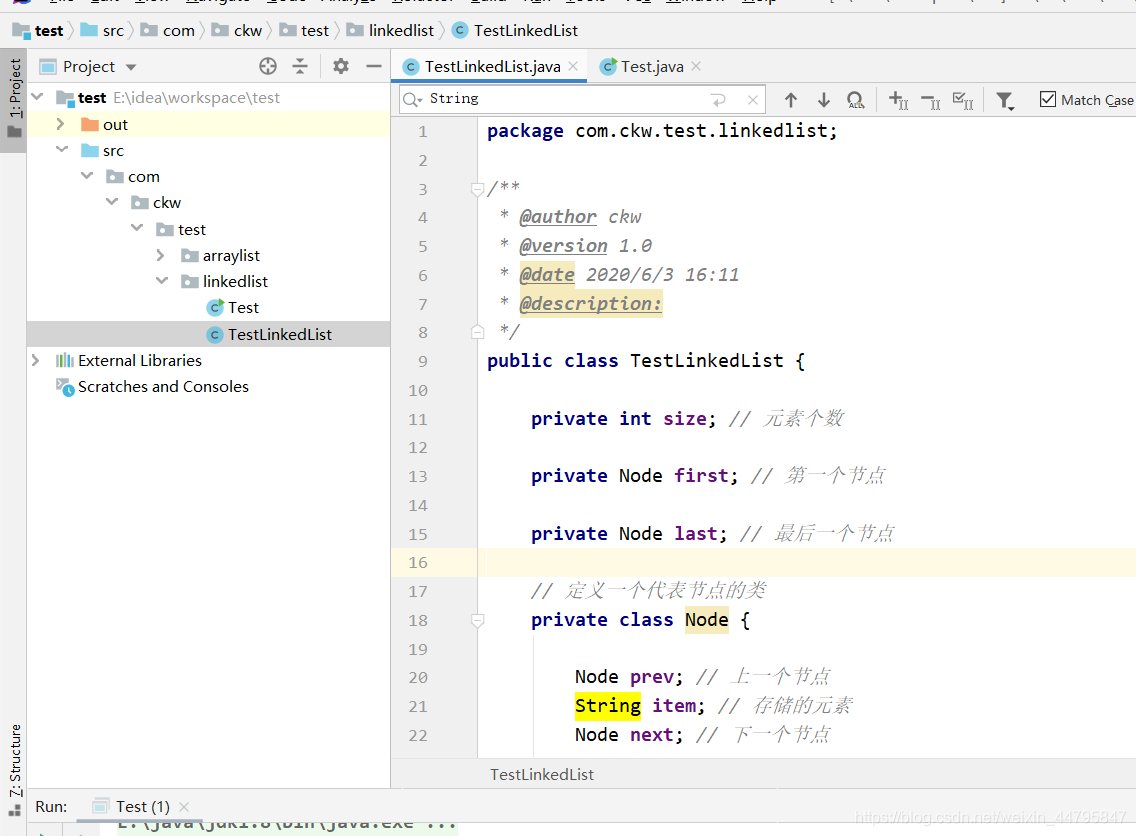
二、
package com.ckw.test.linkedlist;
/**
* @author ckw
* @version 1.0
* @date 2020/6/3 16:11
* @description:
*/
public class TestLinkedList {
private int size; // 元素个数
private Node first; // 第一个节点
private Node last; // 最后一个节点
// 定义一个代表节点的类
private class Node {
Node prev; // 上一个节点
String item; // 存储的元素
Node next; // 下一个节点
public Node(Node prev, String item, Node next) {
this.prev = prev;
this.item = item;
this.next = next;
}
}
public void add(String str) {
// 创建一个节点存储这个元素
Node node = new Node(this.last, str, null);
// 判断是否是第一个节点
if (size == 0) {
// 由于是第一个节点,所以头节点指向这个节点
this.first = node;
} else {
// 原来的尾节点的下一位指向新节点
this.last.next = node;
}
// 新节点现在是链表的最后一个节点
this.last = node;
size++;
}
private Node getNode(int index) {
Node no = this.first;
for (int i = 1; i <= index; i++) {
no = no.next;
}
return no;
}
public void add(int index, String str) {
// 判断下标越界
if (index > size || index < 0)
throw new IndexOutOfBoundsException("Index:" + index + ", Size:" + size);
// 表示在尾部插入
if (index == size) {
add(str);
return;
}
Node no = new Node(null, str, null);
// 判断是否是头节点
if (index == 0) {
// 新节点的下一位指向原来的头节点
no.next = this.first;
// 原来的头节点的上一位指向新节点
this.first.prev = no;
// 将头节点挪到新节点上
this.first = no;
} else {
Node node = getNode(index);
// 原节点的上一个节点的下一位指向新节点
node.prev.next = no;
// 新节点的上一位指向原节点的上一个节点
no.prev = node.prev;
// 新节点的下一个节点指向原节点
no.next = node;
// 原节点的上一位指向新节点
node.prev = no;
}
size++;
}
//判断是否下标越界
private void out(int index) {
if (index >= size || index < 0)
throw new IndexOutOfBoundsException("Index:" + index + ", Size:" + size);
}
public void remove(int index) {
this.out(index);
// 判断是否只有一个节点,如果只有一个节点,清空集合
if (size == 1) {
this.first = null;
this.last = null;
size--;
return;
}
// 判断头节点
if (index == 0) {
// 将头节点挪到下一位上
this.first = this.first.next;
// 新的头节点的上一位置为null
this.first.prev = null;
} /* 判断尾节点 */ else if (index == size - 1) {
// 将尾节点挪到上一个节点上
this.last = this.last.prev;
// 新的尾节点的下一位置为null
this.last.next = null;
} else {
Node node = this.getNode(index);
// 原节点的上一位的下一个节点指向原节点的下一位
node.prev.next = node.next;
// 原节点的下一位的上一个节点指向原节点的上一位
node.next.prev = node.prev;
}
size--;
}
public int indexOf(String str) {
Node node = this.first;
for (int i = 1; i < size; i++) {
String item = node.item;
if (str != null || str == item && str.equals(item)) {
return i;
}
node = node.next;
}
return -1;
}
//移除元素
public void remove(String str) {
int index = indexOf(str);
if (index != -1)
this.remove(index);
}
//根据索引位置设置元素
public void set(int index, String str) {
this.out(index);
Node node = this.getNode(index);
node.item = str;
}
public String get(int index) {
this.out(index);
return this.getNode(index).item;
}
public boolean contains(String str) {
return this.indexOf(str) != -1;
}
// 提供翻转列表的操作
public TestLinkedList reverse() {
TestLinkedList list = new TestLinkedList();
Node node = this.last;
for (int i = size - 1; i > 0; i--) {
list.add(node.item);
node = node.prev;
}
return list;
}
public String toString() {
StringBuilder sb = new StringBuilder("[");
Node node = this.first;
for (int i = 0; i < size; i++) {
sb.append(node.item).append(", ");
node = node.next;
}
String str = sb.toString();
if (size != 0)
str = str.substring(0, str.length() - 2);
return str += "]";
}
}