题目:
- 给定两个数,求这两个数的最大公约数
- 求10 个整数中最大值
- 分数求值:算1/1-1/2+1/3-1/4+1/5 …… + 1/99 - 1/100 的值
- 打印100~200之间的素数
- 实现函数判断year是不是润年
- 实现一个函数,打印乘法口诀表
- 写一个函数打印arr整型一维数组的内容,不使用数组下标,使用指针
- 实现一个函数来交换两个整数的内容
- 递归方式实现打印一个整数的每一位
1.给定两个数,求这两个数的最大公约数
例如:
输入:20 40
输出:20
#include <stdio.h>
#include <windows.h>
#pragma warning (disable:4996) //scanf 编译通过
int main()
{
//给定两个数,求这两个数的最大公约数
int a = 0;
int b = 0;
int c = 0;
printf("请输入两个整数:");
scanf("%d%d", &a, &b);
while (c = a%b)
{
a = b;
b = c;
}
printf("它们的最大公约数是:%d\n", b);
system("pause");
return 0;
}
运行结果
2.求10 个整数中最大值
#include <stdio.h>
int main()
{
//求10 个整数中最大值
int arr[10] = { 3, 34, 56, 3, 45, 87, 45, 32, 12, 46 };
int max = arr[0]; //先设第一个数是最大值
int a = 0;
for (int a = 0; a < 10; a++)
{
if (max > arr[a]) //判断后边的数是否大于前面的数
{
continue; //若后边的数大于前面的数 则执行for循环
}
else
{
max = arr[a]; //反之 则确定出最大值
}
}
printf("max=%d\n", max); //打印输出最大值
system("pause");
return 0;
}
运行结果
3.分数求值
计算1/1-1/2+1/3-1/4+1/5 …… + 1/99 - 1/100 的值,并打印出结果
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include <windows.h>
int main()
{
//计算1/1-1/2+1/3-1/4+1/5 …… + 1/99 - 1/100 的值,打印出结果
int i = 0;
int n = 0;
float sum = 0.0; //浮点数
for (i = 0; i <= 100; i++)
{
sum = sum + ((1 / (n + 1.0))*(pow(-1, n))); // pow :求(-1)^n
++n;
}
printf("sum=%f\n", sum);
system("pause");
return 0;
}
运行结果
4.打印100~200之间的素数 (分文件)
头文件 test.h
#ifndef __TEST_H__
#define __TEST_H__
#include <stdio.h>
#include <Windows.h>
extern void ShowPrime(int start, int end); //确定区间
#endif
源文件 main.c
#include "test.h"
int main()
{
ShowPrime(100, 200); //确定打印的区间 [100,200] 可以任意选择区间哦~
system("pause");
return 0;
}
源文件 test.c
#include "test.h"
static int IsPrime(int n)
{
int i = 2;
for (; i < n/2 ; i++) // n/2 是为了防止重复运算
{
if (n % i == 0)
{
return 0;
}
}
return 1;
}
void ShowPrime(int start, int end) //任意确定要打印的区间
{
for (; start <= end; start++)
{
if (IsPrime(start))
{
printf("%d ", start);
}
}
printf("\n");
}
运行结果
5.实现函数判断year是不是润年 (分文件)
头文件 test.h
#ifndef __TEST_H__
#define __TEST_H__
#include <stdio.h>
#include <Windows.h>
extern void ShowLeap(int start, int end);
#endif
源文件main.c
#include "test.h"
int main()
{
ShowLeap(1000,2000); //判断的区间[1000,2000]
system("pause");
return 0;
}
源文件 test.c
#include "test.h"
//实现函数判断year是不是润年
static int IsLeap(int year)
{
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
//4年一闰,百年不闰,400年再闰
}
void ShowLeap(int start, int end) //确定判断的区间
{
for (int year = start; year <= end; year++)
{
if (IsLeap(year))
{
printf("%d ", year);
}
}
printf("\n");
}
运行结果
6.实现一个函数,打印乘法口诀表。
头文件 test.h
#ifndef __TEST_H__
#define __TEST_H__
#include <stdio.h>
#include <Windows.h>
extern void PfMulTable(int base);
#endif
源文件 main.c
#include "test.h"
int main()
{
PfMulTable(9);
system("pause");
return 0;
}
源文件 test.c
#include "test.h"
void PfMulTable(int base)
{
for (int i = 1; i <= base; i++)
{
for (int j = 1; j <= i; j++)
{
printf("%d*%d=%d ", j, i, j*i);
}
printf("\n");
}
}
运行结果
7.写一个函数打印arr整型一维数组的内容,不使用数组下标,使用指针。
#include <stdio.h>
#include <Windows.h>
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 67 }; //arr是一个整型一维数组
int *p = arr;
int length = sizeof(arr) / sizeof(arr[0]); //求数组长度 arr[0] 每个数组至少有一个元素
for (int i = 0; i < length; i++) //数组下标从0开始
{
printf("%d ", *p);
++p;
}
system("pause");
return 0;
}
运行结果
8.实现一个函数来交换两个整数的内容
#define _CRT_SECURE_NO_WARNINGS //scanf 编译通过
#include <stdio.h>
#include <windows.h>
#include <stdlib.h>
void ChangeNum(int* x, int* y)
{
int temp = 0; //定义了临时变量
temp = *x; //交换
*x = *y; //交换
*y = temp; //交换
}
int main()
{
int* a = 0;
int* b = 0;
printf("Please enter two number (a b)# "); //用户输入两个整型数
scanf("%d %d", &a, &b);
ChangeNum(&a, &b); //交换后的数
printf("After perform the swap: a=%d b=%d \n", a, b);
system("pause");
return 0;
}
运行结果
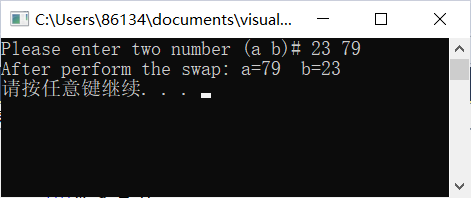
9.递归方式实现打印一个整数的每一位
#define _CRT_SECURE_NO_WARNINGS //scanf 编译通过
#include <stdio.h>
#include <stdlib.h>
int Digit_Every(int num)
{
if (num > 9)
{
Digit_Every(num / 10);
}
printf("%d ", num % 10);
}
int main()
{
int num;
printf("Please input number: ");
scanf("%d", &num);
Digit_Every(num);
system("pause");
return 0;
}
运行结果