目录
一、系统包含的技术:
后端:SpringBoot maven
前端:layui,js,css等
开发工具:IDEA
数据库:MySQL
JDK版本:jdk1.8
二、模块设计思路
用户端模块设计:首页、安全动态、政策法规、通知公告、安全教育评测、在线留言、个人中心;用户登录系统后可在心理评测页面进行答题评分,系统会根据答题情况进行分析,包括安全知识掌握优秀、良好、一般、很差
登录注册模块:密码加密、增加验证码、身份验证
管理端模块设计思路: 文章管理,留言管理,用户管理,心理测评,公告管理,系统设置
文章列表:文章预览、点击对应板块可进行查看
三、项目运行截图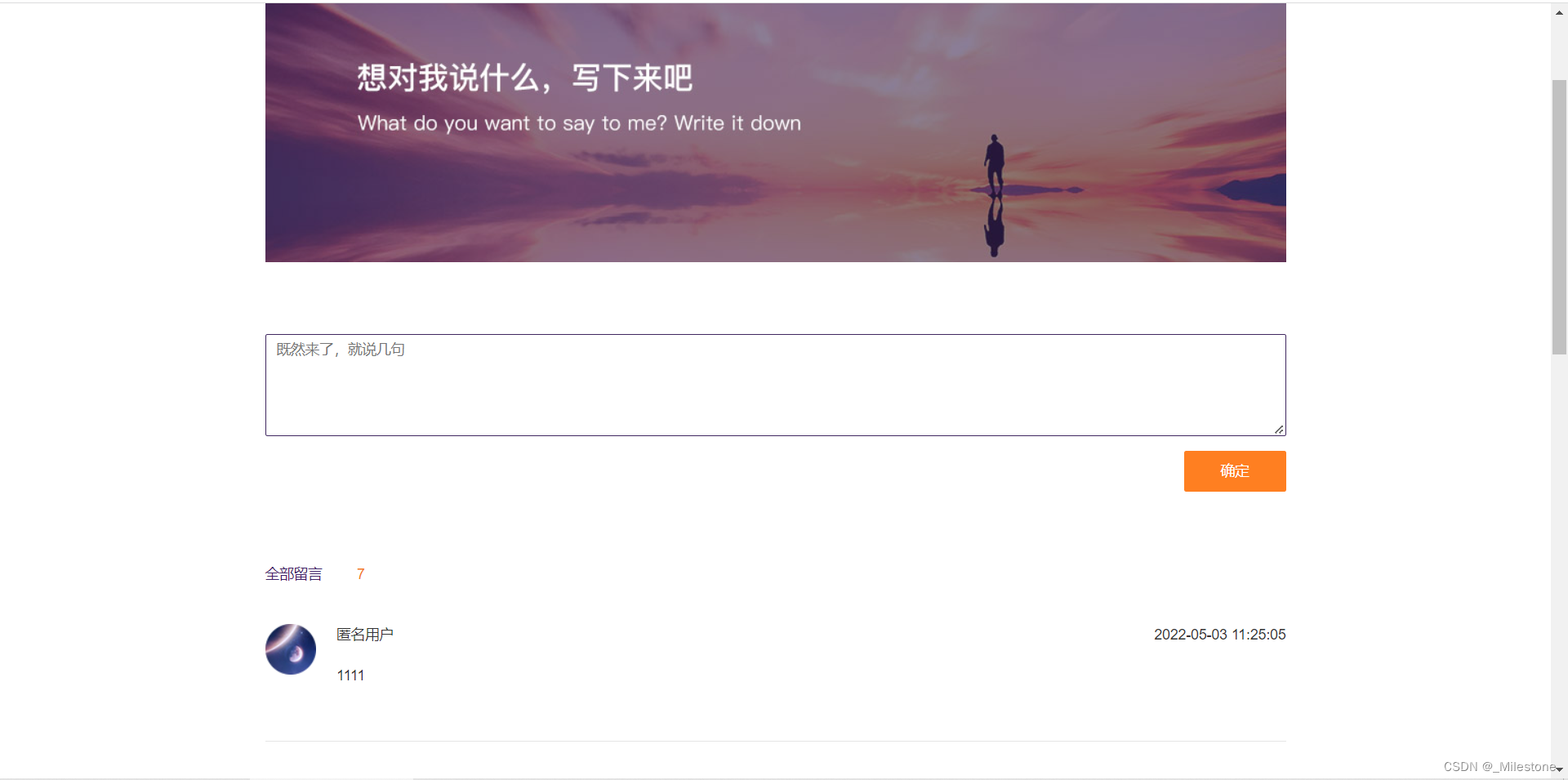
咨询老师板块:可以在线预约
安全知识测评板块:测试完成系统会出现对应的安全知识分数
留言板块:
老师界面:公告查询、公告添加、查看、编辑、删除等;
四、项目代码分析
验证码框架配置
package com.yuanlrc.xinli.config;
import com.google.code.kaptcha.Producer;
import com.google.code.kaptcha.util.Config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.Properties;
/**
* 验证码框架配置
* @author hairong
*/
@Configuration
public class KaptchaConfig {
@Bean
public Producer KaptchaProducer() {
Properties kaptchaProperties = new Properties();
kaptchaProperties.put("kaptcha.border", "no");
kaptchaProperties.put("kaptcha.textproducer.char.length", "4");
kaptchaProperties.put("kaptcha.textproducer.char.space", "6");
kaptchaProperties.put("kaptcha.image.height", "80");
kaptchaProperties.put("kaptcha.obscurificator.impl", "com.google.code.kaptcha.impl.WaterRipple");
kaptchaProperties.put("kaptcha.textproducer.font.color", "blue");
kaptchaProperties.put("kaptcha.textproducer.font.size", "60");
kaptchaProperties.put("kaptcha.noise.impl", "com.google.code.kaptcha.impl.DefaultNoise");
kaptchaProperties.put("kaptcha.textproducer.char.string", "123456789");
Config config = new Config(kaptchaProperties);
return config.getProducerImpl();
}
}
拦截器
package com.yuanlrc.xinli.config;
import com.yuanlrc.xinli.controller.login.CheckLoginInterceptor;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/**
* 配置类
*
* @author hairong
*/
@Configuration
public class LoginConfiguration implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**").addResourceLocations("classpath:/static/");
WebMvcConfigurer.super.addResourceHandlers(registry);
}
/**
* 注册拦截器
*
* @param registry
*/
@Override
public void addInterceptors(InterceptorRegistry registry) {
CheckLoginInterceptor interceptor = new CheckLoginInterceptor();
InterceptorRegistration interceptorRegistration = registry.addInterceptor(interceptor);
//拦截路径
interceptorRegistration.addPathPatterns("/**");
// 排除路径
interceptorRegistration.excludePathPatterns("/defaultKaptcha/**");
interceptorRegistration.excludePathPatterns("/login/**");
interceptorRegistration.excludePathPatterns("/article/list");
interceptorRegistration.excludePathPatterns("/article/newsList");
interceptorRegistration.excludePathPatterns("/article/activityList");
interceptorRegistration.excludePathPatterns("/article/mentalList");
interceptorRegistration.excludePathPatterns("/article/get");
interceptorRegistration.excludePathPatterns("/consult/**");
interceptorRegistration.excludePathPatterns("/yuyue/**");
interceptorRegistration.excludePathPatterns("/leacots/**");
interceptorRegistration.excludePathPatterns("/index");
interceptorRegistration.excludePathPatterns("/teacher/**");
interceptorRegistration.excludePathPatterns("/student/**");
interceptorRegistration.excludePathPatterns("/**");
interceptorRegistration.excludePathPatterns("/leacotsUi");
interceptorRegistration.excludePathPatterns("/noticeClientUi/list");
interceptorRegistration.excludePathPatterns("/noticeClientUi/get");
interceptorRegistration.excludePathPatterns("/login_do");
interceptorRegistration.excludePathPatterns("/loginout");
interceptorRegistration.excludePathPatterns("/articleUi1");
interceptorRegistration.excludePathPatterns("/articleUiAdd");
interceptorRegistration.excludePathPatterns("/admin/**");
// 排除静态资源请求
interceptorRegistration.excludePathPatterns("/static/upload/**");
interceptorRegistration.excludePathPatterns("/layuiadmin/**");
interceptorRegistration.excludePathPatterns("/res/**");
interceptorRegistration.excludePathPatterns("/webjars/**");
interceptorRegistration.excludePathPatterns("/photo/**");
interceptorRegistration.excludePathPatterns("/upload/**");
}
}
全局异常处理
package com.yuanlrc.xinli.exception;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.Map;
/**
* 统一异常处理
*
* @author hairong
*/
@ControllerAdvice
public class GlobalException {
@ExceptionHandler(value = Exception.class)
@ResponseBody
private Map<String, Object> exceptionHandler(HttpServletRequest request, Exception e) {
Map<String, Object> modelMap = new HashMap<String, Object>();
modelMap.put("success", false);
modelMap.put("errMsg", e.getMessage());
return modelMap;
}
}
………
五、项目优化升级
前端:三件套 + React + 组件库 Ant Design + Umi + Ant Design Pro(现成的管理系统)
后端:
-
java
-
spring(依赖注入框架,帮助你管理 Java 对象,集成一些其他的内容)
-
springmvc(web 框架,提供接口访问、restful接口等能力)
-
mybatis(Java 操作数据库的框架,持久层框架,对 jdbc 的封装)
-
mybatis-plus(对 mybatis 的增强,不用写 sql 也能实现增删改查)
-
springboot(快速启动 / 快速集成项目。不用自己管理 spring 配置,不用自己整合各种框架)
-
junit 单元测试库
-
mysql
部署:服务器 / 容器(平台)
六、结语
感谢大家的阅读!