使用MP,Service实现类中报错提示需要实现很多增删改查方法,是因为Mapper中没有继承BaseMapper,所以无法继承到这些方法,就会提示需要实现这些方法。
使用MP,Service实现类中提示需要实现方法
最新推荐文章于 2024-06-07 00:48:13 发布
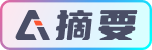
使用MP,Service实现类中报错提示需要实现很多增删改查方法,是因为Mapper中没有继承BaseMapper,所以无法继承到这些方法,就会提示需要实现这些方法。