系列文章目录
前言
记录一些工作中常用的方法,方便以后开发的查阅
一、函数
1. 通用函数 util/xxx.js
/**
* 判断是否为空
*/
export function validatenull(val) {
if (typeof val == 'boolean') {
return false;
}
if (typeof val == 'number') {
return false;
}
if (val instanceof Array) {
if (val.length == 0) return true;
} else if (val instanceof Object) {
if (JSON.stringify(val) === '{}') return true;
} else {
if (val == 'null' || val == null || val == 'undefined' || val == undefined || val == '') return true;
return false;
}
return false;
}
/**
* 对象深拷贝
*/
export const deepClone = data => {
var type = getObjType(data);
var obj;
if (type === 'array') {
obj = [];
} else if (type === 'object') {
obj = {};
} else {
//不再具有下一层次
return data;
}
if (type === 'array') {
for (var i = 0, len = data.length; i < len; i++) {
obj.push(deepClone(data[i]));
}
} else if (type === 'object') {
for (var key in data) {
obj[key] = deepClone(data[key]);
}
}
return obj;
};
/**
* 加密处理
*/
export const encryption = (params) => {
let {
data,
type,
param,
key
} = params;
let result = JSON.parse(JSON.stringify(data));
if (type == 'Base64') {
param.forEach(ele => {
result[ele] = btoa(result[ele]);
})
} else if (type == 'Aes') {
param.forEach(ele => {
result[ele] = window.CryptoJS.AES.encrypt(result[ele], key).toString();
})
}
return result;
};
/**
* 浏览器判断是否全屏
*/
export const fullscreenToggel = () => {
if (fullscreenEnable()) {
exitFullScreen();
} else {
reqFullScreen();
}
};
/**
* esc监听全屏
*/
export const listenfullscreen = (callback) => {
function listen() {
callback()
}
document.addEventListener("fullscreenchange", function () {
listen();
});
document.addEventListener("mozfullscreenchange", function () {
listen();
});
document.addEventListener("webkitfullscreenchange", function () {
listen();
});
document.addEventListener("msfullscreenchange", function () {
listen();
});
};
/**
* 浏览器判断是否全屏
*/
export const fullscreenEnable = () => {
var isFullscreen = document.isFullScreen || document.mozIsFullScreen || document.webkitIsFullScreen
return isFullscreen;
}
/**
* 浏览器全屏
*/
export const reqFullScreen = () => {
if (document.documentElement.requestFullScreen) {
document.documentElement.requestFullScreen();
} else if (document.documentElement.webkitRequestFullScreen) {
document.documentElement.webkitRequestFullScreen();
} else if (document.documentElement.mozRequestFullScreen) {
document.documentElement.mozRequestFullScreen();
}
};
/**
* 浏览器退出全屏
*/
export const exitFullScreen = () => {
if (document.documentElement.requestFullScreen) {
document.exitFullScreen();
} else if (document.documentElement.webkitRequestFullScreen) {
document.webkitCancelFullScreen();
} else if (document.documentElement.mozRequestFullScreen) {
document.mozCancelFullScreen();
}
};
/**
* 递归寻找子类的父类
*/
export const findParent = (menu, id) => {
for (let i = 0; i < menu.length; i++) {
if (menu[i].children.length != 0) {
for (let j = 0; j < menu[i].children.length; j++) {
if (menu[i].children[j].id == id) {
return menu[i];
} else {
if (menu[i].children[j].children.length != 0) {
return findParent(menu[i].children[j].children, id);
}
}
}
}
}
};
/**
* 判断2个对象属性和值是否相等
*/
/**
* 动态插入css
*/
export const loadStyle = url => {
const link = document.createElement('link');
link.type = 'text/css';
link.rel = 'stylesheet';
link.href = url;
const head = document.getElementsByTagName('head')[0];
head.appendChild(link);
};
/**
* 判断路由是否相等
*/
export const diff = (obj1, obj2) => {
delete obj1.close;
var o1 = obj1 instanceof Object;
var o2 = obj2 instanceof Object;
if (!o1 || !o2) { /* 判断不是对象 */
return obj1 === obj2;
}
if (Object.keys(obj1).length !== Object.keys(obj2).length) {
return false;
//Object.keys() 返回一个由对象的自身可枚举属性(key值)组成的数组,例如:数组返回下表:let arr = ["a", "b", "c"];console.log(Object.keys(arr))->0,1,2;
}
for (var attr in obj1) {
var t1 = obj1[attr] instanceof Object;
var t2 = obj2[attr] instanceof Object;
if (t1 && t2) {
return diff(obj1[attr], obj2[attr]);
} else if (obj1[attr] !== obj2[attr]) {
return false;
}
}
return true;
}
/**
* 根据字典的value显示label
*/
export const findByvalue = (dic, value) => {
let result = '';
if (validatenull(dic)) return value;
if (typeof (value) == 'string' || typeof (value) == 'number' || typeof (value) == 'boolean') {
let index = 0;
index = findArray(dic, value);
if (index != -1) {
result = dic[index].label;
} else {
result = value;
}
} else if (value instanceof Array) {
result = [];
let index = 0;
value.forEach(ele => {
index = findArray(dic, ele);
if (index != -1) {
result.push(dic[index].label);
} else {
result.push(value);
}
});
result = result.toString();
}
return result;
};
/**
* 根据字典的value查找对应的index
*/
export const findArray = (dic, value) => {
for (let i = 0; i < dic.length; i++) {
if (dic[i].value == value) {
return i;
}
}
return -1;
};
/**
* 生成随机len位数字
*/
export const randomLenNum = (len, date) => {
let random = '';
random = Math.ceil(Math.random() * 100000000000000).toString().substr(0, len ? len : 4);
if (date) random = random + Date.now();
return random;
};
/**
* 打开小窗口
*/
export const openWindow = (url, title, w, h) => {
// Fixes dual-screen position Most browsers Firefox
const dualScreenLeft = window.screenLeft !== undefined ? window.screenLeft : screen.left
const dualScreenTop = window.screenTop !== undefined ? window.screenTop : screen.top
const width = window.innerWidth ? window.innerWidth : document.documentElement.clientWidth ? document.documentElement.clientWidth : screen.width
const height = window.innerHeight ? window.innerHeight : document.documentElement.clientHeight ? document.documentElement.clientHeight : screen.height
const left = ((width / 2) - (w / 2)) + dualScreenLeft
const top = ((height / 2) - (h / 2)) + dualScreenTop
const newWindow = window.open(url, title, 'toolbar=no, location=no, directories=no, status=no, menubar=no, scrollbars=no, resizable=yes, copyhistory=no, width=' + w + ', height=' + h + ', top=' + top + ', left=' + left)
// Puts focus on the newWindow
if (window.focus) {
newWindow.focus()
}
}
/**
* 获取顶部地址栏地址
*/
export const getTopUrl = () => {
return window.location.href.split("/#/")[0];
}
/**
* 获取url参数
* @param name 参数名
*/
export const getQueryString = (name) => {
let reg = new RegExp("(^|&)" + name + "=([^&]*)(&|$)", "i");
let r = window.location.search.substr(1).match(reg);
if (r != null) return unescape(decodeURI(r[2]));
return null;
}
/**
* 替换url参数
* @param url www.baidu.com?sex=男
* @param arg 需要替换的参数名 eg: sex
* @param val 参数值替换成 eg: 女
*/
changeUrlArg(url, arg, val) {
var pattern = arg + '=([^&]*)';
var replaceText = arg + '=' + val;
return url.match(pattern) ? url.replace(eval('/(' + arg + '=)([^&]*)/gi'), replaceText) : (url.match('[\?]') ? url + '&' + replaceText : url + '?' + replaceText);
},
使用方式
import {
validatenull
} from '@/util/xxx';
2. util/func.js
export default class func {
/**
* 不为空
* @param val
* @returns {boolean}
*/
static notEmpty(val) {
return !this.isEmpty(val);
}
/**
* 是否为定义
* @param val
* @returns {boolean}
*/
static isUndefined(val) {
return val === null || typeof val === 'undefined';
}
/**
* 为空
* @param val
* @returns {boolean}
*/
static isEmpty(val) {
if (
val === null ||
typeof val === 'undefined' ||
(typeof val === 'string' && val === '' && val !== 'undefined')
) {
return true;
}
return false;
}
}
使用方式
import func from "@/util/func";
3. util/store.js
import {
validatenull
} from '@/util/validate';
const keyName = '自定义前缀' + '-';
/**
* 存储localStorage
*/
export const setStore = (params = {}) => {
let {
name,
content,
type,
} = params;
name = keyName + name
let obj = {
dataType: typeof (content),
content: content,
type: type,
datetime: new Date().getTime()
}
if (type) window.sessionStorage.setItem(name, JSON.stringify(obj));
else window.localStorage.setItem(name, JSON.stringify(obj));
}
/**
* 获取localStorage
*/
export const getStore = (params = {}) => {
let {
name,
debug
} = params;
name = keyName + name
let obj = {},
content;
obj = window.sessionStorage.getItem(name);
if (validatenull(obj)) obj = window.localStorage.getItem(name);
if (validatenull(obj)) return;
try {
obj = JSON.parse(obj);
} catch{
return obj;
}
if (debug) {
return obj;
}
if (obj.dataType == 'string') {
content = obj.content;
} else if (obj.dataType == 'number') {
content = Number(obj.content);
} else if (obj.dataType == 'boolean') {
content = eval(obj.content);
} else if (obj.dataType == 'object') {
content = obj.content;
}
return content;
}
/**
* 删除localStorage
*/
export const removeStore = (params = {}) => {
let {
name,
type
} = params;
name = keyName + name
if (type) {
window.sessionStorage.removeItem(name);
} else {
window.localStorage.removeItem(name);
}
}
/**
* 获取全部localStorage
*/
export const getAllStore = (params = {}) => {
let list = [];
let {
type
} = params;
if (type) {
for (let i = 0; i <= window.sessionStorage.length; i++) {
list.push({
name: window.sessionStorage.key(i),
content: getStore({
name: window.sessionStorage.key(i),
type: 'session'
})
})
}
} else {
for (let i = 0; i <= window.localStorage.length; i++) {
list.push({
name: window.localStorage.key(i),
content: getStore({
name: window.localStorage.key(i),
})
})
}
}
return list;
}
/**
* 清空全部localStorage
*/
export const clearStore = (params = {}) => {
let { type } = params;
if (type) {
window.sessionStorage.clear();
} else {
window.localStorage.clear()
}
}
使用方式
import {getStore, setStore} from '@/util/store'
4. util/bus.js
import Vue from 'vue'
const bus = new Vue()
export default bus
import $bus from '@/util/bus'
5. tree数据操作相关
// 样例数据
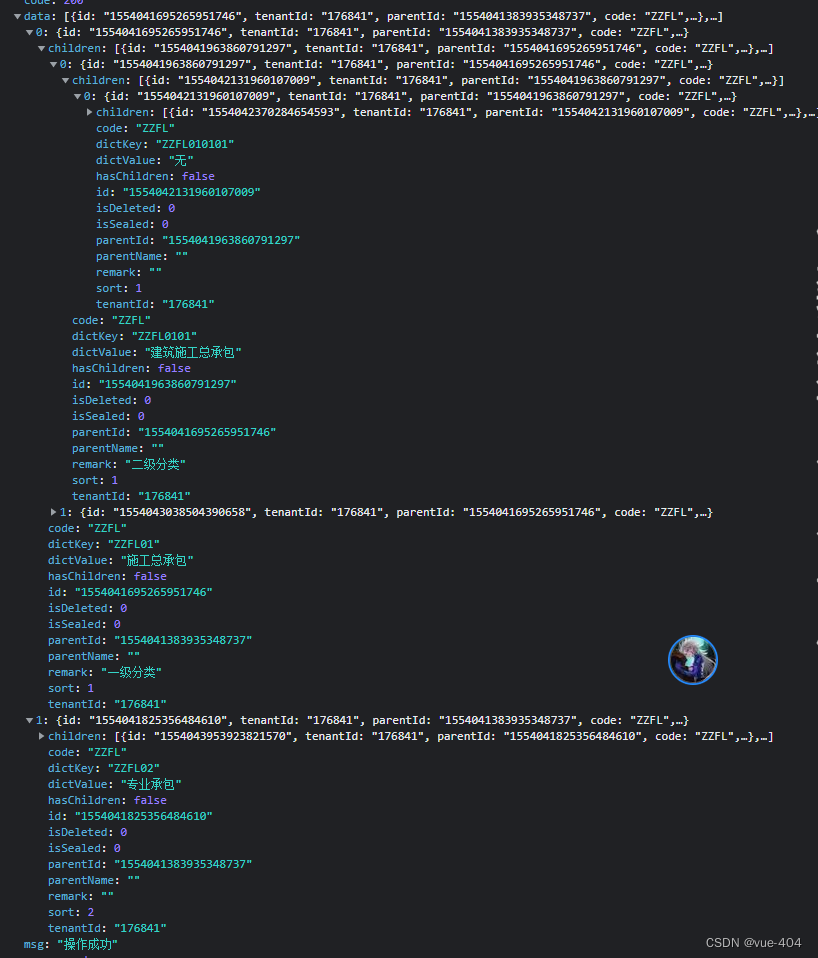
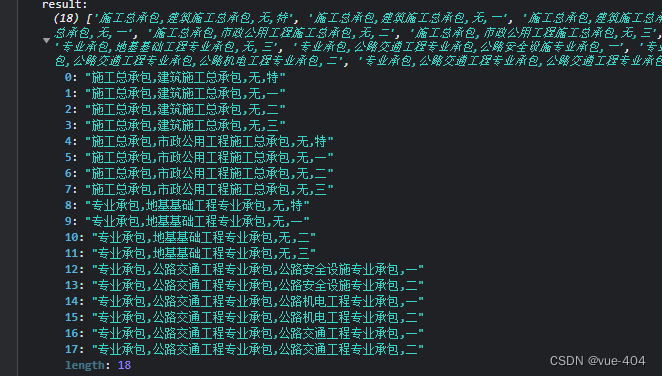
// 递归遍历树型数据,获取所有子节点的全路径,
getChildAllData(data) {
const result = [];
function getChildren(data, str = "") {
if (data.length == 0) return;
data.forEach((item) => {
let parentName = "";
if (str) {
parentName = str + "," + item.dictValue;
} else {
parentName = item.dictValue;
}
if (item.children) {
getChildren(item.children, parentName);
} else {
result.push(parentName);
}
});
}
getChildren(data);
return result;
},