二叉树的锯齿形层次遍历
给定一个二叉树,返回其节点值的锯齿形层次遍历。(即先从左往右,再从右往左进行下一层遍历,以此类推,层与层之间交替进行)。
例如:
给定二叉树 [3,9,20,null,null,15,7]
,
3
/ \
9 20
/ \
15 7
返回锯齿形层次遍历如下:
[ [3], [20,9], [15,7] ]
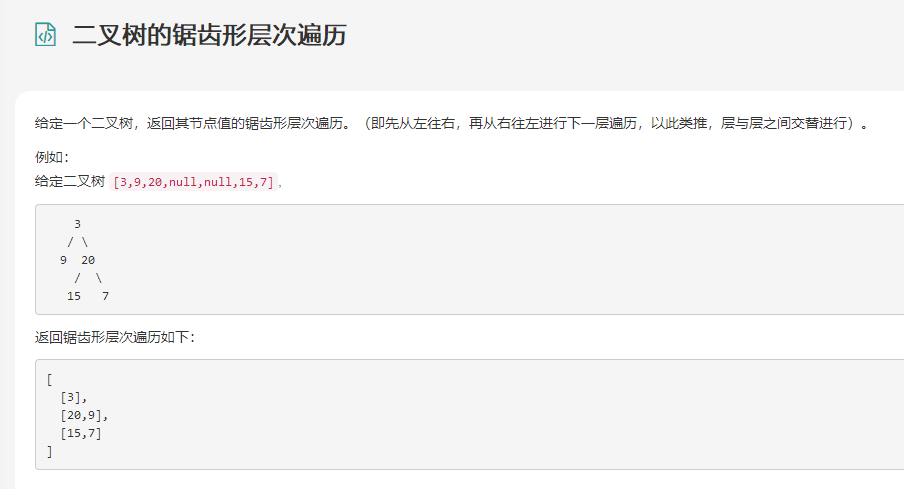
我的想法是把树的每一层存起来,然后 技术层次的结点顺序翻转一下
1 # Definition for a binary tree node.
2 # class TreeNode:
3 # def __init__(self, x):
4 # self.val = x
5 # self.left = None
6 # self.right = None
7
8 class Solution:
9 def zigzagLevelOrder(self, root):
10 """
11 :type root: TreeNode
12 :rtype: List[List[int]]
13 """
14 if root is None:
15 return []
16 res = [[root]]
17 res_int = [[root.val]]
18 i = 0
19 while i < len(res):
20 cur = res[i]
21 nLayer = []
22 nLyayer_int = []
23 for n in cur:
24 if n.left is not None:
25 nLayer.append(n.left)
26 nLyayer_int.append(n.left.val)
27 if n.right is not None:
28 nLayer.append(n.right)
29 nLyayer_int.append(n.right.val)
30 if nLayer:
31 res.append(nLayer)
32 if i % 2 == 0:
33 nLyayer_int.reverse()
34 res_int.append(nLyayer_int)
35
36 i += 1
37
38 return res_int