一、例题1
请使用Pandas工具库对数据集“data1.xls”进行相关的数据处理。
数据集“data1.xls”介绍:
数据集共有7列数据,7列数据分别代表的是:贷款号、账户号、发放贷款日期、贷款金额、每月归还款、还贷状态。
题目要求:
① 导入必要的库。(6分)
② 读入数据集。(6分)
③ 按“发放贷款日期”降序,“贷款金额”升序打印输出,新增一列“每月归还额= 贷款金额/贷款期限”。(8分)
④ 输出账户号在3000-5000之间的数据。(8分)
⑤ 删除账户号列,增加每月还款额列(每月还款额列=贷款金额/贷款期限)。(8分)
⑥ 对还款状态进行计数。(8分)
⑦ 按照还款状态进行分组,计算贷款金额的最大值。(8分)
⑧ 输出每月还款额的最大值与中位数。(8分)
代码实现
① 导入必要的库。(6分)
import pandas as pd
# ② 读入数据集。(6分)
df = pd.read_excel('data1.xls')
# ③ 按“发放贷款日期”降序,“贷款金额”升序打印输出,新增一列“每月归还额= 贷款金额/贷款期限”。(8分)
df.sort_values(['发放贷款日期','贷款金额'],ascending=[False,True],inplace=True)
df['每月归还额'] = df['贷款金额'] / df['贷款期限']
# ④ 输出账户号在3000-5000之间的数据。(8分)
print( df[(df['账户号']>3000)&(df['账户号']>5000)] )
# ⑤ 删除账户号列,增加每月还款额列(每月还款额列=贷款金额/贷款期限)。(8分)
del df['账户号']
# df.drop(columns=['账户号'],inplace=True)
df['每月还款额'] = df['贷款金额'] / df['贷款期限']
# ⑥ 对还款状态进行计数。(8分)
print(df['还款状态'].value_counts())
# ⑦ 按照还款状态进行分组,计算贷款金额的最大值。(8分)
print(df[['还款状态','贷款金额']].groupby('还款状态').max())
# ⑧ 输出每月还款额的最大值与中位数。(8分)
a = df['每月归还额']
print('最大值=',a.max())
print('中位数=',a.median())
输出结果
贷款号 账户号 发放贷款日期 贷款金额 贷款期限 每月归还额 还款状态
18 6876 9236 1993-12-21 86616 12 7218.0 A
17 6820 9034 1993-12-16 38148 12 3179.0 A
16 7104 10320 1993-12-13 259740 60 4329.0 A
15 6456 7123 1993-12-09 47016 12 3918.0 A
13 6356 6701 1993-12-08 95400 36 2650.0 A
12 6228 6034 1993-12-01 464520 60 7742.0 B
11 6077 5270 1993-11-22 79608 24 3317.0 A
10 7121 10364 1993-11-10 21924 36 609.0 A
8 7235 10973 1993-10-13 154416 48 3217.0 A
7 6111 5428 1993-09-24 174744 24 7281.0 B
6 7284 11265 1993-09-15 52788 12 4399.0 A
5 6687 8261 1993-09-13 87840 24 3660.0 A
4 7240 11013 1993-09-06 274740 60 4579.0 A
2 6863 9188 1993-07-28 127080 60 2118.0 A
A 16
B 3
Name: 还款状态, dtype: int64
贷款金额
还款状态
A 274740
B 464520
最大值= 8033.0
中位数= 3918.0
例1数据集
贷款号 账户号 发放贷款日期 贷款金额 贷款期限 每月归还额 还款状态
5314 1787 1993-07-05 96396 12 8033 B
5316 1801 1993-07-11 165960 36 4610 A
6863 9188 1993-07-28 127080 60 2118 A
5325 1843 1993-08-03 105804 36 2939 A
7240 11013 1993-09-06 274740 60 4579 A
6687 8261 1993-09-13 87840 24 3660 A
7284 11265 1993-09-15 52788 12 4399 A
6111 5428 1993-09-24 174744 24 7281 B
7235 10973 1993-10-13 154416 48 3217 A
5997 4894 1993-11-04 117024 24 4876 A
7121 10364 1993-11-10 21924 36 609 A
6077 5270 1993-11-22 79608 24 3317 A
6228 6034 1993-12-01 464520 60 7742 B
6356 6701 1993-12-08 95400 36 2650 A
5523 2705 1993-12-08 93888 36 2608 A
6456 7123 1993-12-09 47016 12 3918 A
7104 10320 1993-12-13 259740 60 4329 A
6820 9034 1993-12-16 38148 12 3179 A
6876 9236 1993-12-21 86616 12 7218 A
二、例题2
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sn
# 正常显示中文
plt.rcParams['font.sans-serif'] = ['SimHei']
# 正常显示负数
plt.rcParams['axes.unicode_minus'] = False
#读取数据
df = pd.read_excel('ftx.xlsx')
#把日期 '年'改为 ‘季度’
def fn(n):
if n>0 and n<4:
return 's1'
elif n>3 and n<7:
return 's2'
elif n>6 and n<10:
return 's3'
else:
return 's4'
# 划分季度
df['季度'] = df['月'].map(fn)
# 1.使用所学知识,使用EXCEL数据透视表功能,
# 从数据源中筛选出2010年第3季度北京和上海两个城市的销售数量总和,将结果输出到新表a。
a = df[ df['销售区域'].isin(['北京','上海']) & (df['年']==2010) & (df['季度']=='s3') ] [['销售区域','销售数量']].groupby(['销售区域']).sum()
a.to_csv("a.csv")
# 2.利用python环境下的数据分析包pandas编程实现筛选出
#2010年第3季度北京和广州两个城市的销售数量总和,将结果输出到新表b。
b = df[ df['销售区域'].isin(['北京','广州']) & (df['年']==2010) & (df['季度']=='s3') ] [['销售区域','销售数量']].groupby(['销售区域']).sum()
b.to_csv("b.csv")
# 3.利用python环境下的数据分析包pandas编程实现
# 求出2009年和2010年的四个季度(第一季度、第二季度、第三季度和第四季度)共8个时间节点,
# 求出各节点对应销售数量总和,并用柱状图可视化展现。
df['季度']=df['年'].astype(str)+df['季度']
df = df[['季度','销售数量']].groupby(['季度']).sum()
sn.barplot(df.index,df['销售数量'])
plt.show()
结果展示
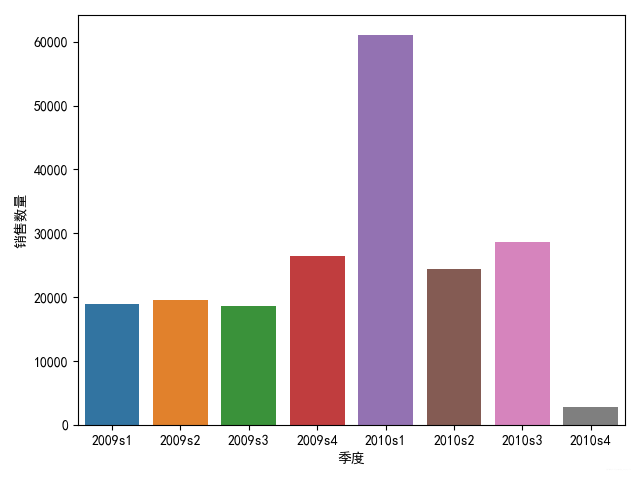
例2数据集样式(数据过多没有展示,可自己编写数据集)
年 月 销售区域 销售数量
2009 1 广州 70
2009 1 南宁 25
2009 1 北京 17
2009 1 广州 99
2009 1 北京 43
2009 1 广州 57
2009 1 上海 47
2009 1 南宁 73
2009 1 广州 32
2009 1 北京 77
2009 1 广州 41
2009 1 上海 12
2009 1 北京 18
2009 1 杭州 13
2009 1 杭州 87
2009 1 南昌 17
2009 1 广州 76
2009 1 杭州 45
2009 1 沈阳 81