一、定义Employee
这是所有员工总的父类。
属性:员工的姓名,员工的生日月份。
方法:getSalary(int month) 根据参数月份来确定工资,如果该月员工过生日,则公司会额外奖励100元。
/**
* 所有员工的父类
*/
public abstract class Employee {
private String name; //员工姓名
private int month; // 员工生日月份
public abstract double salary(int month);
public Employee() {
}
public Employee(String name, int month) {
this.name = name;
this.month = month;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
}
二、定义各种员工类
1.SalariedEmployee: Employee的子类,拿固定工资的员工。
public class SalariedEmployee extends Employee{
private double monthSalary;
public SalariedEmployee(double monthSalary) {
this.monthSalary = monthSalary;
}
public SalariedEmployee(String name, int month, double monthSalary) {
super(name, month);
this.monthSalary = monthSalary;
}
public double getMonthSalary() {
return monthSalary;
}
public void setMonthSalary(double monthSalary) {
this.monthSalary = monthSalary;
}
@Override
public double salary(int month) {
double salary = this.monthSalary;
if (super.getMonth() == month){
salary += 400;
System.out.println(super.getName() + "本月过生日,奖励400元");
}
return salary;
}
}
2.HourlyEmployee: Employee的子类,按小时拿工资的员工,每月工作超出160小时的部分按照1.5倍工资发放。属性:每小时的工资、每月工作的小时数
public class HourlyEmployee extends Employee{
private double hourSalary;
private int hours;
public HourlyEmployee(double hourSalary, int hours) {
this.hourSalary = hourSalary;
this.hours = hours;
}
public HourlyEmployee(String name, int month, double hourSalary, int hours) {
super(name, month);
this.hourSalary = hourSalary;
this.hours = hours;
}
public double getHourSalary() {
return hourSalary;
}
public void setHourSalary(double hourSalary) {
this.hourSalary = hourSalary;
}
public int getHours() {
return hours;
}
public void setHours(int hours) {
this.hours = hours;
}
@Override
public double salary(int month) {
double salary = 0;
if (hours > 160){
salary = 160 * this.hourSalary + (this.hours - 160) * 1.5 * this.hourSalary;
}
if (super.getMonth() == month){
salary += 400;
System.out.println(super.getName() + "本月过生日,奖励400元");
}
return salary;
}
}
SalesEmployee: Employee的子类,销售人员,工资由月销售额和提成率决定。属性:月销售额、提成率
public class SalesEmployee extends Employee{
private double monthSum;
private double upRate;
public SalesEmployee(double monthSum, double upRate) {
this.monthSum = monthSum;
this.upRate = upRate;
}
public SalesEmployee(String name, int month, double monthSum, double upRate) {
super(name, month);
this.monthSum = monthSum;
this.upRate = upRate;
}
@Override
public double salary(int month) {
double salary = this.monthSum * this.upRate;
if (super.getMonth() == month){
salary += 400;
System.out.println(super.getName() + "本月过生日,奖励400元");
}
return salary;
}
public SalesEmployee() {
}
public double getMonthSum() {
return monthSum;
}
public void setMonthSum(double monthSum) {
this.monthSum = monthSum;
}
public double getUpRate() {
return upRate;
}
public void setUpRate(double upRate) {
this.upRate = upRate;
}
}
BasePlusSalesEmployee: SalesEmployee的子类,有固定底薪的销售人员,工资由底薪加上销售提成部分。 属性:底薪
public class BasePlusSaleEmployee extends SalesEmployee{
private double baseSalary;
public double getBaseSalary() {
return baseSalary;
}
public void setBaseSalary(double baseSalary) {
this.baseSalary = baseSalary;
}
public BasePlusSaleEmployee(String name, int month, double monthSum, double upRate, double baseSalary) {
super(name, month, monthSum, upRate);
this.baseSalary = baseSalary;
}
public BasePlusSaleEmployee(double baseSalary) {
this.baseSalary = baseSalary;
}
@Override
public double salary(int month) {
double finalSalary = baseSalary + super.getUpRate() * super.getMonthSum();
if (super.getMonth() == month){
finalSalary += 400;
System.out.println(super.getName() + "过生日,奖励400元");
}
return finalSalary;
}
}
三、测试类
写一个程序,把若干各种类型的员工放在一个Employee数组里,写一个方法,打印出某月每个员工的工资数额。
public class Test {
public static void main(String[] args) {
Employee[] employees = new Employee[10];
employees[0] = new SalariedEmployee("张三",3,3000);
employees[1] = new HourlyEmployee("李四",5,15.0,240);
employees[2] = new SalesEmployee("王五",6,5000,0.8);
employees[3] = new BasePlusSaleEmployee("张安", 6, 5000.0, 0.6, 3500);
for (int i = 0; i < employees.length; i++){
if (employees[i] != null){
double salary = employees[i].salary(6);
System.out.println(employees[i].getName() + "的工资是" + salary);
}
}
}
}
运行效果
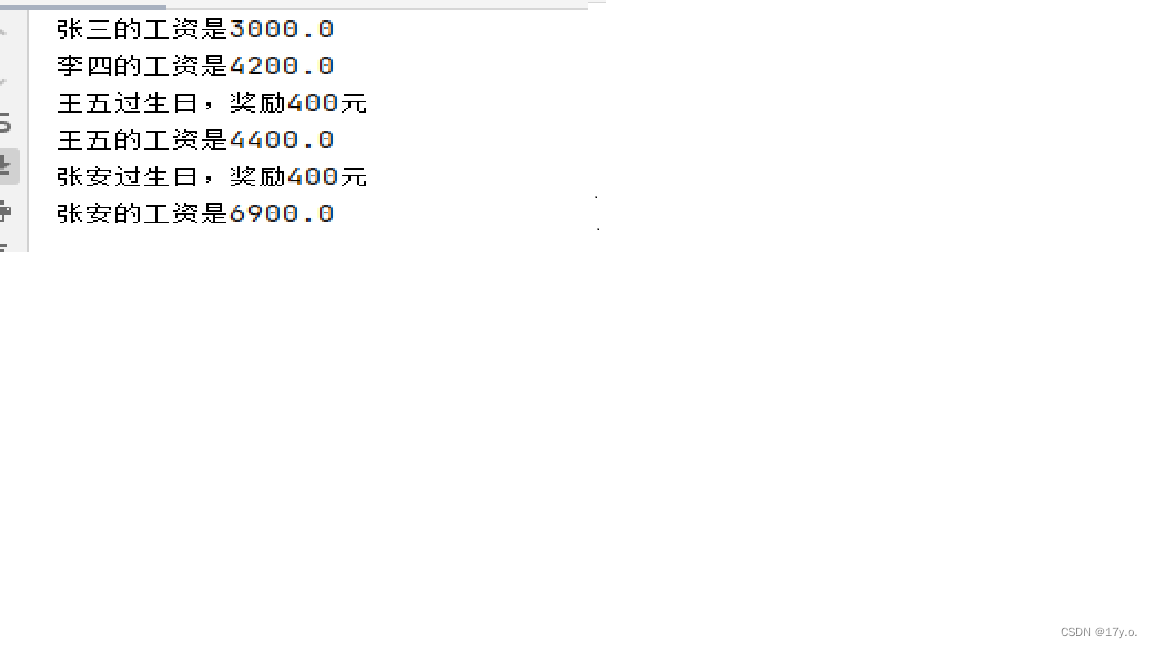