DOM(document object model)文档对象模型
一、获取DOM元素对象
1.document.documentElement
2.document.head
3.document.title
4.document.body
二、获取元素对象的方法
document.getElementById()//通过id找到对象
document.getElementsByTagName()//通过标签名<>找到对象
document.getElementsByClassName()//通过类名class找到对象
document.getElementsByName()//通过名字name的值找到对象(极少使用,表单会用)
<html>
<head>
<meta charset="UTF-8" />
<title>Document</title>
</head>
<body>
<h1 id="hid">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
<h1 class="hcls">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
<h1 class="hcls">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
<h1 class="hcls">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
<h1 class="hcls">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
<h1 class="hcls">aaaaaaaaaaaaaaaaaaaaaaaaa</h1>
</body>
<script>
hobj=document.getElementById('hid');
hobjs=document.getElementsByClassName('hcls');
hobj.style.background='#f0f';
for(i=0;i<hobjs.length;i++){
hobjs[i].style.background='#afb';
}
</script>
</html>
三、元素对象标准属性
obj.id;
obj.className;
obj.style;
obj.title;
<form action="" method=""></form>
//其中action method为标准属性
四、元素对象非标准属性
非标准属性不能用.获取和修改
<form action="" method="" age='5' sex='female' index=4></form>
//其中age sex index是非标准属性
1.obj.getAttribute('age');//获取非标准属性
2.obj.setAttribute('age','50');//设置非标准属性
<body>
<h1 id="hid" title="javascript" class="hcls" info="123">javascript is useful!</h1>
</body>
<script>
hobj=document.getElementById('hid');
alert(hobj.getAttribute('info'));
</script>
实例:把getAttribute()和setAttribute()写成函数来使用
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>index</title>
<style>
*{
font-family: 微软雅黑;
}
</style>
</head>
<body>
<h1 info='linux is very much!' id='hid' class='hcls'>linux is very much!</h1>
</body>
<script>
hidobj=document.getElementById('hid');
function getA(obj,attr){
return obj.getAttribute(attr);
}
alert(getA(hidobj,'class'));
function setA(obj,attr,value){
obj.setAttribute(attr,value);
}
setA(hidobj,'info','php is very much!');
</script>
</html>
五、元素对象JS属性
obj.tagName;//获取标签名
obj.innerHTML;//获取标签内部内容
obj.outerHTML;//获取标签的全部内容
obj.textContent;//获取标签内部的文本内容,过滤掉标签元素
<body>
<h1 id='hid'>linux <i>is</i> very much!</h1>
</body>
<script>
hobj=document.getElementById('hid');
document.write(hobj.tagName);//H1
document.write(hobj.innerHTML);//linux <i>is</i> very much!
document.write(hobj.outerHTML);//<h1 id='hid'>linux <i>is</i> very much!</h1>
document.write(hobj.textContent);//linux is very much!
</script>
六、直接获取DOM元素对象集合
1.document.links;
<body>
<p><a href="http://www.baidu.com">百度</a></p>
<p><a href="http://www.163.com">网易</a></p>
<p><a href="http://www.qq.com">腾讯</a></p>
</body>
<script>
objs=document.links;
alert(objs[0].outerHTML);//<a href="http://www.baidu.com">百度</a>
</script>
2.document.images;收集img标签
<body>
<p><img src="bk.png" width="100px" /></p>
<p><img src="dog.png" width="100px" /></p>
</body>
<script>
imgobjs=document.images;
for(i=0;i<imgobjs.length;i++){
imgobjs[i].style.background='#afb';//图片背景色改为绿色
}
</script>
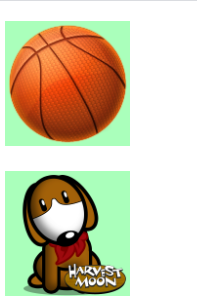
3.document.forms;
<body>
<form action="go1.php">
<p>username:</p>
<p><input type="text" /></p>
</form>
<hr />
<form action="go2.php">
<p>username:</p>
<p><input type="text" /></p>
</form>
<button id="btn1">表单1</button>
<button id="btn2">表单2</button>
</body>
<script>
btn1=document.getElementById('btn1');
btn2=document.getElementById('btn2');
objs=document.forms;
btn1.onclick=function(){
objs[0].submit();//单击button1提交表单1
}
btn2.onclick=function(){
objs[1].submit();//单击button2提交表单2
}
</script>
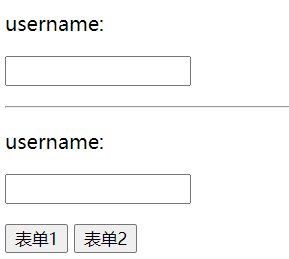
4.document.anchors;锚点
<body>
<p><a href="http://www.baidu.com" name="baidu">百度</a></p>
<p><a href="http://www.163.com" name="163">网易</a></p>
<p><a href="http://www.qq.com" name="qq">腾讯</a></p>
</body>
<script>
objs=document.anchors;
for(i=0;i<objs.length;i++){
objs[i].style.textDecoration='none';//超链接下划线被去掉
}
</script>
5.tableObj.rows;
6.tableRowObj.cells;
<body>
一个表格
</body>
<script>
tobj=document.getElementById('tid');
function set(i,j){
tobj.rows[i-1].cells[j-1].innerHTML='abc';
}
set(2,3);//将第二行第三列的内容改为abc
</script>

7.selectObj.options;
8.selectObj.selectedIndex获取下拉菜单中第几个option选项被选中.
<body>
<p>选择城市:</p>
<p>
<select id="sid">
<option value="北京">北京</option>
<option value="上海">上海</option>
<option value="广州">广州</option>
</select>
</p>
<p><input type="button" value="OK" id="btid" /></p>
</body>
<script>
btobj=document.getElementById('btid');
sobj=document.getElementById('sid');
btobj.onclick=function(){
idx=sobj.selectedIndex;//获取选中下拉菜单的元素排第几:北京-0;上海-1;广州-2
opts=sidobj.options;
alert(opts[idx].value);//选中某个城市OK后弹出该城市的值
}
</script>