蒙特科勒法(概率法)求pi
所需注意
主要思想就是生成随机数,但是注意C语言中rand()不是线程安全的函数-多线程应使用rand_r()!!!
原理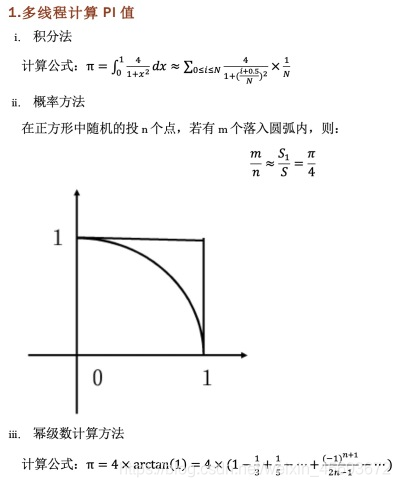
采用图中第二种方法
代码如下
//
// pi_2.c
// pi
// 蒙特科勒法
// Created by Yibin on 2021/3/28.
// Copyright © 2021 Yibin. All rights reserved.
//
#include <iostream>
#include <pthread.h>
#include <iomanip>
#include <ctime>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
double pi = 0.0;
int N,T;
pthread_mutex_t mut;
//线程入口函数
void *thread_function(void *arg){
unsigned int seed = 123;
int sample = N/T;
int demo = 0;
for(int i=0;i<sample;i++){
double x = 1.0*rand_r(&seed)/RAND_MAX;
double y = 1.0*rand_r(&seed)/RAND_MAX;
if(x*x + y*y < 1.0){
demo++;
}
}
pthread_mutex_lock(&mut);
pi = (pi + demo);
pthread_mutex_unlock(&mut);
return NULL;
}
int main(int argc, const char * argv[]) {
N = atoi(argv[1]);
T = atoi(argv[2]);
pthread_t thread[T];
int x[T];
for(int i=0;i<T;i++){
x[i] = i;
pthread_create(&thread[i], NULL, thread_function, &x[i]);
}
for(int i=0;i<T;i++){
pthread_join(thread[i], NULL);
}
pi = 4*pi/N;
printf("pi is %f",pi);
exit(0);
}