vue 之 商品列表的左右联动
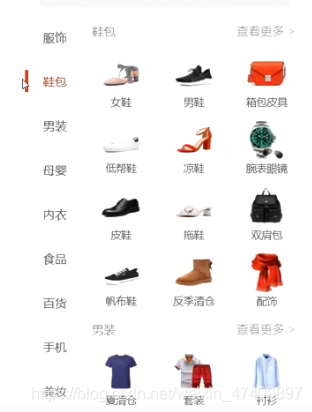
思路
- 1:初始化的时候,左右两侧实现 可以滚动
- 监听右侧的滚动事件,把具体的滚动y轴的值,赋值给 scrollY
- 2:然后计算出右侧的每一个板块的距离顶部高度,为一个高度数组
- 3:计算属性之中,设置当前高亮 返回当前索引
- 需要找到当前索引,然后根据这个高度数组,和scrollY进行比较,需要返回那个索引
- 4:点击左侧切换的时候,拿当前的索引号,然后再拿到右侧的滚动位置,赋值给scrollY,最后调用右侧的滚动事件
- 5:右侧滚动时候,实现左侧的联动,
- 先拿到当前索引(这需要在计算属性之中的,获取当前索引内调用)
- 然后拿到右侧的 每一个li中的ul父节点,这个一个外容器
- 在拿到当前的节点,最后滚动到该节点
实现代码
<!-- -->
<template>
<div class="chat_wrap">
<div class="shop">
<div class="menu_wrap">
<ul>
<li
v-for="(item, index) in leftD"
:key="index"
:class="{ current: index === currentIndex }"
@click="clickLeftItem(index)"
ref="meneList"
>
<span>{{ item }}</span>
</li>
</ul>
</div>
<div class="shop_wrap">
<ul ref="shopListP">
<li class="shopList" v-for="item in rightD" :key="item.title">
<div class="shop_title">
<div class="title_t">
<h4>{{ item.title }}</h4>
<a href="###">{{ item.msg }}</a>
</div>
<ul>
<li v-for="items in item.list" :key="items">
<img :src="items.imgUrl" alt />
<span>{{ items.title }}</span>
</li>
</ul>
</div>
</li>
</ul>
</div>
</div>
</div>
</template>
<script>
import BScroll from "better-scroll";
export default {
name: "Chat",
data() {
return {
scrollY: 0,
rightItemTop: [],
};
},
watch: {
},
mounted() {
this.$nextTick(() => {
this.init();
this.rightListTop();
});
},
computed: {
currentIndex() {
const { scrollY, rightItemTop } = this;
return rightItemTop.findIndex((LiTop, index) => {
this._leftScroll(index);
return scrollY >= LiTop && scrollY < rightItemTop[index + 1];
});
}
},
methods: {
init() {
this.leftScroll = new BScroll(".menu_wrap", {
scrollY: true,
click: true
});
this.rightScroll = new BScroll(".shop_wrap", {
scrollY: true,
click: true,
probeType: 3
});
this.rightScroll.on("scrollEnd", pos => {
this.scrollY = Math.abs(pos.y);
});
},
rightListTop() {
const tempArr = [];
let top = 0;
tempArr.push(top);
let allList = this.$refs.shopListP.querySelectorAll(".shopList");
allList.forEach(item => {
top += item.clientHeight;
tempArr.push(top);
});
this.rightItemTop = tempArr;
},
clickLeftItem(index) {
this.scrollY = this.rightItemTop[index];
this.rightScroll.scrollTo(0, -this.scrollY, 300);
},
_leftScroll(index) {
let meneList = this.$refs.meneList;
let el = meneList[index];
this.leftScroll.scrollToElement(el, 300, 0, -100);
}
},
components: {}
};
</script>
<style lang="scss" scoped>
ul,
li {
list-style: none;
margin: 0;
padding: 0;
}
.chat_wrap {
padding: 0;
margin: 0;
width: 100%;
height: 100%;
overflow: hidden;
.shop {
width: 100%;
height: 100%;
display: flex;
overflow: hidden;
.menu_wrap {
width: 75px;
height: 100%;
background: #ccc;
& > ul > li {
width: 75px;
height: 60px;
background: #fafafa;
span {
display: flex;
justify-content: center;
align-items: center;
width: 100%;
height: 100%;
}
}
.current {
position: relative;
color: red;
&::before {
position: absolute;
left: 0;
top: 15px;
content: "";
height: 30px;
background: red;
width: 4px;
}
}
}
.shop_wrap {
width: 300px;
height: 100%;
overflow-x: hidden;
overflow-y: scroll;
.shop_title {
& > .title_t {
display: flex;
justify-content: space-between;
align-items: center;
}
& > ul {
display: flex;
flex-wrap: wrap;
}
& > ul > li {
width: 50%;
height: 130px;
margin-bottom: 5px;
background: pink;
img {
width: 100%;
height: 85%;
}
span {
display: block;
height: 20px;
line-height: 20px;
color: #fff;
text-align: center;
background: #000;
}
}
}
}
}
}
</style>
leftD: [
"女装",
"鞋包",
"T恤",
"靴子",
"男装",
"珠宝",
"首饰",
"鞋子",
"帽子",
"水果",
"家电",
"蓝莓",
"五金"
],
rightD: [
{
title: "女装",
msg: "查看更多",
list: [
{
title: "女装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "女装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "女装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "女装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "鞋包",
msg: "查看更多",
list: [
{
title: "鞋包",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋包",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋包",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋包",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "T恤",
msg: "查看更多",
list: [
{
title: "T恤",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "T恤",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "T恤",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "T恤",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "靴子",
msg: "查看更多",
list: [
{
title: "靴子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "靴子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "靴子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "靴子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "男装",
msg: "查看更多",
list: [
{
title: "男装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "男装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "男装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "男装",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "珠宝",
msg: "查看更多",
list: [
{
title: "珠宝",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "珠宝",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "珠宝",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "珠宝",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "首饰",
msg: "查看更多",
list: [
{
title: "首饰",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "首饰",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "首饰",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "首饰",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "鞋子",
msg: "查看更多",
list: [
{
title: "鞋子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "鞋子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "帽子",
msg: "查看更多",
list: [
{
title: "帽子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "帽子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "帽子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "帽子",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "水果",
msg: "查看更多",
list: [
{
title: "水果",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "水果",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "水果",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "水果",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "家电",
msg: "查看更多",
list: [
{
title: "家电",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "家电",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "家电",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "家电",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "蓝莓",
msg: "查看更多",
list: [
{
title: "蓝莓",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "蓝莓",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "蓝莓",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "蓝莓",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
},
{
title: "五金",
msg: "查看更多",
list: [
{
title: "五金",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "五金",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "五金",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
},
{
title: "五金",
imgUrl:
"https://t00img-c.yangkeduo.com/goods/images/2020-08-13/ce99a1b2f665ba478d9573c314686c5d.jpeg"
}
]
}
]