狂神说Mybatis视频链接:
Mybatis官方文档:
MyBatis
1、简介
1.1 什么是Mybatis
- MyBatis 是一款优秀的持久层框架; 持久层即dao层
- 它支持自定义 SQL、存储过程以及高级映射。MyBatis 免除了几乎所有的 JDBC 代码以及设置参数和获取结果集的工作。MyBatis可以通过简单的 XML或注解,来配置和映射原始类型、接口和 Java POJO(Plain Old Java Objects,普通老式 Java 对象)为数据库中的记录。
1.2 持久化
数据持久化
- 持久化就是将程序的数据在持久状态和瞬时状态转化的过程
- 内存:断电即失
- 数据库(Jdbc),io文件持久化。
为什么要持久化?
有一些对象,不能让他丢掉
内存太贵
1.3 持久层
Dao层、Service层、Controller层,持久层即Dao层
- 完成持久化工作的代码块
- 层界限十分明显
1.4 为什么需要MyBatis
-
帮助程序员将数据存入到数据库中
-
方便
-
传统的JDBC代码太复杂了,简化,框架,自动化
-
不用MyBatis也可以,技术没有高低之分
-
优点:
- 简单易学
- 灵活
- sql和代码的分离,提高了可维护性。
- 提供映射标签,支持对象与数据库的orm字段关系映射
- 提供对象关系映射标签,支持对象关系组建维护
- 提供xml标签,支持编写动态sql
2、第一个Mybatis程序
思路:搭建环境 --> 导入MyBatis --> 编写代码 --> 测试
2.1 搭建环境
新建项目
1.创建一个普通的maven项目
2.删除src目录 (就可以把此工程当做父工程了,然后创建子工程)
3.导入maven依赖
<!--导入依赖-->
<dependencies>
<!--mysqlq驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.12</version>
</dependency>
<!--mybatis-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.4</version>
</dependency>
<!--junit-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
4.创建一个Module
2.2 创建一个模块
- 编写mybatis的核心配置文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<!--configuration核心配置文件-->
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
//最好在URL中设置时区,要不idea也会提示设置时区
<property name="url" value="jdbc:mysql://localhost:3306/mybatis?userSSL=true&useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<!--每一个Mapper.xml都需要在mybatis核心配置文件中注册!-->
<mappers>
<mapper resource="com/yff/dao/UserMapper.xml"/>
</mappers>
</configuration>
注意点:驱动driver:com.mysql.jdbc.Driver和com.mysql.cj.jdbc.Driver的区别
com.mysql.jdbc.Driver 是 mysql-connector-java 5中的,
com.mysql.cj.jdbc.Driver 是 mysql-connector-java 6中的
详见博客:com.mysql.jdbc.Driver 和 com.mysql.cj.jdbc.Driver的区别 serverTimezone设定 - 山高我为峰 - 博客园,目前springboot 2.37默认mysql-connector-java是8.0.22的
- 编写mybatis工具类(目的获取SqlSession对象:既然有了 SqlSessionFactory,顾名思义,我们可以从中获得 SqlSession 的实例。SqlSession 提供了在数据库执行 SQL 命令所需的所有方法。你可以通过 SqlSession 实例来直接执行已映射的 SQL 语句。)
//sqlSessionFactory --> sqlSession
public class MybatisUtils {
static SqlSessionFactory sqlSessionFactory = null;
static {
try {
//使用Mybatis第一步 :获取sqlSessionFactory对象
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
//既然有了 SqlSessionFactory,顾名思义,我们可以从中获得 SqlSession 的实例.
// SqlSession 提供了在数据库执行 SQL 命令所需的所有方法。SqlSession相当于jdbc中的statement和PreparedStatement
public static SqlSession getSqlSession(){
return sqlSessionFactory.openSession(); //sqlSessionFactory.openSession(true):开启自动提交事务,不用commit了,mybatis默认未开启自动提交事务
}
}
2.3 编写代码
-
实体类
package com.yff.pojo;
public class User {
private int id;
private String name;
private String pwd;
public User(int id, String name, String pwd) {
this.id = id;
this.name = name;
this.pwd = pwd;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public User() {
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", pwd='" + pwd + '\'' +
'}';
}
}
-
Dao接口
public interface UserDao {
public List<User> getUserList();
}
- 接口实现类 (由原来的UserDaoImpl转变为一个Mapper配置文件,之前是应该写UserDao的实现类了)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!--namespace=绑定一个指定的Dao/Mapper接口;id绑定实现的接口的方法;resultType绑定返回的类型(泛型,指List<User>中的User,且需要全路径(编译后target中classes下的全路径))。
全限定名(比如 “com.mypackage.MyMapper.selectAllThings)将被直接用于查找及使用。这里尽量使用全限定名。
-->
<mapper namespace="com.kuang.dao.UserDao">
<select id="getUserList" resultType="com.kuang.pojo.User">
select * from USER
</select>
</mapper>
- 使用junit测试(1、测试代码最好和工程代码层级一致 2、执行sql的方式一是重点,方式二已基本不用)
代码:
package com.yff.dao;
import com.yff.pojo.User;
import com.yff.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
import java.util.List;
public class UserDaoTest {
@Test
public void test(){
SqlSession sqlSession = null;
try {
//第一步 获取sqlsession对象
sqlSession = MybatisUtils.getSqlSession();
//第二步: 方式1: getmMpper执行sql
UserDao userdao = sqlSession.getMapper(UserDao.class);
List<User> userList = userdao.getUserList();
//第二步: 方式2: 指定执行sql
// List<User> userList = sqlSession.selectList("com.yff.dao.UserDao.getUserList");
for (User user : userList) {
System.out.println(user);
}
}catch (Exception e){
e.printStackTrace();
}finally {
//关闭sqlsession
sqlSession.close();
}
}
}
结果:
可能会遇到的执行问题:
1.配置文件没有注册
报错:
org.apache.ibatis.binding.BindingException: Type interface com.kuang.dao.UserDao is not known to the MapperRegistry.
MapperRegistry是什么?
核心配置文件中注册mappers
2.绑定接口错误
3.方法名不对
4.返回类型不对
5.Maven导出资源问题
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
3、CURD和万能map、模糊查询
3.1. namespace
namespace中的包名要和Dao/Mapper接口的包名一致,需要全限定名
3.2. select
1:如果只是查询一个字段,用String类型就可以,resultType="String";
2:如果是多个字段,可以用相关的类名作为返回类型,例如 你的monitor_entity表对应的实体类为Monitor,
就可以这样写:resultType="Monitor";
3:解决实体类属性名和数据库字段名不一致的问题用resultMap
3.3. Insert
MySQL自增ID的场景下推荐在 Mybatis 中使用 useGeneratedKeys:
使用useGeneratedKeys="true" keyProperty="userId"可以在user对象中返回userId,
<insert id="insertUser" parameterType="SysUser" useGeneratedKeys="true" keyProperty="userId">
insert into sys_user(
<if test="userId != null and userId != 0">user_id,</if>
<if test="deptId != null and deptId != 0">dept_id,</if>
<if test="userName != null and userName != ''">user_name,</if>
<if test="nickName != null and nickName != ''">nick_name,</if>
<if test="email != null and email != ''">email,</if>
<if test="avatar != null and avatar != ''">avatar,</if>
<if test="phonenumber != null and phonenumber != ''">phonenumber,</if>
<if test="sex != null and sex != ''">sex,</if>
<if test="password != null and password != ''">password,</if>
<if test="status != null and status != ''">status,</if>
<if test="createBy != null and createBy != ''">create_by,</if>
<if test="remark != null and remark != ''">remark,</if>
<if test="shipIMONo != null and shipIMONo != ''">ship_imo_no,</if>
create_time
)values(
<if test="userId != null and userId != ''">#{userId},</if>
<if test="deptId != null and deptId != ''">#{deptId},</if>
<if test="userName != null and userName != ''">#{userName},</if>
<if test="nickName != null and nickName != ''">#{nickName},</if>
<if test="email != null and email != ''">#{email},</if>
<if test="avatar != null and avatar != ''">#{avatar},</if>
<if test="phonenumber != null and phonenumber != ''">#{phonenumber},</if>
<if test="sex != null and sex != ''">#{sex},</if>
<if test="password != null and password != ''">#{password},</if>
<if test="status != null and status != ''">#{status},</if>
<if test="createBy != null and createBy != ''">#{createBy},</if>
<if test="remark != null and remark != ''">#{remark},</if>
<if test="shipIMONo != null and shipIMONo != ''">#{shipIMONo},</if>
sysdate()
)
</insert>
useGeneratedKeys的详解_小草dym的博客-CSDN博客_usegeneratedkeys
3.4. update
3.5. Delete
-
id:就是对应的namespace中的方法名;
-
resultType : Sql语句执行的返回值;
-
parameterType : 参数类型;
1.编写接口
package com.yff.dao;
import com.yff.pojo.User;
import java.util.List;
public interface UserMapper {
//获取用户列表
List<User> getUserList();
//查询指定用户
User getUser(int id);
//新增用户
int insertUser(User user);
//修改用户
int updateUser(User user);
//删除用户
int deleteUser(int id);
}
2.编写对应的mapper中的sql语句
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!--命名空间:namespace:绑定一个对应的Dao/Mapper接口-->
<mapper namespace="com.yff.dao.UserMapper">
<select id="getUserList" resultType="com.yff.pojo.User">
select * from user
</select>
<select id="getUser" resultType="com.yff.pojo.User" parameterType="int">
select * from user where id=#{id}
</select>
<!--可以通过#{}直接取到对象中的属性-->
<update id="updateUser" parameterType="com.yff.pojo.User">
update user set name = #{name},pwd=#{pwd} where id=#{id}
</update>
<insert id="insertUser" parameterType="com.yff.pojo.User">
insert into user values(#{id},#{name},#{pwd});
</insert>
<delete id="deleteUser" parameterType="int" >
delete from user where id = #{id}
</delete>
</mapper>
3.测试
package com.yff.dao;
import com.yff.pojo.User;
import com.yff.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
import java.util.List;
public class UserMapperTest {
@Test
public void test(){
//第一步获取sqlsession对象
SqlSession sqlSession = null;
try {
sqlSession = MybatisUtils.getSqlSession();
//第二步: 方式1: getmMpper执行sql
UserMapper userdao = sqlSession.getMapper(UserMapper.class);
List<User> userList = userdao.getUserList();
//第二步: 方式2: 指定执行sql
// List<User> userList = sqlSession.selectList("com.yff.dao.UserDao.getUserList");
for (User user : userList) {
System.out.println(user);
}
}catch (Exception e){
e.printStackTrace();
}finally {
//关闭sqlsession
sqlSession.close();
}
}
@Test
public void getUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User user = mapper.getUser(1);
System.out.println(user);
sqlSession.close();
}
@Test
public void updateUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int i = mapper.updateUser(new User(1, "test", "12345"));
if (i>0){
System.out.println("更新ok");
}
sqlSession.commit();
sqlSession.close();
}
@Test
public void insertUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int i = mapper.insertUser(new User(9, "liu", "8888"));
if (i > 0) {
System.out.println("插入ok");
}
sqlSession.commit();
sqlSession.close();
}
@Test
public void deleteUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int i = mapper.deleteUser(3);
if (i > 0) {
System.out.println("删除ok");
}
sqlSession.commit();
sqlSession.close();
}
}
注意:增删改查一定要提交事务(mybatis默认未开启自动提交事务):
sqlSession.commit();
3.6. 万能Map(用作接口传参)
假设,我们的实体类,或者数据库中的表,字段或者参数过多,我们应该考虑使用Map!
原因如下:
1、如果需要数据库字段过多时,使用实体类对象传参的话,要不需要多写组合各种参数的几个构造方法,要不就需要把全部参数传进去,因为一般实体类构造方法中只有无参和所有参数的,所以这个时候直接使用map会省事很多。(其实new的时候传全部的参数也是可以,不会报错的,因为#{}中只获取自己用到的参数,但是尽量使用map,更便利)
----------------------------------------
int insertUser(User user);
----------------------------------------
<insert id="insertUser" parameterType="com.yff.pojo.User">
insert into user(id,pwd) values(#{id},#{pwd});
</insert>
----------------------------------------
//传全部参数,因为构造方式只有无参和全部参数,这样不会报错,#{}只会取用到的
@Test
public void insertUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int i = mapper.insertUser(new User(99, "meiren","8888"));
if (i > 0) {
System.out.println("插入ok");
}
sqlSession.commit();
sqlSession.close();
}
--------------------------------------------
//传部分参数,new User时会报错没有构造方法,需要补充构造方法
@Test
public void insertUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int i = mapper.insertUser(new User(100, "8888")); //new User(100, "8888")报错,需要补充构造方法
if (i > 0) {
System.out.println("插入ok");
}
sqlSession.commit();
sqlSession.close();
}
2、两个参数在查询结果中并不需要,但是如果使用的一个javaBean对象作为selecetAll的条件参数,如果javaBean中没有这两个参数对应的属性或get方法,在调用这个selecetAll方法时就会报找不到其对应的属性和get方法,如果是使用Map则不存在此问题
1.UserMapper接口
//用万能Map插入用户
int insertUserMap(Map<String,Object> map);
2.UserMapper.xml
<!--对象中的属性可以直接取出来 传递map的key-->
<insert id="insertUserMap" parameterType="map">
insert into user(id,name) values(#{idyff},#{nameyff});
</insert>
3.测试
@Test
public void testMap(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
Map<String, Object> map = new HashMap<String,Object>();
map.put("idyff",10);
map.put("nameyff","吴彦祖");
int i = mapper.insertUserMap(map);
if (i > 0) {
System.out.println("插入成功");
}
sqlSession.commit();
sqlSession.close();
}
3.7. 通过实体传参(用作接口传参)
<sql id="selectConfigVo">
select config_id, config_name, config_key, config_value, config_type, create_by, create_time, update_by, update_time, remark
from sys_config
</sql>
<!-- 查询条件 -->
<sql id="sqlwhereSearch">
<where>
<if test="configId !=null">
and config_id = #{configId}
</if>
<if test="configKey !=null and configKey != ''">
and config_key = #{configKey}
</if>
</where>
</sql>
<select id="selectConfig" parameterType="SysConfig" resultMap="SysConfigResult">
<include refid="selectConfigVo"/>
<include refid="sqlwhereSearch"/>
</select>
SysConfig是实体类
service中的用法:
@Override
public SysConfig selectConfigById(Long configId)
{
SysConfig config = new SysConfig();
config.setConfigId(configId); (set查询条件的字段)
return configMapper.selectConfig(config); (重点)
}
(service层到mapper.xml)小结:
Map传递参数,直接在sql中取出key即可! 【parameterType=“map”】
对象传递参数,直接在sql中取出对象的属性即可! 【parameterType=“Object”】
只有一个基本类型参数的情况下,可以直接在sql中取到 【parameterType="_int"】
多个参数尽量用Map , 或者注解!
万能map是一个办法,实际工作中通过bean入参也用的很多。
(以上是service到mapper.xml层的传递参数)
备注:前端到controller解析的参数传递,详见自己博客:
springMVC_狂神_02_配置版和注解版编码、restful风格、数据跳转、Json处理(重点)_keep one's resolveY的博客-CSDN博客
3.8. 模糊查询
模糊查询这么写?
1.Java代码执行的时候,传递通配符% %
List<User> userList = mapper.getUserLike("%李%");
2.在sql拼接中使用通配符
select * from user where name like "%"#{value}"%"
解决MyBatis中模糊搜索使用like匹配带%字符时失效问题_java_脚本之家
and idwl.detail like concat(
'%'
, #{paramVo.detail, jdbcType=VARCHAR},
'%'
) escape
'/'
detail = detail.replaceAll(
"%"
,
"/%"
).replaceAll("_"
,
"/_"
);
4、配置解析
4.1. 核心配置文件
-
mybatis-config.xml
-
Mybatis的配置文件包含了会深深影响MyBatis行为的设置和属性信息。
configuration(配置)
properties(属性)
settings(设置)
typeAliases(类型别名)
typeHandlers(类型处理器)
objectFactory(对象工厂)
plugins(插件)
environments(环境配置)
environment(环境变量)
transactionManager(事务管理器)
dataSource(数据源)
databaseIdProvider(数据库厂商标识)
mappers(映射器)
4.2. 环境配置 environments
MyBatis 可以配置成适应多种环境,default就代表当前环境
<environments default="test">
<environment id="development">
....
</environment>
<environment id="test">
....
</environment>
</environments>
不过要记住:尽管可以配置多个环境,但每个 SqlSessionFactory 实例只能选择一种环境
学会使用配置多套运行环境!
MyBatis默认的事务管理器就是JDBC ,连接池:POOLED
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--引入外部配置文件-->
<properties resource="db.properties"></properties>
<!-- <properties resource="db.properties"/> 推荐使用自闭合/ -->
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/> //事务管理器,一般使用jdbc,有2种:JDBC | MANAGED
<dataSource type="POOLED"> //连接池,一般使用pooled,有3种:UNPOOLED|POOLED|JNDI
<property name="driver" value="${driver}"/> //通过${}获取properties文件中的配置
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<!--每一个Mapper.xml都需要在mybatis核心配置文件中注册!-->
<mappers>
<mapper resource="com/yff/dao/UserMapper.xml"/>
</mappers>
</configuration>
4.3. 属性 properties
我们可以通过properties属性来实现引用配置文件
这些属性可以在外部进行配置,并可以进行动态替换。你既可以在典型的 Java 属性文件中配置这些属性,也可以在 properties 元素的子元素中设置。【db.poperties】
1.编写一个配置文件
db.properties
driver=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/mybatis?userSSL=true&useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
username=root
password=123
1.可以直接引入外部文件
<properties resource="db.properties"/> 后通过${}获取
2.可以在其中增加一些属性配置,eg:增加username和pwd
3.如果两个文件有同一个字段,优先使用外部配置文件的,eg:
driver=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/mybatis?userSSL=true&useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
username=root
password=root
----------------------------------------------
4.备注:mybatis-config.xml核心配置文件中,标签是有顺序的,必须遵守:
-----------------------------------------------
4.4. 类型别名 typeAliases
-
类型别名可为 Java 类型设置一个缩写名字。 它仅用于 XML 配置.
-
意在降低冗余的全限定类名书写。
第一种:给实体类起别名
<!--可以给实体类起别名-->
<typeAliases>
<typeAlias type="com.kuang.pojo.User" alias="User"/>
</typeAliases>
第二种:指定一个包,每一个在包 com.kuang.pojo 中的 Java Bean,在没有注解的情况下,会使用 Bean 的首字母小写的非限定类名来作为它的别名。 比如 com.kuang.pojo.User 的别名为 user,;这是官方文档推荐的,用这种方式的话类的开头字母要使用小写,但是使用User(大写)测试后其实也是可以的
<typeAliases>
<package name="com.kuang.pojo"/>
</typeAliases>
若有注解,则别名为其注解值。见下面的例子:
@Alias("hello")
public class User{
...
}
如何选择:
在实体类比较少的时候,使用第一种方式。
如果实体类十分多,建议用第二种扫描包的方式。
第一种可以自定义别名,第二种不行,如果非要改,需要在实体上增加注解。
注意:下面是一些为常见的 Java 类型内建的类型别名。它们都是不区分大小写的,注意,为了应对原始类型的命名重复,采取了特殊的命名风格。
别名 映射的类型
_byte byte
_long long
_short short
_int int
_integer int
_double double
_float float
_boolean boolean
string String
byte Byte
long Long
short Short
int Integer
integer Integer
double Double
float Float
boolean Boolean
date Date
decimal BigDecimal
bigdecimal BigDecimal
object Object
map Map
hashmap HashMap
list List
arraylist ArrayList
collection Collection
iterator Iterator
4.5. 设置 Settings
这是 MyBatis 中极为重要的调整设置,它们会改变 MyBatis 的运行时行为。(官网设置Settings有全面解释:mybatis – MyBatis 3 | 配置)
重点:
4.6. 其他配置(了解即可)
- typeHandlers(类型处理器)
- objectFactory(对象工厂)
- plugins 插件
- mybatis-generator-core
- mybatis-plus
- 通用mapper
4.7. 映射器 mappers
MapperRegistry:注册绑定我们的Mapper文件;
方式一:【推荐使用】
<!--每一个Mapper.xml都需要在MyBatis核心配置文件中注册-->
<mappers>
<mapper resource="com/kuang/dao/UserMapper.xml"/>
</mappers>
方式二:使用class文件绑定注册
<!--每一个Mapper.xml都需要在MyBatis核心配置文件中注册-->
<mappers>
<mapper class="com.kuang.dao.UserMapper"/>
</mappers>
注意点:
- 接口UserMapper和他的Mapper配置文件必须同名
- 接口UserMapper和他的Mapper配置文件必须在同一个包下
方式三:使用包扫描进行注入(注意点和方式二一样)
<mappers>
<package name="com.kuang.dao"/>
</mappers>
注意点:
- 接口UserMapper和他的Mapper配置文件必须同名
- 接口UserMapper和他的Mapper配置文件必须在同一个包下
4.8. 作用域和生命周期
声明周期和作用域是至关重要的,因为错误的使用会导致非常严重的并发问题。
SqlSessionFactoryBuilder:
-
一旦创建了SqlSessionFactory,就不再需要它了
-
局部变量
SqlSessionFactory:
-
说白了就可以想象为:数据库连接池
-
SqlSessionFactory一旦被创建就应该在应用的运行期间一直存在,没有任何理由丢弃它或重新创建一个实例。
-
因此SqlSessionFactory的最佳作用域是应用作用域(ApplocationContext)。
-
最简单的就是使用单例模式或静态单例模式。
SqlSession:
- 连接到连接池的一个请求
- SqlSession 的实例不是线程安全的,因此是不能被共享的,所以它的最佳的作用域是请求或方法作用域。
- 用完之后需要赶紧关闭,否则资源被占用!
其中的每一个mapper都是代表的一个具体的业务(增删改查)
5、解决实体类属性名和数据库字段名不一致的问题(和10关联起来看)
(1、字段起别名 2、resultMap)
数据库中的字段
新建一个项目,拷贝之前的,测试实体类字段不一致的情况
// select * from user where id = #{id}
或
// select id,name,pwd from user where id = #{id}
测试出现问题
解决方法1:
- 起别名
<select id="getUserById" resultType="com.kuang.pojo.User">
select id,name,pwd as password from USER where id = #{id}
</select>
5.2. resultMap(解决方法2即是使用resultMap)
结果集映射
id name pwd
id name password
<!--结果集映射-->
<resultMap id="UserMap" type="User">
<!--column数据库中的字段,property实体类中的属性-->
<result column="id" property="id"></result>
<result column="name" property="name"></result>
<result column="pwd" property="password"></result>
</resultMap>
<select id="getUserList" resultMap="UserMap">
select * from USER
</select>
-
ResultMap 元素是 MyBatis 中最重要最强大的元素。
-
ResultMap 的设计思想是,对简单的语句做到零配置,对于复杂一点的语句,只需要描述语句之间的关系就行了。
-
ResultMap 的优秀之处——你完全可以不用显式地配置它们。
resultMap的作用(工作中必用)
<resultMap>标签用于封装sql的查询结果,可以包装成一个简单POJO对象,也可以包装成我们自定义的对象,只要我们使用<result>子标签指定好查询结果的列和对象的属性之间的对应关系就好了。
官方原因
说是用于提高性能,但是在一些情况下,没有Id的话结果会出错。
官方doc传送门(中文):https://mybatis.org/mybatis-3/zh/sqlmap-xml.html
博客:MyBatis <resultMap>中的<id>的作用到底是什么? - 简书
6、日志
6.1 日志工厂
如果一个数据库操作,出现了异常,我们需要排错,日志就是最好的助手!
曾经排错:sout、debug
现在排错:日志工厂
- SLF4J
- LOG4J 【掌握】
- LOG4J2
- JDK_LOGGING
- COMMONS_LOGGING
- STDOUT_LOGGING 【掌握】
- NO_LOGGING
在MyBatis中具体使用哪一个日志实现,在设置中设定
STDOUT_LOGGING
<settings>
//标准的日志工厂
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
6.2 Log4j
什么是Log4j?
-
Log4j是Apache的一个开源项目,通过使用Log4j,我们可以控制日志信息输送的目的地是控制台、文件、GUI组件;
-
我们也可以控制每一条日志的输出格式;
-
通过定义每一条日志信息的级别,我们能够更加细致地控制日志的生成过程;
-
最令人感兴趣的就是,这些可以通过一个配置文件来灵活地进行配置,而不需要修改应用的代码。
1.先导入log4j的包
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
2.log4j.properties
#将等级为DEBUG的日志信息输出到console和file这两个目的地,console和file的定义在下面的代码
log4j.rootLogger=DEBUG,console,file
#控制台输出的相关设置
log4j.appender.console = org.apache.log4j.ConsoleAppender
log4j.appender.console.Target = System.out
log4j.appender.console.Threshold=DEBUG
log4j.appender.console.layout = org.apache.log4j.PatternLayout
log4j.appender.console.layout.ConversionPattern=[%c]-%m%n
#文件输出的相关设置
log4j.appender.file = org.apache.log4j.RollingFileAppender
log4j.appender.file.File=./log/yff.log
log4j.appender.file.MaxFileSize=10mb
log4j.appender.file.Threshold=DEBUG
log4j.appender.file.layout=org.apache.log4j.PatternLayout
log4j.appender.file.layout.ConversionPattern=[%p][%d{yy-MM-dd}][%c]%m%n
#日志输出级别
log4j.logger.org.mybatis=DEBUG
log4j.logger.java.sql=DEBUG
log4j.logger.java.sql.Statement=DEBUG
log4j.logger.java.sql.ResultSet=DEBUG
log4j.logger.java.sq1.PreparedStatement=DEBUG
3.配置settings为log4j实现
<settings>
<setting name="logImpl" value="LOG4J"/>
</settings>
4.直接测试运行
Log4j简单使用
1.在要使用Log4j的类中,导入包 import org.apache.log4j.Logger;
2.日志对象,参数为当前类的class对象
Logger logger = Logger.getLogger(UserDaoTest.class);
3.日志级别:分为OFF、FATAL、ERROR、WARN、INFO、DEBUG、ALL或者您定义的级别。Log4j建议只使用四个级别,优 先级从高到低分别是ERROR、WARN、INFO、DEBUG
@Test
public void testLog4j() {
logger.info("info日志");
logger.debug("debug日志");
logger.warn("warn日志");
logger.error("error日志");
try {
int i = 1;
int y = 0;
int z = i / y;
} catch (Exception e) {
logger.error(e);
}
}
7、分页
思考:为什么分页?
- 减少数据的处理量
7.1 使用Limit分页
SELECT * from user limit startIndex,pageSize
使用MyBatis实现分页,核心SQL
1.接口
//分页
List<User> getUserByLimit(Map<String,Integer> map);
2.Mapper.xml
<!--分页查询-->
<select id="getUserByLimit" parameterType="map" resultMap="UserMap">
select * from user limit #{startIndex},#{pageSize}
</select>
3.测试
@Test
public void getUserByLimit(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
HashMap<String, Integer> map = new HashMap<String, Integer>();
map.put("startIndex",1);
map.put("pageSize",2);
List<User> list = mapper.getUserByLimit(map);
for (User user : list) {
System.out.println(user);
}
}
7.2 RowBounds分页
不再使用SQL实现分页
1.接口
//分页2
List<User> getUserByRowBounds();
2.mapper.xml
<!--分页查询2-->
<select id="getUserListRowBounds" resultMap="UserMap">
select * from user;
</select>
3.测试
public void getUserByRowBounds(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
//RowBounds实现
RowBounds rowBounds = new RowBounds(1, 2);
//通过Java代码层面RowBounds实现分页 RowBounds依赖sqlSession.selectList第二种实现执行sql
List<User> userList = sqlSession.selectList("com.kaung.dao.UserMapper.getUserByRowBounds", null, rowBounds);
for (User user : userList) {
System.out.println(user);
}
sqlSession.close();
}
7.3 分页插件
8、使用注解开发
8.1 面向接口开发
三个面向区别
- 面向对象是指,我们考虑问题时,以对象为单位,考虑它的属性和方法;
- 面向过程是指,我们考虑问题时,以一个具体的流程(事务过程)为单位,考虑它的实现;
- 接口设计与非接口设计是针对复用技术而言的,与面向对象(过程)不是一个问题,更多的体现就是对系统整体的架构;
8.2 mybatis使用注解
官网:
使用注解来映射简单语句会使代码显得更加简洁,但对于稍微复杂一点的语句,Java 注解不仅力不从心,还会让你本就复杂的 SQL 语句更加混乱不堪。 因此,如果你需要做一些很复杂的操作,最好用 XML 来映射语句。
选择何种方式来配置映射,以及认为是否应该要统一映射语句定义的形式,完全取决于你和你的团队。 换句话说,永远不要拘泥于一种方式,你可以很轻松的在基于注解和 XML 的语句映射方式间自由移植和切换。
sql 类型主要分成 :
@select ()
@update ()
@Insert ()
@delete ()
1.注解在接口上实现
@Select("select * from user")
List<User> getUsers();
2.必须在核心配置文件中绑定接口
<mappers>
<mapper class="com.kuang.dao.UserMapper"/>
</mappers>
3.测试
@Test public void testGetAllUser() {
SqlSession session = MybatisUtils.getSession();
//本质上利用了jvm的动态代理机制
UserMapper mapper = session.getMapper(UserMapper.class);
List<User> users = mapper.getUsers();
for (User user : users){
System.out.println(user);
}
session.close();
}
session.getMapper(UserMapper.class);本质:反射机制实现
mybatis底层:动态代理
MyBatis详细执行流程
8.3 mybatis注解CURD
前提条件:必须在核心配置文件中绑定接口
//编写接口,增加注解
-------------------------------------------------------
public interface UserMapper {
//查询指定用户
@Select("select * from user")
List<User> getUser();
//查询指定用户
@Select("select * from user where id =#{id} and name =#{name}")
User getUser1(@Param("id") int id,@Param("name") String name);
//更新
@Update("update user set pwd =#{password}, name=#{name} where id =#{id}")
int updateUser(User user);
//插入
@Insert("insert into user values(#{id},#{name},#{paaword})")
int insertUser(User user);
//删除
@Delete("delete from user where id=#{uid}")
int deleteUser(@Param("uid") int id);
}
//测试
----------------------------------------------------------
public class UserMapperTest {
static Logger logger = Logger.getLogger(UserMapperTest.class);
@Test
public void getUserTest(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User u = mapper.getUser1(1, "test");
System.out.println(u);
sqlSession.close();
}
@Test
public void getUserTest1(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int num = mapper.updateUser(new User(99, "yyy", "9999"));
System.out.println(num);
sqlSession.close();
}
}
关于@Param( )注解
- @Param("xx")只是针对基本类型,引用类型不需要
- 如果只有一个基本类型的话,可以忽略,但是建议大家都加上
- @Param("uid")和sql中#{uid}必须对应一致,且以@Param为准,我们在SQL中引用的就是我们这里的@Param()中设定的属性名,sql中通过#{} 或 ${}的方式引用
- 方法存在多个参数,所有的参数前面必须加上@Param("id")注解
- 通过#{} 或 ${}可以直接取到参数对象中的属性
- 使用mybatis时,我们在不使用mybatis注解开发时,也可以在接口中使用@Param( )注解,也遵守上面的规则
- 当接口中只有一个参数(并且没有用@Param())时候,需要在xml中添加响应的参数类型parameterType;
如果是多个参数(每个参数都是用@Param())的时候,就不会去读参数类型parameterType,
直接取得参数里面的值。
#{}和${}区别,#{}可以防止sql注入,尽量使用#{}
https://blog.csdn.net/weixin_45433031/article/details/123208290
9、Lombok
Lombok依赖和插件的关系:
依赖中被你调用过的函数会与你的代码一起进行编译。对于插件来说呢,比如有些插件是帮助你进行编译工作的,你不用手动写。比如lombok依赖是为了方便用里面的注解@Data等,lombok插件是为了编译这些注解的,识别这些注解。
Lombok项目是一个Java库,它会自动插入编辑器和构建工具中,Lombok提供了一组有用的注释,用来消除Java类中的大量样板代码。仅五个字符(@Data)就可以替换数百行代码从而产生干净,简洁且易于维护的Java类。但是优缺点很明显
使用步骤:
1.在IDEA中安装Lombok插件
2.在项目中导入lombok的jar包
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.10</version>
<scope>provided</scope>
</dependency>
3、lombok的所有注解
常用注解
@Data 注解在类上;提供类所有属性的 getting 和 setting 方法,还提供了equals、canEqual、hashCode、toString 方法、无参构造方法
@Setter :注解在属性上;为属性提供 setting 方法
@Setter :注解在属性上;为属性提供 getting 方法
@Log4j :注解在类上;为类提供一个 属性名为log 的 log4j 日志对象
@Slf4j:注解在类上;为类提供一个 属性名为log 的 Logback日志对象
@NoArgsConstructor :注解在类上;为类提供一个无参的构造方法
@AllArgsConstructor :注解在类上;为类提供一个全参的构造方法
@Cleanup : 可以关闭流 (不建议使用)
@Builder : 被注解的类加个构造者模式
eg:
UploadLog.builder().supplierId(supplierId).url(request.getRequestURI()) .requestBody(ServletUtil.getRequestBody()).uploadDate(new Date()).build();
@Synchronized : 加个同步锁
@SneakyThrows : 等同于try/catch 捕获异常
@NonNull : 如果给参数加个这个注解 参数为null会抛出空指针异常
@Value : 注解和@Data类似,区别在于它会把所有成员变量默认定义为private final修饰,并且不会生成set方法。
@EqualsAndHashCode 注解:
1. 此注解会生成equals(Object other) 和 hashCode()方法。
2. 它默认使用非静态,非瞬态的属性
3. 可通过参数exclude排除一些属性
4. of 用来指明你要用什么字段来重写equals和hashcode
5. 它默认仅使用该类中定义的属性且不调用父类的方法
6. 可通过callSuper=true解决上一点问题。让其生成的方法中调用父类的方法。callSuper 这个选项只能用在有父类情况下,如上图如果没有Father那么会报错。默认是false,当改成true后,会调用父类的equals方法通过官方文档,可以得知,当使用@Data注解时,则有了@EqualsAndHashCode注解,那么就会在此类中存在equals(Object other) 和 hashCode()方法,且默认不会使用父类的属性,这就导致了可能的问题。
比如,有多个类有相同的部分属性,把它们定义到父类中,恰好id(数据库主键)也在父类中,那么就会存在部分对象在比较时,它们并不相等,却因为lombok自动生成的equals(Object other) 和 hashCode()方法判定为相等,从而导致出错。修复此问题的方法很简单:
1. 使用@Getter @Setter @ToString代替@Data并且自定义equals(Object other) 和 hashCode()方法,比如有些类只需要判断主键id是否相等即足矣。
2. 或者使用在使用@Data时同时加上@EqualsAndHashCode(callSuper=true)注解。
举例:
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
private int id;
private String name;
private String password;
}
注意:多表关联查询 或 多表嵌套联查时,也可以建vo,使用resultType,很简便
<select id="getRentalVehicleFailure" resultType="com.byd.rental.rentalbusiness.entity.po.RentalVehicleFailure">
select <include refid="Base_Column_List"/>
from rental_vehicle_failure rvf
left join rental_vehicle rv on rvf.plate_no = rv.plate_no
left join rental_car_type rc on rv.car_type_id = rc.car_type_id where rvf.id = #{id}
</select>
10、多对一处理(和5关联起来看)
【深入浅出MyBatis系列十二】终结篇:MyBatis原理深入解析3 - 简书(里面mybatis对于嵌套查询的原理解释)
mybatis多对一和一对多查询数据处理解读_mybatisplus一对多查询-CSDN博客(重点)
(多对一和一对多处理,本质还是解决实体类属性名和数据库字段名不一致的问题,是resultmap的使用进阶)
站在学生的角度,多个学生对应一个老师
预置数据库表:
CREATE TABLE `teacher` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8
INSERT INTO teacher(`id`, `name`) VALUES (1, '秦老师');
CREATE TABLE `student` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
`tid` INT(10) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `fktid` (`tid`),
CONSTRAINT `fktid` FOREIGN KEY (`tid`) REFERENCES `teacher` (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('1', '小明', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('2', '小红', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('3', '小张', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('4', '小李', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('5', '小王', '1');
多个学生一个老师;
alter table student ADD CONSTRAINT fk_tid foreign key (tid) references teacher(id)
10.1. 测试环境搭建
1.导入lombok
2.新建实体类Teacher,Student
----------------------------
package com.yff.pojo;
import lombok.Data;
@Data
public class Student {
private int id;
private String name;
//学生需要关联一个老师
private Teacher teacher;
}
-----------------------------
package com.yff.pojo;
import lombok.Data;
@Data
public class Teacher {
private int id;
private String name;
}
3.建立Mapper接口
public interface StudentMapper {
//查询所有学生信息及对应的老师的信息1(子查询实现)
List<Student> getStudent1();
//查询所有学生信息及对应的老师的信息1(联表查询实现)
List<Student> getStudent2();
}
4.建立Mapper.xml文件
5.在核心配置文件中绑定注册我们的Mapper接口或者文件 【方式很多,随心选】
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--引入外部配置文件-->
<properties resource="db.properties"></properties>
<!--<properties resource="db.properties"/> 推荐使用自闭合-->
<settings>
<setting name="logImpl" value="LOG4J"/>
</settings>
<!--全限定名-别名-->
<typeAliases>
<package name="com.yff.pojo"/>
<!--<typeAlias type="com.yff.pojo.User" alias="users"/>-->
</typeAliases>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper class="com.yff.dao.TeacherMapper"/>
<mapper class="com.yff.dao.StudentMapper"/>
</mappers>
</configuration>
6.测试查询是否能够成功
10.2. 按照查询嵌套处理(子查询)
<!--
思路:
1. 查询所有的学生信息
2. 根据查询出来的学生的tid寻找特定的老师 (子查询)
-->
<select id="getStudent" resultMap="StudentTeacher">
select * from student
</select>
<!--column数据库中的字段,property实体类中的属性,要实体类和数据库对应起来看-->
<resultMap id="StudentTeacher" type="student"> //type="student"因为mybatis核心配置文件中有全限定名的别名,并使用指定包
<result property="id" column="id"/>
<result property="name" column="name"/>
<!--复杂的属性,我们需要单独出来 对象:association 集合:collection-->
<association property="teacher" column="tid" javaType="teacher" select="getTeacher"/>
</resultMap>
<select id="getTeacher" resultType="teacher">
select * from teacher where id = #{id} //#{id}会自动推断入参为column="tid",所以可以随意写#{xx},但尽量一致写成#{tid}
</select>
10.3.按照结果嵌套处理(联表查询)
<!--按照结果进行查询-->
<select id="getStudent2" resultMap="StudentTeacher2">
select s.id sid , s.name sname, t.name tname
from student s,teacher t
where s.tid=t.id //联表查询sql
</select>
<!--结果封装,将查询出来的列封装到对象属性中-->
<resultMap id="StudentTeacher2" type="student">
<result property="id" column="sid"/> //column="sid", sql中有别名时必须使用别名
<result property="name" column="sname"/>
<association property="teacher" javaType="teacher"> //javaType="teacher"因为mybatis核心配置文件中有全限定名的别名
<result property="name" column="tname"></result>
</association>
</resultMap>
Mybatis多表关联查询的实现(DEMO)_JAVA教程_服务器之家
回顾Mysql多对一查询方式:
- 子查询 (按照查询嵌套)
- 联表查询 (按照结果嵌套)
11、一对多处理
站在老师的角度,一个老师对应多个学生
11.1. 环境搭建
实体类
@Data
public class Student {
private int id;
private String name;
private int tid;
}
@Data
public class Teacher {
private int id;
private String name;
//一个老师拥有多个学生
private List<Student> students;
}
public interface TeacherMapper {
//获取指定老师下的所有学生和老师的信息
Teacher getTeacher1(@Param("tid") int id);
Teacher getTeacher2(@Param("tid") int id);
}
11.2. 按照结果嵌套处理
<!--按结果嵌套查询-->
<select id="getTeacher" resultMap="StudentTeacher">
SELECT s.id sid, s.name sname,t.name tname,t.id tid FROM student s, teacher t
WHERE s.tid = t.id AND tid = #{tid}
</select>
<resultMap id="StudentTeacher" type="Teacher">
<result property="id" column="tid"/>
<result property="name" column="tname"/>
<!--复杂的属性,我们需要单独处理 对象:association 集合:collection
javaType=""指定属性的类型!
集合中的泛型信息,我们使用ofType获取
因为上面是多对一,多个学生关联一个老师,实体类中是单个对象,使用javaType,而现在一个老师关联多个学生,实体类中属性为List<Student>,所以用ofType获取
-->
<collection property="students" ofType="Student">
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<result property="tid" column="tid"/>
</collection>
</resultMap>
11.3. 按照查询嵌套处理
<select id="getTeacher2" resultMap="StudentTeacher2">
select * from teacher where id =#{tid};
</select>
<resultMap id="StudentTeacher2" type="teacher">
<result property="id" column="id"/>
<result property="name" column="name"/>
<collection property="students" javaType="ArrayList" ofType="student" select="getstudentbyteacherId" column="id"/>
</resultMap>
<select id="getstudentbyteacherId" resultType="student">
select * from student where tid =#{uid}
</select>
测试
多对一和一对多也可以这样用:
<mapper namespace="com.oceansite.system.mapper.SysUserMapper">
<resultMap type="SysUser" id="SysUserResult">
<id property="userId" column="user_id" />
<result property="deptId" column="dept_id" />
<result property="userName" column="user_name" />
<result property="nickName" column="nick_name" />
<result property="email" column="email" />
<result property="phonenumber" column="phonenumber" />
<result property="sex" column="sex" />
<result property="avatar" column="avatar" />
<result property="password" column="password" />
<result property="status" column="status" />
<result property="delFlag" column="del_flag" />
<result property="loginIp" column="login_ip" />
<result property="loginDate" column="login_date" />
<result property="createBy" column="create_by" />
<result property="createTime" column="create_time" />
<result property="updateBy" column="update_by" />
<result property="updateTime" column="update_time" />
<result property="remark" column="remark" />
<result property="signImg" column="image"/>
<association property="dept" column="dept_id" javaType="SysDept" resultMap="deptResult" />
<collection property="roles" javaType="java.util.List" resultMap="RoleResult" />
</resultMap>
<resultMap id="deptResult" type="SysDept"> 多对一
<id property="deptId" column="dept_id" />
<result property="parentId" column="parent_id" />
<result property="deptName" column="dept_name" />
<result property="orderNum" column="order_num" />
<result property="leader" column="leader" />
<result property="status" column="dept_status" />
</resultMap>
<resultMap id="RoleResult" type="SysRole"> 一对多
<id property="roleId" column="role_id" />
<result property="roleName" column="role_name" />
<result property="roleKey" column="role_key" />
<result property="roleSort" column="role_sort" />
<result property="dataScope" column="data_scope" />
<result property="status" column="role_status" />
</resultMap>
小结
1.关联 - association 【多对一】
2.集合 - collection 【一对多】
3.javaType & ofType
- JavaType用来指定实体类中的类型
- ofType用来指定映射到List或者集合中的pojo类型,泛型中的约束类型
注意点:
- 保证SQL的可读性,尽量保证通俗易懂
- 注意一对多和多对一,属性名和字段的问题
- 如果问题不好排查错误,可以使用日志,建议使用Log4j
备注(column有多个参数时怎么办?):
面试高频
- Mysql引擎
- InnoDB底层原理
- 索引
- 索引优化
12、动态SQL
什么是动态SQL:(使用动态标签)所谓的动态sql本质还是sql语句,只是我们在sql层面,执行了逻辑代码,(sql尽量还是标准)
- if
- choose (when, otherwise)
- trim (where, set)
- foreach
动态SQL就是根据不同的条件生成不同的SQL语句,所谓的动态SQL,本质上还是SQL语句,只是我们可以在SQL层面,去执行一个逻辑代码
动态 SQL 是 MyBatis 的强大特性之一。如果你使用过 JDBC 或其它类似的框架,你应该能理解
根据不同条件拼接 SQL 语句有多痛苦,例如拼接时要确保不能忘记添加必要的空格,还要注意去
掉列表最后一个列名的逗号。利用动态 SQL,可以彻底摆脱这种痛苦。
搭建环境并插入数据
vCREATE TABLE `mybatis`.`blog` (
`id` int(10) NOT NULL AUTO_INCREMENT COMMENT '博客id',
`title` varchar(30) NOT NULL COMMENT '博客标题',
`author` varchar(30) NOT NULL COMMENT '博客作者',
`create_time` datetime(0) NOT NULL COMMENT '创建时间',
`views` int(30) NOT NULL COMMENT '浏览量',
PRIMARY KEY (`id`)
)
IF详解:
注意:当treatmentStatus为integer或Long类型时,使用failure.treatmentStatus != ''会导致不能识别为0的参数,下面的写法会出问题!
错误写法
<if test="failure.treatmentStatus != null and failure.treatmentStatus != ''">
and rvf.treatment_status = #{failure.treatmentStatus}
</if>
正确写法:
<if test="failure.treatmentStatus != null">
and rvf.treatment_status = #{failure.treatmentStatus}
</if>
创建一个基础工程
1.导包
2.编写配置文件
3.编写实体类
@Data
public class Blog {
private String id;
private String title;
private String author;
private Date createTime; //属性名和字段名不一致,通过setting解决
private int views;
}
4.编写实体类对应Mapper接口
Mapper.xml文件(IF),注意下面xml可以优化使用where标签,省略where 1=1,实际工作中这么写不好
choose (when, otherwise)详解:
有时候,我们不想使用所有的条件,而只是想从多个条件中选择一个使用。针对这种情况,MyBatis 提供了 choose 元素,它有点像 Java 中的 switch 语句。
还是上面的例子,但是策略变为:传入了 “title” 就按 “title” 查找,传入了 “author” 就按 “author” 查找的情形。若两者都没有传入,就返回默认的views, choose (when, otherwise)只会选择其中的一条语句去执行。为了安全建议加上where,第一个when可以不加and,其余建议也加上and
测试:
下面通过map传了3个参数进行测试,由于是choose (when, otherwise)语句,所以只会执行第一个,查询出title等于"java如此简单"
trim、where、set详解:
where 元素:只会在子元素返回任何内容的情况下才插入 “WHERE” 子句。而且,若子句的开头为 “AND” 或 “OR”,where 元素也会将它们去除。
例如(有where元素这样就很完美,解决了这2个问题):
<select id="findActiveBlogLike"
resultType="Blog">
SELECT * FROM BLOG
<where>
<if test="state != null">
state = #{state}
</if>
<if test="title != null">
AND title like #{title}
</if>
<if test="author != null and author.name != null">
AND author_name like #{author.name}
</if>
</where>
</select>
set元素:set 元素会动态地在行首插入 SET 关键字,并会删掉额外的逗号(这些逗号是在使用条件语句给列赋值时引入的)
tirm元素:
TRIM标签的prefix/suffix属性:如果trim后内容不为空,则增加某某字符串(作前缀/后缀);
TRIM标签的prefixOverrides/suffixOverrides属性:如果trim后内容不为空,则删掉(前缀/后缀的)某某字符串。
https://www.jb51.net/article/217277.htm
prefix 字首, prefixOverrides 字首覆盖, suffix后缀, suffixOverrides 后缀覆盖
例如:与 where 元素等价的自定义 trim 元素为:(注意此例中的空格是必要的)
<trim prefix="WHERE" prefixOverrides="AND |OR ">
...
</trim>
例如:与 set 元素等价的自定义 trim 元素为:
foreach详解:
List<Blog> queryBlogForeach(Map map);
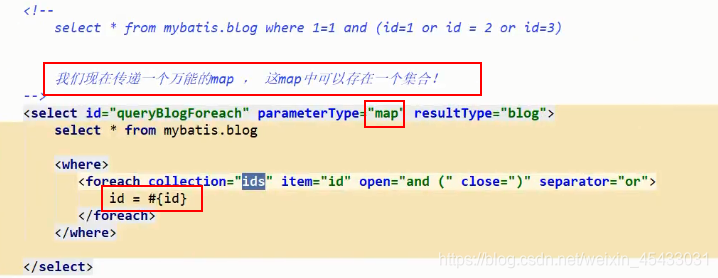
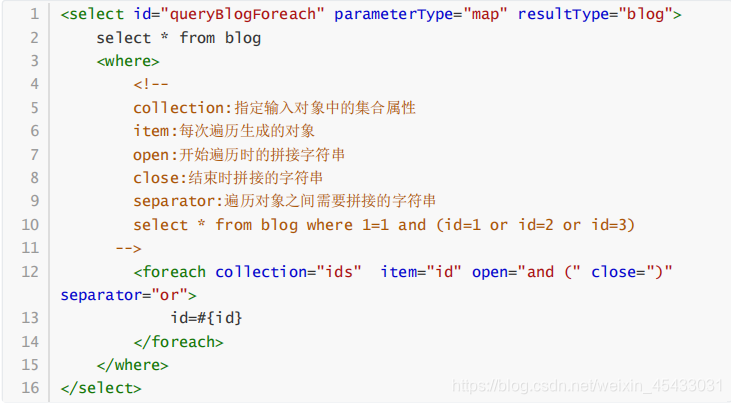
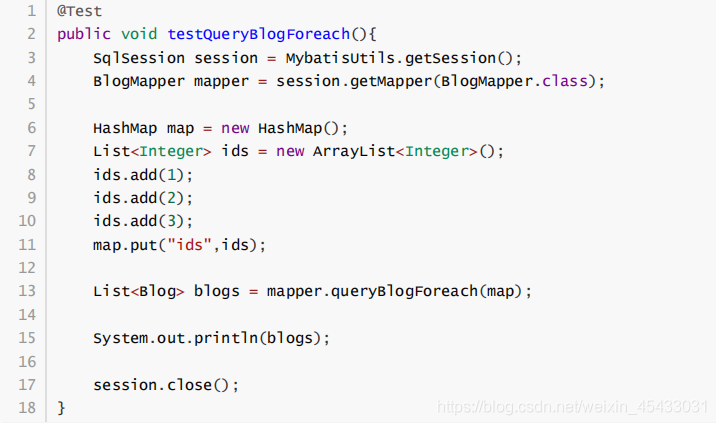
工作举例:
Integer deleteAlarmRecordByIds(Long[] alarmId);
<delete id="deleteAlarmRecordByIds" parameterType="Long">
delete from alarm_record where alarm_id in
<foreach collection="array" item="alarmId" open="(" separator="," close=")">
#{alarmId}
</foreach>
</delete>
--------------------------------------------------------------
Integer deleteDict(@Param("dictType") String dictType,@Param("shipImo")String shipImo, @Param("dictIds")Long[] dictIds);
<delete id="deleteDict">
delete from cctv_dict where dict_type = #{dictType} and ship_imo = #{shipImo} and dict_id in
<foreach collection="dictIds" item="dictId" open="(" separator="," close=")">
#{dictId}
</foreach>
</delete>
sql片段详解:
有的时候,我们可能会将一些公共的sql抽取出来,方便使用!
1.使用SQL标签抽取公共部分
<sql id="if-title-author">
<if test="title!=null">
title = #{title}
</if>
<if test="author!=null">
and author = #{author}
</if>
</sql>
2.使用 include 引用sql片段
sql片段扩展:
mybatis xml 文件中对于重复出现的sql 片段可以使用标签提取出来,在使用的地方使用标签引用即可具体用法如下:
<sql id="someSQL">
id,name
</sql>
<select id="selectSome" >
select
<include refid="someSQL"/>
from t
</select>
在中可以使用${}传入参数,如下:
<sql id="someSQL">
${tableName}.id,${tableName}.name
</sql>
<select id="selectSome" >
select
<include refid="someSQL"><property name="tableName" value="t"/></include>
from t
</select>
对于多个xml文件需要同时引用一段相同的 可以在某个xml 中定义这个 sql 代码片段,在需要引用的地方使用全称引用即可,例子如下:
ShareMapper.xml
<mapper namespace="com.company.ShareMapper">
<sql id="someSQL">
id,name
</sql>
</mapper>
CustomMapper.xml
<mapper namespace="com.company.CustomMapper">
<select id="selectSome" >
select
<include refid="com.company.ShareMapper.someSQL"/>
from t
</select>
</mapper>
注意事项:
-
最好基于单表来定义SQL片段
-
不要在sql片段中存在where标签
动态sql小结:
- 先在Mysql中写出完整的SQL,再对应的去修改成我们的动态SQL实现通用即可
- 动态SQL就是在拼接SQL语句,我们只要保证SQL的正确性,按照SQL的格式,去排列组合就可以了
- 通过我们的入参的变化形成了动态的效果,如map.put 和 list.add
- 实际工作中的sql会非常多,会联很多的表
13、缓存
13.1 简介
查询 : 连接数据库,耗资源
一次查询的结果,给他暂存一个可以直接取到的地方 --> 内存:缓存
我们再次查询的相同数据的时候,直接走缓存,不走数据库了
1.什么是缓存[Cache]?
存在内存中的临时数据,将用户经常查询的数据放在缓存(内存)中,用户去查询数据就不用从磁盘上(关系型数据库文件)查询,从缓存中查询,从而提高查询效率,解决了 高并发系统的性能问题
2.为什么使用缓存?
减少和数据库的交互次数,减少系统开销,提高系统效率
3.什么样的数据可以使用缓存?
经常查询并且不经常改变的数据 【可以使用缓存】。简单理解,只有查询才会用到缓存!!!
13.2 MyBatis缓存 (目前Redis使用最多)
- MyBatis包含一个非常强大的查询缓存特性,它可以非常方便的定制和配置缓存,缓存可以极大的提高查询效率。
- MyBatis系统中默认定义了两级缓存:一级缓存和二级缓存
- 默认情况下,一级缓存开启(SqlSession级别的缓存,也称为本地缓存)
- 二级缓存需要手动开启和配置,他是基于namespace级别的缓存。 举例:namespace="com.yff.dao.BlogMapper" namespace级别即接口级别
- 为了提高可扩展性,MyBatis定义了缓存接口Cache。我们可以通过实现Cache接口来定义二级缓存。
13.3 一级缓存
- 一级缓存也叫本地缓存:SqlSession
- 与数据库同一次会话期间查询到的数据会放在本地缓存中
- 以后如果需要获取相同的数据,直接从缓存中拿,没必要再去查询数据库
- 默认情况下,只有一级缓存开启(SqlSession级别的缓存,也称为本地缓存),只在SqlSession中有效,也无法关闭一级缓存,也就是拿到连接到关闭连接这个区间,一级缓存本质就是一个Map
测试步骤:
1.开启日志
2.测试在一个SqlSession中查询两次相同记录
@Test
public void test1() {
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User user = mapper.getUserById(1); -----
System.out.println(user);
System.out.println("=====================================");
User user2 = mapper.getUserById(1); ------
System.out.println(user2 == user);
}
3.查看日志输出,数据库只查询了一次,第二次使用的缓存
一级缓存SqlSession失效的情况:
1.查询不同的东西(查询id=1和id=2)
2.增删改操作,可能会改变原来的数据,所以必定会刷新缓存,缓存会失效,会重新查询数据库
3.查询不同的Mapper.xml
二级缓存也会失效,更别提一级缓存了
4.手动清理缓存
sqlSession.clearCache();
13.4 二级缓存
概念:
1、二级缓存与一级缓存区别在于二级缓存的范围更大,多个sqlSession可以共享一个mapper中的二级缓存区域。
2、mybatis是如何区分不同mapper的二级缓存区域呢?它是按照不同mapper有不同的namespace来区分的,也就是说,如果两个mapper的namespace相同,即使是两个mapper,那么这两个mapper中执行sql查询到的数据也将存在相同的二级缓存区域中。
3、由于mybaits的二级缓存是mapper范围级别,所以除了在SqlMapConfig.xml设置二级缓存的总开关外,还要在具体的mapper.xml中开启二级缓存。
4、二级缓存是事务性的。这意味着,当 SqlSession 完成并提交时,或是完成并回滚,但没有执行 flushCache=true 的 insert/delete/update 语句时,缓存会获得更新。
使用步骤:
1.开启全局缓存(在mybatis核心配置文件里setting)
<!--显示的开启全局缓存-->
<setting name="cacheEnabled" value="true"/>
2.在Mapper.xml中使用缓存
<!--在当前Mapper.xml中使用二级缓存,这是复杂用法,设置了参数-->
<cache
eviction="FIFO"
flushInterval="60000"
size="512"
readOnly="true"/>
<!--在当前Mapper.xml中使用二级缓存,这是简单用法,就使用默认的即可--> 一般就这样用
<cache/>
上述代码含义为:创建了一个 FIFO (io流的)缓存,每隔 60 秒刷新,最多可以存储结果对象或列表的 512 个引用,而且返回的对象被认为是只读的。
flushInterval:(刷新间隔)属性可以被设置为任意的正整数,设置的值应该是一个以毫秒为单位的合理时间量。 默认情况是不设置,也就是没有刷新间隔,缓存仅仅会在调用语句时刷新。
size:(引用数目)属性可以被设置为任意正整数,要注意欲缓存对象的大小和运行环境中可用的内存资源。默认值是 1024。
readOnly:(只读)属性可以被设置为 true 或 false。只读的缓存会给所有调用者返回缓存对象的相同实例。 因此这些对象不能被修改。这就提供了可观的性能提升。而可读写的缓存会(通过序列化)返回缓存对象的拷贝。 速度上会慢一些,但是更安全,因此默认值是 false。
3.测试
@Test
public void testCache2() throws Exception {
SqlSession sqlSession1 = sqlSessionFactory.openSession();
SqlSession sqlSession2 = sqlSessionFactory.openSession();
SqlSession sqlSession3 = sqlSessionFactory.openSession();
// 创建代理对象
UserMapper userMapper1 = sqlSession1.getMapper(UserMapper.class);
// 第一次发起请求,查询id为1的用户
User user1 = userMapper1.findUserById(1);
System.out.println(user1);
//这里执行关闭操作,将sqlsession中的数据写到二级缓存区域,重点-->执行关闭操作才会把将sqlsession中的数据写到二级缓存区域
sqlSession1.close();
//sqlSession3用来清空缓存的,如果要测试二级缓存,需要把该部分注释掉
//使用sqlSession3执行commit()操作
UserMapper userMapper3 = sqlSession3.getMapper(UserMapper.class);
User user = userMapper3.findUserById(1);
user.setUsername("倪升武");
userMapper3.updateUser(user);
//执行提交,清空UserMapper下边的二级缓存
sqlSession3.commit();
sqlSession3.close();
UserMapper userMapper2 = sqlSession2.getMapper(UserMapper.class);
// 第二次发起请求,查询id为1的用户
User user2 = userMapper2.findUserById(1);
System.out.println(user2);
sqlSession2.close();
}
我们先把sqlSession3部分注释掉来测试一下二级缓存的结果:
当我们把sqlSession3部分加上后,再测试一下二级缓存结果:
到这里,就明白了mybatis中二级缓存的执行原理
其他配置(useCache和flushCache)
useCache:
mybatis中还可以配置userCache和flushCache等配置项,userCache是用来设置是否禁用二级缓存的,在statement中设置useCache=false可以禁用当前select语句的二级缓存,即每次查询都会发出sql去查询,默认情况是true,即该sql使用二级缓存。这种情况是针对每次查询都需要最新的数据sql,要设置成useCache=false,禁用二级缓存,直接从数据库中获取。
<select id="findOrderListResultMap" resultMap="ordersUserMap" useCache="false">
flushCache:
在mapper的同一个namespace中,如果有其它insert、update、delete操作数据后需要刷新缓存,如果不执行刷新缓存会出现脏读。 设置statement配置中的flushCache=”true” 属性,默认情况下为true,即刷新缓存,如果改成false则不会刷新。使用缓存时如果手动修改数据库表中的查询数据会出现脏读。一般下执行完commit操作都需要刷新缓存,flushCache=true表示刷新缓存,这样可以避免数据库脏读。一般我们不用设置,默认即可,这里只是提一下。
<insert id="insertUser" parameterType="cn.itcast.mybatis.po.User" flushCache="true">
问题:我们需要将实体类序列化,否则就会报错
将pojo类实现Serializable接口
开启了二级缓存后,还需要将要缓存的pojo实现Serializable接口,为了将缓存数据取出执行反序列化操作,因为二级缓存数据存储介质多种多样,不一定只存在内存中,有可能存在硬盘中,如果我们要再取这个缓存的话,就需要反序列化了。所以建议mybatis中的pojo都去实现Serializable接口。下面以User为例截个图:
小结:
- 只要开启了二级缓存,在同一个Mapper下就有效
- 所有的数据都会先放在一级缓存中
- 只有当前会话提交,或者关闭的时候,才会提交到二级缓存中
13.5 缓存原理
13.6 自定义缓存-ehcache(用得少,Redis多)
Ehcache是一种广泛使用的开源Java分布式缓存框架。
1.导包
<dependency>
<groupId>org.mybatis.caches</groupId>
<artifactId>mybatis-ehcache</artifactId>
<version>1.2.1</version>
</dependency>
2.在mapper中指定使用我们的 Ehcache 缓存实现类
<cache type="org.mybatis.caches.ehcache.EhcacheCache"/>
13.7 cache-ref:引用缓存
回想一下上一节的内容,对某一命名空间的语句,只会使用该命名空间的缓存进行缓存或刷新。 但你可能会想要在多个命名空间中共享相同的缓存配置和实例。要实现这种需求,你可以使用 cache-ref 元素来引用另一个缓存。
<cache-ref namespace="com.someone.application.data.SomeMapper"/>
13.8 . MyBatis整合ehcache分布式缓存框架
3.1 问题的由来
上面的部分主要总结了一下mybatis中二级缓存的使用,但是mybatis中默认自带的二级缓存有个弊端,即无法实现分布式缓存,什么意思呢?就是说缓存的数据在自己的服务器上,假设现在有两个服务器A和B,用户访问的时候访问了A服务器,查询后的缓存就会放在A服务器上,假设现在有个用户访问的是B服务器,那么他在B服务器上就无法获取刚刚那个缓存,如下图所示:
所以我们为了解决这个问题,就得找一个分布式的缓存,专门用来存储缓存数据的,这样不同的服务器要缓存数据都往它那里存,取缓存数据也从它那里取,如下图所示:
这样就能解决上面所说的问题,为了提高系统并发性能、我们一般对系统进行上面这种分布式部署(集群部署方式),所以要使用分布式缓存对缓存数据进行集中管理。但是mybatis无法实现分布式缓存,需要和其它分布式缓存框架进行整合,这里主要介绍ehcache。
3.2 整合方法
上文一开始提到过,mybatis提供了一个cache接口,如果要实现自己的缓存逻辑,实现cache接口开发即可。mybatis本身默认实现了一个,但是这个缓存的实现无法实现分布式缓存,所以我们要自己来实现。ehcache分布式缓存就可以,mybatis提供了一个针对cache接口的ehcache实现类,这个类在mybatis和ehcache的整合包中。所以首先我们需要导入整合包(点我下载)。
导入了jar包后,配置mapper中cache中的type为ehcache对cache接口的实现类型。ehcache对cache接口有一个实现类为:
我们将该类的完全限定名写到type属性中即可,如下:
OK,配置完成,现在mybatis就会自动去执行这个ehcache实现类了,就不会使用自己默认的二级缓存了,但是使用ehcache还有一个缓存配置别忘了,在classpath下新建一个ehcache.xml文件:
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="../config/ehcache.xsd">
<diskStore path="F:\develop\ehcache"/>
<defaultCache
maxElementsInMemory="10000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
maxElementsOnDisk="10000000"
diskExpiryThreadIntervalSeconds="120"
memoryStoreEvictionPolicy="LRU">
<persistence strategy="localTempSwap"/>
</defaultCache>
</ehcache>
编写ehcache.xml文件,如果在 加载时 未找到 /ehcache.xml 资源或出现问题,则将使用默认配置。
<?xml version="1.0" encoding="UTF-8"?>
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://ehcache.org/ehcache.xsd"
updateCheck="false">
<!--
diskStore:为缓存路径,ehcache分为内存和磁盘两级,此属性定义磁盘的缓存位
置。参数解释如下:
user.home – 用户主目录
user.dir – 用户当前工作目录
java.io.tmpdir – 默认临时文件路径
-->
<diskStore path="./tmpdir/Tmp_EhCache"/>
<defaultCache
eternal="false"
maxElementsInMemory="10000"
overflowToDisk="false"
diskPersistent="false"
timeToIdleSeconds="1800"
timeToLiveSeconds="259200"
memoryStoreEvictionPolicy="LRU"/>
<cache
name="cloud_user"
eternal="false"
maxElementsInMemory="5000"
overflowToDisk="false"
diskPersistent="false"
timeToIdleSeconds="1800"
timeToLiveSeconds="1800"
memoryStoreEvictionPolicy="LRU"/>
<!--
defaultCache:默认缓存策略,当ehcache找不到定义的缓存时,则使用这个缓存策
略。只能定义一个。
-->
<!--
name:缓存名称。
maxElementsInMemory:缓存最大数目
maxElementsOnDisk:硬盘最大缓存个数。
eternal:对象是否永久有效,一但设置了,timeout将不起作用。
overflowToDisk:是否保存到磁盘,当系统当机时
timeToIdleSeconds:设置对象在失效前的允许闲置时间(单位:秒)。仅当
eternal=false对象不是永久有效时使用,可选属性,默认值是0,也就是可闲置时间无穷大。
timeToLiveSeconds:设置对象在失效前允许存活时间(单位:秒)。最大时间介于创建
时间和失效时间之间。仅当eternal=false对象不是永久有效时使用,默认是0.,也就是对象存
活时间无穷大。
diskPersistent:是否缓存虚拟机重启期数据 Whether the disk store
persists between restarts of the Virtual Machine. The default value is
false.
diskSpoolBufferSizeMB:这个参数设置DiskStore(磁盘缓存)的缓存区大小。默
认是30MB。每个Cache都应该有自己的一个缓冲区。
diskExpiryThreadIntervalSeconds:磁盘失效线程运行时间间隔,默认是120秒。
memoryStoreEvictionPolicy:当达到maxElementsInMemory限制时,Ehcache将
会根据指定的策略去清理内存。默认策略是LRU(最近最少使用)。你可以设置为FIFO(先进先
出)或是LFU(较少使用)。
clearOnFlush:内存数量最大时是否清除。
memoryStoreEvictionPolicy:可选策略有:LRU(最近最少使用,默认策略)、
FIFO(先进先出)、LFU(最少访问次数)。
FIFO,first in first out,这个是大家最熟的,先进先出。
LFU, Less Frequently Used,就是上面例子中使用的策略,直白一点就是讲一直以
来最少被使用的。如上面所讲,缓存的元素有一个hit属性,hit值最小的将会被清出缓存。
LRU,Least Recently Used,最近最少使用的,缓存的元素有一个时间戳,当缓存容
量满了,而又需要腾出地方来缓存新的元素的时候,那么现有缓存元素中时间戳离当前时间最远的
元素将被清出缓存。
-->
</ehcache>
最后,练习smbms的spring项目中的29道mybatis题