目录
Swing简介和使用
Swing是AWT的封装
功能更加全面
窗口、面板
public class JFrameDemo {
//init();初始化
public void init(){
//JFrame是一个顶级窗口
JFrame jf = new JFrame("这是一个JFrame窗口");
jf.setVisible(true);
jf.setBounds(100,100,200,200);
//关闭事件
//参数里面有,隐藏、什么都不做关闭、关闭、dispose(只剩下最后一个窗口时可以关闭)
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//设置文字
JLabel jlabel = new JLabel("一个标签");
jf.add(jlabel);
//让文本内容居中
jlabel.setHorizontalAlignment(SwingConstants.CENTER);
//和AWT不一样的地方
//需要获得一个容器
Container contentPane = jf.getContentPane();
//容器中设置背景颜色
contentPane.setBackground(Color.pink);
}
public static void main(String[] args) {
//建立一个窗口
new JFrameDemo().init();
}
}
JDialog弹窗
//主窗口
public class DialogTest extends JFrame {
public DialogTest(){
setVisible(true);
setBounds(1,1,700,500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//JFrame 放东西,容器
Container contentPane = getContentPane();
//绝对布局,直接使用坐标定位
contentPane.setLayout(null);
//按钮
JButton jButton = new JButton("点击弹出一个对话框");
jButton.setBounds(30,30,200,50);
//点击这个按钮的时候弹出一个弹窗
jButton.addActionListener((event)->{//监听器
//弹出弹窗
new MyDialog();
});
contentPane.add(jButton);
}
public static void main(String[] args) {
new DialogTest();
}
}
class MyDialog extends JDialog{
public MyDialog() {
this.setVisible(true);
this.setBounds(100,100,500,500);
//这里有默认关闭事件不用再添加关闭,会报错
// this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Container contentPane = this.getContentPane();
contentPane.setLayout(null);
JLabel label = new JLabel("XXX");
//当设置了绝对布局时需要设定组件的坐标及大小
label.setBounds(100,100,100,50);
contentPane.add(label);
}
}
点击后
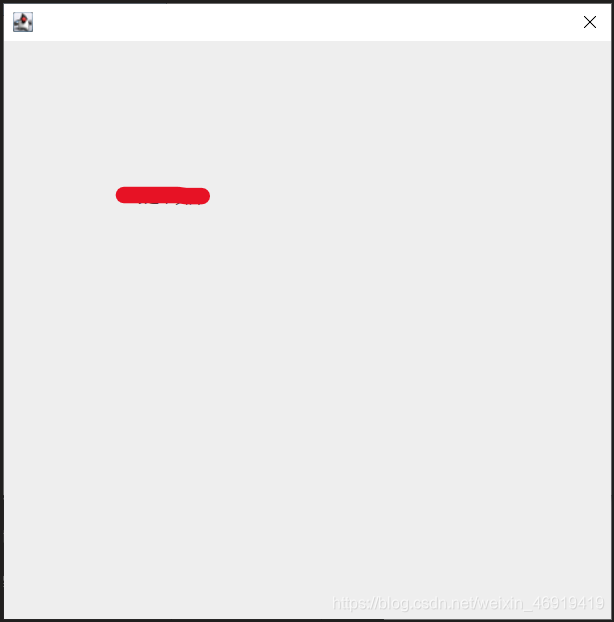
Icon、ImageIcon标签
标签
new Jlabel("xxx")
图标
public class ImageIconDemo extends JFrame {
public ImageIconDemo(){
//获取图片的地址
URL resource = ImageIconDemo.class.getResource("1.png");
System.out.println(resource);
JLabel jlabel = new JLabel("imageIcon");
//放入图片地址
ImageIcon imageIcon = new ImageIcon(resource);
jlabel.setIcon(imageIcon);
jlabel.setHorizontalAlignment(SwingConstants.CENTER);
Container contentPane = getContentPane();
contentPane.add(jlabel);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
setBounds(100,100,200,200);
}
public static void main(String[] args) {
new ImageIconDemo();
}
}
面板JPanel
JPanel
public class TestJPanel extends JFrame {
public TestJPanel(){
Container contentPane = getContentPane();
contentPane.setLayout(new GridLayout(2,1,10,10));//后面两参数是间距
JPanel jPanel1 = new JPanel(new GridLayout(1,3));
JPanel jPanel2 = new JPanel(new GridLayout(1,2));
JPanel jPanel3 = new JPanel(new GridLayout(2,1));
JPanel jPanel4 = new JPanel(new GridLayout(2,2));
//放这么多是为了测试间距
jPanel1.add(new JButton("1"));
jPanel1.add(new JButton("1"));
jPanel1.add(new JButton("1"));
jPanel2.add(new JButton("1"));
jPanel2.add(new JButton("1"));
jPanel3.add(new JButton("1"));
jPanel3.add(new JButton("1"));
jPanel4.add(new JButton("1"));
jPanel4.add(new JButton("1"));
jPanel4.add(new JButton("1"));
jPanel4.add(new JButton("1"));
jPanel4.add(new JButton("1"));
jPanel4.add(new JButton("1"));
setVisible(true);
setBounds(200,200,500,500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
contentPane.add(jPanel1);
contentPane.add(jPanel2);
contentPane.add(jPanel3);
contentPane.add(jPanel4);
}
public static void main(String[] args) {
new TestJPanel();
}
}
滚动面板JScrollPanel
JScrollPanel
public class JScrollPanelTest extends JFrame {
public JScrollPanelTest(){
Container contentPane = this.getContentPane();
//文本域
JTextArea jTextArea = new JTextArea(20,50);
jTextArea.setText("djsakhozxchasdouifhaofjhaodfj");
//JScroll面板
JScrollPane jScrollPane = new JScrollPane(jTextArea);
contentPane.add(jScrollPane);
setVisible(true);
setBounds(100,100,500,450);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JScrollPanelTest();
}
}
图片按钮
public class JButtonDemo1 extends JFrame {
public JButtonDemo1(){
Container contentPane = getContentPane();
URL resource = JButtonDemo1.class.getResource("1.png");
Icon imageIcon = new ImageIcon(resource);
//将图片放在按钮
JButton jButton = new JButton();
jButton.setIcon(imageIcon);
//按钮触摸提示
jButton.setToolTipText("图片按钮");
contentPane.add(jButton);
setBounds(100,100,400,400);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JButtonDemo1();
}
}
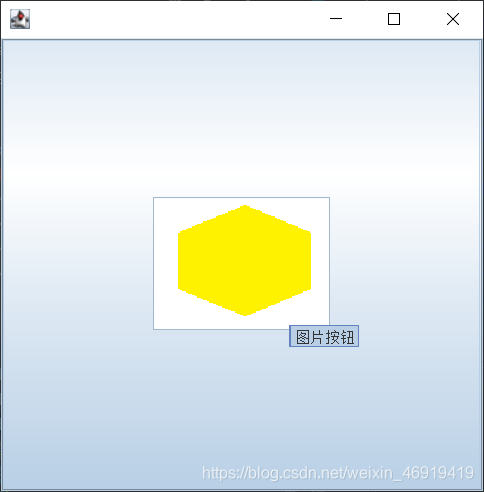
单选框,多选框
单选框
public JButtonDemo1(){
Container contentPane = getContentPane();
//单选框
JRadioButton jRadioButton1 = new JRadioButton("JRadioButton01");
JRadioButton jRadioButton2 = new JRadioButton("JRadioButton02");
JRadioButton jRadioButton3 = new JRadioButton("JRadioButton03");
//由于单选框只能选择一个,分组,一个组中只能选择一个
ButtonGroup buttonGroup = new ButtonGroup();
//按钮进组
buttonGroup.add(jRadioButton1);
buttonGroup.add(jRadioButton2);
buttonGroup.add(jRadioButton3);
contentPane.add(jRadioButton1,BorderLayout.CENTER);
contentPane.add(jRadioButton2,BorderLayout.NORTH);
contentPane.add(jRadioButton3,BorderLayout.SOUTH);
setBounds(100,100,400,400);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
多选框
public JButtonDemo1(){
Container contentPane = getContentPane();
//多选框
JCheckBox jCheckBox1 = new JCheckBox("checkBox01");
JCheckBox jCheckBox2 = new JCheckBox("checkBox02");
contentPane.add(jCheckBox1,BorderLayout.SOUTH);
contentPane.add(jCheckBox2,BorderLayout.NORTH);
setBounds(100,100,400,400);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
下拉框、列表框
列表框
public TestCombobox() {
setVisible(true);
setBounds(100,100,500,500);
Container contentPane = getContentPane();
//创建下拉框
JComboBox<Object> JComboBox = new JComboBox<>();
//添加下拉框的内容
JComboBox.addItem(null);
JComboBox.addItem("正在上映");
JComboBox.addItem("已下架");
JComboBox.addItem("即将上映");
contentPane.add(JComboBox,BorderLayout.NORTH);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
publicstaticvoidmain(String[]args){
newTestCombobox();
}
}
列表框
应用场景:选择地区,或者一些单个选项。列表,展示信息,一般是动态扩容
public TestCombobox() {
setVisible(true);
setBounds(100,100,500,500);
Container contentPane = getContentPane();
//列表框的内容
String[] contents={"1","2","3"};
//列表中需要放内容
//也可以放Vector
JList<Object> JList = new JList<>(contents);
contentPane.add(JList);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestCombobox();
}
文本框、密码框、文本域
文本框
public TestTextDemo1() {
Container contentPane = getContentPane();
//设置文本框
TextField textField = new TextField("hello");
TextField textField2 = new TextField("world",20);
contentPane.add(textField,BorderLayout.NORTH);
contentPane.add(textField2,BorderLayout.SOUTH);
setVisible(true);
setBounds(100,100,500,500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestTextDemo1();
}
密码框
public TestTextDemo1() {
Container contentPane = getContentPane();
//设置密码框
JPasswordField passwordField = new JPasswordField();
contentPane.add(passwordField);
setVisible(true);
setBounds(100,100,500,500);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestTextDemo1();
}
}
文本域
在滑动面板中有提到
一般配合滑动面板使用
//文本域
JTextArea jTextArea = new JTextArea(20,50);
jTextArea.setText("djsakhozxchasdouifhaofjhaodfj");
//JScroll面板
JScrollPane jScrollPane = new JScrollPane(jTextArea);
contentPane.add(jScrollPane);