游戏截图:
代码如下:
第1个文件:StartGames.java
package com.cao.snake;
import javax.swing.*;
public class StartGames {
public static void main(String[] args) {
//1静态窗口
JFrame frame=new JFrame("贪吃狗");
frame.setBounds(100,100,1500,1300);
frame.setResizable(false);//大小不可变
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//关闭
//2面板
frame.add(new GamePanel());
frame.setVisible(true);
}
}
第2个文件:Date.java
package com.cao.snake;
import javax.swing.*;
import java.net.URL;
public class Date {
//头图片
public static URL headURL=Date.class.getResource("/statics/head.jpg");
public static ImageIcon header=new ImageIcon(headURL);
//头部:
public static URL upURL=Date.class.getResource("/statics/up.jpg");
public static ImageIcon up=new ImageIcon(upURL);
public static URL rightURL=Date.class.getResource("/statics/right.jpg");
public static ImageIcon right=new ImageIcon(rightURL);
public static URL leftURL=Date.class.getResource("/statics/left.jpg");
public static ImageIcon left=new ImageIcon(leftURL);
public static URL downURL=Date.class.getResource("/statics/down.jpg");
public static ImageIcon down=new ImageIcon(downURL);
//身体
public static URL bodyURL=Date.class.getResource("/statics/body.jpg");
public static ImageIcon body=new ImageIcon(bodyURL);
//实物
public static URL foodURL=Date.class.getResource("/statics/food.jpg");
public static ImageIcon food=new ImageIcon(foodURL);
}
第3个文件:GamePanl.java
package com.cao.snake;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
public class GamePanel extends JPanel implements KeyListener,ActionListener{
int len;//蛇的长度
int[] sx = new int[1000]; //放蛇的数组
int[] sy=new int[1000];//蛇y坐标
boolean isStar=false;//游戏是否开始
String fx;//方向
//死亡判断
boolean isFail=false;
//积分
int sc;
//随机数和食物
Random r=new Random();
int foodx;
int foody;
Timer timer=new Timer (200, this);
//构造器
public GamePanel(){
init();
//获取键盘监听事件
this.setFocusable(true);
this.addKeyListener(this);
timer.start();
}
//初始化
public void init() {
len=3;
sx[0]=600;sy[0]=600;
sx[1]=550;sy[1]=600;
sx[2]=500;sy[2]=600;
fx="R";
//食物坐标
foodx=50*r.nextInt(29);
foody=300+50*r.nextInt(19);
System.out.println(foodx);
System.out.println(foody);
sc=0;
}
//画板
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);//清屏
this.setBackground(Color.black);//背景黑色
//绘制头部广告栏
Date.header.paintIcon(this,g,0,0);
//绘制游戏区域
g.fillRect(0,300,1500,1000);
//画一条蛇
if (fx.equals("R")){
Date.right.paintIcon(this,g,sx[0],sy[0]);
}else if(fx.equals("L")){
Date.left.paintIcon(this,g,sx[0],sy[0]);
}else if(fx.equals("U")){
Date.up.paintIcon(this,g,sx[0],sy[0]);
}else if(fx.equals("D")){
Date.down.paintIcon(this,g,sx[0],sy[0]);
}
//画身体
for(int i=1;i<len;i++) {
Date.body.paintIcon(this, g, sx[i],sy[i]);
}
//画积分
g.setColor(Color.white);
g.setFont(new Font("微软雅黑",Font.BOLD,40));//字体
g.drawString("积分:"+sc,1000,150);
//画食物
Date.food.paintIcon(this,g,foodx,foody);
//提示是否开始
if(isStar==false){
g.setColor(Color.WHITE);
g.setFont(new Font("微软雅黑",Font.BOLD,40));//字体
g.drawString("按下空格开始/暂停!",600,700);
}
//死亡提醒
if (isFail){
g.setColor(Color.RED);
g.setFont(new Font("微软雅黑",Font.BOLD,50));//字体
g.drawString("游戏失败,按空格重新开始!!",600,700);
}
}
//键盘监听
@Override
public void keyPressed(KeyEvent e) {
// 键盘按下未释放
int keyCode=e.getKeyCode();
//按下空格键
if(keyCode==KeyEvent.VK_SPACE){
if(isFail){//失败
isFail=false;
init();//
}else {
isStar = !isStar;
}
repaint();//刷新界面
}
//键盘控制走向
if(keyCode==KeyEvent.VK_LEFT){
fx="L";
}else if (keyCode==KeyEvent.VK_RIGHT){
fx="R";
}else if(keyCode==KeyEvent.VK_UP){
fx="U";
}else if(keyCode==KeyEvent.VK_DOWN){
fx="D";
}
}
//定时器
@Override
public void actionPerformed(ActionEvent e) {
//如果游戏开始
if(isStar&&isFail==false){
for (int i=len-1;i>0;i--){//身体移动
sx[i]=sx[i-1];
sy[i]=sy[i-1];
}
//方向控制头部移动
if (fx.equals("R")) {
sx[0] = sx[0] + 50;//头移动
if (sx[0] > 1500) {//边界判断
sx[0] = 0;
}
}else if(fx.equals("L")){
sx[0] = sx[0] - 50;//头移动
if (sx[0] <0 ) {//边界判断
sx[0] = 1500;
}
}else if(fx.equals("U")){
sy[0] = sy[0] - 50;//头移动
if (sy[0] < 300 ) {//边界判断
sy[0] = 1300;
}
}else if(fx.equals("D")){
sy[0] = sy[0] + 50;//头移动
if (sy[0] >1300 ) {//边界判断
sy[0] = 300;
}
}
//如果小蛇头部和实物重合
if(sx[0]==foodx&&sy[0]==foody){
//积分+1
sc++;
//长度+1
len++;
//重新生成实物
foodx=50*r.nextInt(29);
foody=300+50*r.nextInt(19);
}
//结束判断
for (int i=1;i<len;i++){
if(sx[0]==sx[i]&&sy[0]==sy[i]){
isFail=true;
}
}
//刷新
repaint();
}
timer.start();
}
@Override
public void keyTyped(KeyEvent e) {
// 键盘按下
}
@Override
public void keyReleased(KeyEvent e) {
// 键盘按下弹起
}
}
文件目录结构如下:
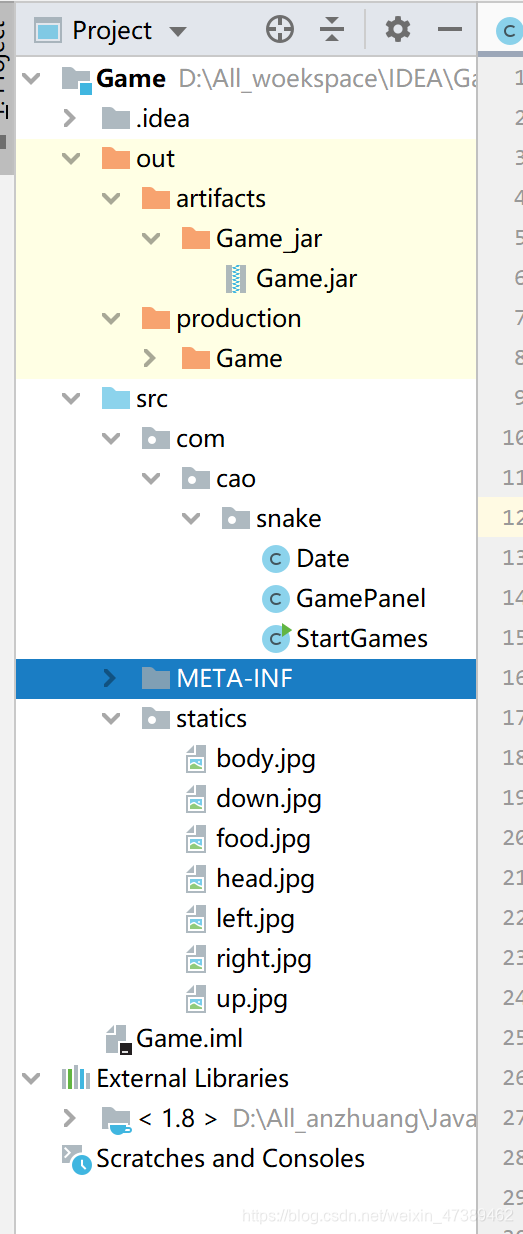