openlayers 实现 聚合点删除 和聚合点弹框的效果
我的地图用的是父传子的方法写的 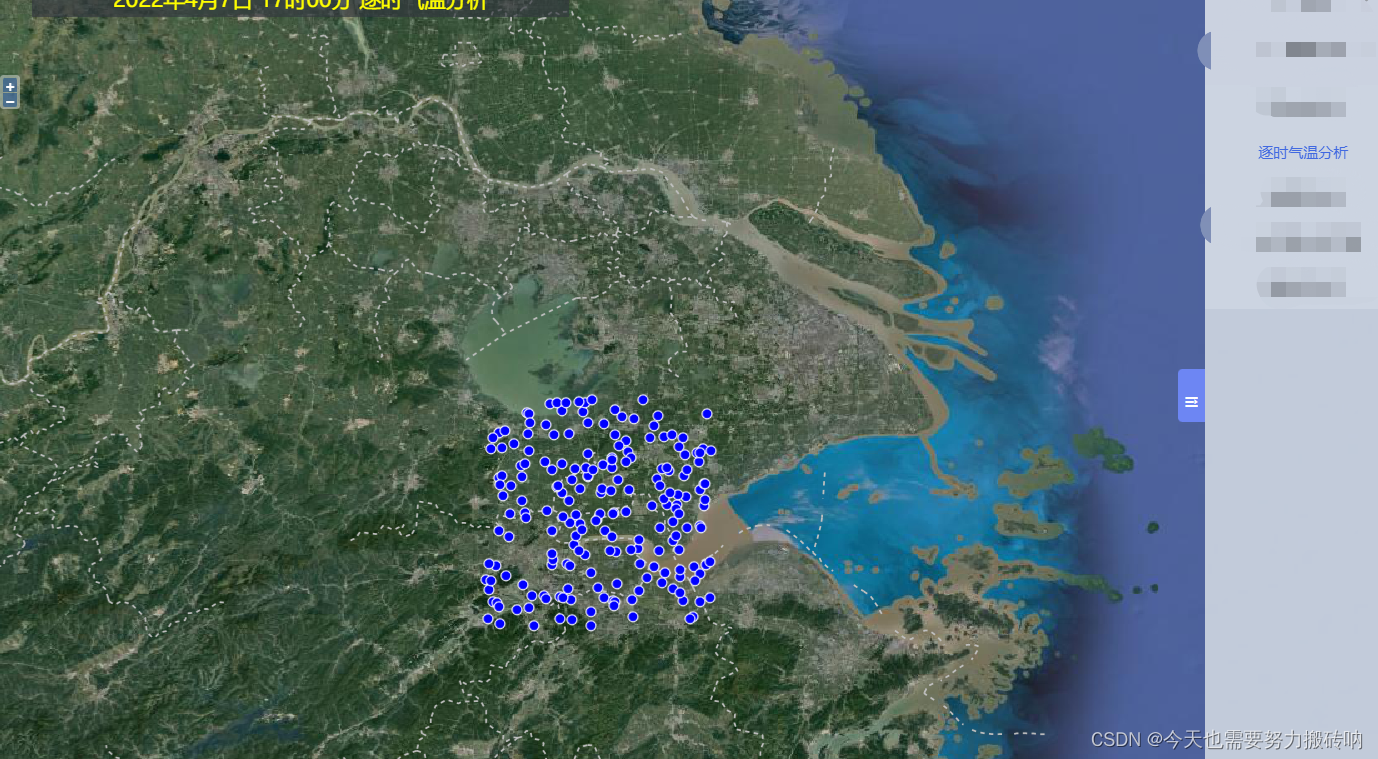
<template>
<div>
<el-dialog
title="提示"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose"
>
<!-- <span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取 消</el-button>
<el-button type="primary" @click="dialogVisible = false">确 定</el-button>
</span> -->
</el-dialog>
</div>
</template>
<script>
import { Circle as CircleStyle } from "ol/style";
import { Map, View, Feature, Overlay } from "ol";
import { Tile as TileLayer, Vector as VectorLayer } from "ol/layer";
import { Point } from "ol/geom";
import Style from "ol/style/Style";
import Fill from "ol/style/Fill";
import Stroke from "ol/style/Stroke";
import Text from "ol/style/Text";
import { Vector as SourceVec, Cluster } from "ol/source";
export default {
props: ["map"],
data() {
return {
size: "1",
layer: null,
sourceArr: null,
dialogVisible: false,
};
},
mounted() {
this.addMarker();
this.singleclick();
},
methods: {
addMarker() {
if (this.layer) {
let flag = this.layer.getVisible();
this.layer.setVisible(!flag);
} else {
const map = window.map;
this.sourceArr = new SourceVec({});
for (let i = 1; i <= 200; i++) {
let coordinates = [120.0 + Math.random(), 30.0 + Math.random()];
let feature = new Feature(new Point(coordinates));
let markerStyle = new Style({
image: new CircleStyle({
radius: 4,
stroke: new Stroke({
color: "#ffffff",
width: 1,
}),
fill: new Fill({
color: "blue",
}),
}),
text: new Text({
scale: 1.4,
fill: new Fill({
color: "#ffffff",
}),
offsetX: 30,
offsetY: 0,
}),
});
feature.setStyle(markerStyle);
this.sourceArr.addFeature(feature);
}
this.layer = new VectorLayer({
source: this.sourceArr,
name: "xxx",
});
map.addLayer(this.layer);
}
},
setVisibleZSQWFXLayer(flag) {
this.layer.setVisible(flag);
},
clearZSQWFXLayer() {
if (this.layer) {
this.layer.getSource().clear();
}
},
singleclick() {
const map = window.map;
map.on("singleclick", (e) => {
let feature = map.forEachFeatureAtPixel(e.pixel, (feature) => feature);
if (feature) {
this.dialogVisible = true;
let coordinates = feature.getGeometry().getCoordinates();
} else {
this.dialogVisible = false;
}
});
},
handleClose() {
this.dialogVisible=false
},
},
};
</script>
<style scoped>
</style>