订餐系统结构
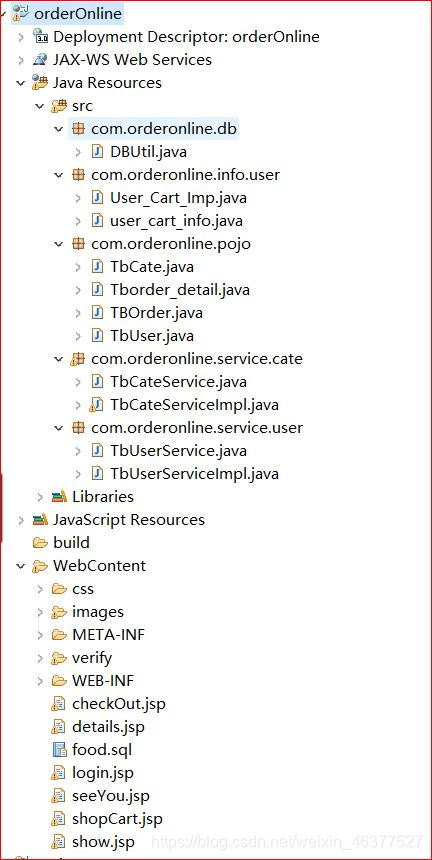
- 登录界面
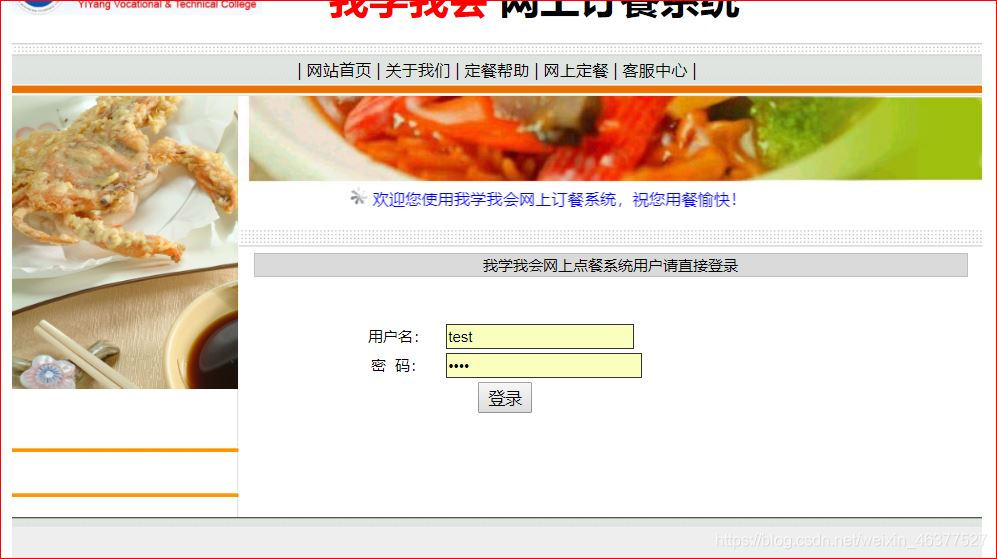
- 商品界面
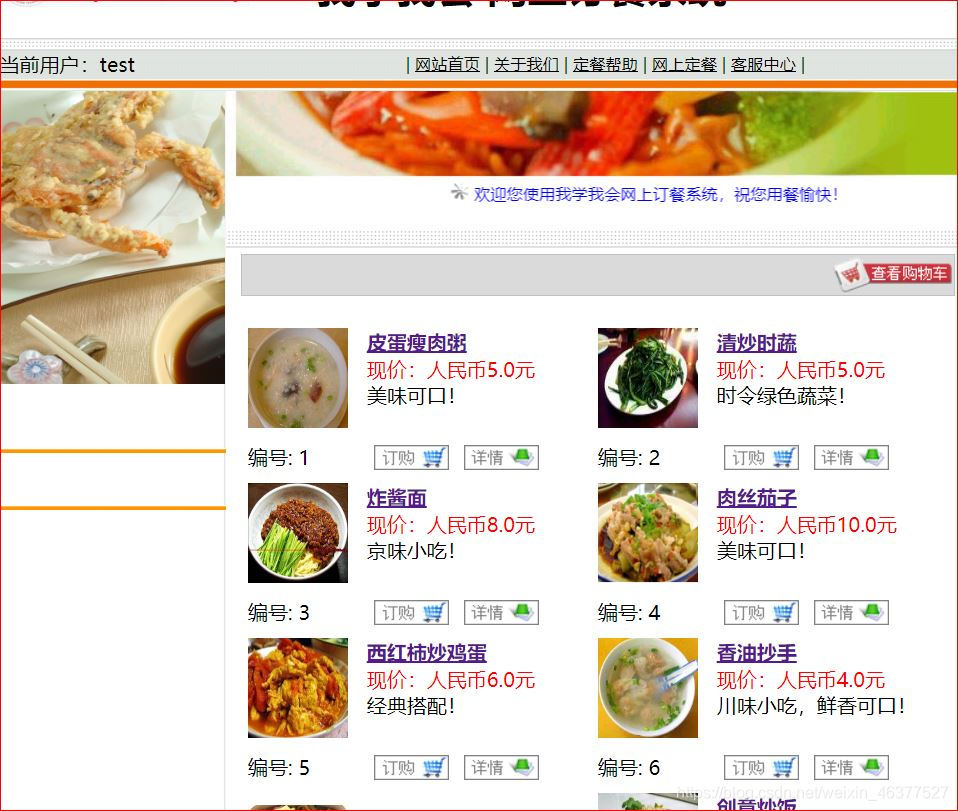
- 详细商品界面
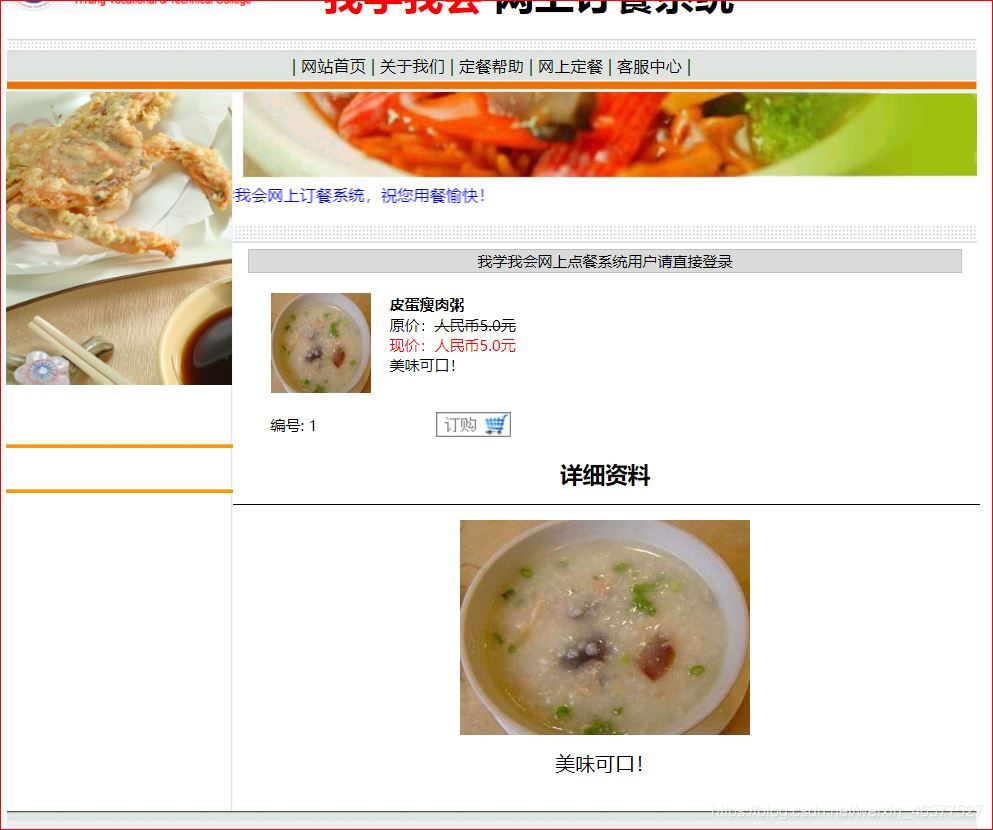
- 购物车列表界面

- 下单界面
10.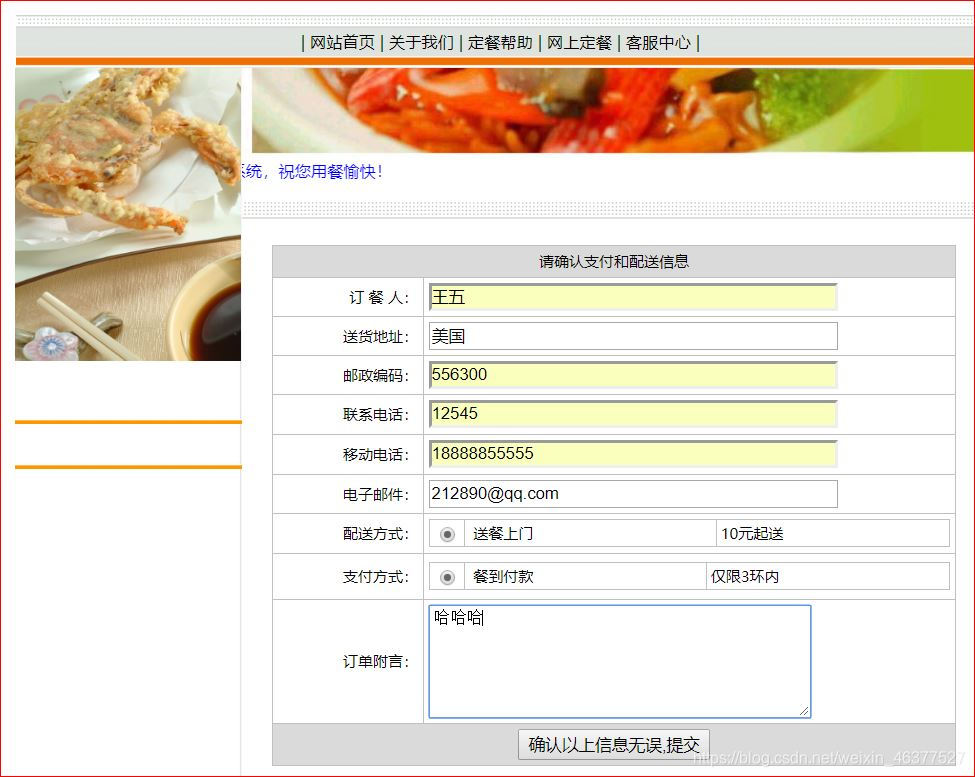
- 订单成功界面
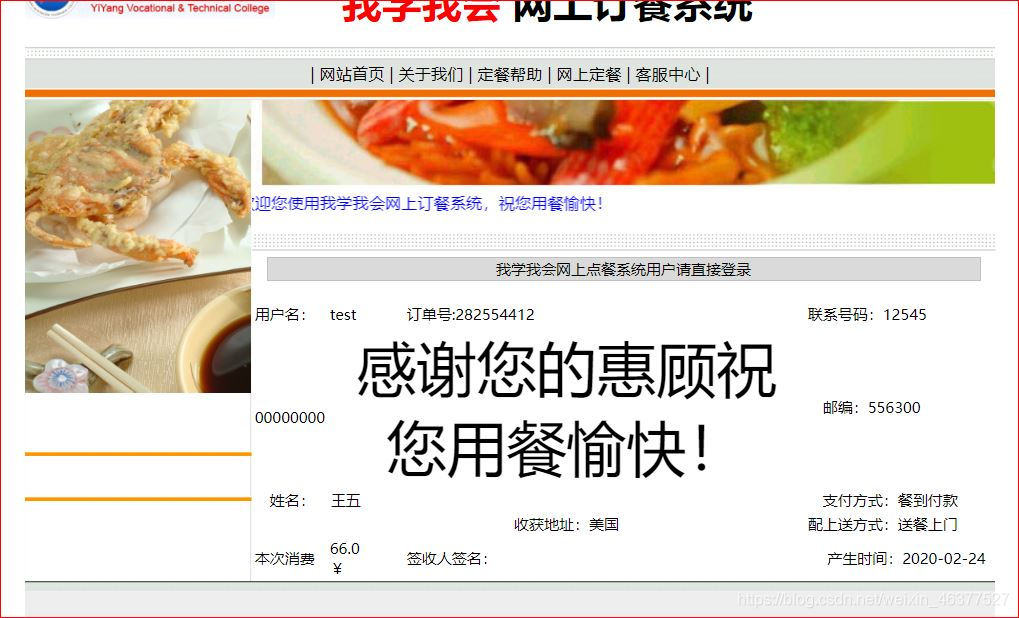
package com.orderonline.db;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
/**
* @author yangxiaoqiang
*
* */
/**数据库连接类*/
public class DBUtil {
private static final String CLASSNAME = "com.mysql.jdbc.Driver";
private static final String URL = "jdbc:mysql://localhost:3306/food_db?characterEncoding=utf-8&serverTimezone=GMT";
private static final String USERNAME = "root";
private static final String PASSWORD = "443650890";
static {
try {
Class.forName(CLASSNAME);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConnection() {
Connection conn = null;
try {
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
public static void closeAll(Connection conn, PreparedStatement pstmt, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
package com.orderonline.info.user;
import com.orderonline.pojo.TBOrder;
import com.orderonline.pojo.TbCate;
import com.orderonline.pojo.TbUser;
import com.orderonline.pojo.Tborder_detail;
/**
* @author yangxiaoqiang
*
* */
/**操作接口--查询和检查用户信息是否存在*/
public interface User_Cart_Imp {
/**通过user_id找到用户信息-----针对页面details.jsp and shopCart.jsp**/
public TbUser userInfo(Integer user_id);
/**通过cart_id找到该商品信息-----针对页面details.jsp and shopCart.jsp*/
public TbCate cartInfo(Integer cart_id);
/**通过order_id找到用户是否存相同订单id*/
public boolean ordercheck(Integer order_id);
/**通过order_id找到用户是否存相同订单id*/
public TBOrder order(Integer order_id);
/**查出用户下的所有下单信息*/
public TBOrder orderinfo(Integer user_id);
/**订单信息表*/
public int InsertOrder(TBOrder Order);
/**购物车信息添加——订单详细信息表*/
public int InsertTborder_detail(Integer order_id,Tborder_detail detail);
}
package com.orderonline.info.user;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.orderonline.db.DBUtil;
import com.orderonline.pojo.TBOrder;
import com.orderonline.pojo.TbCate;
import com.orderonline.pojo.TbUser;
import com.orderonline.pojo.Tborder_detail;
/**
* @author yangxiaoqiang
*
* */
/**实现操作类--查询和检查用户信息是否存在*/
public class user_cart_info implements User_Cart_Imp {
private Connection conn = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
@Override
public TbUser userInfo(Integer user_id) {
TbUser user = null;
String sql = "select * from tb_user where user_id=? ";
// 获取数据库链接
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
pstmt.setObject(1, user_id);
rs = pstmt.executeQuery();
if (rs.next()) {// 当登录成功
user = new TbUser();
user.setUser_id(rs.getInt("user_id"));
user.setUser_name(rs.getString("user_name"));
user.setCh_name(rs.getString("ch_name"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return user;
}
@Override
public TbCate cartInfo(Integer cart_id) {
TbCate cate=new TbCate();
String sql="select * from tb_cate where cate_id=? ";
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
pstmt.setObject(1, cart_id);
// 执行SQL语句
rs = pstmt.executeQuery();
while(rs.next()) {
cate = new TbCate();
cate.setCate_id(rs.getInt("cate_id"));
cate.setCate_name(rs.getString("cate_name"));
cate.setCur_price(rs.getFloat("cur_price"));
cate.setDescript(rs.getString("descript"));
cate.setImg_path(rs.getString("img_path"));
cate.setOri_price(rs.getFloat("ori_price"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return cate;
}
@Override
public boolean ordercheck(Integer order_id) {
String sql = "select * from tb_order where order_id=? ";
// 获取数据库链接
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, order_id);
rs = pstmt.executeQuery();
if(rs.next()) {
return true;
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return false;
}
@Override
public TBOrder orderinfo(Integer user_id) {
TBOrder Order = new TBOrder() ;
String sql = "select * from tb_order where user_id=? ";
// 获取数据库链接
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, user_id);
rs = pstmt.executeQuery();
if(rs.next()) {
Order.setOrder_id(rs.getInt("order_id"));
Order.setAddress(rs.getString("address"));
Order.setCh_name(rs.getString("ch_name"));
Order.setEmail(rs.getString("email"));
Order.setMobile(rs.getString("mobile"));
Order.setPayType(rs.getString("payType"));
Order.setPhone(rs.getString("phone"));
Order.setPostalcode(rs.getString("postalcode"));
Order.setPostscript(rs.getString("postscript"));
Order.setSendType(rs.getString("sendType"));
Order.setUser_id(rs.getInt("user_id"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return Order;
}
//测试成功!
public static void main(String[] args){
//System.out.println(new user_cart_info().ordercheck(1));
//System.out.println(new user_cart_info().userInfo(1));
//System.out.println(new user_cart_info().cartInfo(1));
//System.out.println(new user_cart_info().orderinfo(1));
/* TBOrder Order=new TBOrder();
Order.setAddress("sadf");
Order.setCh_name("556300");
Order.setEmail("556300");
Order.setMobile("556300");
Order.setOrder_id(456);
Order.setPayType("d");
Order.setPhone("556300");
Order.setPostalcode("556300");
Order.setPostscript("不好");
Order.setSendType("s");
Order.setUser_id(1);
int i=new user_cart_info().InsertOrder(Order);
System.out.println(i);
*/
/*Tborder_detail detail=new Tborder_detail();
detail.setCate_id(1);
detail.setCate_name("fas");
detail.setCOUNT(5);
detail.setDetail_id(1);
detail.setMoney(22);
detail.setPrice(55);
detail.setOrder_id(1);
int i=new user_cart_info().InsertTborder_detail(1, detail);
System.out.println(i);
*/
}
@Override
public int InsertTborder_detail(Integer order_id, Tborder_detail detail) {
String sql = "insert into tb_order_detail values (?,?,?,?,?,?,?) ";
conn = DBUtil.getConnection();
int count = 0; // 受影响的行数
try {
pstmt = (PreparedStatement) conn.prepareStatement(sql);
// 设置参数
pstmt.setObject(1,0 );
pstmt.setObject(2,order_id );
pstmt.setObject(3, detail.getCate_id());
pstmt.setObject(4, detail.getCate_name());
pstmt.setObject(5, detail.getPrice());
pstmt.setObject(6, detail.getCOUNT());
pstmt.setObject(7, detail.getMoney());
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return count;
}
@Override
public int InsertOrder(TBOrder Order) {
String sql = "insert into tb_order VALUES(?,?,?,?,?,?,?,?,?,?,?) ";
conn = DBUtil.getConnection();
int count = 0; // 受影响的行数
try {
pstmt = (PreparedStatement) conn.prepareStatement(sql);
// 设置参数
pstmt.setObject(1,Order.getOrder_id() );
pstmt.setObject(2,Order.getUser_id() );
pstmt.setObject(3, Order.getCh_name());
pstmt.setObject(4, Order.getAddress());
pstmt.setObject(5, Order.getPostalcode());
pstmt.setObject(6, Order.getPhone());
pstmt.setObject(7, Order.getMobile());
pstmt.setObject(8, Order.getEmail());
pstmt.setObject(9, Order.getSendType());
pstmt.setObject(10, Order.getPayType());
pstmt.setObject(11, Order.getPostscript());
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return count;
}
@Override
public TBOrder order(Integer order_id) {
TBOrder Order = null ;
String sql = "select * from tb_order where order_id=? ;";
// 获取数据库链接
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
pstmt.setObject(1, order_id);
rs = pstmt.executeQuery();
if (rs.next()) {// 当登录成功
Order = new TBOrder();
Order.setCh_name(rs.getString("ch_name"));
Order.setAddress(rs.getString("address"));
Order.setPhone(rs.getString("phone"));
Order.setEmail(rs.getString("email"));
Order.setOrder_id(rs.getInt("order_id"));
Order.setMobile(rs.getString("mobile"));
Order.setPostscript(rs.getString("postscript"));
Order.setSendType(rs.getString("sendType"));
Order.setPostalcode(rs.getString("postalcode"));
Order.setPayType(rs.getString("payType"));;
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return Order;
}
}
package com.orderonline.pojo;
/**
* @author yangxiaoqiang
*
* */
/***商品信息类*/
public class TbCate {
private Integer cate_id;
private String cate_name;
private Float ori_price;
private Float cur_price;
private String img_path;
private String descript;
public Integer getCate_id() {
return cate_id;
}
public void setCate_id(Integer cate_id) {
this.cate_id = cate_id;
}
public String getCate_name() {
return cate_name;
}
public void setCate_name(String cate_name) {
this.cate_name = cate_name;
}
public Float getOri_price() {
return ori_price;
}
public void setOri_price(Float ori_price) {
this.ori_price = ori_price;
}
public Float getCur_price() {
return cur_price;
}
public void setCur_price(Float cur_price) {
this.cur_price = cur_price;
}
public String getImg_path() {
return img_path;
}
public void setImg_path(String img_path) {
this.img_path = img_path;
}
public String getDescript() {
return descript;
}
public void setDescript(String descript) {
this.descript = descript;
}
public TbCate(Integer cate_id, String cate_name, Float ori_price, Float cur_price, String img_path,
String descript) {
this.cate_id = cate_id;
this.cate_name = cate_name;
this.ori_price = ori_price;
this.cur_price = cur_price;
this.img_path = img_path;
this.descript = descript;
}
public TbCate() {
}
@Override
public String toString() {
return "TbCate [cate_id=" + cate_id + ", cate_name=" + cate_name + ", ori_price=" + ori_price + ", cur_price="
+ cur_price + ", img_path=" + img_path + ", descript=" + descript + "]";
}
}
package com.orderonline.pojo;
/**
* @author yangxiaoqiang
*
* */
/**订单详细信息表--类*/
public class Tborder_detail {
private Integer detail_id ; // '订单详细id',
private Integer order_id ;// '订单id' ,
private Integer cate_id ;//'商品id',
private String cate_name ; //'商品名称',
private double price ;//'价格',
private Integer COUNT ; //'数量',
private double money ;// '金额'
public Integer getDetail_id() {
return detail_id;
}
public void setDetail_id(Integer detail_id) {
this.detail_id = detail_id;
}
public Integer getOrder_id() {
return order_id;
}
public void setOrder_id(Integer order_id) {
this.order_id = order_id;
}
public Integer getCate_id() {
return cate_id;
}
public void setCate_id(Integer cate_id) {
this.cate_id = cate_id;
}
public String getCate_name() {
return cate_name;
}
public void setCate_name(String cate_name) {
this.cate_name = cate_name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Integer getCOUNT() {
return COUNT;
}
public void setCOUNT(Integer cOUNT) {
COUNT = cOUNT;
}
public double getMoney() {
return money;
}
public void setMoney(double money) {
this.money = money;
}
public Tborder_detail() {
super();
// TODO Auto-generated constructor stub
}
public Tborder_detail(Integer detail_id, Integer order_id, Integer cate_id,
String cate_name, Float price, Integer cOUNT, Float money) {
super();
this.detail_id = detail_id;
this.order_id = order_id;
this.cate_id = cate_id;
this.cate_name = cate_name;
this.price = price;
COUNT = cOUNT;
this.money = money;
}
@Override
public String toString() {
return "Tborder_detail [detail_id=" + detail_id + ", order_id="
+ order_id + ", cate_id=" + cate_id + ", cate_name="
+ cate_name + ", price=" + price + ", COUNT=" + COUNT
+ ", money=" + money + "]";
}
}
package com.orderonline.pojo;
/**
* @author yangxiaoqiang
*
* */
/**订单类*/
public class TBOrder {
private Integer order_id; // '订单id',
private Integer user_id ;//'用户id',
private String ch_name ; // '订餐人',
private String address ;// '送货地址',
private String postalcode ;// '邮政编码',
private String phone ; //'联系电话',
private String mobile ; // '移动电话',
private String email ; //'电子邮件',
private String sendType ; // '配送方式',
private String payType ; // '支付方式',
private String postscript ; //'订单附言'
public Integer getOrder_id() {
return order_id;
}
public void setOrder_id(Integer order_id) {
this.order_id = order_id;
}
public Integer getUser_id() {
return user_id;
}
public void setUser_id(Integer user_id) {
this.user_id = user_id;
}
public String getCh_name() {
return ch_name;
}
public void setCh_name(String ch_name) {
this.ch_name = ch_name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPostalcode() {
return postalcode;
}
public void setPostalcode(String postalcode) {
this.postalcode = postalcode;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getMobile() {
return mobile;
}
public void setMobile(String mobile) {
this.mobile = mobile;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getSendType() {
return sendType;
}
public void setSendType(String sendType) {
this.sendType = sendType;
}
public String getPayType() {
return payType;
}
public void setPayType(String payType) {
this.payType = payType;
}
public String getPostscript() {
return postscript;
}
public void setPostscript(String postscript) {
this.postscript = postscript;
}
public TBOrder() {
}
public TBOrder(Integer order_id, Integer user_id, String ch_name,
String address, String postalcode, String phone, String mobile,
String email, String sendType, String payType, String postscript) {
super();
this.order_id = order_id;
this.user_id = user_id;
this.ch_name = ch_name;
this.address = address;
this.postalcode = postalcode;
this.phone = phone;
this.mobile = mobile;
this.email = email;
this.sendType = sendType;
this.payType = payType;
this.postscript = postscript;
}
@Override
public String toString() {
return "TBOrder [order_id=" + order_id + ", user_id=" + user_id
+ ", ch_name=" + ch_name + ", address=" + address
+ ", postalcode=" + postalcode + ", phone=" + phone
+ ", mobile=" + mobile + ", email=" + email + ", sendType="
+ sendType + ", payType=" + payType + ", postscript="
+ postscript + "]";
}
}
package com.orderonline.pojo;
/**
* @author yangxiaoqiang
*
* */
/**用户表信息类*/
public class TbUser {
private Integer user_id; // 用户ID
private String user_name; // 用户名称
private String ch_name; // 真实姓名
private String password; // 用户密码
public Integer getUser_id() {
return user_id;
}
public void setUser_id(Integer user_id) {
this.user_id = user_id;
}
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getCh_name() {
return ch_name;
}
public void setCh_name(String ch_name) {
this.ch_name = ch_name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public TbUser(Integer user_id, String user_name, String ch_name, String password) {
this.user_id = user_id;
this.user_name = user_name;
this.ch_name = ch_name;
this.password = password;
}
public TbUser() {
}
@Override
public String toString() {
return "TbUser [user_id=" + user_id + ", user_name=" + user_name + ", ch_name=" + ch_name + ", password="
+ password + "]";
}
}
package com.orderonline.service.cate;
import java.util.List;
import com.orderonline.pojo.TbCate;
public interface TbCateService {
public List<TbCate> cateList();
}
package com.orderonline.service.cate;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.orderonline.db.DBUtil;
import com.orderonline.pojo.TbCate;
public class TbCateServiceImpl implements TbCateService {
private Connection conn = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
@Override
public List<TbCate> cateList() {
List<TbCate> list = new ArrayList<TbCate>();
TbCate cate = null;
String sql = "select * from tb_cate";
conn = DBUtil.getConnection();
try {
pstmt = conn.prepareStatement(sql);
// 执行SQL语句
rs = pstmt.executeQuery();
while(rs.next()) {
cate = new TbCate();
cate.setCate_id(rs.getInt("cate_id"));
cate.setCate_name(rs.getString("cate_name"));
cate.setCur_price(rs.getFloat("cur_price"));
cate.setDescript(rs.getString("descript"));
cate.setImg_path(rs.getString("img_path"));
cate.setOri_price(rs.getFloat("ori_price"));
list.add(cate);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pstmt, rs);
}
return list;
}
/*
//测试两个商品一行
public static void main(String[] args) {
TbCateService tcs = new TbCateServiceImpl();
Iterator<TbCate> it = tcs.cateList().iterator();
// 3,4,5,6,7,8,9,10
while(it.hasNext()) { // 判断迭代器里是否还有值存在
TbCate t1 = it.next();
System.out.print(t1.getCate_name() + "\t");
//第二个商品
if (it.hasNext()) {
TbCate t2 = it.next();
System.out.print(t2.getCate_name());
}
System.out.println("\n");
}
}*/
}
package com.orderonline.service.user;
import com.orderonline.pojo.TbUser;
public interface TbUserService {
public TbUser userLogin(String user,String pass);
}
package com.orderonline.service.user;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.orderonline.db.DBUtil;
import com.orderonline.pojo.TbUser;
public class TbUserServiceImpl implements TbUserService {
private Connection conn = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
@Override
public TbUser userLogin(String username, String pass) {
TbUser user = null;
String sql = "select * from tb_user where user_name=? and password=?";
// 获取数据库链接