ele之Table表格的封装
ListTable.vue
- this.$refs.listTable.rowIndex = ‘’; // 清空当前选中的
<template>
<div class='table-wrap'>
<el-table
v-loading='isLoading'
ref="listTable"
:class="`list-table`"
:data="data.tableData"
border
stripe
height="100%"
:header-row-class-name="headerColor"
:summary-method="summaryMethod"
:show-summary="showSummary"
:span-method="spanMethod"
:cell-class-name="cellClassName"
:header-cell-class-name="`list-table-header`"
@select="handleSelectionChange"
style="width: 100%"
@row-click="rowClick"
:row-class-name="tableRewClassName">
<el-table-column
v-if="isSelect"
type="selection"
align="center"
width="50">
</el-table-column>
<el-table-column
v-if="isSerial"
type="index"
:label="'序号'"
width="50">
</el-table-column>
<el-table-column
v-for="(item, index) in data.tableCol"
:key="index"
:show-overflow-tooltip="true"
:align="item.align"
:prop="item.prop"
:label="item.label"
:width="item.width">
<template slot-scope="scope">
{{thousand(scope.row[item.prop])}}
</template>
</el-table-column>
<!-- 多级表头插槽 示例:<template v-slot:multistageHeader></template> -->
<slot name="multistageHeader"></slot>
<el-table-column :label="operationText" v-if="isOperation">
<!-- 操作按钮需要放在模板标签里面: 例:"<template scope="scopeVal" v-slot:operation><button @click="edit(scopeVal)">编辑</button></template>" -->
<template slot-scope="scope" >
<slot :scope="scope" name="operation"></slot>
</template>
</el-table-column>
</el-table>
<!-- 分页 -->
<div class="clear_fix page-bottom" v-if="pageData">
<div class="page-wrap">
<span style="font-size: 14px">第{{serialNumber}}条/总共{{pageData.total}}条</span>
<el-pagination
class="page"
@size-change="handleSizeChange"
@current-change="handleCurrentChange"
:current-page="pageData.currentPage"
:page-sizes="[5, 10, 15, 20]"
:page-size="pageData.pageSize"
layout="prev, pager, next, sizes"
:total="pageData.total">
</el-pagination>
</div>
</div>
</div>
</template>
<script>
/**
* list-table
*
* @module components/list-table
* @desc 表格列表
* @param data {Object} - tableData 表格内容列表并设置align对齐方式 tableCol 表格标题列表 必传
* @param isLoading {Boolean} - 请求数据时是显示loading 默认不显示(false)
* @param isSerial {Boolean} - 是否显示序号 默认不显示(false)
* @param isRowClick {Boolean} - 是否选显示选中行 高亮红色框默认不显示(false)
*
* @param showSummary {Boolean} - 是否在表尾显示合计行 默认不显示(false)
* @param summaryMethod {Funtion} - 传入一个方法定义自己的合计逻辑
* @param spanMethod {Funtion} - 传入一个方法定义自己的合并单元格逻辑
* @param cellClassName {Funtion} - 传入一个方法定义自己的合并单元格后的样式逻辑
*
* button
* @param isOperation {Boolean} - 是否需要操作按钮
* @param operationText {String} - `操作`标题改
* Select
* @param isSelect {Boolean} - 是否需要选择多行数据时使用 Checkbox(false)
* @param selectData {Array} - selectData 选中的数组 初始化选择
* @methods tableMultipleSelection 多选方法回调 ((当前操作的数据row, 是否勾选isPick) )
*
import {numStandard} from "@/utils/tool.js"
export default {
name: 'table-wrap',
components: {},
props: {
data: {
type: Object,
required: true
},
isLoading: {
type: Boolean,
default: false
},
isSerial: {
type: Boolean,
default: false
},
showSummary: {
type: Boolean,
default: false
},
summaryMethod: {
type: Function
},
spanMethod: {
type: Function
},
cellClassName: {
type: Function
},
isOperation: {
type: Boolean,
default: false
},
operationText: {
type: String,
default: '操作'
},
isSelect: {
type: Boolean,
default: false
},
selectData: {
type: Array
},
pageData: {
type: Object
},
isRowClick:{
type: Boolean,
default: false
}
},
computed: {
serialNumber() {
let pageData = this.pageData
return `${(pageData.currentPage - 1) * pageData.pageSize + 1}-${(pageData.currentPage * pageData.pageSize) > pageData.total ? pageData.total: (pageData.currentPage * pageData.pageSize)} `
}
},
watch:{
data(val, old) {
this.selectInit()
}
},
data () {
return {
rowIndex: '' // 当前选中的
};
},
methods: {
thousand(val, type) {
return typeof(val)=== 'number'? numStandard(val) : val
},
headerColor({row, column, rowIndex, columnIndex}){
if (rowIndex > 0) return 'header-color'
},
handleSizeChange(val) {
this.$emit('changSize', val)
},
handleCurrentChange(val) {
this.$emit('changeCurrent', val)
},
handleSelectionChange(val, row) {
let isPick = val.findIndex(oo => oo.id === row.id) > -1;
this.$emit("tableMultipleSelection", row, isPick);
},
selectInit() {
let that= this
if (this.selectData) {
setTimeout(() => {
this.data.tableData.map(item => {
let hasPicked = this.selectData.findIndex(it => it.id === item.id) > -1;
if (hasPicked) {
that.$refs.listTable.toggleRowSelection(item);
}
})
})
}
},
tableRewClassName({row, rowIndex}){
if (!this.isRowClick) return
row.index = rowIndex
if (this.rowIndex === rowIndex ) return 'row-style'
},
rowClick(row){
if (!this.isRowClick) return
this.rowIndex = row.index;
this.$emit("rowClick", row)
}
},
mounted () {
this.selectInit()
}
}
</script>
<style lang="scss" scoped>
.table-wrap{
width: 100%;
height: 100%;
.page-bottom{
margin-top: 8px;
}
.page-wrap{
float: right;
display: flex;
line-height: 32px;
::v-deep .el-pager li.active {
color: #e82323;
border: solid 1px #e82323;
}
::v-deep .el-pager li {
border: solid 1px transparent;
border-radius: 2px;
font-weight: 400;
color: #1a1a1e;
margin: 0 4px;
font-size: 14px;
min-width: 20px;
}
}
.list-table{
height: calc(100% - 50px);
overflow: auto;
}
::v-deep .row-style {
position: relative;
&::after {
content: '';
position: absolute;
left: 0;
right: 0;
z-index: 100;
width: 100%;
height: 100%;
border: 1px solid #e82323;
}
// border: 1px solid #ff0;
// box-shadow: inset 0px 0px 6px 0px rgba(255, 0, 0, .6);
}
::v-deep .el-table--striped .el-table__body tr.el-table__row--striped td{
background: #d5dffc;
}
::v-deep .list-table-header{
background: #e82323;
color: #fff ;
text-align: center;
// text-align: center ;
}
::v-deep .header-color{
.list-table-header{
background: #133891;
}
}
::v-deep .el-table__row--striped td{
background: #d5dffc;
}
::v-deep .has-gutter {
font-weight: bold;
}
::v-deep .el-table .cell{
line-height: 15px;
}
}
</style>
简单的使用
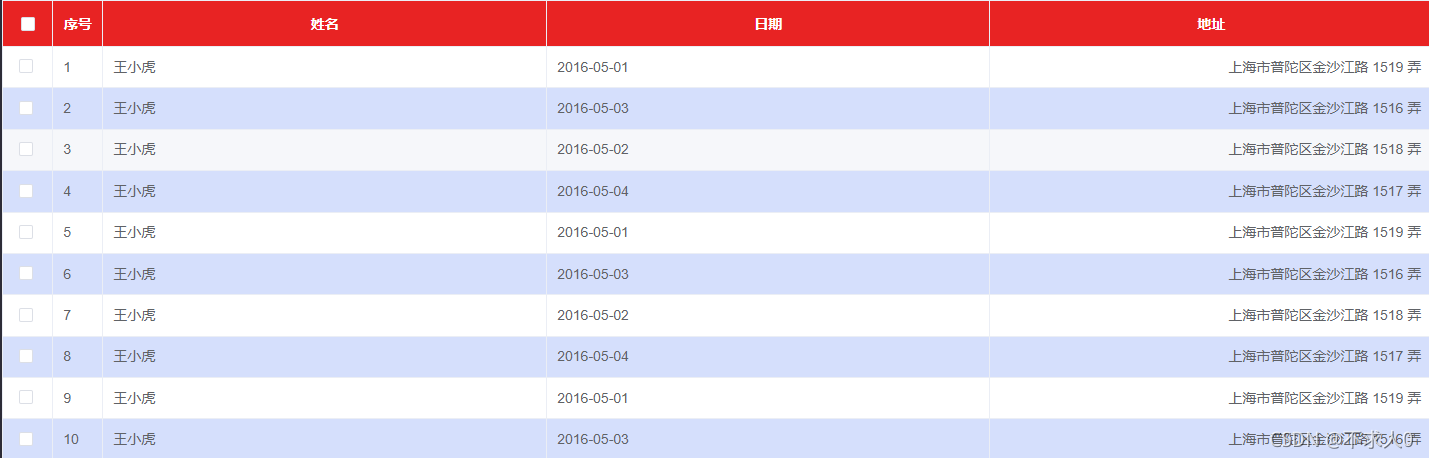
<template>
<div style="height: 100%">
<div class="tab_sec">
<list-table :data="TestData" isSerial isSelect />
</template>
<script>
import listTable from "@/components/commom/conTable/listTable.vue";
export default {
components:{
listTable
},
data() {
return {
TestData:{
tableData: [{
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
},{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
},{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
}
],
tableCol : [
{
label: "姓名",
prop: "name",
showOverFlowTooltip: true,
},
{
label: "日期",
prop: "date",
showOverFlowTooltip: true,
},
{
label: "地址",
prop: "address",
align: "right",
showOverFlowTooltip: true,
}
]
},
}
};
</script>
带有操作按钮的table
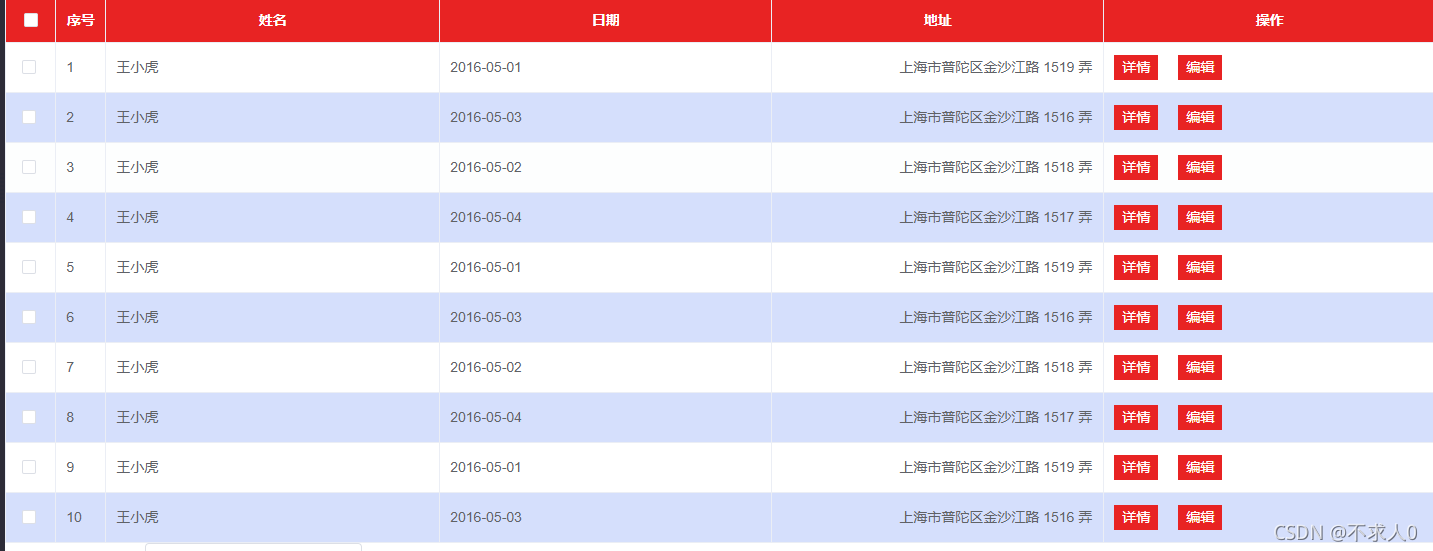
<template>
<div style="height: 100%">
<div class="tab_sec">
<list-table :data="TestData" isSerial isSelect isOperation operationText="操作" >
<template v-slot:operation="scopeVal">
<button class="blue" @click="view(scopeVal.scope.row)">详情</button>
<button class="blue" @click="edit(scopeVal.scope.row)">编辑</button>
</template>
</list-table>
</div>
</div>
</template>
<script>
import listTable from "@/components/commom/conTable/listTable.vue";
export default {
name: "TestTable",
components:{
listTable
},
data() {
return {
TestData:{
tableData: [{
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
},{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
},{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
}
],
tableCol : [
{
label: "姓名",
prop: "name",
showOverFlowTooltip: true,
},
{
label: "日期",
prop: "date",
showOverFlowTooltip: true,
},
{
label: "地址",
prop: "address",
align: "right",
showOverFlowTooltip: true,
}
]
},
}
},
methods: {
},
};
</script>
<style lang="scss" scoped>
.blue {
padding: 5px 8px;
background: #E82323;
margin-right: 20px;
}
</style>
多行表头table
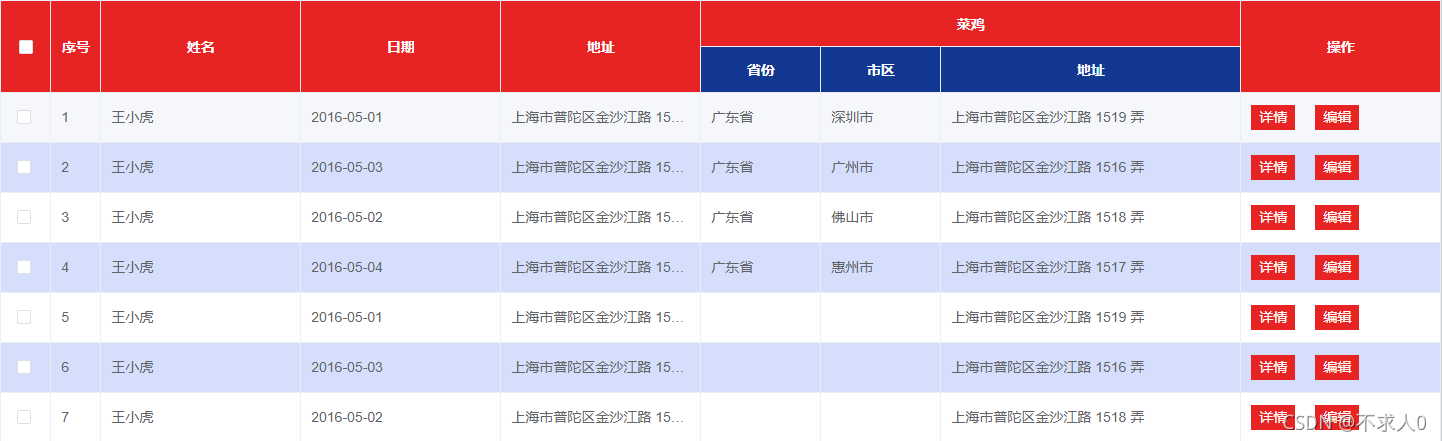
<template>
<div style="height: 100%">
<div class="tab_sec">
<list-table
:data="TestData"
isSerial
isSelect
isOperation
operationText="操作"
>
<template v-slot:multistageHeader>
<el-table-column label="菜鸡" width="120">
<el-table-column prop="province" label="省份" width="120">
</el-table-column>
<el-table-column prop="city" label="市区" width="120">
</el-table-column>
<el-table-column prop="address" label="地址" width="300">
</el-table-column>
</el-table-column>
</template>
<template v-slot:operation="scopeVal">
<button class="blue" @click="view(scopeVal.scope.row)">详情</button>
<button class="blue" @click="edit(scopeVal.scope.row)">编辑</button>
</template>
</list-table>
</div>
</div>
</template>
<script>
import listTable from "@/components/commom/conTable/listTable.vue";
export default {
name: "TestTable",
components: {
listTable,
},
data() {
return {
TestData: {
tableData: [
{
date: "2016-05-01",
name: "王小虎",
address: "上海市普陀区金沙江路 1519 弄",
province:"广东省",
city:"深圳市"
},
{
date: "2016-05-03",
name: "王小虎",
address: "上海市普陀区金沙江路 1516 弄",
province:"广东省",
city:"广州市"
},
{
date: "2016-05-02",
name: "王小虎",
address: "上海市普陀区金沙江路 1518 弄",
province:"广东省",
city:"佛山市"
},
{
date: "2016-05-04",
name: "王小虎",
address: "上海市普陀区金沙江路 1517 弄",
province:"广东省",
city:"惠州市"
},
{
date: "2016-05-01",
name: "王小虎",
address: "上海市普陀区金沙江路 1519 弄",
},
{
date: "2016-05-03",
name: "王小虎",
address: "上海市普陀区金沙江路 1516 弄",
},
{
date: "2016-05-02",
name: "王小虎",
address: "上海市普陀区金沙江路 1518 弄",
},
{
date: "2016-05-04",
name: "王小虎",
address: "上海市普陀区金沙江路 1517 弄",
},
{
date: "2016-05-01",
name: "王小虎",
address: "上海市普陀区金沙江路 1519 弄",
},
{
date: "2016-05-03",
name: "王小虎",
address: "上海市普陀区金沙江路 1516 弄",
},
],
tableCol: [
{
label: "姓名",
prop: "name",
showOverFlowTooltip: true,
},
{
label: "日期",
prop: "date",
showOverFlowTooltip: true,
},
{
label: "地址",
prop: "address",
align: "right",
showOverFlowTooltip: true,
},
],
},
};
},
methods: {
view(row){
console.log("view", row);
},
edit(row){
console.log("edit", row);
},
},
};
</script>
<style lang="scss" scoped>
.blue {
padding: 5px 8px;
background: #e82323;
margin-right: 20px;
}
</style>
选中某一个行
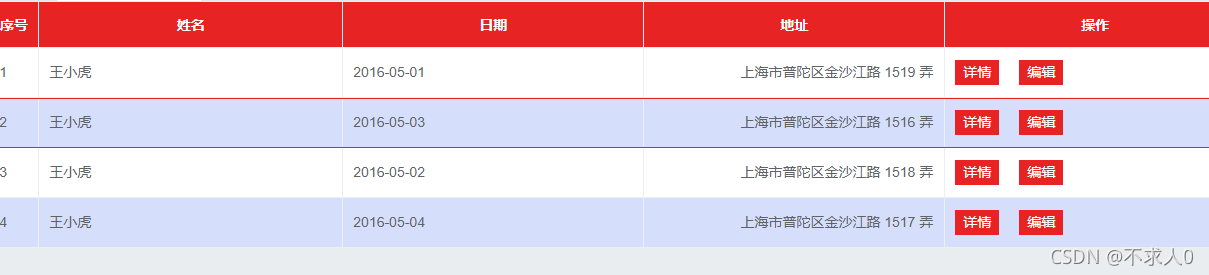
<template>
<div style="height: 100%">
<div class="tab_sec">
<list-table
:data="TestData"
isSerial
isOperation
operationText="操作"
isRowClick
@rowClick="rowClick"
>
<template v-slot:operation="scopeVal">
<button class="blue" @click="view(scopeVal.scope.row)">详情</button>
<button class="blue" @click="edit(scopeVal.scope.row)">编辑</button>
</template>
</list-table>
</div>
</div>
</template>
<script>
import listTable from "@/components/commom/conTable/listTable.vue";
export default {
name: "TestTable",
components: {
listTable,
},
data() {
return {
TestData: {
tableData: [
{
date: "2016-05-01",
name: "王小虎",
address: "上海市普陀区金沙江路 1519 弄",
},
{
date: "2016-05-03",
name: "王小虎",
address: "上海市普陀区金沙江路 1516 弄",
},
{
date: "2016-05-02",
name: "王小虎",
address: "上海市普陀区金沙江路 1518 弄",
},
{
date: "2016-05-04",
name: "王小虎",
address: "上海市普陀区金沙江路 1517 弄",
},
],
tableCol: [
{
label: "姓名",
prop: "name",
showOverFlowTooltip: true,
},
{
label: "日期",
prop: "date",
showOverFlowTooltip: true,
},
{
label: "地址",
prop: "address",
align: "right",
showOverFlowTooltip: true,
},
],
},
};
},
methods: {
view(row){
console.log("view", row);
},
edit(row){
console.log("edit", row);
},
rowClick( row ) {
console.log("rowClick", row);
}
},
};
</script>
<style lang="scss" scoped>
.blue {
padding: 5px 8px;
background: #e82323;
margin-right: 20px;
}
</style>