1. 准备工作
- Vue项目(以@vue/cli 4.5.13为例)
2. 实现Stomp
2.1 安装Stompjs
npm install stompjs
2.2 配置Stomp
import SockJS from "sockjs-client";
import Stomp from "stompjs";
export class StompHandle {
/**
* 创建一个通讯通道
* @param proxyUrl
* @param headers
*/
constructor(proxyUrl,headers){
this.headers = headers;
this.proxyUrl = proxyUrl;
this.stompClient = null;
this.connectedFunList = [];
this.connection(this.headers);
this.subscribeIDMap = new Map();
}
/**
* 轮训连接模式,当网络发生异常断网或终止时,尝试继续连接直至网络继续正常通讯
* @constructor
*/
TryConnectionWebSocket(){
let that = this;
// 断开重连机制,尝试发送消息,捕获异常发生时重连
let timer = setTimeout(() => {
console.log("协同中断,正在重新连接...");
that.connection(this.headers);
}, 5000);
}
/**
* 连接后台ws
* @param headers
*/
connection(headers) {
// 建立连接对象
let socket = new SockJS(this.proxyUrl + "/gs-guide-websocket");
// 获取Stomp子协议的客户端对象
this.stompClient = Stomp.over(socket);
// 关闭调试输出
this.stompClient.debug = null;
let _this = this;
// 向服务器发起webSocket连接
this.stompClient.connect(
params,
function (res) {
console.log("建立连接成功!")
for (let i = 0; i < _this.connectedFunList.length; i++){
let fun = _this.connectedFunList[i];
fun(_this.stompClient);
}
},
function (err) {
_this.TryConnectionWebSocket();
}
);
}
/**
* 初始化连接和监听
* @param connectedFun
*/
listenerConnected(connectedFun){
this.connectedFunList.push(connectedFun);
}
/**
* 断开连接
* 慎用! 只有在关闭程序时,调用该接口
*/
disconnect(){
let _this = this;
if (this.stompClient != null && this.stompClient != undefined){
this.stompClient.disconnect(function () {
_this.stompClient.send("test"); // 关闭测试
});
}
}
/**
* 添加订阅接口
* @param id
* @param url
* @param fun
*/
addSubscribe(id, url, fun){
let data = this.stompClient.subscribe(url, fun);
let arr = this.subscribeIDMap.get(id);
if (!arr){
arr = [];
this.subscribeIDMap.set(id,arr);
}
arr.push(data.id)
}
/**
* 注销订阅接口
* @param id
*/
unSubscribe(id){
let subscribeIDList = this.subscribeIDMap.get(id);
if (subscribeIDList){
for (let i = 0; i < subscribeIDList.length; i++) {
let data = subscribeIDList[i];
this.stompClient.unsubscribe(data);
}
}
}
isConnect(){
return this.stompClient.connected;
}
}
StompHandle.getInstance = (function (proxyUrl, headers) {
let stompHandle;
return function (proxyUrl, headers) {
if (!stompHandle && proxyUrl){
stompHandle = new StompHandle(proxyUrl, headers);
}
return stompHandle;
}
})();
2.3 订阅Stomp接口
<script>
import {StompHandle} from "../js/stomp/StompHandle";
export default {
data(){
return{
stompHandle: null,
}
},
created() {
this.initStomp();
},
methods: {
initStomp(){
let headers = {
userId: "user01"
}
// "http://127.0.0.1:8081"为需要进行通讯的服务器端口,headers为客户端的认证信息
this.stompHandle = StompHandle.getInstance("http://127.0.0.1:8081", headers);
this.stompHandle.listenerConnected((stompClient) => {
this.initStompListenerPort(stompClient);
});
},
initStompListenerPort(stompClient){
// 添加一
stompClient.subscribe(
"/topic/webSocketTest/test1",
(msg) => {
console.log(msg)
}
)
// 添加二
this.stompHandle.addSubscribe("test2",
"/topic/webSocketTest/test2",
(msg) => {
console.log(msg)
}
)
// 删除
this.stompHandle.unSubscribe("test2")
}
}
}
</script>
2.4 WebSocket配置成功
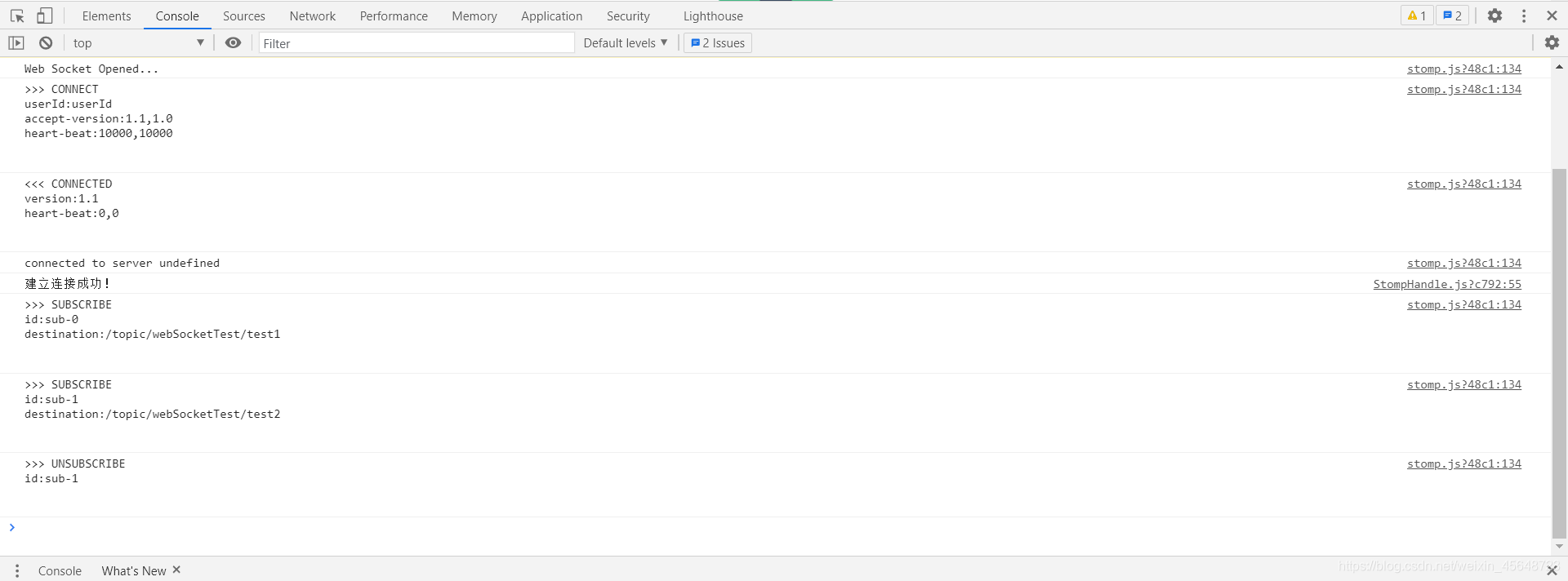
2.5 后端实现
WebSocket(1)——SpringBoot