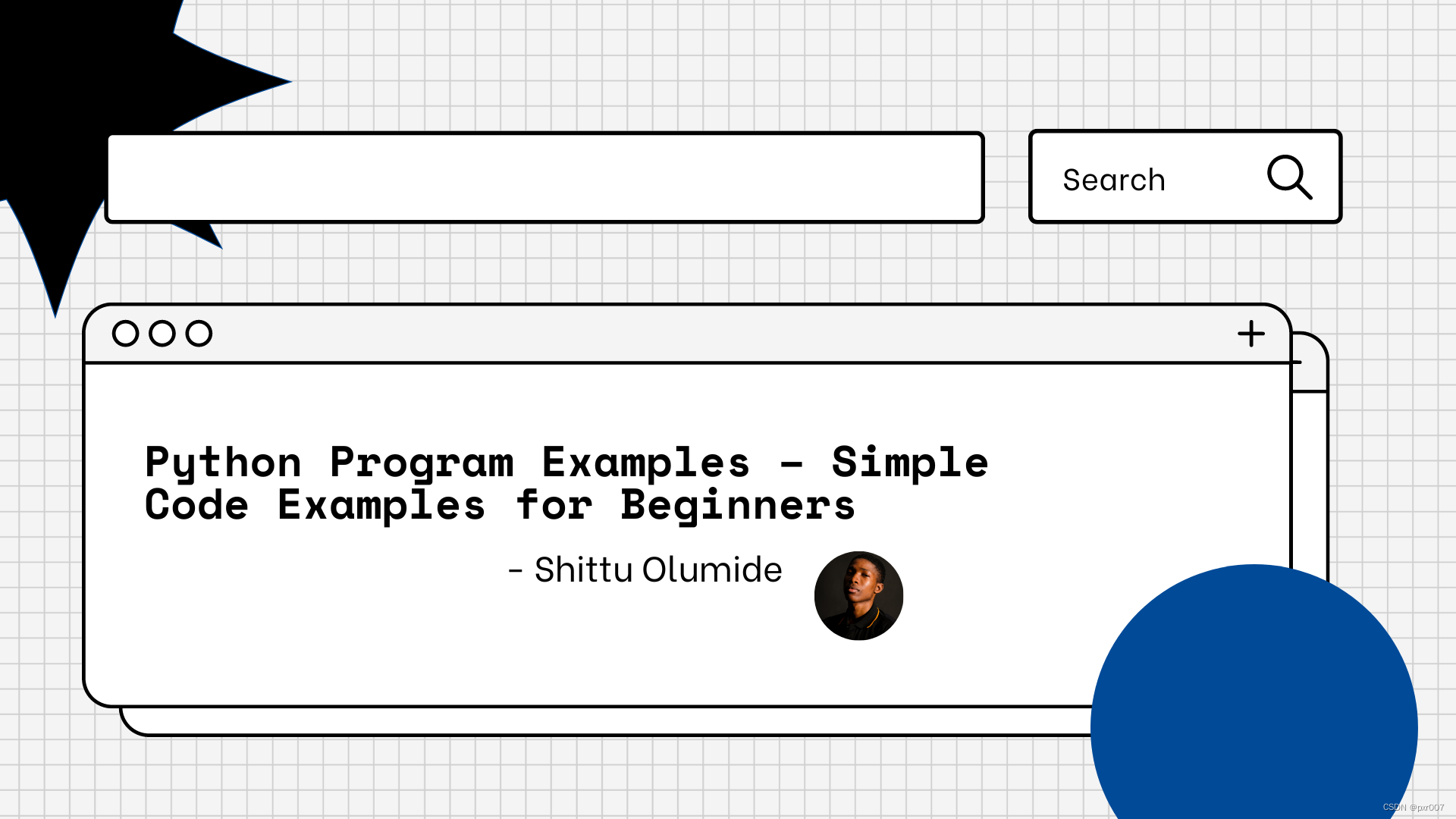
马克吐温说过,出人头地的秘诀就是开始。编程对于初学者来说似乎令人望而生畏,但最好的入门方式是直接投入并开始编写代码。
简单的代码示例是初学者入门和学习编程基础知识的好方法。在本文中,我将提供一系列非常适合 Python 初学者的简单代码示例。
这些示例涵盖了一系列编程概念,将帮助您打下坚实的编程基础。无论您是编程新手还是只是想温习一下自己的技能,修复笔记本电脑上未检测到电池错误 – Windows 11/10这些代码示例都将帮助您开始编码之旅。
如果您需要学习一些 Python 基础知识,我在本教程末尾添加了一些有用的资源。
如何用 Python 构建猜数游戏
在这个项目中,您将创建一个简单的猜数字游戏,让用户猜一个 1 到 100 之间的随机数。程序会在用户每次猜测后给提示,指出他们的猜测是太高还是太低,直到用户猜对了数字。
代码:
import random
secret_number = random.randint(1, 100)
while True:
guess = int(input("Guess the number between 1 and 100: "))
if guess == secret_number:
print("Congratulations! You guessed the number!")
break
elif guess < secret_number:
print("Too low! Try again.")
else:
print("Too high! Try again.")
解释:
首先导入random模块,这将允许您生成一个随机数。
randint()使用模块中的函数生成 1 到 100 之间的随机数random,并将其分配给变量。
创建一个循环,允许用户猜测数字,直到他们猜对为止。在循环内,提示用户使用该input()函数输入他们的猜测,并使用该函数将他们的输入转换为整数int()。
在循环内添加条件语句,检查用户的猜测是否正确、过高或过低。如果猜测正确,则打印祝贺信息并跳出循环。如果猜测的数字太高或太低,则打印提示消息以帮助用户正确猜出数字。
运行程序,玩猜数字游戏吧!
如何在 Python 中构建一个简单的密码生成器
顾名思义,密码生成器使用不同的字符组合和特殊字符生成特定长度的随机密码。
代码:
import random
import string
def generate_password(length):
"""This function generates a random password
of a given length using a combination of
uppercase letters, lowercase letters,
digits, and special characters"""
# Define a string containing all possible characters
all_chars = string.ascii_letters + string.digits + string.punctuation
# Generate a password using a random selection of characters
password = "".join(random.choice(all_chars) for i in range(length))
return password
# Test the function by generating a password of length 10
password = generate_password(10)
print(password)
解释:
我们分别导入用于生成随机值和处理字符串的random和模块。string
接下来,我们定义一个名为的函数,它generate_password接受一个参数length,指定需要生成的密码的长度。
在函数内部,我们定义了一个名为的字符串all_chars,其中包含可用于生成密码的所有可能字符。我们使用string.ascii_letters、string.digits和string.punctuation常量来创建这个字符串。
然后,我们使用列表推导式从使用该函数的字符串中生成length随机字符列表。最后,我们使用该函数将这些字符连接成一个字符串并返回结果。all_charsrandom.choice()"".join()
为了测试该函数,我们使用参数10调用它以生成长度为 10 的密码并打印结果。
请注意,这是一个非常简单的密码生成器,可能不适合在关注安全性的现实场景中使用。
如何在 Python 中构建密码检查器
我们将在本节中构建一个密码检查器。它的工作是根据我们设置的一些标准检查密码是否足够强。如果不满足任何密码条件,它将打印错误。
代码:
# Define a function to check if the password is strong enough
def password_checker(password):
# Define the criteria for a strong password
min_length = 8
has_uppercase = False
has_lowercase = False
has_digit = False
has_special_char = False
special_chars = "!@#$%^&*()-_=+[{]}\|;:',<.>/?"
# Check the length of the password
if len(password) < min_length:
print("Password is too short!")
return False
# Check if the password contains an uppercase letter, lowercase letter, digit, and special character
for char in password:
if char.isupper():
has_uppercase = True
elif char.islower():
has_lowercase = True
elif char.isdigit():
has_digit = True
elif char in special_chars:
has_special_char = True
# Print an error message for each missing criteria
if not has_uppercase:
print("Password must contain at least one uppercase letter!")
return False
if not has_lowercase:
print("Password must contain at least one lowercase letter!")
return False
if not has_digit:
print("Password must contain at least one digit!")
return False
if not has_special_char:
print("Password must contain at least one special character!")
return False
# If all criteria are met, print a success message
print("Password is strong!")
return True
# Prompt the user to enter a password and check if it meets the criteria
password = input("Enter a password: ")
password_checker(password)
解释:
在此代码中,我们定义了一个名为的函数password_checker(),该函数将密码作为参数并检查它是否满足特定的强度标准。
我们首先定义强密码的标准——最少 8 个字符的长度,至少一个大写字母、一个小写字母、一个数字和一个特殊字符。
然后,我们使用遍历密码中每个字符的 for 循环检查密码的长度以及它是否包含所需类型的字符。
如果密码不符合任何条件,我们将打印一条错误消息并返回False以指示密码不够强。否则,我们打印一条成功消息并返回True。
最后,我们提示用户使用该函数输入密码input(),并将其传递给该password_checker()函数以检查其是否符合条件。
如何在 Python 中构建网络爬虫
网络抓取工具从网页上抓取/获取数据并将其保存为我们想要的任何格式,.csv 或 .txt。在本节中,我们将使用名为 Beautiful Soup 的 Python 库构建一个简单的网络抓取工具。
代码:
import requests
from bs4 import BeautifulSoup
# Set the URL of the webpage you want to scrape
url = 'https://www.example.com'
# Send an HTTP request to the URL and retrieve the HTML content
response = requests.get(url)
# Create a BeautifulSoup object that parses the HTML content
soup = BeautifulSoup(response.content, 'html.parser')
# Find all the links on the webpage
links = soup.find_all('a')
# Print the text and href attribute of each link
for link in links:
print(link.get('href'), link.text)
解释:
在这段代码中,我们首先导入分别用于发出 HTTP 请求和解析 HTML 内容的requests和模块。BeautifulSoup
接下来,我们在名为 的变量中设置要抓取的网页的 URL url。
然后,我们使用该requests.get()函数向 URL 发送 HTTP GET 请求,并检索网页的 HTML 内容作为响应。
我们创建一个BeautifulSoup名为的对象soup,它使用解析器解析响应的 HTML 内容html.parser。
然后我们使用该soup.find_all()方法找到网页上的所有链接并将它们存储在一个名为 的变量中links。
最后,我们使用 for 循环遍历每个链接,links并使用该方法打印每个链接的 text 和 href 属性link.get()。
如何在 Python 中构建货币转换器
货币转换器是帮助用户将一种货币的价值转换为另一种货币的程序。您可以将其用于多种用途,例如计算国际采购成本、估算差旅费用或分析财务数据。
注意:我们将使用 ExchangeRate-API 获取汇率数据,这是一个免费开源的货币汇率 API。但是还有其他可用的 API,它们可能具有不同的使用限制或要求。
代码:
# Import the necessary modules
import requests
# Define a function to convert currencies
def currency_converter(amount, from_currency, to_currency):
# Set the API endpoint for currency conversion
api_endpoint = f"https://api.exchangerate-api.com/v4/latest/{from_currency}"
# Send a GET request to the API endpoint
response = requests.get(api_endpoint)
# Get the JSON data from the response
data = response.json()
# Extract the exchange rate for the target currency
exchange_rate = data["rates"][to_currency]
# Calculate the converted amount
converted_amount = amount * exchange_rate
# Return the converted amount
return converted_amount
# Prompt the user to enter the amount, source currency, and target currency
amount = float(input("Enter the amount: "))
from_currency = input("Enter the source currency code: ").upper()
to_currency = input("Enter the target currency code: ").upper()
# Convert the currency and print the result
result = currency_converter(amount, from_currency, to_currency)
print(f"{amount} {from_currency} is equal to {result} {to_currency}")
解释:
在此代码中,我们定义了一个名为的函数,currency_converter()该函数将金额、源货币代码和目标货币代码作为参数并返回转换后的金额。
from_currency我们首先使用参数和模块设置用于货币转换的 API 端点requests,以向端点发送 GET 请求。
然后,我们使用参数从 API 返回的 JSON 数据中提取目标货币的汇率to_currency,并通过将汇率乘以amount参数来计算转换后的金额。
最后,我们提示用户输入amount、from_currency和to_currencyusinginput()函数并将它们传递给currency_converter()函数以转换货币。然后使用字符串格式打印转换后的金额。
结论
所有这些项目都非常简单且易于构建。如果你真的想提高你的 Python 技能,我建议你获取代码,修改和编辑它,并在它的基础上构建。如果需要,您可以将这些简单项目中的许多项目变成复杂得多的应用程序。