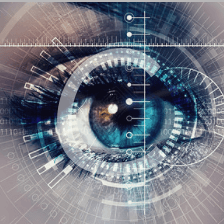
算法/OJ题目
牛客, LeetCode上的OJ题目, 以及算法题目,思想的分享
殇&璃
这个作者很懒,什么都没留下…
展开
-
LeetCode 692 : 前K个高频单词
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示带思路 vector<string> topKFrequent(vector<string>& words, int k) { //把单词和频率建立map映射关系, 单词作为key map<string, int> mp; //map的[]可以进行插入操作 //两种情况: 1.wd不存在,原创 2021-03-04 09:46:18 · 208 阅读 · 3 评论 -
牛客KY264 : 单词识别
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析这道题的思路比较简单将字符串统一变成大写或者小写将句子划分成单词把单词插入map容器遍历map容器, 输出序列即为所求3. 代码展示代码一#include<iostream>#include<string>#include<vector>#include<map>#include<functional>using namesp原创 2021-03-04 09:15:43 · 134 阅读 · 1 评论 -
LeetCode145 : 二叉树的后序遍历 (非递归)
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析后序 : 左 – 右 – 根思路和前序中序大体一致, 只是多了一个右子树是否访问的判断下面给出前序和中序的以供参考二叉树的前序遍历二叉树的中序遍历3. 代码展示class Solution {public: vector<int> postorderTraversal(TreeNode* root) { stack<TreeNode*> st;原创 2021-02-25 23:51:15 · 178 阅读 · 0 评论 -
LeetCode94 : 二叉树的中序遍历 (非递归)
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: vector<int> inorderTraversal(TreeNode* root) { stack<TreeNode*> st; vector<int> ret; TreeNode* cur = root; //当前节点原创 2021-02-25 23:22:53 · 144 阅读 · 0 评论 -
LeetCode144 : 二叉树的前序遍历 (非递归)
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: vector<int> preorderTraversal(TreeNode* root) { stack<TreeNode*> st; vector<int> ret; TreeNode* cur = root; //当前节点不为空 或者原创 2021-02-25 23:01:09 · 189 阅读 · 1 评论 -
LeetCode106 : 从中序与后序遍历序列构造二叉树
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: TreeNode* _build(vector<int>& inorder, int L, int R, vector<int>& postorder, int& idx) { //左区间 > 右区间, 说明没有元素, 是空树 if(L > R)原创 2021-02-24 10:26:40 · 120 阅读 · 0 评论 -
LeetCode105 : 从前序与中序遍历序构造二叉树
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: TreeNode* _build(vector<int>& preorder, int& idx, vector<int>& inorder, int L, int R) { //左区间>右区间, 说明没有元素, 是空树 if(L > R)原创 2021-02-24 10:24:10 · 84 阅读 · 0 评论 -
牛客JZ26 : 二叉搜索树与双向链表
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: //这里prev要传指针的引用或者二级指针, //如果只传一个指针的话, 中序遍历往下层递归没有问题, //但是往上层返回的时候, prev一直是初始值prev, 也就是底层修改的prev没有影响到上层 //所以要保证在底层修改prev要能影响到上层 void _convert(TreeNode* roo原创 2021-02-23 21:57:37 · 125 阅读 · 0 评论 -
LeetCode236 : 二叉树的最近公共祖先
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: //获取目标结点的路径 bool getPath(TreeNode* root, TreeNode* dest, deque<TreeNode*>& dq) { //空树, 返回false if(root == nullptr) return false;原创 2021-02-23 16:41:34 · 108 阅读 · 0 评论 -
LeetCode102 : 二叉树的层序遍历
文章目录1. 题目描述2. 思路分析3. 代码展示1. 题目描述2. 思路分析3. 代码展示class Solution {public: vector<vector<int>> levelOrder(TreeNode* root) { queue<TreeNode*> q; vector<vector<int>> ret; TreeNode* cur =原创 2021-02-23 09:54:40 · 89 阅读 · 2 评论 -
LeetCode606 : 根据二叉树创建字符串
文章目录1. 题目概述2. 核心分析3. 代码示例1. 题目概述2. 核心分析3. 代码示例class Solution {public: void _treeTostr(TreeNode* root, string& str) { //如果为空, 则返回 if(root == nullptr) return; //转换当前结点的数据: int --> string原创 2021-02-23 09:17:06 · 171 阅读 · 0 评论 -
OJ ----- LeetCode215 : 数组中的第K个最大元素 [优先级队列的使用]
class Solution {public: int findKthLargest(vector<int>& nums, int k) { //通过优先级队列建立大堆, 通过出堆找到元素 priority_queue<int> pq(nums.begin(), nums.end()); //将优先级队列中的前k-1个元素删除 for (int i = 0; i < k - 1; i++) {.原创 2020-11-24 16:12:17 · 121 阅读 · 0 评论 -
OJ ----- LeetCode232 : 用栈实现队列
class MyQueue {private: stack<int> pushst; stack<int> popst;public: /** Initialize your data structure here. */ MyQueue() { } /** Push element x to the back of queue. */ void push(int x) { //push栈入.原创 2020-11-24 10:45:05 · 82 阅读 · 0 评论 -
OJ ----- LeetCode225 : 用队列实现栈
class MyStack {private: queue<int> q;public: /** Initialize your data structure here. */ MyStack() { } /** Push element x onto stack. */ void push(int x) { //用队列实现栈, 入队相当于尾插 //需要把前面的元素都出队再入队, 这样处理就模拟实.原创 2020-11-24 10:08:12 · 105 阅读 · 0 评论 -
(详细图解) 逆波兰表达式
下面给出图解:下面给出代码:class Solution {public: int evalRPN(vector<string>& tokens) { stack<int> st; //循环遍历表达式 范围for for(const auto& str : tokens) { if(str == "+" || str == "-" || str == .原创 2020-11-23 22:35:24 · 3354 阅读 · 2 评论 -
OJ -----牛客JZ21 : 栈的压入弹出序列
class Solution {public: bool IsPopOrder(vector<int> pushV,vector<int> popV) { /* 思路: 利用一个栈进行模拟入栈出栈 把压入序列的数据进行入栈, 当栈顶元素等于弹出序列的元素时, 循环出栈, 直到栈顶元素不等于弹出序列的元素 循环结束时, 查看栈是否为空, 为空表示弹出顺序正确, 反之则表示错误 */ .原创 2020-11-23 16:32:48 · 140 阅读 · 0 评论 -
OJ ----- LeetCode155 : 最小栈
class MinStack {private: //用两个栈实现, 一个普通栈,进行普通操作, 一个最小栈, 放最小值 stack<int> st; stack<int> minst;public: /** initialize your data structure here. */ MinStack() { } void push(int x) { //普通栈直接入栈 .原创 2020-11-23 15:59:17 · 104 阅读 · 0 评论 -
OJ ----- 牛客JZ30 : 连续子数组的最大和
class Solution {public: int FindGreatestSumOfSubArray(vector<int> array) { //这里不能给0作为初值, 因为数组中可能全部都是负数, 导致整个算法无法正常工作 int max = array[0]; int tmp = array[0]; vector<int>::iterator it = array.begin() + 1; .原创 2020-11-14 10:06:55 · 193 阅读 · 0 评论 -
OJ ----- 牛客 JZ28 : 数组中出现次数超过一半的数字
class Solution {public: int MoreThanHalfNum_Solution(vector<int> numbers) { if (numbers.empty()) return 0; //思路: //给数组排序, 如果符合要求那么这个数字一定就是数组中间靠右的那个数字 //取到这个数字, 进行遍历验证一下是否符合要求 .原创 2020-11-14 09:19:58 · 152 阅读 · 0 评论 -
OJ ----- LeetCode 260 : 只出现一次的数字III
class Solution {public: vector<int> singleNumber(vector<int>& nums) { sort(nums.begin(), nums.end()); //遍历的索引 int i = 0; //结果保存在ret中 vector<int> ret; //循环执行到size - 1 的位置是为了判断最后一个数.原创 2020-11-13 10:03:45 · 148 阅读 · 0 评论 -
OJ ------ LeetCode 137 : 只出现一次的数字II
class Solution {public: int singleNumber(vector<int>& nums) { if (nums.size() == 1) return nums[0]; //排序 sort(nums.begin(), nums.end()); int i = 0; while (i < nums.size()) { .原创 2020-11-11 18:31:37 · 172 阅读 · 0 评论 -
OJ ------ LeetCode 26 : 删除排序数组中的重复项
class Solution {public: int removeDuplicates(vector<int>& nums) { if (nums.size() == 1) return 1; //排序好的数组, 只需要查找到相同的区间, 然后删除区间,留下一个元素即可 vector<int>::iterator it = nums.begin(); vector<i.原创 2020-11-11 18:20:33 · 104 阅读 · 0 评论 -
OJ -------- LeetCode118 : 杨辉三角
class Solution {public: vector<vector<int>> generate(int numRows) { //vector<vector<int>> 相当于一个二维数组 //注意这里创建的vector对象是空的, 要自己开空间 vector<vector<int>> v; v.resize(numRo.原创 2020-11-11 18:10:46 · 132 阅读 · 0 评论 -
OJ ------ LeetCode136 : 只出现一次的数字
class Solution {public: int singleNumber(vector<int>& nums) { // //排序法 sort(nums.begin(), nums.end()); int i = 0; while (i < nums.size() - 1) { if (nums[i] == nums[i + 1]) { i +.原创 2020-11-11 16:48:18 · 125 阅读 · 0 评论 -
(很强的思路) LeetCode 43 : 字符串相乘
class Solution {public: string multiply(string num1, string num2) { int len1 = num1.size(); int len2 = num2.size(); //两个数相乘, 乘机的位数不会超过这两个数的位数之和 //创建一个保存结果的对象,长度为len1+len2, 初始化为全'0' string ret(len1 + len2, '0').原创 2020-11-05 16:49:17 · 119 阅读 · 0 评论 -
(带思路, 详解) 牛客 HJ59 : 找出字符串中第一个只出现一次的字符
#include<iostream>#include<string>using namespace std;//思路: //先遍历一遍字符串, 给每次出现的字母对应的位置标记 //再次遍历数组, 找出第一次出现的字母, 返回void findFirstChar() { string s; //循环输入, 多组测试用例 while(cin >> s) { //标记数组, 直接128对应ASCII码, 无.原创 2020-11-05 15:33:10 · 313 阅读 · 0 评论 -
(带思路, 详解) LeetCode 557 : 翻转字符串中的单词3
class Solution {public: string reverseWords(string s) { //循环查找空格, 然后翻转中间的内容 size_t begin = 0; size_t pos = 0; string::iterator it = s.begin(); //循环查找空格, 翻转单词, 最后剩下一个待处理 while(pos < s.size()) { .原创 2020-11-05 15:08:01 · 75 阅读 · 0 评论 -
LeetCode 541 : 反转字符串
class Solution {public: string reverseStr(string s, int k) { int len = s.size(); //字符少于k个, 全部翻转 if(k > len) { reverse(s.begin(), s.end()); return s; } int i = 0; //循环遍历, 2k个字.原创 2020-11-03 22:41:30 · 70 阅读 · 0 评论 -
OJ ---- LeetCode 125 : 验证回文串
class Solution {public: //判断当前位置是否是有效字符 bool isVal(const char& ch) { return (ch >= '0' && ch <= '9') || (ch >= 'a' && ch <= 'z'); } bool isPalindrome(string s) { //思路: //先把大.原创 2020-11-03 19:02:44 · 93 阅读 · 0 评论 -
牛客 HJ1 : 字符串最后一个单词的长度
#include<iostream>#include<string>using namespace std;//思路: 接受一个串单词, 然后反向查找第一个空格, 计算长度int main() { string s; getline(cin, s);//接收带空格的字符串要用getline //反向寻找空格 size_t pos = s.rfind(' '); if(pos != string::npos) { .原创 2020-11-03 17:14:55 · 578 阅读 · 0 评论 -
OJ ----- LeetCode 387 : 字符串中的第一个唯一字符
class Solution {public: int firstUniqChar(string s) { //思路: //先遍历一次字符串, 把遇到的字母记录起来 //然后再遍历记录的数组, 把第一次遇到的字符索引返回 int count[128] = {0}; //记录数组, 直接128也就不用算偏移量了 //第一次遍历, 记录 for(auto& c.原创 2020-11-03 17:01:25 · 77 阅读 · 0 评论 -
OJ ---- LeetCode 344 : 翻转字符串
class Solution {public: void reverseString(vector<char>& s) { if (s.empty() == 1) return; int begin = 0; int end = s.size() - 1; //循环, 交换 while(begin < end) { swap(s[begin],.原创 2020-11-03 16:19:34 · 66 阅读 · 0 评论 -
OJ ---- LeetCode 415: 字符串相加
class Solution {public: string addStrings(string num1, string num2) { //两个索引 int len1 = num1.size() - 1; int len2 = num2.size() - 1; int step = 0; //进位信息 int curnum = 0; //当前位的数值 string ret; //保存结果 .原创 2020-11-01 16:09:20 · 127 阅读 · 0 评论 -
牛客 JZ49 : 把字符串转换成整数
class Solution {public: //判断字符是不是数字 bool isDigit(const char& ch) { if(ch >= '0' && ch <= '9') return true; return false; } int StrToInt(string str) { //判空 if(str.empt.原创 2020-11-01 15:24:46 · 87 阅读 · 0 评论 -
牛客 KY258 : 日期累加
#include<iostream>using namespace std;int main(){ int m; cin >> m; int year, month, day, num; static int arr[13] = {0,31,28,31,30,31,30,31,31,30,31,30,31}; for(int i = 0; i < m; ++i){ cin >> y.原创 2020-10-24 21:54:34 · 230 阅读 · 0 评论 -
牛客 KY222 : 打印日期
#include<iostream>using namespace std;int main(){ int year, num; static int arr[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; //多组输入 while(cin >> year >> num){ //判断是否为闰年, 确定2月的天数 if(.原创 2020-10-24 21:53:34 · 222 阅读 · 0 评论 -
牛客 KY111 : 日期差值
#include<iostream>using namespace std;int arr[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};//计算一个日期与0000年1月1日的天数差int getDay(int y, int m, int d){ //计算 0-y年的天数差 int days1 = y*365 + y/4 - y/100 + y/400; //计算1 - (m-1)月天数.原创 2020-10-24 21:51:59 · 364 阅读 · 0 评论 -
牛客 HJ73 : 计算日期到天数转换
#include<iostream>using namespace std;int main(){ static int days[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; int year, month, day; while(cin >> year >> month >> day){ int ret = 0; .原创 2020-10-24 21:50:56 · 241 阅读 · 0 评论 -
牛客: JZ47 求1+2+3+...+n
题目描述: 求1+2+3+…+n,要求不能使用乘除法、for、while、if、else、switch、case等关键字及条件判断语句(A?B:C)。思路: 利用内部类和直接访问外部类的静态变量, 可以通过创建一个内部类的对象数组, 调用n次构造函数, 实现累加需要注意的是题目的多次用例, 为了保证每次计算的结果不影响下一次计算, 每次计算前应该把变量设为初值class Solution {public: //内部类可以直接访问外部类的静态成员 class Sum{原创 2020-10-24 21:49:08 · 80 阅读 · 0 评论