axios
- 基于promise用于浏览器和node.js的http客户端
- 支持浏览器和node.js
- 支持promise
- 能拦截请求和响应
- 自动转换JSON数据
- 能转换请求和响应数据
axios基础用法
- get和 delete请求传递参数
- 通过传统的url 以 ? 的形式传递参数
- restful 形式传递参数
- 通过params 形式传递参数
- post 和 put 请求传递参数
- 通过选项传递参数
- 通过 URLSearchParams 传递参数
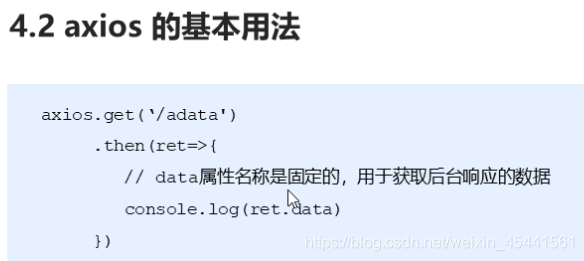
-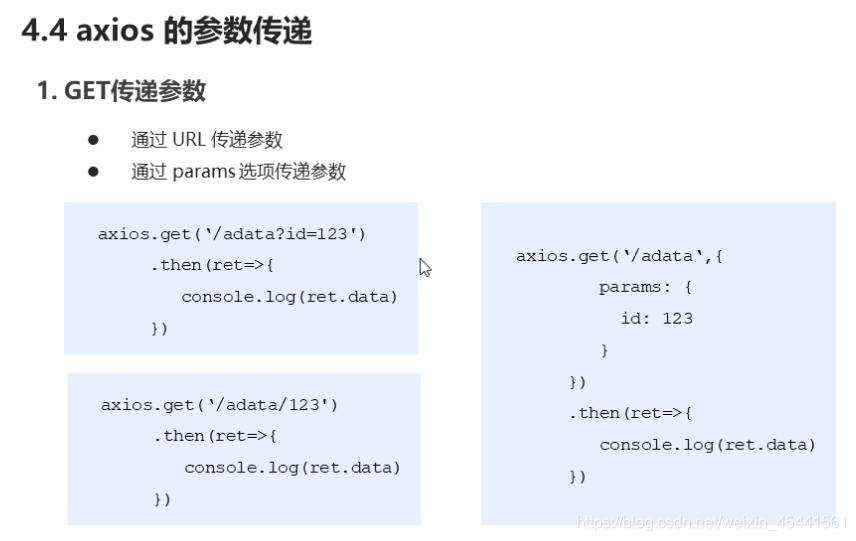
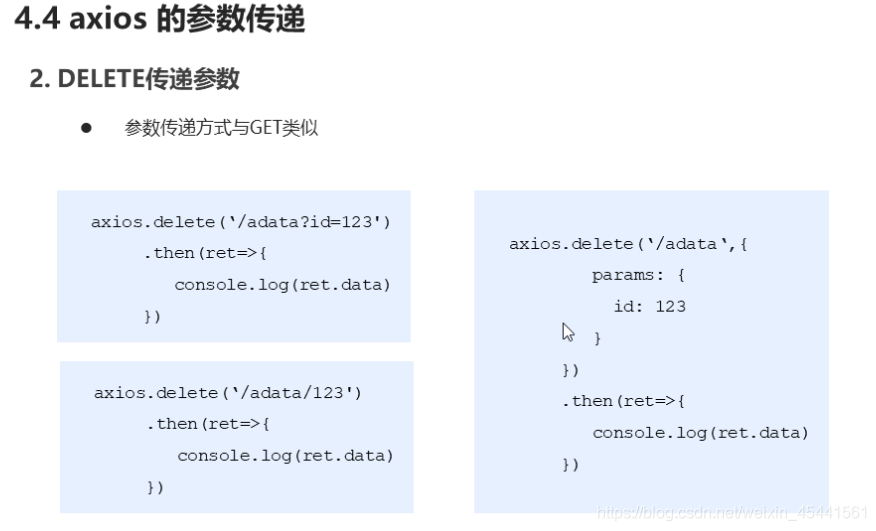
# 1. 发送get 请求
axios.get('http://localhost:3000/adata').then(function(ret){
# 拿到 ret 是一个对象 所有的对象都存在 ret 的data 属性里面
console.log(ret)
})
# 2. get 请求传递参数
# 2.1 通过传统的url 以 ? 的形式传递参数
axios.get('http://localhost:3000/axios?id=123').then(function(ret){
console.log(ret.data)
})
# 2.2 restful 形式传递参数
axios.get('http://localhost:3000/axios/123').then(function(ret){
console.log(ret.data)
})
# 2.3 通过params 形式传递参数
axios.get('http://localhost:3000/axios', {
params: {
id: 789
}
}).then(function(ret){
console.log(ret.data)
})
#3 axios delete 请求传参 传参的形式和 get 请求一样
axios.delete('http://localhost:3000/axios', {
params: {
id: 111
}
}).then(function(ret){
console.log(ret.data)
})
# 4 axios 的 post 请求
# 4.1 通过选项传递参数默认传递的json格式的数据
axios.post('http://localhost:3000/axios', {
uname: 'lisi',
pwd: 123
}).then(function(ret){
console.log(ret.data)
})
# 4.2 通过 URLSearchParams 传递参数
var params = new URLSearchParams();
params.append('uname', 'zhangsan');
params.append('pwd', '111');
axios.post('http://localhost:3000/axios', params).then(function(ret){
console.log(ret.data)
})
#5 axios put 请求传参 和 post 请求一样
axios.put('http://localhost:3000/axios/123', {
uname: 'lisi',
pwd: 123
}).then(function(ret){
console.log(ret.data)
})
后台服务index.js
const express = require('express')
const app = express()
const bodyParser = require('body-parser')
// 处理静态资源
app.use(express.static('public'))
// 处理参数
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
// 设置允许跨域访问该服务
app.all('*', function(req, res, next) {
res.header('Access-Control-Allow-Origin', '*')
res.header('Access-Control-Allow-Methods', 'PUT, GET, POST, DELETE, OPTIONS')
res.header('Access-Control-Allow-Headers', 'X-Requested-With')
res.header('Access-Control-Allow-Headers', 'Content-Type')
res.header('Access-Control-Allow-Headers', 'mytoken')
next()
})
// 路由接口
app.get('/adata', (req, res) => {
res.send('Hello axios!')
})
app.get('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
app.get('/axios/:id', (req, res) => {
res.send('axios get (Restful) 传递参数' + req.params.id)
})
app.delete('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
app.delete('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
app.post('/axios', (req, res) => {
res.send('axios post 传递参数' + req.body.uname + '---' + req.body.pwd)
})
app.put('/axios/:id', (req, res) => {
res.send('axios put 传递参数' + req.params.id + '---' + req.body.uname + '---' + req.body.pwd)
})
// 启动监听
app.listen(3000, () => {
console.log('服务器running...')
})
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<script type="text/javascript" src="js/axios.js"></script>
<script type="text/javascript">
/*
axios请求传递
*/
//axios基本用法
axios.get('http://localhost:3000/adata').then(function(ret) {
// 注意data属性是固定的用法,用于获取后台的实际数据
console.log(ret.data)
//ret拿到所有数据
// console.log(ret)
})
app.get('/adata', (req, res) => {
res.send('Hello axios!')
})
// axios get请求传参问号
axios.get('http://localhost:3000/axios?id=123').then(function(ret) {
console.log(ret.data)
})
app.get('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
// axios get请求传参restful 形式传递参数
axios.get('http://localhost:3000/axios/123').then(function(ret) {
console.log(ret.data)
})
app.get('/axios/:id', (req, res) => {
res.send('axios get (Restful) 传递参数' + req.params.id)
})
// axios get请求传参通过params 形式传递参数
axios.get('http://localhost:3000/axios', {
params: {
id: 789
}
}).then(function(ret) {
console.log(ret.data)
})
app.get('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
// axios delete 请求传参
axios.delete('http://localhost:3000/axios', {
params: {
id: 111
}
}).then(function(ret) {
console.log(ret.data)
})
app.delete('/axios', (req, res) => {
res.send('axios get 传递参数' + req.query.id)
})
// axios post 请求传参默认传递的json格式的数据
axios.post('http://localhost:3000/axios', {
uname: 'lisi',
pwd: 123
}).then(function(ret) {
console.log(ret.data)
})
app.post('/axios', (req, res) => {
res.send('axios post 传递参数' + req.body.uname + '---' + req.body.pwd)
})
// post通过 URLSearchParams 传递参数表单格式 拿到的是键值对
var params = new URLSearchParams();
params.append('uname', 'zhangsan');
params.append('pwd', '111');
axios.post('http://localhost:3000/axios', params).then(function(ret) {
console.log(ret.data)
})
app.post('/axios', (req, res) => {
res.send('axios post 传递参数' + req.body.uname + '---' + req.body.pwd)
})
// axios put 请求传参
axios.put('http://localhost:3000/axios/123', {
uname: 'lisi',
pwd: 123
}).then(function(ret) {
console.log(ret.data)
})
app.put('/axios/:id', (req, res) => {
res.send('axios put 传递参数' + req.params.id + '---' + req.body.uname + '---' + req.body.pwd)
})
</script>
</body>
</html>