一、导入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-spring-boot-starter</artifactId>
<version>4.4.0</version>
</dependency>
二、创建注解导出类
@Data
@EqualsAndHashCode(callSuper = false)
public class Product {
@Excel(name = "商品SN", width = 20)
private String productSn;
@Excel(name = "商品名称", width = 20)
private String name;
@Excel(name = "商品价格", width = 10)
private BigDecimal price;
@Excel(name = "购买数量", width = 10, suffix = "件")
private Integer count;
}
@Data
@EqualsAndHashCode(callSuper = false)
public class Order {
@Excel(name = "创建时间", width = 20, format = "yyyy/MM/dd",needMerge = true)
private Date createTime;
@Excel(name = "收货地址", width = 20,needMerge = true )
private String receiverAddress;
@ExcelCollection(name = "商品列表")
private List<Product> productList;
@ExcelCollection(name = "商品")
private List<Product> productList1;
}
三、导出
1、简单导出
package com.shop.demo.controller;
import cn.afterturn.easypoi.entity.vo.NormalExcelConstants;
import cn.afterturn.easypoi.excel.annotation.Excel;
import cn.afterturn.easypoi.excel.entity.ExportParams;
import cn.afterturn.easypoi.excel.entity.enmus.ExcelType;
import cn.afterturn.easypoi.view.PoiBaseView;
import com.shop.demo.util.Order;
import com.shop.demo.util.Product;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Controller
@RequestMapping("/easyPoi")
public class DemoController {
@RequestMapping(value = "/export", method = RequestMethod.GET)
public void exportOrderList(ModelMap map,
HttpServletRequest request,
HttpServletResponse response) {
List<Order> orderList = new ArrayList<>();
Order order = new Order();
order.setCreateTime(new Date());
order.setReceiverAddress("广东省广州市天河区繁华街道科摩罗远盾杯二街22号305房");
Product product = new Product();
Product product1 = new Product();
product.setName("This is the goods name");
product.setCount(3);
product.setPrice(new BigDecimal(445));
product.setProductSn(UUID.randomUUID().toString());
product1.setName("This is the goods name 1");
product1.setCount(31);
product1.setPrice(new BigDecimal(440));
product1.setProductSn(UUID.randomUUID().toString()+"1");
List<Product> list = new ArrayList<>();
List<Product> list1 = new ArrayList<>();
list.add(product);
list1.add(product1);
order.setProductList(list);
order.setProductList1(list1);
orderList.add(order);
ExportParams params = new ExportParams();
params.setExclusions(new String[]{"ID", "出生日期", "性别"});
map.put(NormalExcelConstants.DATA_LIST, orderList);
map.put(NormalExcelConstants.CLASS, Order.class);
map.put(NormalExcelConstants.PARAMS, params);
map.put(NormalExcelConstants.FILE_NAME, "订单列表复杂表头");
PoiBaseView.render(map, request, response, NormalExcelConstants.EASYPOI_EXCEL_VIEW);
}
}
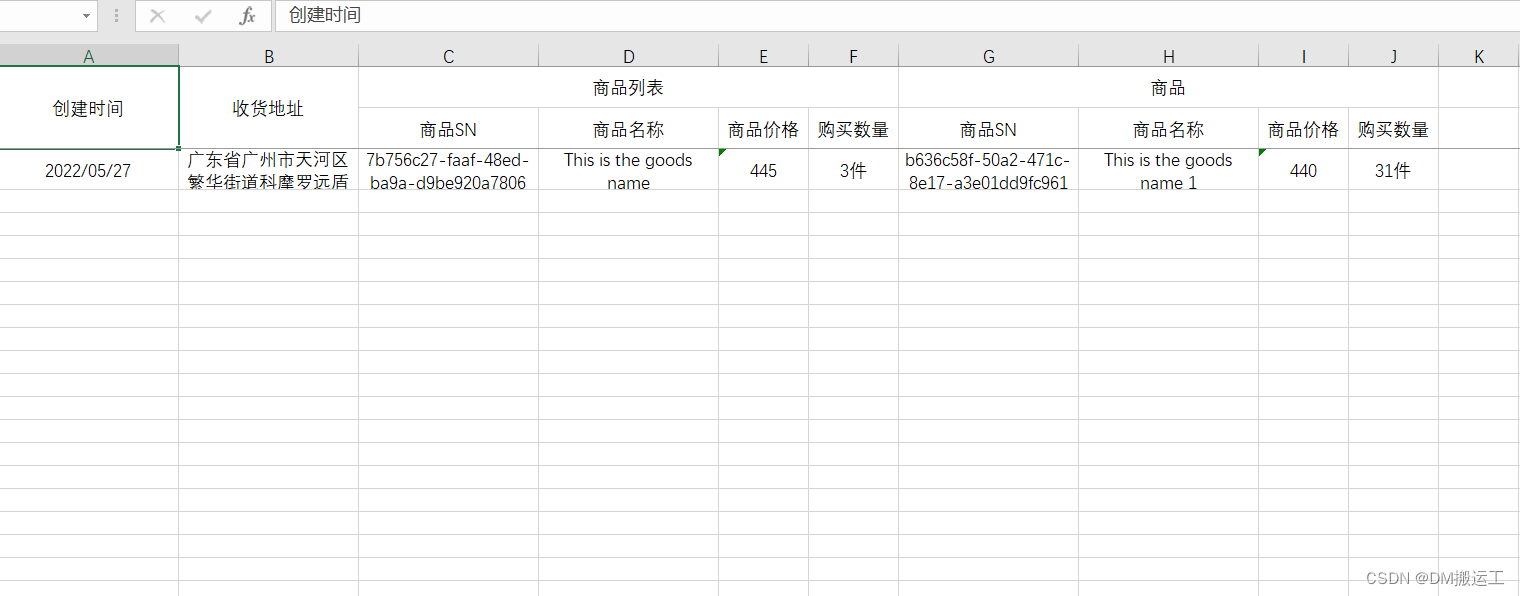
2、自定义样式导出
2.1、创建自定义样式工具类
public class ExcelStyleUtil implements IExcelExportStyler {
private static final short STRING_FORMAT = (short) BuiltinFormats.getBuiltinFormat("TEXT");
private static final short FONT_SIZE_TEN = 9;
private static final short FONT_SIZE_ELEVEN = 10;
private static final short FONT_SIZE_TWELVE = 10;
private CellStyle headerStyle;
private CellStyle titleStyle;
private CellStyle styles;
public ExcelStyleUtil(Workbook workbook) {
this.init(workbook);
}
private void init(Workbook workbook) {
this.headerStyle = initHeaderStyle(workbook);
this.titleStyle = initTitleStyle(workbook);
this.styles = initStyles(workbook);
}
@Override
public CellStyle getHeaderStyle(short color) {
return headerStyle;
}
@Override
public CellStyle getTitleStyle(short color) {
return titleStyle;
}
@Override
public CellStyle getStyles(boolean parity, ExcelExportEntity entity) {
return styles;
}
@Override
public CellStyle getStyles(Cell cell, int dataRow, ExcelExportEntity entity, Object obj, Object data) {
return getStyles(true, entity);
}
@Override
public CellStyle getTemplateStyles(boolean isSingle, ExcelForEachParams excelForEachParams) {
return null;
}
private CellStyle initHeaderStyle(Workbook workbook) {
CellStyle style = getBaseCellStyle(workbook);
return style;
}
private CellStyle initTitleStyle(Workbook workbook) {
CellStyle style = getBaseCellStyle(workbook);
style.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
return style;
}
private CellStyle initStyles(Workbook workbook) {
CellStyle style = getBaseCellStyle(workbook);
style.setDataFormat(STRING_FORMAT);
return style;
}
private CellStyle getBaseCellStyle(Workbook workbook) {
CellStyle style = workbook.createCellStyle();
style.setBorderBottom(BorderStyle.THIN);
style.setBorderLeft(BorderStyle.THIN);
style.setBorderTop(BorderStyle.THIN);
style.setBorderRight(BorderStyle.THIN);
style.setAlignment(HorizontalAlignment.CENTER);
style.setVerticalAlignment(VerticalAlignment.CENTER);
style.setWrapText(true);
return style;
}
private Font getFont(Workbook workbook, short size, boolean isBold) {
Font font = workbook.createFont();
font.setFontName("宋体");
font.setBold(isBold);
font.setFontHeightInPoints(size);
return font;
}
}
2.2、导出
package com.shop.demo.controller;
import cn.afterturn.easypoi.excel.ExcelExportUtil;
import cn.afterturn.easypoi.excel.entity.ExportParams;
import com.shop.demo.util.ExcelExportStyler;
import com.shop.demo.util.ExcelStyleUtil;
import com.shop.demo.util.Order;
import com.shop.demo.util.Product;
import org.apache.poi.ss.usermodel.*;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.math.BigDecimal;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Controller
@RequestMapping("/easyPoi")
public class DemoController01 {
@RequestMapping(value = "/export1", method = RequestMethod.GET)
public void exportOrderList( HttpServletResponse response) throws IOException {
List<Order> orderList = new ArrayList<>();
Order order = new Order();
setData(order,orderList);
ExportParams params = new ExportParams();
params.setExclusions(new String[]{"ID", "出生日期", "性别"});
params.setStyle(ExcelStyleUtil.class);
Workbook workbook = ExcelExportUtil.exportExcel(params, Order.class, orderList);
try {
response.setCharacterEncoding("UTF-8");
response.setHeader("content-Type", "application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode("订单列表复杂表头" + ".xlsx", "UTF-8"));
workbook.write(response.getOutputStream());
} catch (Exception e) {
throw new IOException(e.getMessage());
}
}
public void setData(Order order,List<Order> orderList){
for (int i=0; i< 10;i++){
order.setCreateTime(new Date());
order.setReceiverAddress("广东省广州市天河区繁华街道科摩罗远盾杯二街22号305房");
Product product = new Product();
Product product1 = new Product();
product.setName("This is the goods name");
product.setCount(3 + i);
product.setPrice(new BigDecimal(445 + i));
product.setProductSn(UUID.randomUUID().toString());
product1.setName("This is the goods name 1");
product1.setCount(31 + i);
product1.setPrice(new BigDecimal(440 + i ));
product1.setProductSn(UUID.randomUUID().toString()+"1");
List<Product> list = new ArrayList<>();
List<Product> list1 = new ArrayList<>();
list.add(product);
list1.add(product1);
order.setProductList(list);
order.setProductList1(list1);
orderList.add(order);
}
}
}
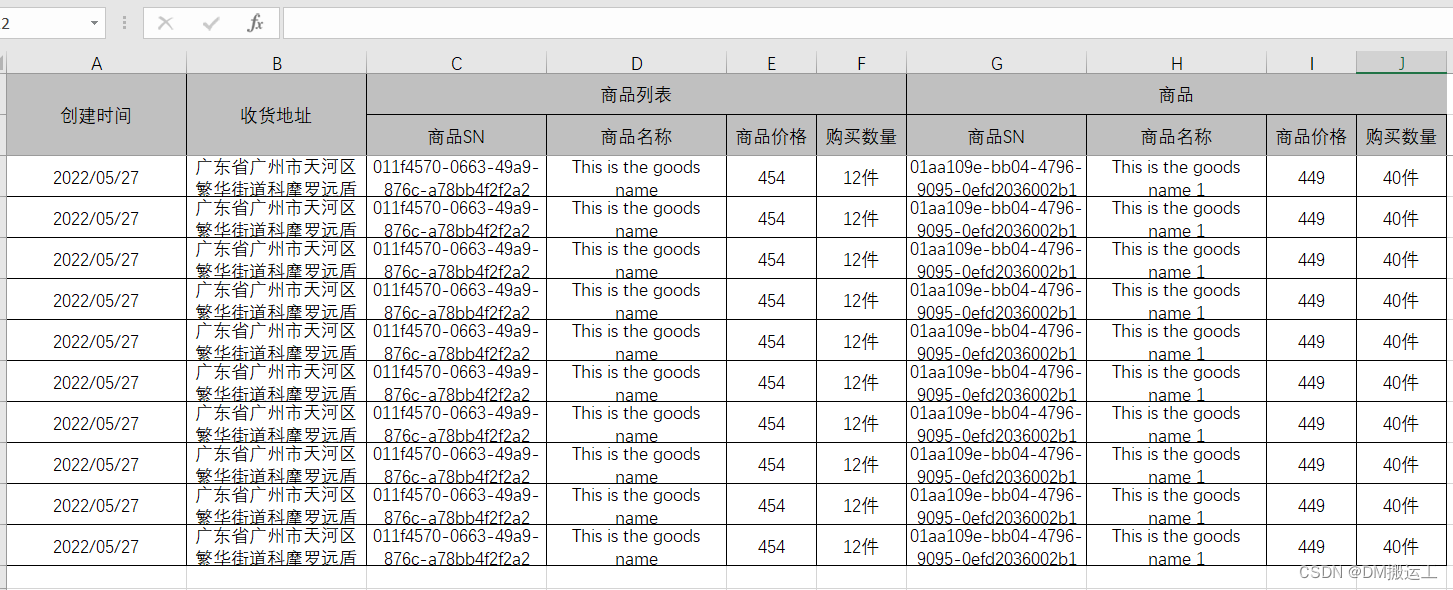
3、自定义标题样式导出
package com.shop.demo.controller;
import cn.afterturn.easypoi.excel.ExcelExportUtil;
import cn.afterturn.easypoi.excel.entity.ExportParams;
import com.shop.demo.util.ExcelStyleUtil;
import com.shop.demo.util.Order;
import com.shop.demo.util.Product;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.ss.util.RegionUtil;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.math.BigDecimal;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Controller
@RequestMapping("/easyPoi")
public class DemoController02 {
@RequestMapping(value = "/export2", method = RequestMethod.GET)
public void exportOrderList( HttpServletResponse response) throws IOException {
List<Order> orderList = new ArrayList<>();
Order order = new Order();
setData(order,orderList);
ExportParams params = new ExportParams();
params.setExclusions(new String[]{"ID", "出生日期", "性别"});
params.setStyle(ExcelStyleUtil.class);
Workbook workbook = ExcelExportUtil.exportExcel(params, Order.class, orderList);
setCellStyle(0,0, 2,IndexedColors.GREY_25_PERCENT.getIndex(),workbook);
setCellStyle(0,2, 1, IndexedColors.LIME.getIndex(),workbook);
setCellStyle(1,2, 4,IndexedColors.LIME.getIndex(),workbook);
setCellStyle(0,6, 1,IndexedColors.TAN.getIndex(),workbook);
setCellStyle(1,6, 4,IndexedColors.TAN.getIndex(),workbook);
try {
response.setCharacterEncoding("UTF-8");
response.setHeader("content-Type", "application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode("订单列表复杂表头" + ".xlsx", "UTF-8"));
workbook.write(response.getOutputStream());
} catch (Exception e) {
throw new IOException(e.getMessage());
}
}
public void setCellStyle(int rowNumber,int cellNumber,int size, short indexedColor,Workbook workbook){
CellStyle style = workbook.createCellStyle();
Sheet sheet = workbook.getSheetAt(0);
style.setFillForegroundColor(indexedColor);
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style.setAlignment(HorizontalAlignment.CENTER);
style.setVerticalAlignment(VerticalAlignment.CENTER);
style.setBorderBottom(BorderStyle.THIN);
style.setBorderLeft(BorderStyle.THIN);
style.setBorderTop(BorderStyle.THIN);
style.setBorderRight(BorderStyle.THIN);
CellRangeAddress mergedRegion = sheet.getMergedRegion(2);
RegionUtil.setBorderRight(BorderStyle.THIN, mergedRegion, sheet);
RegionUtil.setBorderTop(BorderStyle.THIN, mergedRegion, sheet);
CellRangeAddress mergedRegion2 = sheet.getMergedRegion(3);
RegionUtil.setBorderRight(BorderStyle.THIN, mergedRegion2, sheet);
RegionUtil.setBorderTop(BorderStyle.THIN, mergedRegion2, sheet);
for (int i = 0; i < size; i++) {
Row row = sheet.getRow(rowNumber );
Cell cell = row.getCell(cellNumber + i);
cell.setCellStyle(style);
}
}
public void setData(Order order,List<Order> orderList){
for (int i=0; i< 10;i++){
order.setCreateTime(new Date());
order.setReceiverAddress("广东省广州市天河区繁华街道科摩罗远盾杯二街22号305房");
Product product = new Product();
Product product1 = new Product();
product.setName("This is the goods name");
product.setCount(3 + i);
product.setPrice(new BigDecimal(445 + i));
product.setProductSn(UUID.randomUUID().toString());
product1.setName("This is the goods name 1");
product1.setCount(31 + i);
product1.setPrice(new BigDecimal(440 + i ));
product1.setProductSn(UUID.randomUUID().toString()+"1");
List<Product> list = new ArrayList<>();
List<Product> list1 = new ArrayList<>();
list.add(product);
list1.add(product1);
order.setProductList(list);
order.setProductList1(list1);
orderList.add(order);
}
}
}
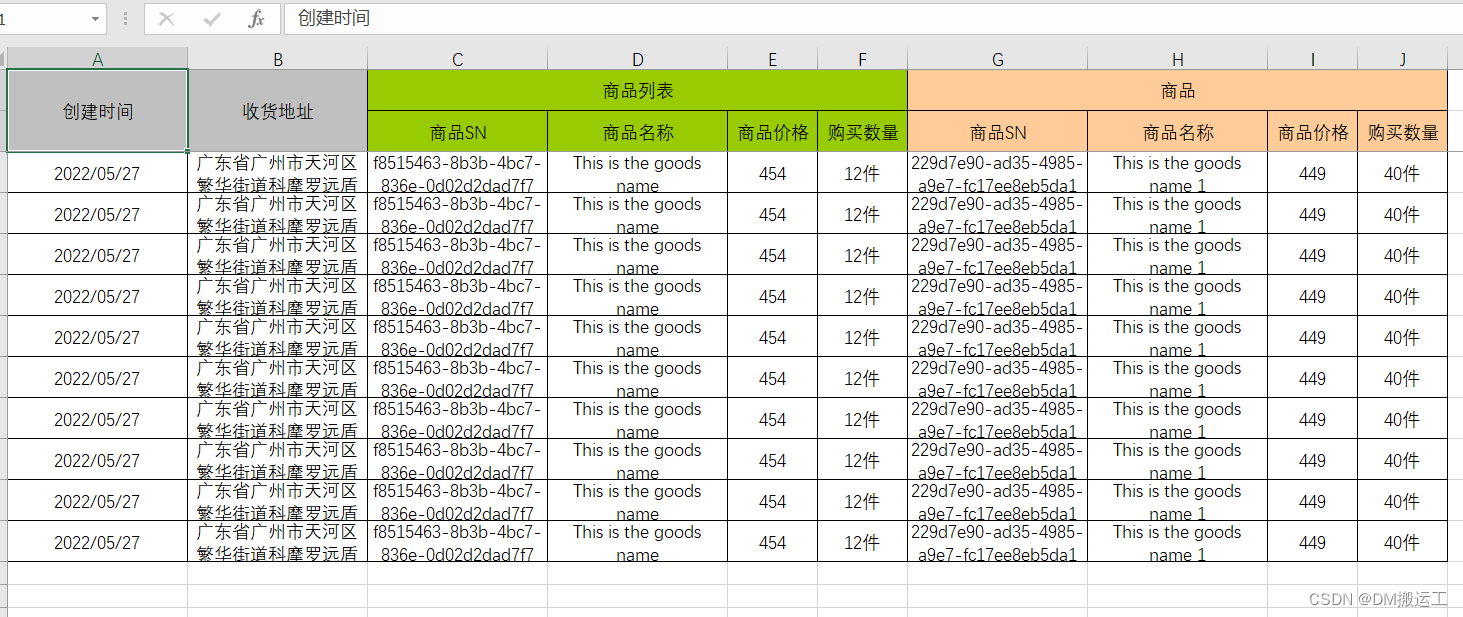