#include <iostream>
#include <cmath>
#include <algorithm>
#include <iomanip>
#include <numeric>
#include <sstream>
#include <string.h>
using namespace std;
#define LIST_INIT_SIZE 100
#define LISTINCREMENT 10
typedef struct{
int *elem;
int length;
int listsize;
}SqList;
typedef struct LNode{
int data;
struct LNode *next;
}LNode, *LinkList;
//2.10
bool DeleteK(SqList &a, int i,int k){
if(i<1||k<0||i+k>a.length){
return false;
}
else{
for(int co=0;co<k;co++){
a.elem[i-1+co]=a.elem[i-1+co+k];
}
a.length-=k;
}
return true;
}
//2.11
SqList insert_x(SqList a,int x){
SqList b;
b.length=0;
b.listsize=a.listsize+1;
memset(b.elem,0,sizeof(b.elem));
int f1=0;
for(int i=0,j=0;i<a.length;i++,j++){
if(a.elem[i]>x&&f1==0){
b.elem[j]=x;
b.length++;
f1=1;
}
b.elem[j]=a.elem[i];
b.length++;
}
return b;
}
//2.12
int comp_a_b(SqList a,SqList b){
int len=a.length<b.length?a.length:b.length;
for(int i=0;i<len;i++){
if(a.elem[i]<b.elem[i]){
return -1;
}
else if(a.elem[i]>b.elem[i]){
return 1;
}
}
if(a.length==b.length){
return 0;
}
else{
if(a.length<b.length){
return -1;
}
else{
return 1;
}
}
}
//2.13
int LOCATE(LinkList L,int x){
int cou=1;
LNode *p;
p=L->next;
while(1){
if(p!=NULL){
if(p->data!=x){
cou++;
p=p->next;
}
else{
return cou;
}
}
else{
return -1;
}
}
}
//2.14
int LENGTH(LinkList L){
int len=0;
LNode *p=L->next;
while(p!=NULL&&p->next!=NULL){
len++;
}
return len;
}
//2.15
LNode *hc;
void link_ha_hb(LinkList &a, LinkList b){
LNode *ha=a;
hc=ha;
while(ha->next!=NULL){
ha=ha->next;
}
ha->next=b->next;
}
//2.16
bool DeleteAndSub(LinkList la,LinkList lb, int i,int j ,int len){
if(i<0||j<0||len<0){
return false;
}
LNode *p=la,*q,*prep,*preq;
int k=1;
while(k<i){
prep=p;
p=p->next;k++;
}
q=p;
k=1;
while(k<=len){
preq=q;
q=q->next;k++;
}
LNode *s=lb,*sn;
k=1;
while(k<j-1){
s=s->next;k++;
}
sn=s->next;
s->next=p;
prep->next=q;
preq->next=sn;
return true;
}
//2.17
void INSERT(LinkList &L,int i,int b){
LNode *s=new LNode;
s->data=b;
LNode *p=L;
if(i==1){
s->next=p;
return;
}
int k=1;
while(k<i-1){
p=p->next;
k++;
}
s->next=p->next;
p->next=s;
return;
}
//2.18
void DELETE(LinkList &L,int i){
LNode *p=L;
if(i==1){
L=L->next;
p->next=NULL;
delete p;
return;
}
int k=1;
while(k<i-1){
if(p==NULL){
return;
}
p=p->next;
k++;
}
LNode *q=p->next;
p->next=q->next;
q->next=NULL;
delete q;
return;
}
//2.19
void DELETE_mink_maxk(LinkList &L,int mink,int maxk){
LNode *p=L->next,*pre=L,*q;
while(p!=NULL){
if(p->data<mink){
pre=p;
p=p->next;
}
else if(p->data>maxk){
break;
}
else{
q=p;
p=p->next;
pre->next=p;
q->next=NULL;
delete q;
}
}
}
//2.20
void DELETE_SAME(LinkList &L){
LNode *p=L->next,*q,*pre=L;
int k=p->data;
while(p!=NULL){
pre=p;
p=p->next;
if(p->data!=k){
k=p->data;
}
else{
q=p;
p=p->next;
pre->next=p;
q->next=NULL;
delete q;
k=p->data;
}
}
}
//2.21
void REVERSE1(SqList &s){
int k=s.length/2,temp;
for(int i=0;i<k;i++){
temp=s.elem[i];
s.elem[i]=s.elem[s.length-1-i];
s.elem[s.length-1-i]=temp;
}
}
//2.22
void REVERSE2(LinkList &L){
LNode *p=L->next,*q;
L->next=NULL;
while(p!=NULL){
q=p->next;
p->next=L->next;
L->next=p;
p=q;
}
}
//2.23
void merge_ab_c1(LinkList &a,LinkList &b, LinkList &c){
LNode *la=a->next,*lb=b->next,*qa,*qb,*preb;
c=a;
while(la!=NULL&&lb!=NULL){
preb=lb;
qa=la->next;
qb=lb->next;
lb->next=qa;
la->next=lb;
la=qa;
lb=qb;
}
if(lb!=NULL){
preb->next=lb;
}
lb=b;
delete lb;
}
//2.24
void merge_ab_c2(LinkList &a,LinkList &b, LinkList &c){
LNode *la=a->next,*lb=b->next,*temp;
a->next=NULL;
while(la!=NULL&&lb!=NULL){
if(la->data<lb->data){
temp=la->next;
la->next=a->next;
a->next=la;
la=temp;
}
else{
temp=lb->next;
lb->next=a->next;
a->next=lb;
lb=temp;
}
}
while(la!=NULL){
temp=la->next;
la->next=a->next;
a->next=la;
la=temp;
}
while(lb!=NULL){
temp=lb->next;
lb->next=a->next;
a->next=lb;
lb=temp;
}
c=a;
lb=b;
delete lb;
}
//2.25
void merge_ab_c3(SqList &a,SqList &b,SqList &c){
int i=0,sa=0,sb=0;
while(sa<a.length&&sb<b.length){
while(a.elem[sa]>b.elem[sb]&&sb<b.length){
sb++;
}
if(a.elem[sa]==b.elem[sb]){
c.elem[i]=a.elem[sa];
i++;sb++;
}
else if(a.elem[sa]<b.elem[sb]){
sa++;
}
}
}
//2.26
void merge_ab_c4(LinkList &a,LinkList &b,LinkList &c){
c=a;
LNode *la=a->next,*lb=b->next,*temp,*pa=a,*pb=b;
while(la!=NULL&&lb!=NULL){
if(la->data<lb->data){
temp=la;
la=la->next;
pa->next=la;
temp->next=NULL;
delete temp;
}
else if(la->data==lb->data){
temp=lb;
pb->next=temp->next;
lb=lb->next;
temp->next=NULL;
delete temp;
pa=la;
la=la->next;
}
else if(la->data>lb->data){
temp=lb;
lb=lb->next;
pb->next=lb;
temp->next=NULL;
delete temp;
}
}
while(la!=NULL){
temp=la;
la=la->next;
pa->next=la;
temp->next=NULL;
delete temp;
}
while(lb!=NULL){
temp=lb;
lb=lb->next;
pb->next=lb;
temp->next=NULL;
delete temp;
}
lb=b;
delete lb;
}
//2.27(1)
void merge_ab_c31(SqList &a,SqList &b,SqList &c){
int i=0,sa=0,sb=0;
while(sa<a.length&&sb<b.length){
while(a.elem[sa]>b.elem[sb]&&sb<b.length){
sb++;
}
if(a.elem[sa]==b.elem[sb]){
if(a.elem[sa]==c.elem[i-1]){
sa++;sb++;
}
else{
c.elem[i]=a.elem[sa];
i++;sb++;
}
}
else if(a.elem[sa]<b.elem[sb]){
sa++;
}
}
}
//2.27(2)
void merge_ab_c32(SqList &a,SqList &b){
int i=0,j=0,k=0;
while(i<a.length&&j<b.length){
if(a.elem[i]<b.elem[j]){
i++;
}
else if(a.elem[i]>b.elem[j]){
j++;
}
else{
a.elem[k++]=a.elem[i];
i++;j++;
}
}
a.length=k;
}
//2.28(1)
void merge_ab_c41(LinkList &a,LinkList &b,LinkList &c){
c=a;
LNode *la=a->next,*lb=b->next,*temp,*pa=a,*pb=b;
while(la!=NULL&&lb!=NULL){
if(la->data<lb->data){
temp=la;
la=la->next;
pa->next=la;
temp->next=NULL;
delete temp;
}
else if(la->data==lb->data){
if(la->data==pa->data){
temp=la;
la=la->next;
pa->next=la;
temp->next=NULL;
delete temp;
temp=lb;
lb=lb->next;
pb->next=lb;
temp->next=NULL;
delete temp;
}
else{
temp=lb;
pb->next=temp->next;
lb=lb->next;
temp->next=NULL;
delete temp;
pa=la;
la=la->next;
}
}
else if(la->data>lb->data){
temp=lb;
lb=lb->next;
pb->next=lb;
temp->next=NULL;
delete temp;
}
}
while(la!=NULL){
temp=la;
la=la->next;
pa->next=la;
temp->next=NULL;
delete temp;
}
while(lb!=NULL){
temp=lb;
lb=lb->next;
pb->next=lb;
temp->next=NULL;
delete temp;
}
lb=b;
delete lb;
}
//2.31
void delete_pres(LNode *s){
LNode *q=s->next;
while(q->next!=s){
q=q->next;
}
s=q;
q=s->next;
s->data=q->data;
s->next=q->next;
delete q;
}
//2.32
typedef struct CircularLNode{
int data;
CircularLNode *next,*prior;
}CicularLNode,*CircularLinkedlist;
void RESET_cln(CircularLinkedlist &c){
CircularLNode *q=c->next,*pre;
while(q!=c){
pre=q;
q=q->next;
q->prior=pre;
}
}
//2.33
typedef struct LNode33{
char data;
LNode33 *next;
}LNode33,*LinkedList33;
void seg_to_3(LinkedList33 &a,LinkedList33 &b,LinkedList33 &c){
LNode33 *la=a->next,*lb=b,*lc=c,
*pa=a,*pb=b,*pc=c;
lb->next=b;lc->next=c;
while(la!=NULL){
if(isdigit(la->data)){
pa=la;
la=la->next;
}
else if(isalpha(la->data)){
LNode33 *temp;
temp=la;
la=la->next;
temp->next=lb->next;
lb->next=temp;
pb=lb;
lb=temp;
delete temp;
}
else{
LNode33 *temp;
temp=la;
la=la->next;
temp->next=lc->next;
lc->next=temp;
pc=lc;
lc=temp;
delete temp;
}
}
pa->next=a;
}
//2.34
typedef struct XorNode{
char data;
struct XorNode *LRPtr;
}XorNode, *XorPointer;
typedef struct{
XorPointer Left,Right;
}XorLinkedList;
XorPointer XorP(XorPointer p,XorPointer q){};
void visitXorList(XorLinkedList l,char a){
XorPointer q,left,right;
if(a=='l'){
q=l.Left;
left=NULL;
while(q!=NULL){
cout<<q->data;
left=q;
q=XorP(left,q->LRPtr);
}
}
else{
q=l.Right;
right=NULL;
while(q!=NULL){
cout<<q->data;
right=q;
q=XorP(right,q->LRPtr);
}
}
}
//2.35
void InsertXor(XorLinkedList &l,int len, XorPointer x){
XorPointer p,temp,left;
p=l.Left;
left=NULL;
int i=0;
if(len<=0){
return;
}
else if(len==1){
temp=XorP(left,p->LRPtr);
x->LRPtr=XorP(left,p);
p->LRPtr=XorP(temp,x);
l.Left=x;
return;
}
while(i<len-1){
left=p;
p=XorP(p->LRPtr,left);
i++;
}
XorPointer pprev=left,pp;
left=p;
p=XorP(p->LRPtr,left);
pp=XorP(p->LRPtr,p);
x->LRPtr=XorP(left,p);
left->LRPtr=XorP(pprev,x);
p->LRPtr=XorP(x,pp);
}
//2.36
void DELETEXorP(XorLinkedList &l,int len){
XorPointer p,pre,temp,pp,ppp;
int cou=1;
p=l.Left;
pre=NULL;
if(len==1){
temp=p;
p=XorP(p->LRPtr,p);
p->LRPtr=XorP(pre,p);
delete temp;
return;
}
while(cou<len-1){
pre=p;
p=XorP(pre,p->LRPtr);
cou++;
}
temp=XorP(pre,p->LRPtr);
pp=XorP(temp,temp->LRPtr);
ppp=XorP(pp,pp->LRPtr);
p->LRPtr=XorP(pre,pp);
pp->LRPtr=XorP(p,ppp);
delete temp;
}
//2.37
typedef struct DuLNode{
int data;
struct DuLNode *prior,*next;
}DuLNode,*DuLinkList;
void exchange_DuL1(DuLinkList &a,int n){
DuLNode *p=a->next,*pre=a,*q;
int cou=1;
a->next=a;
a->prior=a;
while(cou<n){
if(cou%2!=0){
q=p->next;
p->next=pre->next;
p->prior=pre;
pre=p;
p=q;
}
else{
q=p->next;
p->prior=pre->prior;
p->next=pre;
pre=p;
p=q;
}
cou++;
}
}
//2.38
typedef struct DuLNode1{
int data;
struct DuLNode1 *prior,*next;
int freq=0;
}DuLNode1,*DuLinkList1;
void sort_freq(DuLinkList1 &l){
DuLNode1 *q=l->next,*pre;
int c=0;
while(c==0){
pre=q;
q=q->next;
if(pre->freq<q->freq){
DuLNode1 *temp=l->next,*pretemp=l;
while(temp->freq>=q->freq){
pretemp=temp;
temp=temp->next;
}
pre->next=q->next;
q->next->prior=pre;
q->next=temp;
temp->prior=q;
q->prior=pretemp;
pretemp->next=q;
}
}
}
void LOCATE1(DuLinkList1 &l,int x){
DuLNode1 *q=l->next;
while(q->data!=x){
q=q->next;
}
q->freq++;
sort_freq(l);
}
//2.39
typedef struct{
int coef;
int exp;
}PolyTerm;
typedef struct{
PolyTerm *data;
int length;
}SqPoly;
void PolyInit(SqPoly &s){
PolyTerm *p;
cin>>s.length;
s.data=new PolyTerm[s.length];
p=s.data;
for(int i=0;i<s.length;i++){
cin>>p->coef;
cin>>p->exp;
p++;
}
}
int caculate_p(SqPoly s,int x){
PolyTerm *p;
p=s.data;
int m=1,sum=0;
for(int j=0;j<s.length;j++){
m=1;
for(int q=1;q<=p->exp;q++){
m*=x;
}
sum+=p->coef*m;
p++;
}
return sum;
}
//2.40
void meg(SqPoly s1,SqPoly s2,SqPoly &s3){
PolyTerm *p1,*p2,*p3;
p1=s1.data;
p2=s2.data;
p3=s3.data;
int i=0,j=0,k=0;
while(i<=s1.length&&j<=s2.length){
if(p2->exp>p3->exp){
p1->coef=p2->coef;
p1->exp=p2->exp;
p1++;
p2++;
i++;
k++;
}
else{
if(p2->exp<p3->exp){
p1->coef=-p3->coef;
p1->exp=p3->exp;
p3++;
p1++;
j++;
k++;
}
else{
p3++;
p2++;
i++;j++;
}
}
while(i<=s2.length){
p1->coef=p2->coef;
p1->exp=p2->exp;
p1++;p2++;i++;k++;
}
while(j<=s3.length){
p1->coef=-p3->coef;
p1->exp=p3->exp;
p1++;p3++;j++;k++;
}
s1.length=k;
}
}
//2.41
typedef struct PolyNode{
PolyTerm data;
struct PolyNode *next;
}PolyNode,*PolyLink;
typedef PolyLink LinkedPoly;
void cacul_derivative(LinkedPoly &s){
PolyNode *p=s,*q=s->next;
while(p!=NULL){
if(p->data.coef==0){
PolyNode *a=p;
p=p->next;
q->next=p;
delete a;
}
else{
p->data.coef=p->data.coef*p->data.exp;
p->data.exp--;
p=q;
q=q->next;
}
}
}
//2.42
void seg(PolyLink &s1,PolyLink &s2){
PolyNode *p1=s1,*p2=s2;
int i=1;
while(s1!=NULL){
if(i%2==1){
s1=s1->next;
}
else{
PolyLink *temp;
temp=s1->next;
s1->next=s2->next;
s2=s1;
s1=temp;
}
}
}
int main()
{
return 0;
}
数据结构题集 线性表
于 2023-05-05 16:40:36 首次发布
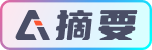