一、原型模式的用途
用原型实例指定创建对象的种类,并通过拷贝这些原型创建新的对象。
二、原型模式的实现
1.如果不适用原型模式我们以克隆羊为例子
public class Sheep {
private String name;
private int age;
private String color;
public Sheep(String name, int age, String color) {
this.name = name;
this.age = age;
this.color = color;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
return "Sheep{" +
"name='" + name + '\'' +
", age=" + age +
", color='" + color + '\'' +
'}';
}
}
如果我们要创建多只一样的羊那么只能通过多次创建
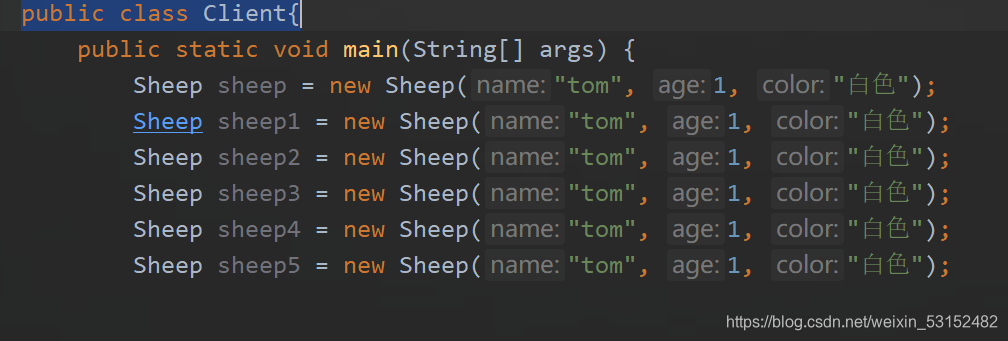
2.我们用原型模式
public class Sheep {
private String name;
private int age;
private String color;
public Sheep(String name, int age, String color) {
this.name = name;
this.age = age;
this.color = color;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
return "Sheep{" +
"name='" + name + '\'' +
", age=" + age +
", color='" + color + '\'' +
'}';
}
}
我们就可以通过这样的方式调用
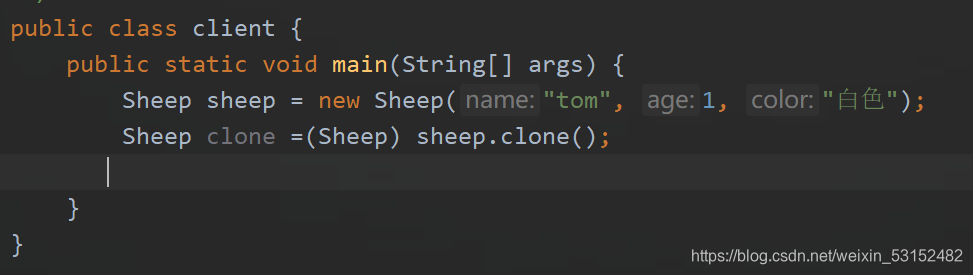
但是默认的是浅拷贝,就是说如果sheep类中有对象,那么我们拷贝的对象里面的对象会指向同一个对象,就是说sheep和clone里的对象变量指向一个对象,那么如果其中一个改变了另一个也会随之改变,那么就要使用深拷贝。 接下来列出两种深拷贝方法。
@Override
protected Object clone() throws CloneNotSupportedException {
Object deep=null;
deep=super.clone();
DeepPprotoType deepPprotoType=(DeepPprotoType) deep;
deepPprotoType.deepCloneableTarget=(DeepCloneableTarget) deepCloneableTarget.clone();
return deepPprotoType;
}
public Object deepClone(){
ByteArrayOutputStream bos=null;
ObjectOutputStream oos=null;
ByteArrayInputStream bis=null;
ObjectInputStream ois=null;
try {
bos=new ByteArrayOutputStream();
oos=new ObjectOutputStream(bos);
oos.writeObject(this);
bis=new ByteArrayInputStream(bos.toByteArray());
ois=new ObjectInputStream(bis);
DeepPprotoType c=(DeepPprotoType) ois.readObject();
return c;
}catch (Exception e){
e.printStackTrace();
return null;
}finally {
try{
bos.close();
bis.close();
oos.close();
ois.close();
}catch (Exception e2){
System.out.println(e2.getMessage());
}
}
}
二、总结
原型模式也是有不足的,他必须实现cloneable类,而且如果之前的项目没有使用你突然想使用了就要修改之前的项目代码,违反了开闭原则。