目录
3.6定义Vue的template(在WebStorm中实现)
7.2三种方案对比——(ES没有闭包、ES5有闭包、ES6let)
1、Vuejs的认识和特点介绍
2、Vuejs安装方式
推荐使用第二种方法:右键——>链接另存为
3、Vue体验
3.1HelloVuejs初体验
体验数据(Vue实例)和界面(元素)完全分离,体验Vue的响应式(当数据发生改变的时候,界面会自动的发生一些响应)
编程范式:声明式编程
编程范式:命令式编程
3.2Vue列表展示
3.3计数器案例
3.4Vue的mvvm
3.5Vue的options选项
3.6定义Vue的template(在WebStorm中实现)
快速根据缩写生成模板文本
4、插值操作——mustache(双大括号)
4.1mustache语法
4.2插值操作其他指令使用
4.2.1v-once指令
4.2.2v-html指令
4.2.3v-cloak指令
4.2.4v-pre指令
5、v-bind学习
5.1v-bind基本使用
5.2v-bind动态绑定class(对象语法)
5.3v-bind动态绑定class(数组语法)
5.4作业(v-for结合v-bind)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<style>
.sjf{
color: deeppink;
}
</style>
<body>
<!--作业要求:点击列表中的哪一项,该项变成红色-->
<div id="app">
<ul>
<!-- <li v-for="item in childrens">{{item}}</li>-->
<!-- <li v-bind:class="sjf" v-for="(item,index) in childrens">{{index}}-{{item}}</li>-->
<li v-for="(item,index) in childrens" :class="{sjf:currentIndex===index}" @click="liclink(index)">{{index}}-{{item}}</li>
<!-- <li :class="{sjf:0===currentIndex}">{{index}}-{{item}}</li>-->
<!-- <li :class="{sjf:0===currentIndex}">{{index}}-{{item}}</li>-->
<!-- <li :class="{sjf:0===currentIndex}">{{index}}-{{item}}</li>-->
<!-- <li :class="{sjf:0===currentIndex}">{{index}}-{{item}}</li>-->
</ul>
</div>
<script src="../js/vue.js"></script>
<script>
const app=new Vue({
el:'#app',
data: {
// sjf:'sjf',
childrens:['大炮','二丫','毛毛','呆瓜' ],
currentIndex:0
},
methods:{
liclink(index){
this.currentIndex=index
}
}
})
</script>
</body>
</html>
5.5v-bind动态绑定style
5.5.1对象语法
5.5.2数组语法
6.计算属性
6.1计算属性的基本使用
6.2计算属性的复杂使用
6.3计算属性的setter和getter(完整写法)
6.4计算属性和methods的比较
7、ES6相关知识
7.1块级作用域——let和var
7.2三种方案对比——(ES没有闭包、ES5有闭包、ES6let)
7.3const的使用
7.4ES6对象字面量的增强写法
8、事件监听
8.1v-on的基本使用和语法糖
8.2v-on的参数传递问题
8.3v-on的修饰符
9、条件判断
9.1三个条件
9.2登录切换案例
登录切换案例:
案例出现的小问题:
9.3v-if和v-show对比
10、循环遍历
10.1遍历数组
10.2遍历对象
10.3v-for使用过程添加key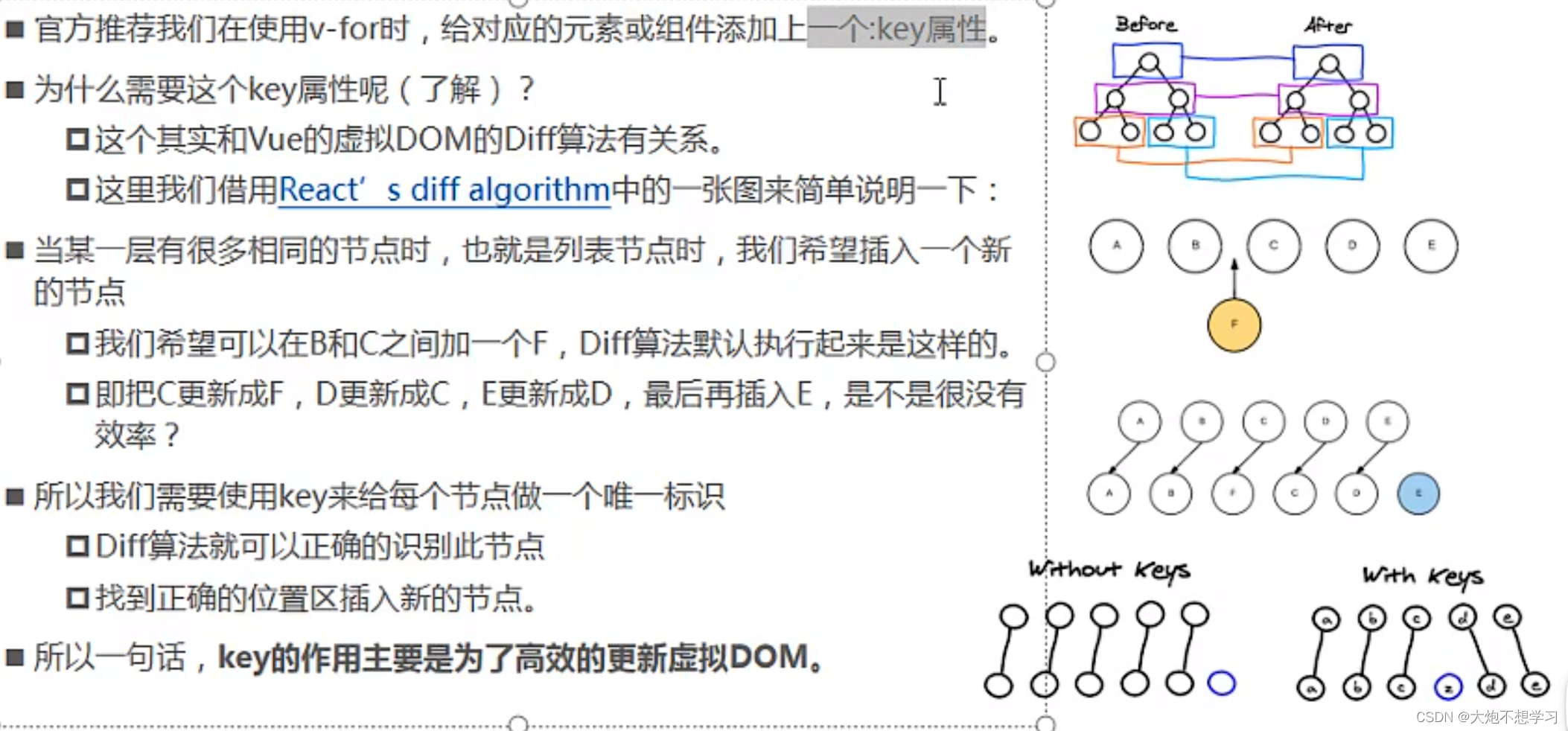
v-for:数组渲染之后,在中间插入元素的渲染过程
10.4数组中哪些方法是响应式的
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<ul>
<li v-for="items in children" >{{items}}</li>
</ul>
<button @click="btnClick">按钮</button>
</div>
<script src="../js/vue.js " ></script>
<script>
const app=new Vue({
el:'#app',
data:{
children:['大炮','二丫','毛毛']
},
methods:{
btnClick(){
// 1 push方法
// this.children.push('124')
// this.children.push('dsa','32542')
// 2 pop()方法:删除数组中的最后一个元素
// this.children.pop()
// 3 shift():删除数组中第一个元素
// this.children.shift()
//4 unshift():再数组最前面添加元素
// this.children.unshift('ashdjn ')
// this.children.unshift('ashdjn','sdsjfhjd',8234783)
// 5 splice作用:删除元素/插入元素/替换元素
// 删除元素:第二个参数传入你要删除几个元素(如果没有传,就删除后面所有的元素)
// this.children.splice(1)
// this.children.splice(1,1)
// 插入元素:第二个参数传入0,并且后面跟上要插入的元素
// this.children.splice(2,0,'4','fch','sjf')
// 替换元素:第二个参数表示我们要替换几个元素,后面是用于替换前面的元素
// this.children.splice(1,2,'fch','sjf')
// 6 sort()
// this.children.sort()
// 7 reverse()
// this.children.reverse()
// 上边的都是响应式的
// 注意:通过索引值修改数组的元素不是响应式的
// this.children[0]='fch'
Vue.set(要修改的对象,索引值,'修改后的值')
Vue.set(this.children,0,'sjf')
}
}
})
</script>
</body>
</html>
11、购物车案例
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <link rel="stylesheet" href="style.css"> </head> <body> <div id="app"> <div v-if="books.length"> <table> <thead> <tr> <th></th> <th>书籍名称</th> <th>出版日期</th> <th>价格</th> <th>购买数量</th> <th>操作</th> </tr> </thead> <tbody> <tr v-for="(item,index) in books"> <td>{{item.id}}</td> <td>{{item.name}}</td> <td>{{item.date}}</td> <!-- <td>{{getFinalPrice(item.price)}}</td>--> <!-- <td>{{item.price|过滤器}}</td>--> <td>{{item.price|showPrice}}</td> <td> <button @click="decrease(index)" v-bind:disabled="item.count<=1">-</button> {{item.count}} <button @click="increase(index)">+</button> </td> <td><button @click="remove">移除</button></td> </tr> </tbody> </table> <h2>总价格:{{totalPrice}}</h2> </div> <h2 v-else>购物车为空</h2> </div> <script src="../js/vue.js"></script> <script src="main.js"></script> </body> </html>
main.js
const app=new Vue({ el:'#app', data:{ books:[ { id:1, name:'《算法导论》', date:'2006-9', price:85.00, count:1 }, { id:1, name:'《算法导论》', date:'2006-9', price:85.00, count:1 }, { id:1, name:'《算法导论》', date:'2006-9', price:85.00, count:1 }, { id:1, name:'《算法导论》', date:'2006-9', price:85.00, count:1 } ] }, methods:{ // getFinalPrice(price){ // return '$'+price.toFixed(2) // } decrease(index){ this.books[index].count-- }, increase(index){ this.books[index].count++ }, remove(index){ this.books.splice(index,1) } }, filters:{ showPrice(price){ return '$'+price.toFixed(2) } }, computed:{ totalPrice(){ let totalPrice=0 for(let i=0;i<this.books.length;i++){ totalPrice+=this.books[i].price*this.books[i].count } return totalPrice } } })
style.css
table { border:1px solid pink; border-collapse: collapse; border-spacing: 0; } th,td { padding: 8px 16px; border:1px solid cadetblue; text-align: left; } th{ background-color: gold; color: aquamarine; font-weight: 100; font-size: 14px; }