import { Toast } from "vant"
import type { ToastWrapperInstance } from "vant/es/toast/types"
import axios, { AxiosError } from "axios"
import type { AxiosInstance } from "axios"
import { localCache } from "@/utils"
import type { IResponseData, ZJRequestConfig } from "@/services/types"
class ZJRequest {
request: AxiosInstance
count: number
loadingToast?: ToastWrapperInstance
constructor(config?: ZJRequestConfig) {
this.count = 0
this.request = axios.create(config)
// 添加请求拦截器
this.request.interceptors.request.use(
async (interceptorsConfig: ZJRequestConfig) => {
this.count += 1
this.loadingToast = Toast.loading({
duration: 0,
message: "加载中...",
forbidClick: true,
})
const token = localCache.getCache("token")
if (token) {
if (interceptorsConfig.headers) {
interceptorsConfig.headers["Authorization"] = token
}
}
return interceptorsConfig
},
(error) => {
this.count -= 1
return Promise.resolve(error)
}
)
// 添加响应拦截器
this.request.interceptors.response.use(
(response) => {
this.count -= 1
if (this.count <= 0) {
this.loadingToast?.clear()
this.count = 0 // 防止过快地请求导致一些不必要的错误
}
const res: IResponseData = response.data
// 判断状态
if (res.code !== 0) {
// const errorMsg = "操作失败:" + response.data.msg
if (res.code === 20001) {
// token不合法,清除token
localCache.removeCache("token")
} else {
// 普通错误消息
Toast.fail(res.msg)
}
}
return response.data
},
(error: AxiosError) => {
this.count -= 1
if (this.count <= 0) {
this.loadingToast?.clear()
this.count = 0
}
const err: IResponseData = {
code: -1,
msg: error.message,
data: error,
}
Toast.fail("请求失败" + error.message)
return Promise.resolve(err)
}
)
}
get<D>(config: ZJRequestConfig) {
const { url = "", ...realConfig } = config
return this.request.get<any, D>(url, realConfig)
}
post<D>(config: ZJRequestConfig) {
const { url = "", data = {}, ...realConfig } = config
return this.request.post<any, D>(url, data, realConfig)
}
put<D>(config: ZJRequestConfig) {
const { url = "", data = {}, ...realConfig } = config
return this.request.put<any, D>(url, data, realConfig)
}
delete<D>(config: ZJRequestConfig) {
const { url = "", ...otherConfig } = config
return this.request.delete<any, D>(url, otherConfig)
}
}
export default ZJRequest
import ZJRequest from "@/services/request"
const zjRequest = new ZJRequest({
baseURL: "/api/client",
timeout: 100000,
})
export default zjRequest
import zjRequest from "@/services"
// 注册
export const register = (data: any) => {
return zjRequest.post<API.IResult_Empty>({
url: "/account/register",
data,
})
}
// 登录
export const login = (data: any) => {
return zjRequest.post<API.IResult_Login>({
url: "/account/login",
data,
})
}
// 退出登录
export const logout = () => {
return zjRequest.post<API.IResult_Empty>({
url: "/account/logout",
})
}
// 修改个人信息
export const updateAccountInfo = (data: any) => {
return zjRequest.post<API.IResult_Empty>({
url: "/account/update",
data,
})
}
API是一个namespace,大致结构长这样:
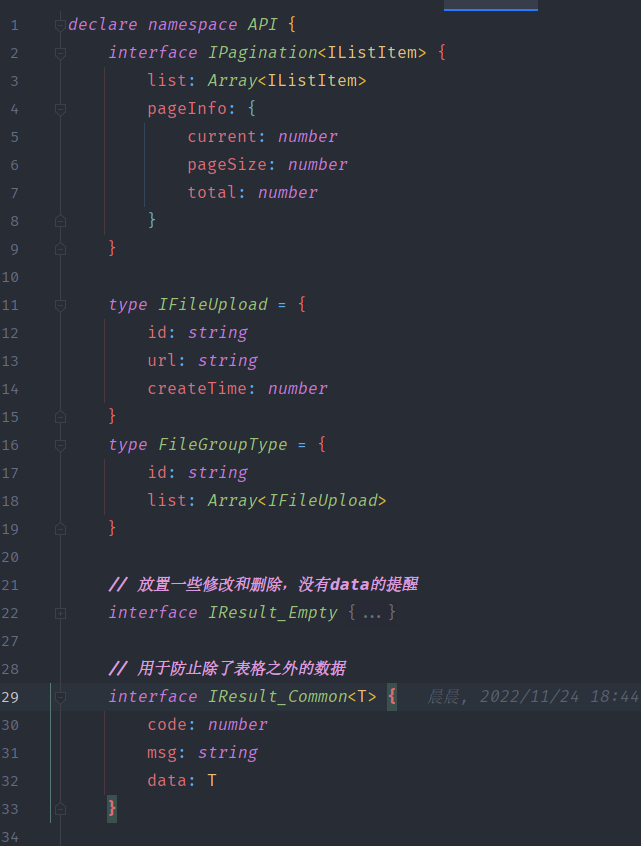