背景:目前业务主要是在shopify平台上,需要经常性的进行下单操作,shopify侧对测试店铺有限制(draft order次数是50次),当达到这个次数后,在shopfy的前端页面下单的操作就会被shopify拦截,为了提升测试效率,所以写了shopify的一键创建订单的脚本;
1.首先创建一个shopify的测试店铺
这个过程不会的自己百度
2.设置店铺的API权限并获取token
进入到Apps>Develop apps

分配API scope
点击create an app,填写任意app name如test后确认;
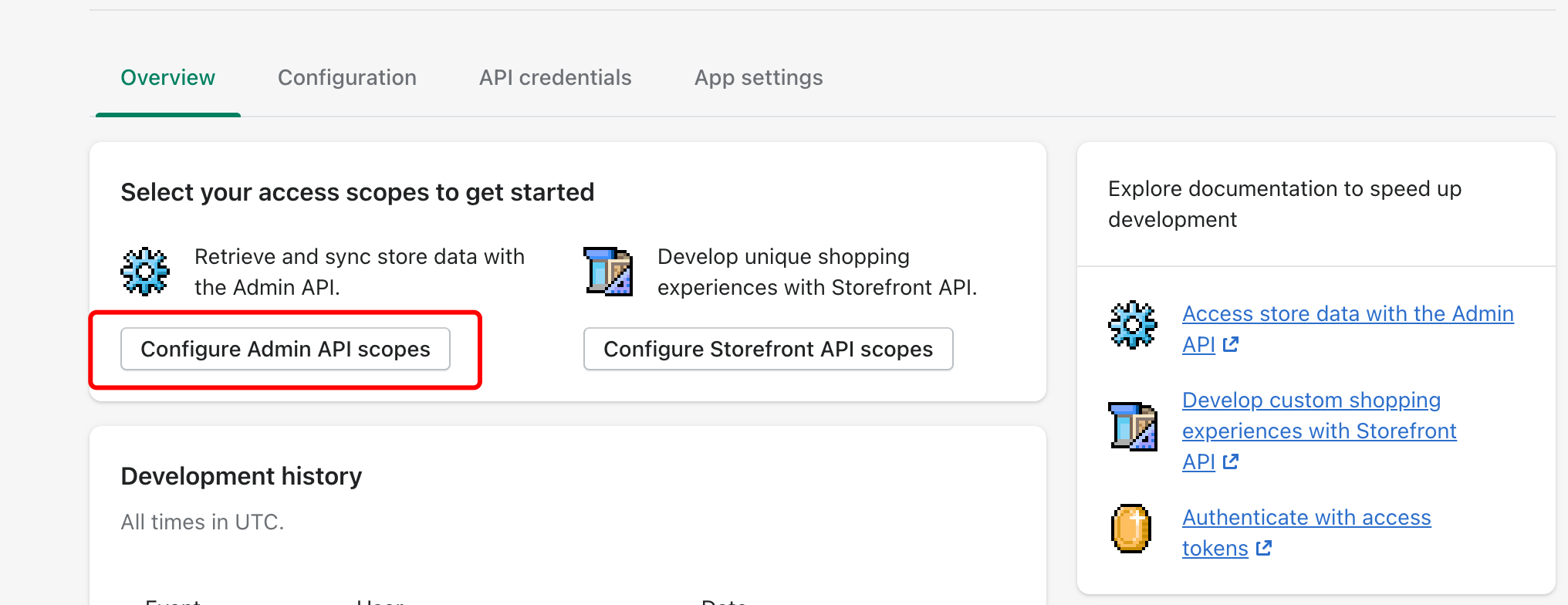
不懂需要勾选哪些权限的那就简单点全部勾选,需要其他的功能可以查阅Shopify API Document ;
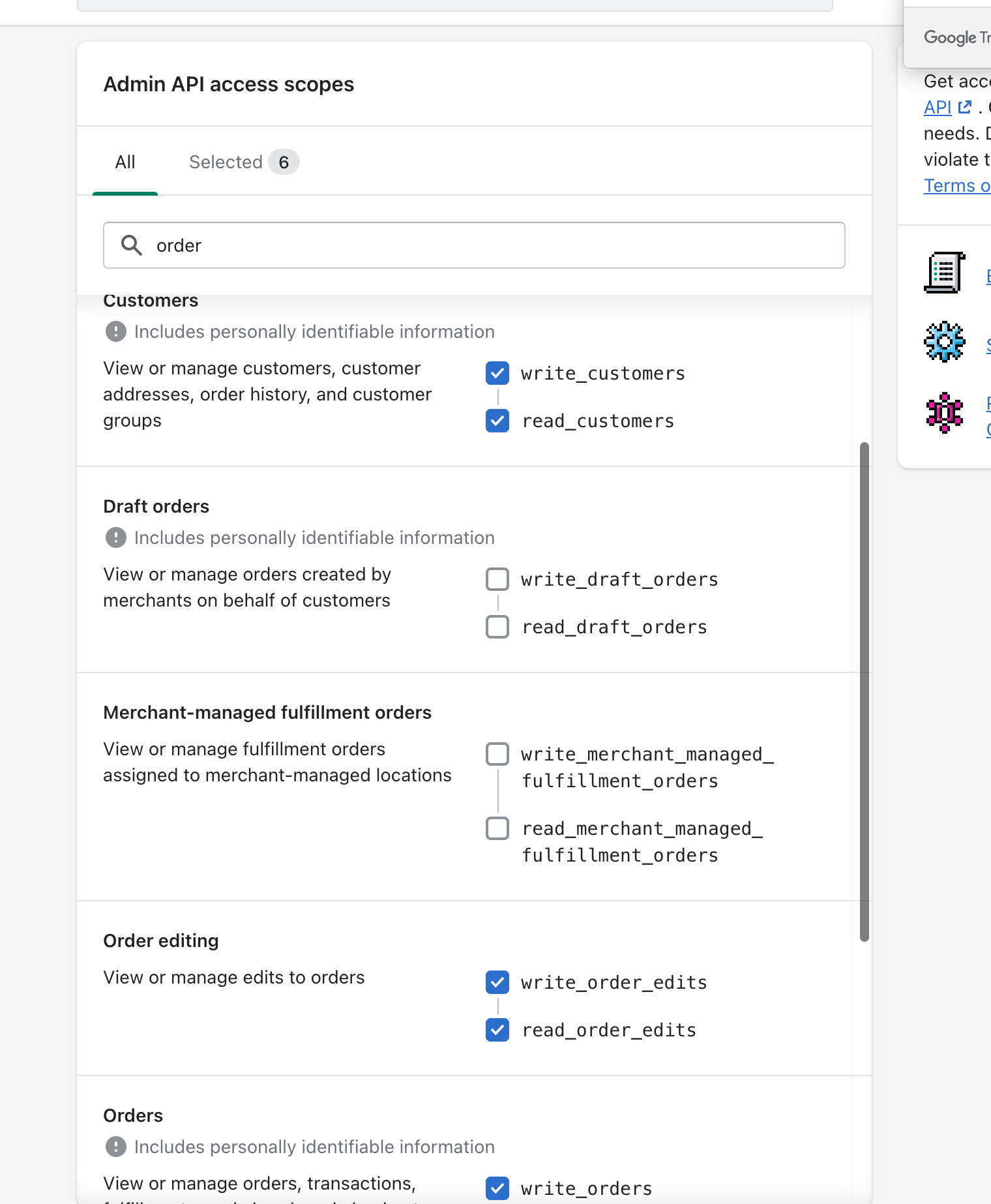
查看 api token,千万记住:Reveal token once 查看token注意保存只展示一次,这个token后续需要:
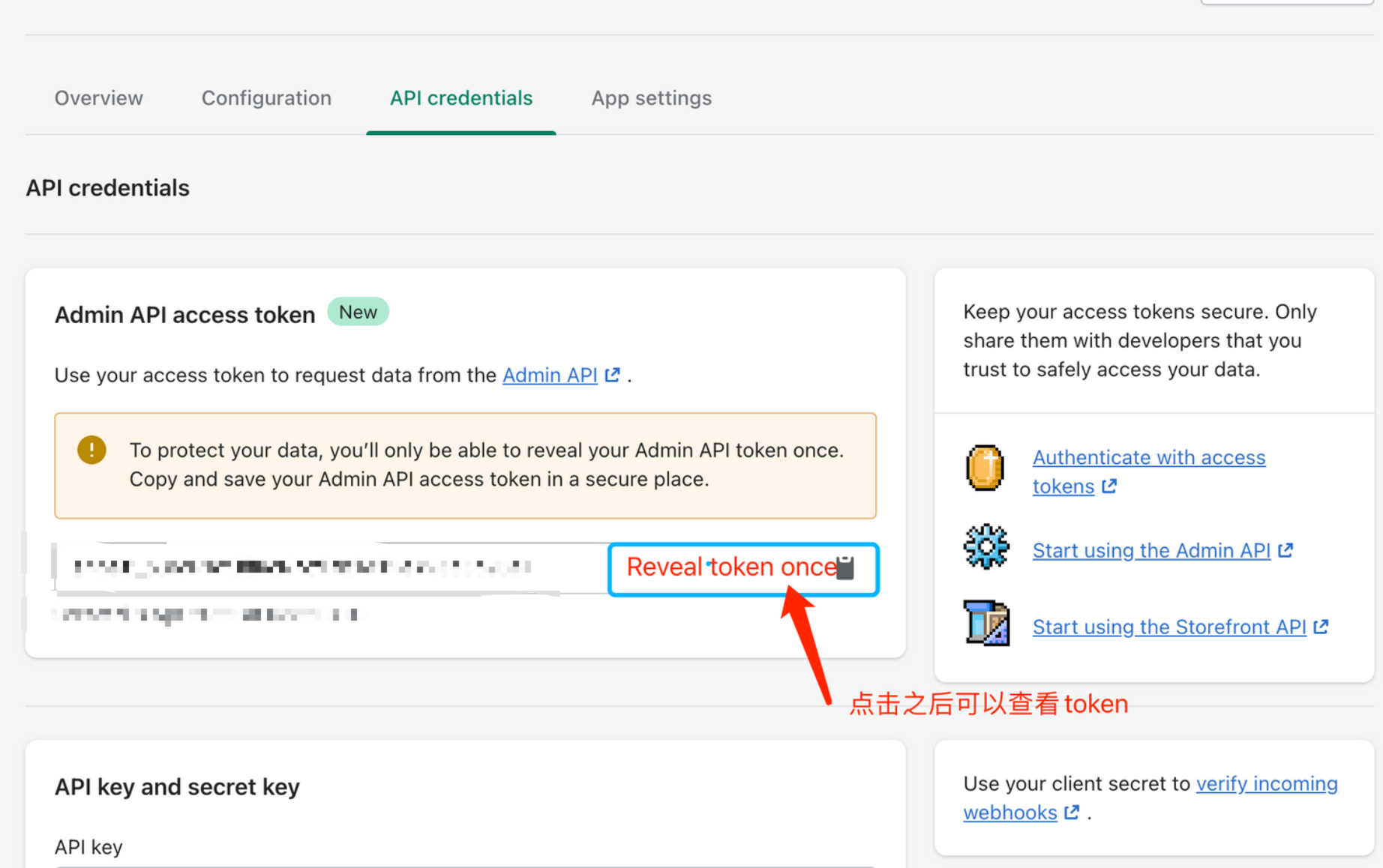
@Configuration
@Getter
@Setter
@Component
public class Line_itemsBean {
private String variant_id;
private Integer quantity;
}
给出的实例如下:
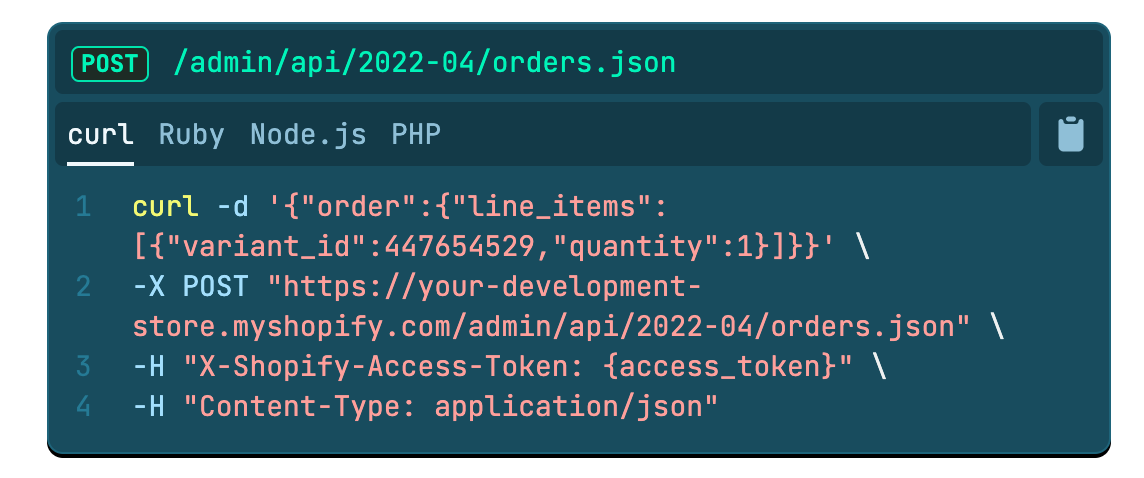
按照实例上面只需要传入对应店铺下面的variant_id,token即可,注意请求地址中需要修改成你自己的店铺地址,token需要带到请求头中。但是这种情况下生成的订单是没有支付的状态,并不能满足我现在要测的业务线的需求,所以
接下来演示下我的实现方法:
Line_itemsBean
@Configuration
@Getter
@Setter
@Component
public class Line_itemsBean {
private String variant_id;
private Integer quantity;
}
OrderBean
@Configuration
@Getter
@Setter
@Component
public class OrderBean {
private String email;
private String financial_status = "pending";
private List<Line_itemsBean> line_items;
private AddressBean shipping_address;
private List<TransactionsBean> transactions;
}
ShopifyBean
@Configuration
@Component
@Data
public class StoreBean {
private String storeName;
private String shopifyStoreUrl;
private String token;
List<Line_itemsBean> line_items;
public String getVariantIid(Integer index) {
return line_items.get(index).getVariant_id();
}
public Integer getVariantIidQuantity(Integer index) {
return line_items.get(index).getQuantity();
}
}
TransactionsBean
@Configuration
@Component
@Data
public class TransactionsBean {
private String kind;
private String status;
private String amount;
private String currency;
private Long parent_id;
private String test;
}
配置文件如下:
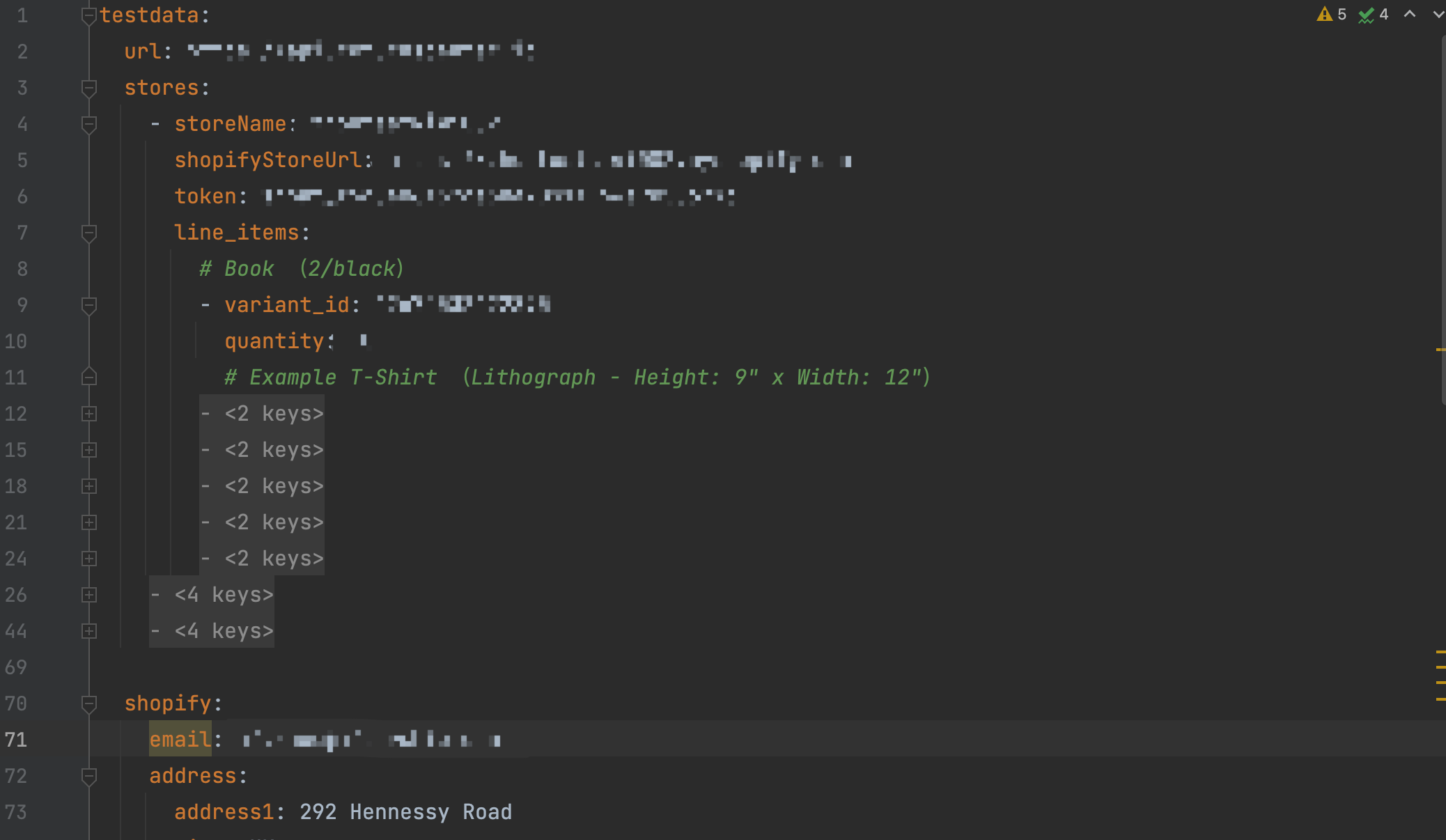
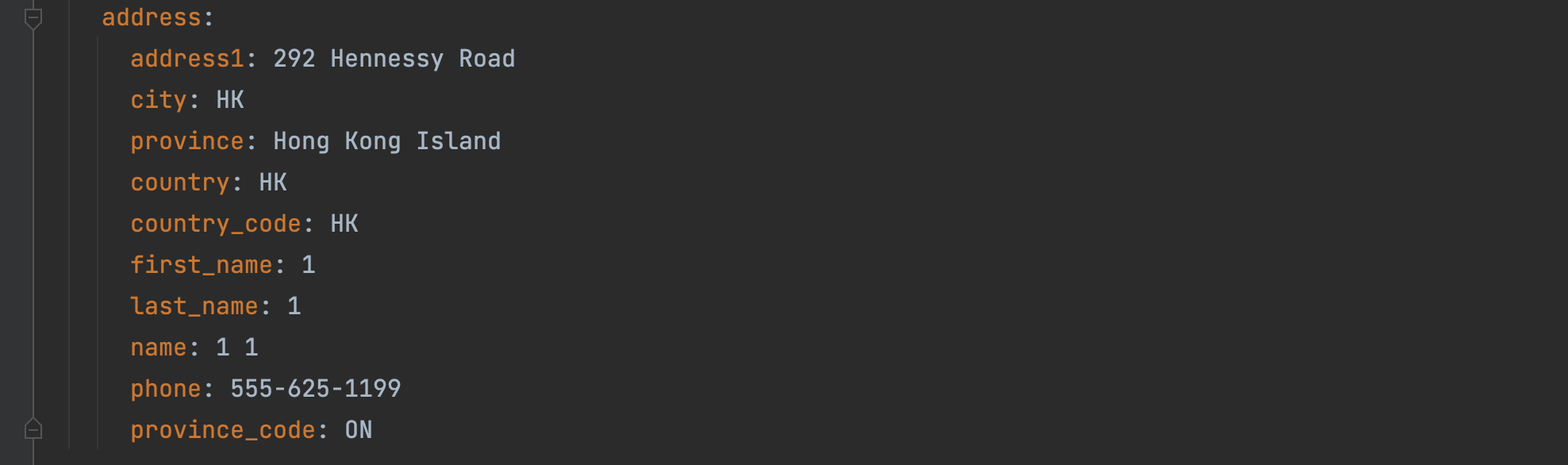
实现类:
@Component
public class ShopifyFlow extends AbstractTest {
public Response newShopifyOrder(StoreBean store,AddressBean address,Integer itemQuantity){
OrderBean orderBean = new OrderBean();
ArrayList<Line_itemsBean> itemList = new ArrayList<>();
for (int i = 0; i < itemQuantity; i++){
Line_itemsBean itemsBean = new Line_itemsBean();
itemsBean.setVariant_id(store.getVariantIid(i));
itemsBean.setQuantity(store.getVariantIidQuantity(i));
itemList.add(itemsBean);
}
//装填TransactionsBean对象,支付订单需要
ArrayList<TransactionsBean> transactionsBeanArrayList = new ArrayList<>();
TransactionsBean transactionsBean = new TransactionsBean();
transactionsBean.setKind("authorization");
transactionsBean.setAmount("10000");
transactionsBean.setStatus("success");
transactionsBeanArrayList.add(transactionsBean);
orderBean.setEmail("test@qq.com");
orderBean.setLine_items(itemList);
orderBean.setShipping_address(address);
orderBean.setTransactions(transactionsBeanArrayList);
HashMap orderList = new HashMap();
orderList.put("order",orderBean);
return HttpUtil.shopifyPost(store.getShopifyStoreUrl() + "/admin/api/2022-04/orders.json" , store.getToken(), JSON.toJSONString(orderList));
}
public Response getTransactionId(@NotNull StoreBean store, Long orderId){
return HttpUtil.get(store.getShopifyStoreUrl() + "/admin/api/2022-04/orders/" + orderId + "/transactions.json",store.getToken());
}
public Response payTestOrder(StoreBean store,Long orderId,String currency,String amount,Long parent_id){
TransactionsBean transactionsBean = new TransactionsBean();
transactionsBean.setCurrency(currency);
transactionsBean.setAmount(amount);
transactionsBean.setKind("capture");
transactionsBean.setParent_id(parent_id);
transactionsBean.setTest("true");
HashMap transaction = new HashMap();
transaction.put("transaction",transactionsBean);
return HttpUtil.shopifyPost(store.getShopifyStoreUrl() + "/admin/api/2022-04/orders/" + orderId + "/transactions.json", store.getToken(), JSON.toJSONString(transaction));
}
}
执行测试代码:
@Test(description = "生成订单并支付")
public void getTesOrder(){
//获取配置文件中的第三个店铺的数据
StoreBean store = getStore(2);
Response response = shopifyFlow.newShopifyOrder(store,address,5);
//下单成功后判断返回的订单id不为空
assertThatJson(response.jsonPath().get("order.id")).isNotNull();
//获取到接口返回的orderId,checkout currency,总金额
Long orderId = response.jsonPath().get("order.id");
String currency = response.jsonPath().get("order.currency");
String amount = response.jsonPath().get("order.current_subtotal_price");
//根据orderId获取transactionId
response = shopifyFlow.getTransactionId(store,orderId);
assertThatJson(response.jsonPath().get("transactions[0].id")).isNotNull();
Long transactionId = response.jsonPath().get("transactions[0].id");
//支付测试订单
response = shopifyFlow.payTestOrder(store,orderId,currency,amount,transactionId);
}