程序线程进程

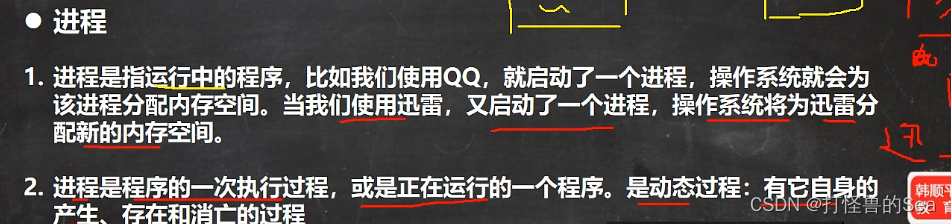
并发并行
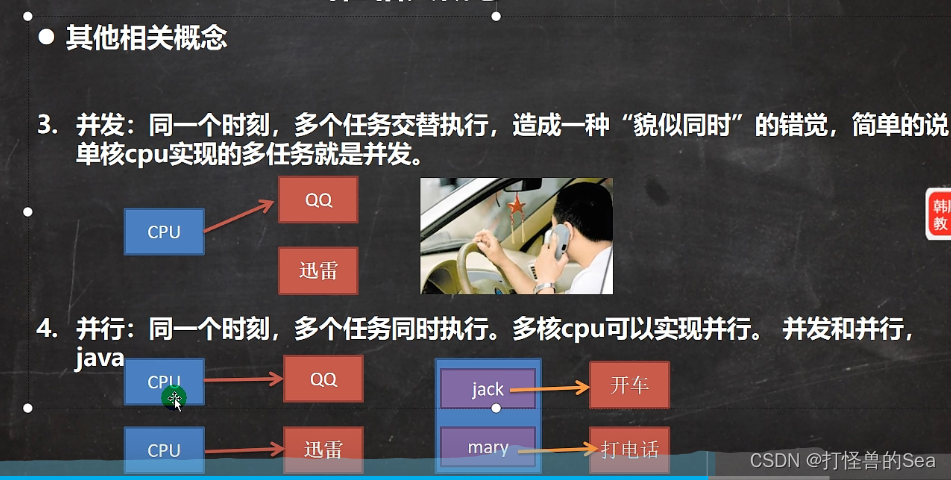
创建线程的基本方式(1)
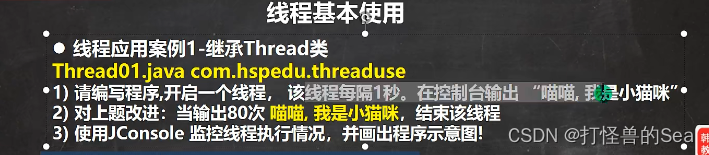
package com.edu.threaduse;
public class Demo01 {
public static void main(String[] args) throws InterruptedException {
//创建Cat对象,可以当线程使用
Cat cat = new Cat();
cat.start();//启动线程
//使用run的话是主线程里面的一个普通方法,只有run执行完毕才结束
//说明:当main线程启动一个子线程Thread-0,主线程不会阻塞,会继续执行
for (int i =0;i<10;i++){
System.out.println("主线程i="+i);
Thread.sleep(1000);
}
}
}
//通过继承Thread类创建线程
/*
当一个类继承类Thread类,该类就可以当做线程使用
我们会重写run方法,写上自己的业务代码
run Thread类实现Runnable接口的run方法
*/
class Cat extends Thread{
@Override
public void run() {
int time =0;
while(time++<80){
//重写run方法,写上自己的业务逻辑
//该线程每隔1秒,在控制台输出"喵喵,我是小猫咪";
System.out.println("喵喵,我是小猫咪"+"线程名称="+Thread.currentThread().getName());
//让线程休眠1s
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
创建线程的基本方式(2) 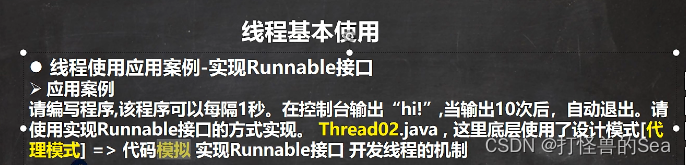
package com.edu.threaduse;
public class Demo02 {
public static void main(String[] args) {
// Dog dog = new Dog();
// //dog.start()无法使用
// Thread thread = new Thread(dog);
// thread.start();
Tiger tiger = new Tiger();
ThreadProxy threadProxy = new ThreadProxy(tiger);
threadProxy.start();
}
}
class Animal{}
class Tiger extends Animal implements Runnable{
@Override
public void run() {
System.out.println("老虎");
}
}
class ThreadProxy implements Runnable{ //看成一个Thread代理类
private Runnable target = null;//属性
@Override
public void run() {
if(target!=null){
target.run(); //运行类型为Tiger
}
}
public ThreadProxy(Runnable target) {
this.target = target;
}
public void start(){
start0();
}
public void start0(){
run();
}
}
class Dog implements Runnable{ //通过实现Runnable接口实现线程
@Override
public void run() {
while(true){
System.out.println("小狗叫");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
售票超卖
package com.edu.ticket;
public class SellTicket {
public static void main(String[] args) {
SellTick02 sellTick02 = new SellTick02();
new Thread(sellTick02).start();
new Thread(sellTick02).start();
new Thread(sellTick02).start();
}
}
//class SellTick01 extends Thread{
// private static int ticketNum = 100;
//
// @Override
// public void run() {
//
// while (true){
// if (ticketNum<=0){
// System.out.println("售票结束");
// break;
// }
// System.out.println("售出一张,还有:"+--ticketNum+"张");
// try {
// Thread.sleep(1000);
// } catch (InterruptedException e) {
// throw new RuntimeException(e);
// }
// }
// }
//}
class SellTick02 implements Runnable{
private int ticketNum = 100;
@Override
public void run() {
while (true){
if (ticketNum<=0){
System.out.println("售票结束");
break;
}
System.out.println("售出一张,还有:"+--ticketNum+"张");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
线程终止
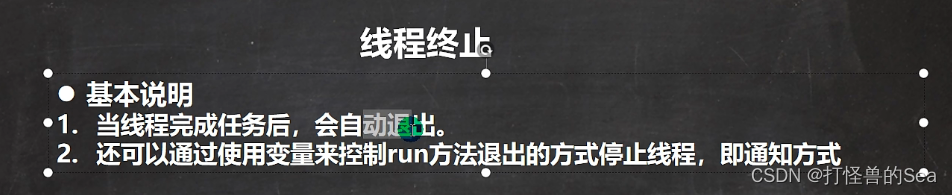
package com.edu.exit;
public class ThreadExit_ {
public static void main(String[] args) throws InterruptedException {
T t1 = new T();
t1.start();
//主线程休眠10s再通知
Thread.sleep(10*1000);
t1.setLoop(false);
}
}
class T extends Thread{
private boolean loop = true;
@Override
public void run() {
while(loop){
try {
Thread.sleep(50);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
System.out.println("AThread 运行中...");
}
}
//方便主线程控制
public void setLoop(boolean loop) {
this.loop = loop;
}
}
常用方法
package com.edu.method;
public class ThreadMethod_ {
public static void main(String[] args) throws InterruptedException {
T t = new T();
t.setName("老虎");
t.setPriority(Thread.MIN_PRIORITY);
System.out.println(t.getName());
t.start();
//主线程打印hi,然后中断子线程
for (int i =0;i<5;i++){
Thread.sleep(1000);
System.out.println("HI");
}
t.interrupt(); //中断t的休眠
}
}
class T extends Thread{
@Override
public void run() {
while (true){
for (int i =0;i<100;i++){
System.out.println(Thread.currentThread().getName()+"吃包子");
}
try {
System.out.println(Thread.currentThread().getName()+"休眠中");
Thread.sleep(20000);
} catch (InterruptedException e) {
//当线程执行道一个中断方法时,可以加入自己的业务代码
System.out.println(Thread.currentThread().getName()+"被中断了");
}
}
}
}
package com.edu.method;
public class ThreadMethod02 {
public static void main(String[] args) throws InterruptedException {
T1 t1 = new T1();
t1.start();
for (int i = 0;i<20;i++){
if(i==5){
Thread.yield();
//t1.join
}
System.out.println("HI"+i);
Thread.sleep(1000);
}
}
}
class T1 extends Thread{
private int loop = 20;
@Override
public void run() {
while (loop-->0){
System.out.println("Hello: "+loop);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
package com.edu.method;
public class ThreadMethod03 {
public static void main(String[] args) throws InterruptedException {
T3 t = new T3();
Thread t1 = new Thread(t);
for (int i = 1 ;i<=10;i++) {
System.out.println("hi:"+i);
if (i==5){
t1.start();
t1.join();
}
Thread.sleep(1000);
}
System.out.println("主进程结束");
}
}
class T3 implements Runnable{
@Override
public void run() {
for (int i=1;i<=10;i++){
System.out.println("HELLO:"+i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
System.out.println("子线程结束");
}
}
守护线程
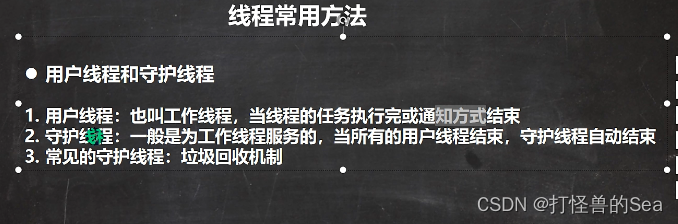
package com.edu.method;
import java.io.FileOutputStream;
public class ThreadMethod04 {
public static void main(String[] args) throws InterruptedException {
MyDaemonThread myDaemonThread = new MyDaemonThread();
myDaemonThread.setDaemon(true);
myDaemonThread.start();
for (int i = 0;i<=10;i++){
System.out.println("大家好");
Thread.sleep(1000);
}
}
}
class MyDaemonThread extends Thread{
@Override
public void run() {
for (;;){
try {
Thread.sleep(50);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
System.out.println("你好");
}
}
}
线程状态
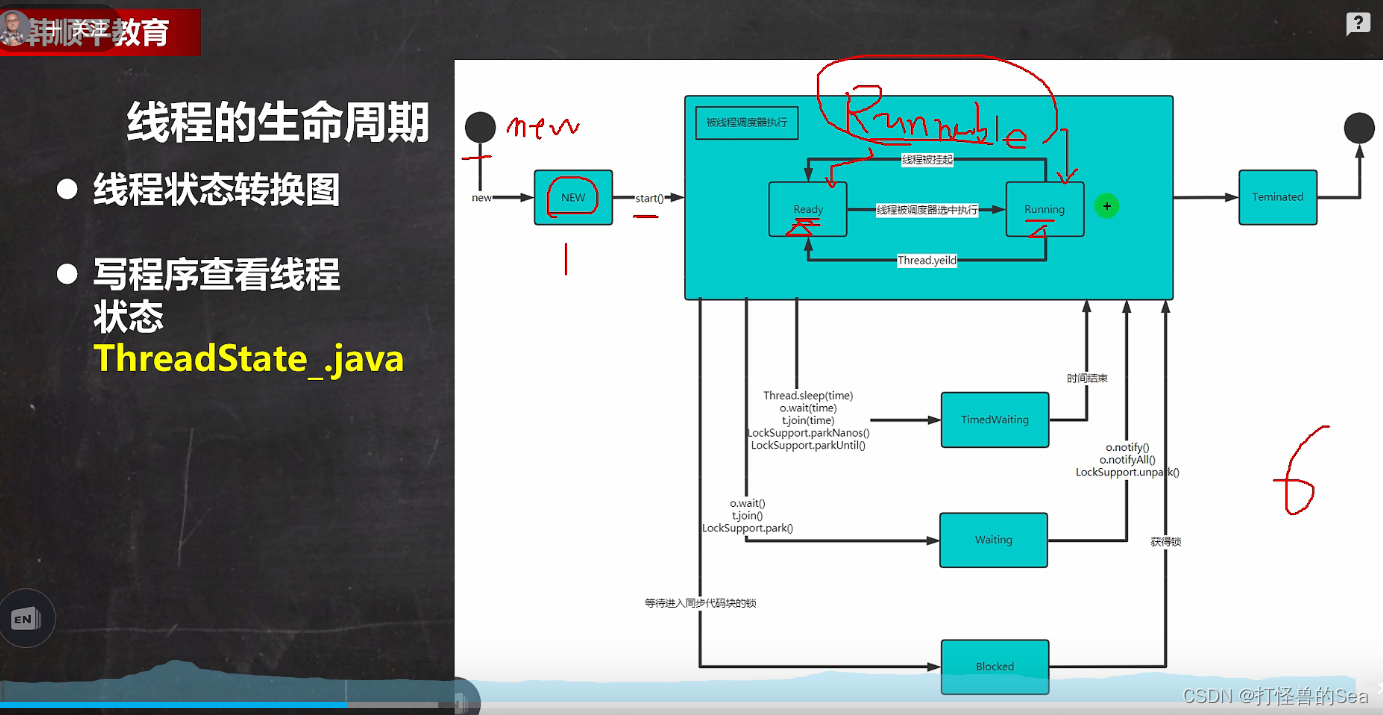
sleep不会释放锁 wait会
package com.edu.method;
public class ThreadState {
public static void main(String[] args) throws InterruptedException {
T5 t = new T5();
System.out.println(t.getName()+"状态"+t.getState());
t.start();
while (Thread.State.TERMINATED!=t.getState()){
System.out.println(t.getName()+"状态"+t.getState());
Thread.sleep(500);
}
System.out.println(t.getName()+"状态"+t.getState());
}
}
class T5 extends Thread{
@Override
public void run() {
while (true){
for (int i = 0 ;i<10;i++){
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
System.out.println("hi"+i);
}
break;
}
}
}
线程同步机制
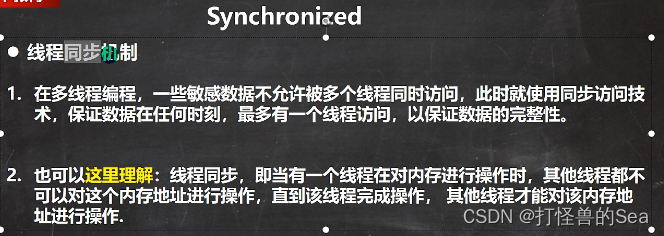
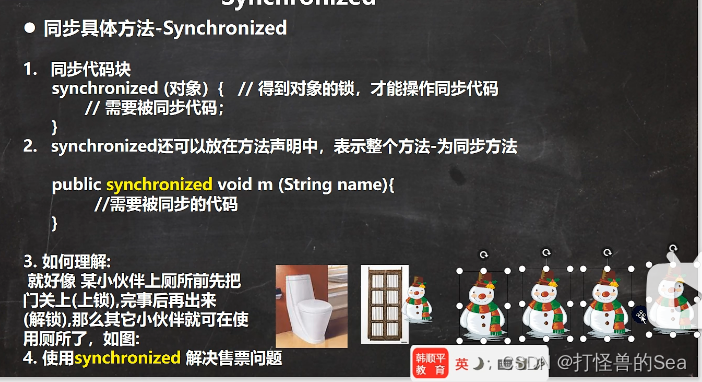
package com.edu.syn;
public class SellTicket {
public static void main(String[] args) {
SellTick03 sellTick02 = new SellTick03();
new Thread(sellTick02).start();
new Thread(sellTick02).start();
new Thread(sellTick02).start();
}
}
//class SellTick01 extends Thread{
// private static int ticketNum = 100;
//
// @Override
// public void run() {
//
// while (true){
// if (ticketNum<=0){
// System.out.println("售票结束");
// break;
// }
// System.out.println("售出一张,还有:"+--ticketNum+"张");
// try {
// Thread.sleep(1000);
// } catch (InterruptedException e) {
// throw new RuntimeException(e);
// }
// }
// }
//}
//实现接口方式,使用synchronized实现
class SellTick03 implements Runnable{
private boolean flag = true;
private int ticketNum = 100;
public /*synchronized*/ void m(){//同步方法
synchronized (this){ //代码块加锁
if (ticketNum<=0){
System.out.println("售票结束");
flag = false;
return;
}
System.out.println(Thread.currentThread().getName()+"售出一张,还有:"+--ticketNum+"张");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
//这里不能锁到while不然会一个窗口执行完全部
@Override
public void run() {
while (flag){
m();
}
}
}
互斥锁
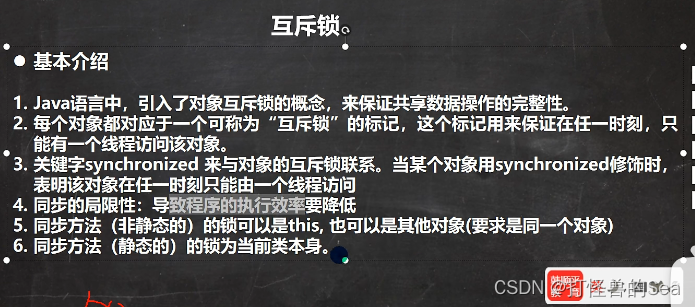
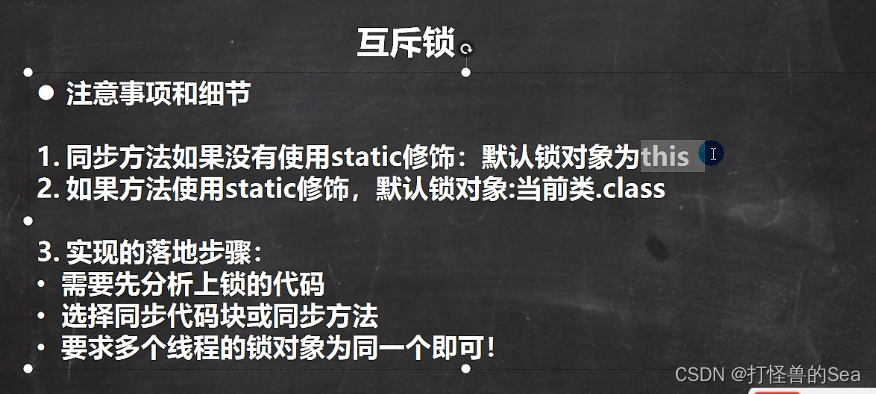
5、6可以参考同步(同步代码块synchronized(this) 同步方法 、全局锁、同步处理方法对比)_同步方法与基于this引用的同步代码块使用的锁-CSDN博客
线程死锁
释放锁
练习
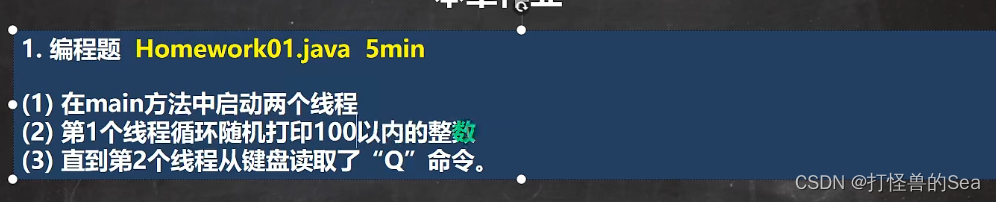
package com.edu.exer;
import com.sun.org.apache.bcel.internal.generic.NEW;
import java.util.Random;
import java.util.Scanner;
public class Homework01 {
public static void main(String[] args) {
Thread1 demo01 = new Thread1();
demo01.start();
Thread2 demo02 = new Thread2(demo01);
demo02.start();
}
}
class Thread1 extends Thread{
private boolean flag = true;
@Override
public void run() {
while (flag){
System.out.println((int) (Math.random()*100+1));
try {
Thread.sleep(500);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
public void setFlag(boolean flag) {
this.flag = flag;
}
}
class Thread2 extends Thread{
private Scanner scanner = new Scanner(System.in);
private char chr;
private Thread1 a;
public Thread2(Thread1 a){
this.a = a;
}
@Override
public void run() {
while (true){
System.out.println(Thread.currentThread().getName()+"启动");
chr = scanner.next().toUpperCase().charAt(0);
if (chr == 'Q'){
a.setFlag(false);
scanner.close();
break;
}
try {
Thread.sleep(500);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
package com.edu.exer;
public class HomeWork02 {
public static void main(String[] args) {
Card card = new Card();
User user1 = new User(card);
User user2 = new User(card);
user1.start();
user2.start();
}
}
class Card{
private int sum = 10000;
public void setSum(int sum) {
this.sum = sum;
}
public int getSum() {
return sum;
}
public void useMoney(){
this.sum-=1000;
}
}
class User extends Thread{
private Card card;
public User(Card card) {
this.card = card;
}
@Override
public void run() {
while (true){
synchronized (Card.class){ //静态方法推荐 也可以拿card来锁
if (card.getSum()>0){
card.useMoney();
System.out.println(Thread.currentThread().getName()+"使用钱 "+"还有:"+card.getSum()+"元");
}else {
System.out.println("余额不足");
break;
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
}
//老师的
package com.edu.exer;
public class HomeWork03 {
public static void main(String[] args) {
T6 t6 = new T6();
Thread thread1 = new Thread(t6);
Thread thread2 = new Thread(t6);
thread2.start();
thread1.start();
}
}
class T6 implements Runnable{
private int money=10000;
private Lock lk = new ReentrantLock(); //Lock的使用
@Override
public void run() {
//lk.lock();
while (true){
synchronized (this){ //锁对象也可以是字符串,只要确保在常量池中唯一即可
//this表示共享资源
if (money<1000){
System.out.println("余额不足");
break;
}
money-=1000;
System.out.println(Thread.currentThread().getName()+"取出1000 当前余额:"+money);
}
}
}
//lk.unlock()
}
线程池
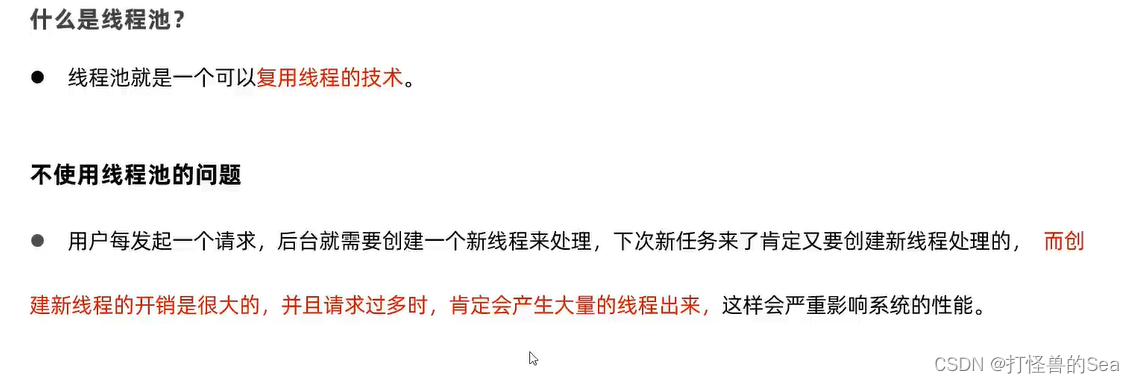
创建线程池
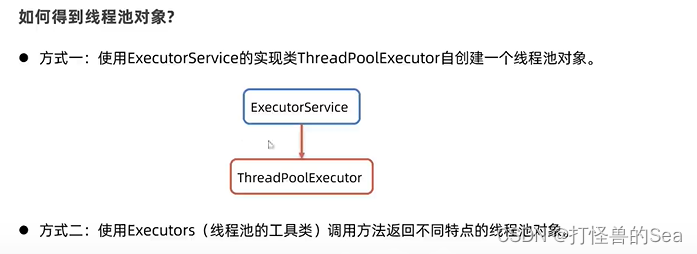
package com.edu.pool;
import java.util.concurrent.*;
public class Demo01 {
public static void main(String[] args) {
//通过ThreadPoolExecutor创建一个线程池对象
ExecutorService pool = new ThreadPoolExecutor(3,5,8,
TimeUnit.SECONDS,new ArrayBlockingQueue<>(4), Executors.defaultThreadFactory(),
new ThreadPoolExecutor.AbortPolicy());
Runnable target = new MyRunnable();
pool.execute(target); //线程池会自动创建一个新线程,自动处理这个任务
pool.execute(target);
pool.execute(target);
pool.execute(target);//复用
pool.execute(target);//复用
pool.shutdown();//等线程池任务全部执行完毕再关闭
pool.shutdownNow();//立即关闭
pool.execute(target); //wait
pool.execute(target); //wait
pool.execute(target); //wait
pool.execute(target);//队列
pool.execute(target);//队列
pool.execute(target);//队列
pool.execute(target);//队列
pool.execute(target); //临时启动
pool.shutdown();//等线程池任务全部执行完毕再关闭
pool.shutdownNow();//立即关闭
}
}
线程池处理Callable任务
package com.edu.pool;
import java.util.concurrent.*;
public class Demo01 {
public static void main(String[] args) {
//通过ThreadPoolExecutor创建一个线程池对象
ExecutorService pool = new ThreadPoolExecutor(3,5,8,
TimeUnit.SECONDS,new ArrayBlockingQueue<>(4), Executors.defaultThreadFactory(),
new ThreadPoolExecutor.CallerRunsPolicy());
Runnable target = new MyRunnable();
pool.execute(target);
MyCallable myCallable = new MyCallable(100);
Future<String> f1 = pool.submit(myCallable); //通过f1接返回的对象
}
}