效果
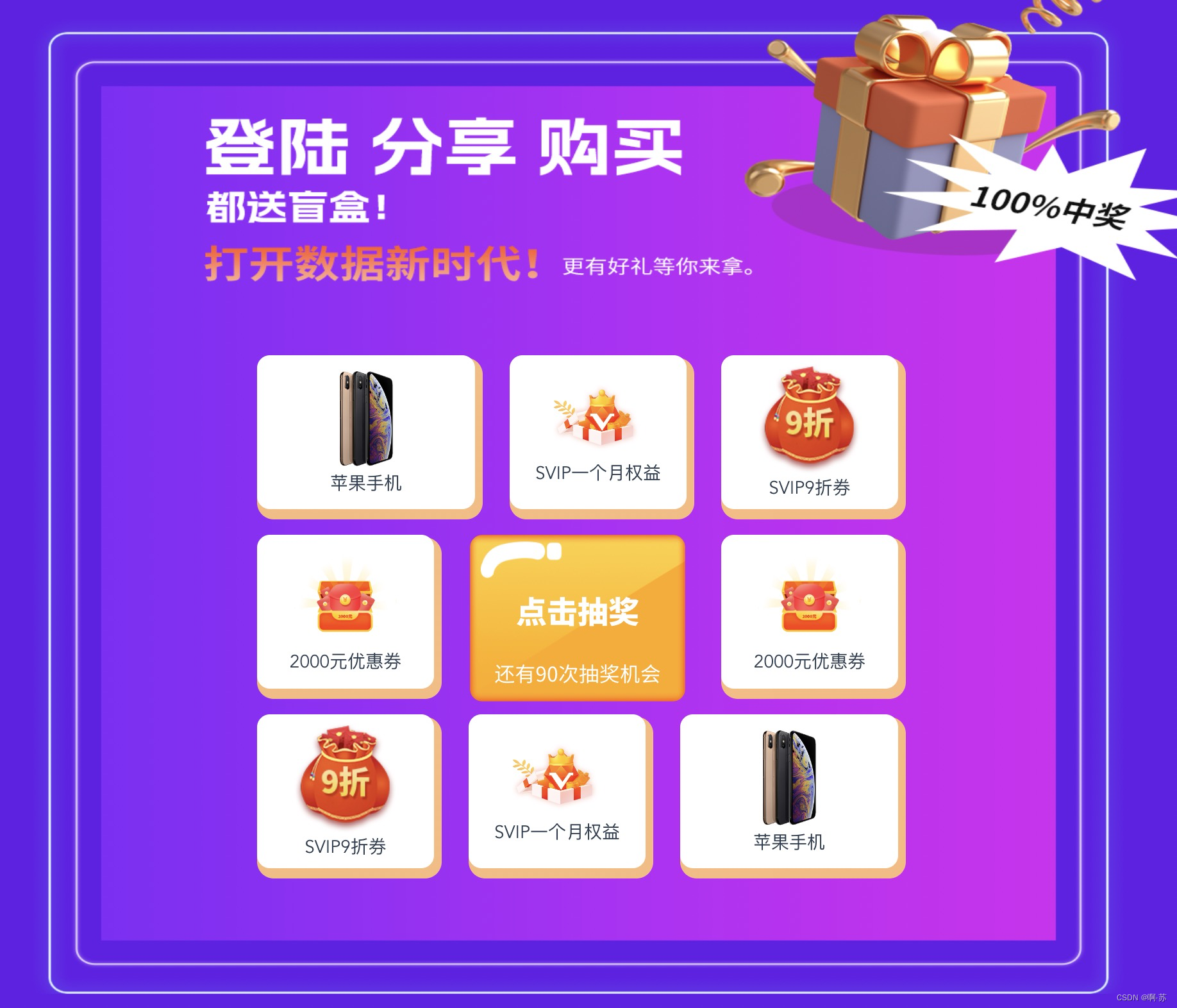
代码
<!-- 文件描述: 九宫格跑马灯游戏 -->
<!-- 创建时间: 2022/10/18;创建人: 阿苏 -->
<!-- 最后修改时间: 2022/10/18;最后修改人: 阿苏-->
<!-- -->
<template>
<div class='game'>
<div class="phone-prize" :class="{active:current==1}">
<img src="@/assets/speaial_activity_img/activity/phone_prize.png" alt="">
<p>苹果手机</p>
</div>
<div class="vip-prize" :class="{active:current==2}">
<img src="@/assets/speaial_activity_img/activity/prize_2.png" alt="">
<p>SVIP一个月权益</p>
</div>
<div class="vip-prize" :class="{active:current==3}">
<img src="@/assets/speaial_activity_img/activity/prize_3.png" alt="">
<p>SVIP9折券</p>
</div>
<div class="vip-prize" :class="{active:current==4}">
<img src="@/assets/speaial_activity_img/activity/prize_1.png" alt="">
<p>2000元优惠券</p>
</div>
<div class="start" @click="start">
<p class="title">点击抽奖</p>
<p class="info">还有{{luckNum}}次抽奖机会</p>
</div>
<div class="vip-prize" :class="{active:current==5}">
<img src="@/assets/speaial_activity_img/activity/prize_1.png" alt="">
<p>2000元优惠券</p>
</div>
<div class="vip-prize" :class="{active:current==6}">
<img src="@/assets/speaial_activity_img/activity/prize_3.png" alt="">
<p>SVIP9折券</p>
</div>
<div class="vip-prize" :class="{active:current==7}">
<img src="@/assets/speaial_activity_img/activity/prize_2.png" alt="">
<p>SVIP一个月权益</p>
</div>
<div class="phone-prize" :class="{active:current==8}">
<img src="@/assets/speaial_activity_img/activity/phone_prize.png" alt="">
<p>苹果手机</p>
</div>
</div>
</template>
<script>
export default {
name: '',
props: {
luckNum: {
type: [String, Number],
},
},
components: {},
data() {
return {
current: 0,
speed: 800,
diff: 20,
award: {},
time: 0,
timer: null,
startState: true,
awards: [
{ id: 1, name: '苹果手机' },
{ id: 2, name: 'SVIP一个月权益' },
{ id: 3, name: 'SVIP9折券' },
{ id: 4, name: '2000元优惠券' },
{ id: 5, name: '2000元优惠券' },
{ id: 6, name: 'SVIP9折券' },
{ id: 7, name: 'SVIP一个月权益' },
{ id: 8, name: '苹果手机' },
],
}
},
computed: {},
watch: {},
methods: {
start() {
if (this.luckNum == 0) {
this.$Message.error('没有抽奖机会!')
return
}
if (this.startState) {
this.getLottery()
this.time = Date.now()
this.speed = 200
this.diff = 12
this.$emit('start')
}
},
getLottery() {
let ind = Math.floor(Math.random() * 2)
let inds = [3, 6][ind]
this.award.name = this.awards[inds - 1].name
this.award.id = inds
this.move()
},
move() {
this.startState = false
this.timer = setTimeout(() => {
this.current++
if (this.current > 8) {
this.current = 0
}
if (this.award.id && (Date.now() - this.time) / 1000 > 3) {
this.speed += this.diff
if (
(Date.now() - this.time) / 1000 > 5 &&
this.award.id == this.awards[this.current].id
) {
clearTimeout(this.timer)
this.current += 1
this.startState = true
setTimeout(() => {
console.log(`恭喜中奖${this.award.name}元`)
this.$emit('result', {
name: this.award.name,
})
}, 0)
return
}
} else {
this.speed -= 6
}
this.move()
}, this.speed)
},
},
created() {},
mounted() {},
}
</script>
<style lang="scss" scoped>
.game {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
width: 500px;
}
.phone-prize {
width: 170px;
height: 120px;
background: #ffffff;
box-shadow: 3px 5px 0px 3px rgba(253, 192, 120, 0.97);
border-radius: 10px;
margin-bottom: 20px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
img {
width: 80px;
}
}
.vip-prize {
width: 138px;
height: 120px;
background: #ffffff;
box-shadow: 3px 5px 0px 3px rgba(253, 192, 120, 0.97);
border-radius: 10px;
margin-bottom: 20px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
img {
width: 80px;
}
}
.start {
width: 168px;
height: 130px;
background: url('../../../assets/speaial_activity_img/activity/start_btn.png')
no-repeat center;
background-size: 100% 100%;
border-radius: 10px;
cursor: pointer;
display: flex;
flex-direction: column;
justify-content: flex-end;
.title {
font-size: 24px;
font-family: Source Han Sans CN;
font-weight: 900;
color: #ffffff;
}
.info {
font-size: 16px;
font-family: Source Han Sans CN;
font-weight: 400;
color: #ffffff;
margin-top: 20px;
margin-bottom: 10px;
}
}
.active {
background: #ffd240;
}
</style>