一:range函数:
1:range(stop)创建[0,stop)整数序列
2:range(start,stop)创建[start,stop)整数序列
3:range(start,stop,step)创建步长为step[start,stop)整数序列
二:列表:
容器(0---n-1)/(-n--- -1)
1:创建
(1):使用[]
(2):使用list()
2:获取单个元素
lst = ['hello','world',98,'hello']
print(lst.index('hello'))# 0
#如果不存在 ValueError
#print(lst.index('hello',1,4)) #ValueError
print(lst.index('hello',1,4)) #3
print(lst[2])#98
print(lst[10])#IndexError
3:获取多个元素
列表名[start:stop:step] 范围:[start:stop)
在末尾添加一个元素lst.append()
在末尾添加至少一个元素lst.extend()
在列表的任意位置添加一个元素 lst.insert(位置,元素)
在列表的任意位置添加至少一个元素:切片:
4:列表的判断和遍历:
lst = [10,20,'python','hello']
print(10 in lst)#true
print(100 in lst)#false
print(10 not in lst)#false
for item in lst:
print(item)#遍历
s.count(x):返回序列s中出现x的总次数
5:元素的添加:
lst = [10,20,30]
lst.append(100)
lst2 = ['hello','world']
lst.extend(lst2)#[10, 20, 30, 100, 'hello', 'world']
lst.insert(1,90)#在1的位置上添加一个元素
#在任意位置添加N多个元素
lst3 = [True,False,'hello']
lst[1:]=lst3 #在1的位置开始切 [10,True,False,'hello]
ls += lt #更新列表ls,将列表lt元素增加到列表ls中
ls *= n #更新列表ls,其元素重复n次
6:元素的删除:
lst = [10,20,30,40,50,60,30]
lst.remove(30)#从列表中移除一个元素,重复元素移除第一个[10,20,40,50,60,30]
lst.pop(1 )#根据索引移除 第一个数被移除 [10,40,50,60,30]
lst.pop()#不指定参数则会删除最后一个元素 [10,40,50,60]
#切片操作删除至少一个元素,产生一个新的列表对象
new_list = lst[1:3]#[40,50]
#不产生
lst[1:3]=[] #lst:[10,60]
#清除所有元素
lst.clear() #[]
#删除列表
del lst
# 删除列表ls中第i元素
del ls[i]
7:列表修改:
lst = [10,20,30,40]
lst[2] = 100#[10,20,100,40]
lst[1:3] = [300,400,500,600]#[10,300,400,500,600,40]
8:列表的排序:
lst = [20,40,10,98,54]
lst.sort() #升序排序[10,20,40,54,98]
lst.sort(reverse=True)#降序排序[98,54,40,21,10]
lst.sort(reverse=False)#升序排序[10,20,40,54,98]
new_list = sorted(lst) #[10,20,40,54,98]
desc_list = sorted(lst,reverse=True)#降序排序
9:列表生成式:
lst = [i for i range(1,10)]#[1,2,3,4,5,6,7,8,9]
lst1 = [i for i range(1,10)]#[1,4,9,16,25,36,49,64,81]
三:字典(键值对):(键不能重复,值可以)(不可变对象)(无序)(可以动态伸缩)(内存大)
10:copy 列表:
# 生成一个新列表,赋值ls中所有元素
ls.copy()
1:创建:
scores = {'张三':100, '李四':98, '王五':45}#用{}
dict(name='jack', age=20)#dict 用()
#空字典:
(1):n = {} 花括号
(2):n = dict() 内置函数
2:字典元素的获取(值):
scores = {'张三':100, '李四':98, '王五':45}
scores = {'张三':100, '李四':98, '王五':45}
print(scores['张三'])
print(scores.get('张三'))
(1)用[] 若查找的键不存在,则会报错
(2):使用get() 若查找的键不存在,则会输出None
3:key 的判断是否存在
scores = {'张三':100, '李四':98, '王五':45}
print('张三' in scores) #True
print('张三' not in scores) #False
4:删除操作:del scores['张三']
5:清空操作:scores.clear()
6:添加操作:scores['陈六'] = 98
7:修改操作:scores['陈六'] = 100#直接重新赋值
8:获取字典的键值对:
scores = {'张三':100, '李四':98, '王五':45}
m = scores.keys()
print(m)
n = scores.values()
print(m)
k = scores.items()
print(m)
9:字典的遍历:
scores = {'张三':100, '李四':98, '王五':45}
for i in scores:
print(i,scores[i],scores.get(i)) 键 值 值
10:字典生成:
四:元组:
不可变序列 ,无删减改操作
创建方式:
1:t=(‘hello’,‘world’)
特殊:若只有一个元素:
t=(‘hello’,) 逗号不能省!
2:内置函数: t=tuple((‘hello’,‘world’) )
3:空元组创建:{
t=()
t=tuple()
}
元组的遍历:
1:for i in t:
print(i)
2:print(t[0])
五:集合:(没有值的字典):
1:转化(特点:没有重复元素)
s1 = {'py',1,3,3,5,5}# 集合中元素不重复 只存储一个
s2 = set(range(5))# 0-5的整数集合
s3 = set([1,2,2,3,3])#将列表转化成了集合 去除了重复元素
s4 = set((3,4,5,5))#将元组转化成了集合 去除了重复元素
s5 = set({1,2,2,3})#集合类型不变 去除了重复元素
2:创建:
s = set()
若直接使用{},类型是字典。
3:元素判断:
in / not in
4:增加操作:
(1):add()
(2):update()
4:删除操作:
remove() 删除指定元素
discard() 删除指定元素
pop() 一次值删除一个任意元素
clear() 清空集合
5:集合的关系(True/False)
相等:== 内容相同
子集:s1.issubest(s) #s1 是s的子集
超集:s1.issuperest(s)#s1是s的超集(母集)
交集:s1.isdisjoint(s)#s1和s是否有交集
|-&^并差交补
六:文件处理:
1:读取:
file = open(打开函数)(‘a.txt(文件名)’,‘r’(只读模式))
print(file.readlines)#读取全部内容 列表形式
file.close(关闭)
2:编写
file = open(打开函数)(‘a.txt(文件名)’,‘w’(覆盖写模式,完全覆盖))
file.write(‘hello’)
file.close(关闭)
file = open(打开函数)(‘a.txt(文件名)’,‘w’(覆盖写模式,完全覆盖))
file.write(‘hello’)
file.close(关闭)
3:追加内容:
file = open(打开函数)(‘a.txt(文件名)’,‘a’(追加写模式,在文件最后追加内容))
file.write(‘hello’)
file.close(关闭)
4:二进制模式
‘b’:不单独使用 ,通常和r或w一起。
5:常用方法:
filr.read([size]) 从文件中读取size个字节或字符的内容返回,若省略[size],则读取到末尾
file.readline() 读取一行内容
file.readlines() 以列表形式返回每一行
file.write(str) 将字符串str内容写入文件
file.writrlines(s_list) 将字符串s_list写入文本 不添加换行符
file.seek(offset[,whence]) 将文件指针移动到新的位置{
offset 为正 正向移动 为负 负向移动
whence :0:文件头 1:当前位置 2:文件尾
}
file.tell():返回指针当前位置
file.close() 关闭文件
七:sorted 函数
sorted(a) a 可以是元组、列表、集合、字符串、字典
八:map
a,b=map(int,input("请输入2个数字(用空格隔开)").split())
九:结巴库
import jieba
#精确模式
jieba.lcut(s)
#全模式
jieba.lcut(s,cut_all=True)
#搜索引擎模式
jieba.lcut_for_search(s)
#增加新词
jieba.add_word(w)
十:string库
十一:math库:
十二:turtle库:
turtle.pensize() #画笔粗细
turtle.color('red') #画笔颜色
turtle.speed() #画笔移动速度 画笔绘制的速度范围[0,10]整数,数字越大越快
turtle.forward() #向前移动
turtle.backward() #向后移动
turtle.right(90) #海龟方向向右转90°
turtle.left(90) #海龟方向向左转90°
turtle.penup() #提笔
turtle.pendown() #落笔
turtle.goto(x,y) #移动到(x,y)位置
turtle.setx(x) #x坐标移动到指定位置
turtle.sety(y) #y坐标移动到指定位置
turtle.circle() #画圆
turtle.dot() #画一个圆点(实心)
turtle.setheading(angle) #设置当前朝向为angle角度
turtle.home() #设置当前画笔位置为原点,朝向东(默认值)
turtle.fillcolor('red') #填充颜色
turtle.color(color1, color2) #设置 画笔颜色为color1,填充颜色为color2
turtle.begin_fill() #开始填充颜色
turtle.end_fill() #填充完成
turtle.hideturtle() #隐藏海龟图标
turtle.showturtle() #显示海龟图标
turtle.clear() #清空turtle窗口,但是turtle的位置和状态不会改变
turtle.reset() #清空turtle窗口,重置turtle状态为起始状态
turtle.undo() #撤销上一个turtle动作
turtle.isvisible() #返回当前turtle是否可见
t.write("文本" ,align="center",font=("微软雅黑",20,"normal")) #写文本
#align(可选):left,right,center;font(可选):字体名称,字体大小,字体类型(normal,bold,italic)
十三:with as :
with(上下文管理器):自动管理上下文资源
十四:类(Class)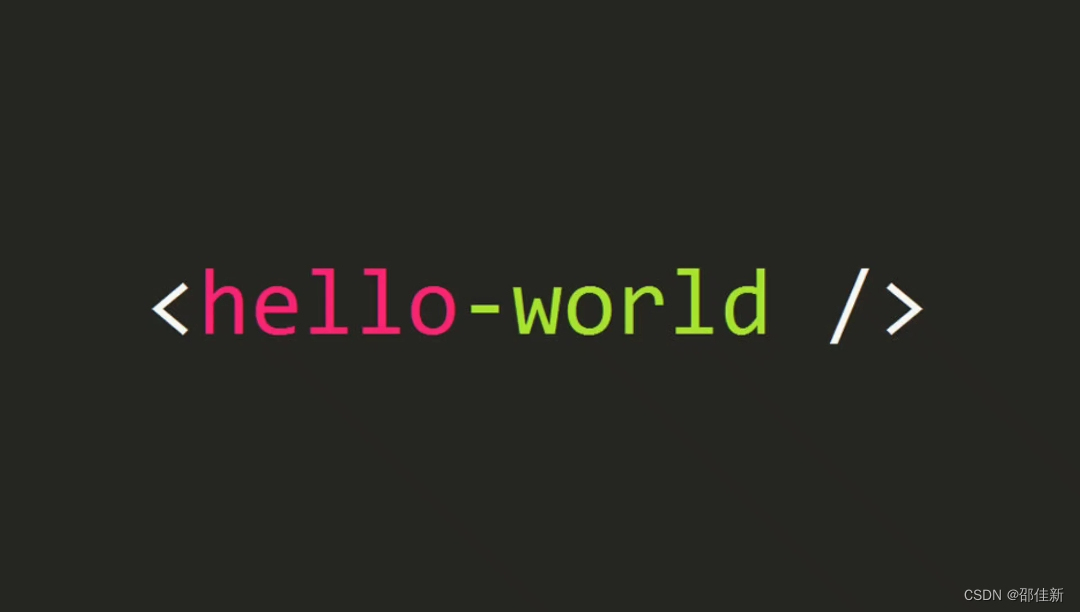
类是模板,而实例则是根据类创建的对象
是面向对象最重要的概念之一:
例如半径是圆的实例属性 pi是圆的类属性
定义类使用class关键字,class后面加类名
进入类定义时,就会创建一个新的命名空间,并把它用作局部作用域,因此函数定义会绑定到这个局部作用域中。通过定义一个特殊的__init__方法,在创建实例的时候,就把name,score等属性绑定。
注意:我们定义的类都会继承于object类,当然也可以不继承object类;两者区别不大,但没有继承于object类使用多继承时可能会出现问题。
class Circle(object): # 创建Circle类
def __init__(self, r): # 初始化一个属性r(不要忘记self参数,他是类下面所有方法必须的参数)
self.r = r # 表示给我们将要创建的实例赋予属性r赋值
十五:CSV文件
逗号分隔值(Comma-Separated Values,CSV,有时也称为字符分隔值,因为分隔字符也可以不是逗号),其文件以纯文本形式存储表格数据(数字和文本)。
csv文件是通用数据处理经常所使用的数据交换文件。其原理是用逗号来分隔数据。
excel及各种数据库软件均支持csv文件的导入和导出。
eg:
以CSV的格式保存
import csv
csvFile = open("","r")#
reader = csv.reader(csvFile)
a = list(reader)
print(a)
十六:numpy库
import numpy as np
# np.array([x,y,z],dtype=int)#从python了列表和元组创造数组
# np.arange(x,y,i)#创建从x到y,步长为i的数组
# np.linspace(x,y,n)#创建从x到y,等分成n个元素的数组
# np.indices((m,n))#创建矩阵
# np.random.rand(m,n)#创建一个随机矩阵
# np.ones((m,n),dtype)#创建一个m行n列的全是1的数组
# np.empty((m,n),dtype)#创建一个m行n列的全是0的数组
a = np.ones((4,5))
print(a)
print(a.ndim)
#创建数组后,可以查看ndarray的基本属性
print(a.ndim)#数组轴的个数,也叫秩 2
print(a.shape)#数组在每个维度上的整数元组 (4,5)
print(a.size)#数组元素的总个数
print(a.dtype)#数据类型 dtype('float64')
print(a.itemsize)#数组中每个元素的字节大小
print(a.data)#包含实际数组元素的缓冲区地址
print(a.flat)#数组元素的迭代器
十六:pandas库
pandas是提高性能易用数据类型和分析工具,常与numpy和matplotlib一起用
import pandas as pd
包含两个数据类型:series(一维)dataframe(二维)
```python
class BertPooler(nn.Module):
def __init__(self, config):
super().__init__()
self.dense = nn.Linear(config.hidden_size, config.hidden_size)
self.activation = nn.Tanh()
def forward(self, hidden_states):
# We "pool" the model by simply taking the hidden state corresponding
# to the first token.
first_token_tensor = hidden_states[:, 0]
pooled_output = self.dense(first_token_tensor)
pooled_output = self.activation(pooled_output)
return pooled_output
from transformers.models.bert.configuration_bert import *
import torch
config = BertConfig.from_pretrained("bert-base-uncased")
bert_pooler = BertPooler(config=config)
print("input to bert pooler size: {}".format(config.hidden_size))
batch_size = 1
seq_len = 2
hidden_size = 768
x = torch.rand(batch_size, seq_len, hidden_size)
y = bert_pooler(x)
print(y.size())
```