Quartz是Job scheduling(作业调度)领域的一个开源项目,Quartz既可以单独使用也可以跟spring框架整合使用,在实际开发中一般会使用后者。使用Quartz可以开发一个或者多个定时任务,每个定时任务可以单独指定执行的时间,例如每隔1小时执行一次、每个月第一天上午10点执行一次、每个月最后一天下午5点执行一次等。
官网:http://www.quartz-scheduler.org/
maven坐标:
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz</artifactId>
<version>2.2.1</version>
</dependency>
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz-jobs</artifactId>
<version>2.2.1</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>5.0.5.RELEASE</version>
</dependency>
定义一个定时任务类(这里做的是七牛云图片的定时删除)
package com.gzy.health.jobs;
import com.gzy.health.common.RedisConst;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import redis.clients.jedis.JedisPool;
import java.util.Iterator;
import java.util.Set;
/**
* @author :gzy
* @date :Created in 2019/6/10
* @description :任务调度类
* @version: 1.0
*/
@Service
public class ClearImageJob {
@Autowired
private JedisPool jedisPool;
/**
* 定义清理图片的任务
*/
public void clearImageJob(){
try{
// 获取两个数据差集
Set<String> set = jedisPool.getResource().sdiff(RedisConst.SETMEAL_PIC_RESOURCES,RedisConst.SETMEAL_PIC_DB_RESOURCES);
if (set == null){
System.out.println("clearImageJob......set is null");
return;
}
Iterator<String> iterator = set.iterator();
while (iterator.hasNext()){
String picName = iterator.next();
QiniuUtils.deleteFileFromQiniu(picName);
jedisPool.getResource().srem(RedisConst.SETMEAL_PIC_RESOURCES,picName);
System.out.println("clearImageJob......del:"+picName);
}
}catch(Exception e){
e.printStackTrace();
}
}
}
配置文件spring-jobs.xml,配置自定义Job、任务描述、触发器、调度工厂等
- 自动扫包
- 注册任务对象
- 注册JobDetail
- 触发器
- 调度工厂
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!--自动扫包,后续注入jedis到任务调度类-->
<context:component-scan base-package="com.gzy.health.jobs"/>
<!--注册一个定义任务对象-->
<bean id = "clearImgJob" class="com.gzy.health.jobs.ClearImageJob"/>
<!-- 注册JobDetail,作用是负责通过反射调用指定的Job -->
<bean id="clearImgJobDetail" class="org.springframework.scheduling.quartz.MethodInvokingJobDetailFactoryBean">
<!--注入对象-->
<property name="targetObject" ref="clearImgJob"/>
<!--注入方法-->
<property name="targetMethod" value="clearImageJob"/>
</bean>
<!--注册一个触发器,指定任务触发的时间(间隔)-->
<bean id="jobTrigger" class="org.springframework.scheduling.quartz.CronTriggerFactoryBean">
<property name="jobDetail" ref="clearImgJobDetail"/>
<property name="cronExpression">
<!-- 每隔10秒执行一次任务 0/10 * * * * ? -->
<!-- 每隔2分钟执行一次任务 0 0/2 * * * ? -->
<!--每天凌晨2点执行一次任务 0 0 2 * * ? -->
<value>0/10 * * * * ?</value>
</property>
</bean>
<!--注册一个统一调用工厂,通过这个调度工厂调度任务-->
<bean id="scheduler" class="org.springframework.scheduling.quartz.SchedulerFactoryBean">
<property name="triggers">
<list>
<ref bean="jobTrigger"/>
</list>
</property>
</bean>
</beans>
修改web.xml,容器启动时,加载spring配置文件
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<display-name>Archetype Created Web Application</display-name>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-*.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app>
cron表达式介绍
这种表达式称为cron表达式,通过cron表达式可以灵活的定义出符合要求的程序执行的时间。
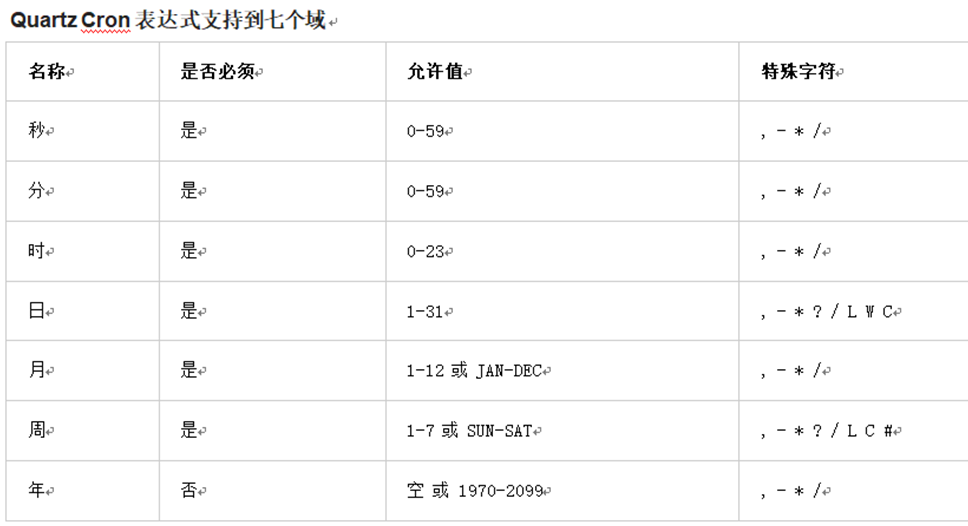
cron表达式分为七个域,之间使用空格分隔。其中最后一个域(年)可以为空。每个域都有自己允许的值和一些特殊字符构成。使用这些特殊字符可以使我们定义的表达式更加灵活。
下面是对这些特殊字符的介绍:
逗号(,):指定一个值列表,例如使用在月域上1,4,5,7表示1月、4月、5月和7月
横杠(-):指定一个范围,例如在时域上3-6表示3点到6点(即3点、4点、5点、6点)
星号(*):表示这个域上包含所有合法的值。例如,在月份域上使用星号意味着每个月都会触发
斜线(/):表示递增,例如使用在秒域上0/15表示每15秒
问号(?):只能用在日和周域上,但是不能在这两个域上同时使用。表示不指定
井号(#):只能使用在周域上,用于指定月份中的第几周的哪一天,例如6#3,意思是某月的第三个周五 (6=星期五,3意味着月份中的第三周)
L:某域上允许的最后一个值。只能使用在日和周域上。当用在日域上,表示的是在月域上指定的月份的最后一天。用于周域上时,表示周的最后一天,就是星期六
W:W 字符代表着工作日 (星期一到星期五),只能用在日域上,它用来指定离指定日的最近的一个工作日
cron表达式在线生成器
可以借助一些cron表达式在线生成器来根据我们的需求生成表达式即可。
http://www.bejson.com/othertools/cron/
附:七牛云删除工具类
package com.gzy.health.jobs;
/**
* @author :gzy
* @date :Created in 2019/6/10
* @description :
* @version: 1.0
*/
import com.qiniu.common.QiniuException;
import com.qiniu.common.Zone;
import com.qiniu.http.Response;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.Configuration;
import com.qiniu.storage.UploadManager;
import com.qiniu.util.Auth;
import java.io.FileInputStream;
import java.util.UUID;
/**
* 七牛云工具类
*/
public class QiniuUtils {
public static String accessKey = "wz3pfgxFmBhU-Ao7-kAXCPNmojAl9BkN2c5HgUun";
public static String secretKey = "74ARWyFtV6fzDPWAS4TDI0UPASNYFvCD5rXMPTMa";
public static String bucket = "gzy_health";
/**
* 删除文件
* @param fileName 服务端文件名
*/
public static void deleteFileFromQiniu(String fileName){
//构造一个带指定Zone对象的配置类
Configuration cfg = new Configuration(Zone.zone0());
String key = fileName;
Auth auth = Auth.create(accessKey, secretKey);
BucketManager bucketManager = new BucketManager(auth, cfg);
try {
bucketManager.delete(bucket, key);
} catch (QiniuException ex) {
//如果遇到异常,说明删除失败
System.err.println(ex.code());
System.err.println(ex.response.toString());
}
}
}