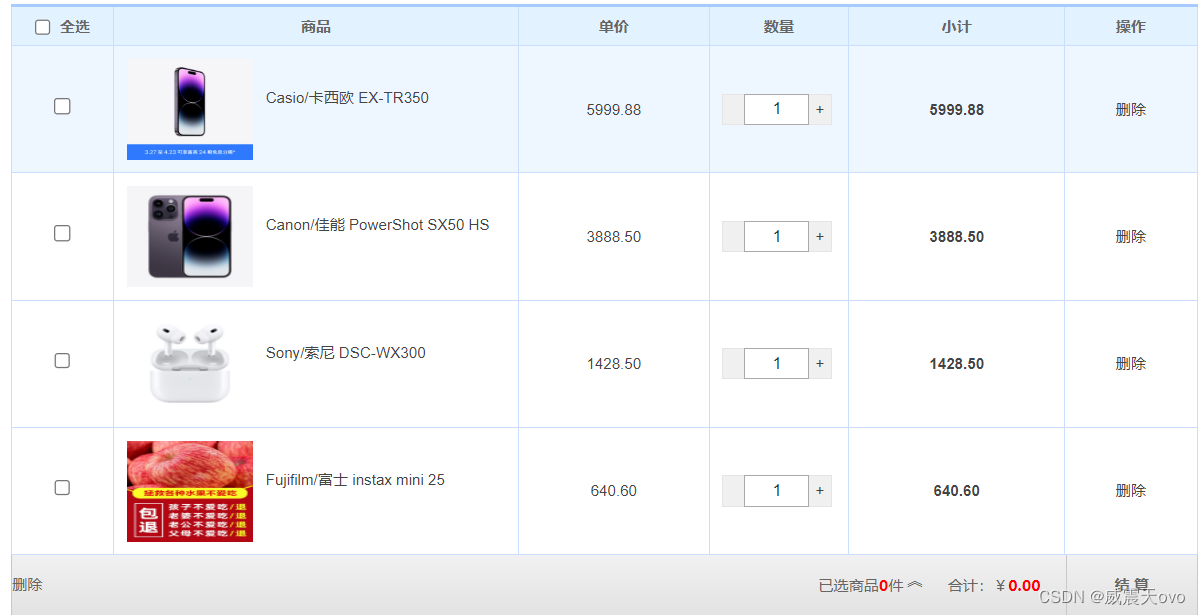
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>原生JS实现购物车结算功能代码</title>
<link rel="stylesheet" href="../css/style.css" />
</head>
<body>
<div class="catbox">
<table id="cartTable">
<thead>
<tr>
<th><label>
<input class="check-all check" type="checkbox" /> 全选</label></th>
<th>商品</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr>
<td class="checkbox"><input class="check-one check" type="checkbox" /></td>
<td class="goods"><img src="https://g-search1.alicdn.com/img/bao/uploaded/i4/imgextra/i2/50078981/O1CN01N3mQ9S2GDLtIrz1af_!!2-saturn_solar.png_580x580Q90.jpg_.webp" alt="" /><span>Casio/卡西欧 EX-TR350</span></td>
<td class="price">5999.88</td>
<td class="count"><span class="reduce"></span>
<input class="count-input" type="text" value="1" />
<span class="add">+</span>
</td>
<td class="subtotal">5999.88</td>
<td class="operation"><span class="delete">删除</span></td>
</tr>
<tr>
<td class="checkbox"><input class="check-one check" type="checkbox" /></td>
<td class="goods"><img src="https://img.alicdn.com/imgextra/i2/1917047079/O1CN01whuqS122AEWlWj2Cf_!!1917047079.png_430x430q90.jpg" alt="" /><span>Canon/佳能 PowerShot SX50 HS</span></td>
<td class="price">3888.50</td>
<td class="count"><span class="reduce"></span>
<input class="count-input" type="text" value="1" />
<span class="add">+</span>
</td>
<td class="subtotal">3888.50</td>
<td class="operation"><span class="delete">删除</span></td>
</tr>
<tr>
<td class="checkbox"><input class="check-one check" type="checkbox" /></td>
<td class="goods"><img src="https://img.alicdn.com/imgextra/i1/1917047079/O1CN01h8eEJX22AEWmwkf4L_!!0-item_pic.jpg_430x430q90.jpg" alt="" /><span>Sony/索尼 DSC-WX300</span></td>
<td class="price">1428.50</td>
<td class="count"><span class="reduce"></span>
<input class="count-input" type="text" value="1" />
<span class="add">+</span>
</td>
<td class="subtotal">1428.50</td>
<td class="operation"><span class="delete">删除</span></td>
</tr>
<tr>
<td class="checkbox"><input class="check-one check" type="checkbox" /></td>
<td class="goods"><img src="https://gw.alicdn.com/imgextra/i4/2200783283789/O1CN011XHtZI1drPToD523b_!!2200783283789.jpg_Q75.jpg_.webp" alt="" /><span>Fujifilm/富士 instax mini 25</span></td>
<td class="price">640.60</td>
<td class="count"><span class="reduce"></span>
<input class="count-input" type="text" value="1" />
<span class="add">+</span>
</td>
<td class="subtotal">640.60</td>
<td class="operation"><span class="delete">删除</span></td>
</tr>
</tbody>
</table>
<div class="foot" id="foot">
<a class="fl" id="deleteAll" href="javascript:;">删除</a>
<div class="fr closing" onclick="getTotal();">结 算</div>
<input type="hidden" id="cartTotalPrice" />
<div class="fr total">合计:¥<span id="priceTotal">0.00</span></div>
<div class="fr selected" id="selected">已选商品<span id="selectedTotal">0</span>件<span
class="arrow up">︽</span><span class="arrow down">︾</span></div>
<div class="selected-view">
<div id="selectedViewList" class="clearfix">
<div><img src="../images/1.jpg"><span>取消选择</span></div>
</div>
<span class="arrow">◆<span>◆</span></span>
</div>
</div>
</div>
</body>
<script>
// 全选 和 反选
// 全选 => 点击全选 所有单选框(check-one)的状态跟随全选(check-all)的状态 (checked)
// 反选 => 点击单选 => 判断所有的单选是否都选中 => 全都选选中 :全选反选 否则:全选不选中
var checkAll = document.getElementsByClassName("check-all")[0]; // 单元素
var checkOneList = document.getElementsByClassName("check-one"); // 多元素
var addList = document.getElementsByClassName("add");
var reduceList = document.getElementsByClassName("reduce");
var delList = document.getElementsByClassName("delete");
var deleteAll = document.getElementById("deleteAll");
var selectedTotal = document.getElementById("selectedTotal");
var priceTotal = document.getElementById("priceTotal");
// 全选 => 点击全选 所有单选框(check-one)的状态跟随全选(check-all)的状态 (checked)
// 1. 获取全选的状态
// 2. 所有单选的状态和全选保持一致
checkAll.onclick = function () {
var status = this.checked;
console.log("点击全选", status);
// for(var i = 0;i<checkOneList.length;i++){
// var checkOne = checkOneList[i];
// checkOne.checked = status;
// }
Array.from(checkOneList).forEach(function (checkOne) {
checkOne.checked = status;
})
getTotal();
}
// 反选 => 每次点击单选 => 判断所有的单选是否都选中 => 全都选选中 :全选反选 否则:全选不选中
for (var i = 0; i < checkOneList.length; i++) {
var checkOne = checkOneList[i];
checkOne.onclick = function () {
// 如何判断所有的单选是否都被选中?
// 假设法 => 先立一个假设(flag),然后尝试从对立面推翻它, => 推翻了,假设不成立 没推翻=> 成立
// var flag = true; // 假设全都被选中(假设)
// // 然后尝试从对立面推翻它 => 有一个未选中的就可以推翻假设
// for(var j=0;j<checkOneList.length;j++){
// var item = checkOneList[j]; // 每一个单选
// // item.checked == false
// // !item.checked == true => 才满足条件 => 取反前item.checked === false
// if(!item.checked){ // 存在未选中的
// flag = false; // 推翻假设
// break;
// }
// }
// checkAll.checked = flag;
// every版 => 判断是否都选中
// var flag = Array.from(checkOneList).every(function(item){
// return item.checked == true;
// })
// checkAll.checked = flag;
// 计数法
// var count = 0;
// for (var j = 0; j < checkOneList.length; j++) {
// var item = checkOneList[j];
// if (item.checked) { // 存在选中的
// count++;
// }
// }
// console.log(count, checkOneList.length);
// checkAll.checked = count == checkOneList.length ? true : false;
// 每次点击点选判断
isAllChecked();
getTotal();
// // 每次点击单选 => 找到所有选中状态的checkOne => 对应找到商品的数量和小计 => 累加
// var sum = 0; // 商品数量之和
// var allPrice = 0; // 商品的合计
// for(var j = 0;j<checkOneList.length;j++){
// var checkOne = checkOneList[j];
// if(checkOne.checked){ // 如果checkOne被选中
// // 对应找到商品的数量和小计
// var tr = parent(parent(checkOne));
// var countInput = tr.getElementsByClassName("count-input")[0];
// var subtotalTd = tr.getElementsByClassName("subtotal")[0];
// var num = countInput.value * 1;
// var subtotal = subtotalTd.textContent*1;
// sum+= num;
// allPrice += subtotal
// }
// }
// selectedTotal.textContent = sum;
// priceTotal.textContent = allPrice.toFixed(2);
}
}
// (1)加法要求
// 点击 “+” 输入框 数量 加一
// 小计更新 = 单价*数量
// 减号按钮的 “-” 出现
for (var i = 0; i < addList.length; i++) {
var add = addList[i];
add.onclick = function () {
console.log("加号被点击", this);
// a. 输入框 数量 加一
var countInput = this.previousElementSibling;
console.log(countInput);
var num = countInput.value; // num = "1" 取值
num++; // 自增
countInput.value = num; // 赋值(更新到页面)
// b. 小计更新 = 单价*数量
// 数量 => num
// 单价 =>
var parentTd = this.parentElement;
var priceTd = parentTd.previousElementSibling;
var subtotalTd = parentTd.nextElementSibling;
// "¥5999" "5999元" => 如果有单位 需要进一步处理
var price = priceTd.textContent;
var subtotal = price * num;
subtotalTd.textContent = subtotal.toFixed(2);
// c. 减号按钮的 “-” 出现
var reduce = countInput.previousElementSibling;
reduce.textContent = "-";
getTotal()
}
}
// 点击减号 输入框 数量 减一 (细节要求 数量为1的时候 不再减)
// 小计更新 = 单价*数量
// 减之前 判断 如果数量为1 不能减 return false
// 减之后 判断 如果数量为1 减号按钮的 “-” 消失
for (var i = 0; i < reduceList.length; i++) {
var reduce = reduceList[i];
reduce.onclick = function () {
console.log("减号被点击", this);
// a. 输入框 数量 减一
var countInput = next(this);
var num = countInput.value;
// c. 减之前判断 如果num == 1 不能减
if (num == 1) return false;
num--; // 自减
// d. 减之后判断 如果 num == 1 (2切1) 减号按钮的 "-" 消失
if (num == 1) {
this.textContent = "";
}
countInput.value = num;
// b. 小计更新 = 单价*数量
// 数量 => num
// 单价 =>
var parentTd = parent(this);
var priceTd = prev(parentTd);
var subtotalTd = next(parentTd);
var price = priceTd.textContent;
var subtotal = price * num;
subtotalTd.textContent = subtotal.toFixed(2);
getTotal()
}
}
// 删除细节
// a. 单删 => 每次删除之后,剩余的商品可能全都被选中 此时也可以反选 => 也需要判断是否反选
// b. 全删 => 所有商品选中后全部删除(单删/全删), 全选依然被选中 => 原因:商品全部删除之后checkOneList.length == 0 => 此时不会进入循环 假设法有问题 => flag = true;
// 解决方法: 商品数 > 0 采用假设法,否则 false
// 3. 再写删除
// 1. 单删 点击每条数据之后的删除 删除该条数据
for (var i = 0; i < delList.length; i++) {
var del = delList[i];
del.onclick = function () {
console.log("点击单删", this);
if (confirm("是否删除商品?")) {
// var tr = this.parentElement.parentElement;
var tr = parent(parent(this));
tr.remove();
// 每次删除之后,剩余的商品可能全都被选中 此时也可以反选 => 也需要判断是否反选
isAllChecked();
getTotal();
}
}
}
// 2. 多删(全删) 判断单选框是否被选中(checked) 选中就删除 该行
// 全删的问题: 删除过程中存在漏网之鱼
// 解析: 全面的tr被删除之后,checkOneList更新 => 后面的元素会批量向前移占据被删除元素的位置 => 删除时可能错过某些元素
// j 0 1 2 3
// [checkOne,checkOne,checkOne,checkOne]
deleteAll.onclick = function () {
// 删除所有选中状态的商品
for (var j = 0; j < checkOneList.length; j++) { // 假设 j=1时 checkOne被选中
var checkOne = checkOneList[j];
if (checkOne.checked) {
var tr = parent(parent(checkOne));
tr.remove();
j--; // j-- => j=0 本次循环结束 j++ => j = 1 下次还从当前位置判断
}
}
isAllChecked();
getTotal();
}
function isAllChecked() {
// 假设法 => 先立一个假设(flag),然后尝试从对立面推翻它, => 推翻了,假设不成立 没推翻=> 成立
// var flag = true; // 假设全都被选中(假设)
// // 然后尝试从对立面推翻它 => 有一个未选中的就可以推翻假设
// for(var j=0;j<checkOneList.length;j++){
// var item = checkOneList[j]; // 每一个单选
// // item.checked == false
// // !item.checked == true => 才满足条件 => 取反前item.checked === false
// if(!item.checked){ // 存在未选中的
// flag = false; // 推翻假设
// break;
// }
// }
// checkAll.checked = flag;
// every版 => 判断是否都选中
var flag = Array.from(checkOneList).every(function (item) {
return item.checked == true;
})
checkAll.checked = checkOneList.length > 0 ? flag : false;
}
// 结算=> 找到所有选中状态的checkOne => 对应找到商品的数量和小计 => 累加
function getTotal() {
var sum = 0; // 商品数量之和
var allPrice = 0; // 商品的合计
for (var j = 0; j < checkOneList.length; j++) {
var checkOne = checkOneList[j];
if (checkOne.checked) { // 如果checkOne被选中
// 对应找到商品的数量和小计
var tr = parent(parent(checkOne));
var countInput = tr.getElementsByClassName("count-input")[0];
var subtotalTd = tr.getElementsByClassName("subtotal")[0];
var num = countInput.value * 1;
var subtotal = subtotalTd.textContent * 1;
sum += num;
allPrice += subtotal
}
}
selectedTotal.textContent = sum;
priceTotal.textContent = allPrice.toFixed(2);
}
function parent(ele) {
return ele.parentElement;
}
function prev(ele) {
return ele.previousElementSibling;
}
function next(ele) {
return ele.nextElementSibling;
}
</script>
</html>
@charset "utf-8";
*{margin:0;padding:0;list-style-type:none;}
a{color:#666;text-decoration:none;}
table{border-collapse:collapse;border-spacing:0;border:0;}
body{color:#666;font:12px/180% Arial, Helvetica, sans-serif, "新宋体";}
clearfix:after{content:".";display:block;height:0;clear:both;visibility:hidden}
.clearfix{display:inline-table}
*html .clearfix{height:1%}
.clearfix{display:block}
*+html .clearfix{min-height:1%}
.fl{float:left;}
.fr{float:right;}
/*素材家园 - www.sucaijiayuan.com*/
.catbox{width:940px;margin:100px auto;}
.catbox table{text-align:center;width:100%;}
.catbox table th,.catbox table td{border:1px solid #CADEFF;}
.catbox table th{background:#e2f2ff;border-top:3px solid #a7cbff;height:30px;}
.catbox table td{padding:10px;color:#444;}
.catbox table tbody tr:hover{background:RGB(238,246,255);}
.checkbox{width:60px;}
.check-all{ vertical-align:middle;}
.goods{width:300px;}
.goods span{width:180px;margin-top:20px;text-align:left;float:left;}
.goods img{width:100px;height:80px;margin-right:10px;float:left;}
.price{width:130px;}
.count{width:90px;}
.count .add, .count input, .count .reduce{float:left;margin-right:-1px;position:relative;z-index:0;}
.count .add, .count .reduce{height:23px;width:17px;border:1px solid #e5e5e5;background:#f0f0f0;text-align:center;line-height:23px;color:#444;}
.count .add:hover, .count .reduce:hover{color:#f50;z-index:3;border-color:#f60;cursor:pointer;}
.count input{width:50px;height:15px;line-height:15px;border:1px solid #aaa;color:#343434;text-align:center;padding:4px 0;background-color:#fff;z-index:2;}
.subtotal{width:150px;color:red;font-weight:bold;}
.operation span:hover,a:hover{cursor:pointer;color:red;text-decoration:underline;}
.foot{margin-top:0px;color:#666;height:48px;border:1px solid #c8c8c8;border-top:0;background-color:#eaeaea;background-image:linear-gradient(RGB(241,241,241),RGB(226,226,226));position:relative;z-index:8;}
.foot div, .foot a{line-height:48px;height:48px;}
.foot .select-all{width:80px;height:48px;line-height:48px;color:#666;text-align:center;}
.foot .delete{padding-left:10px;}
.foot .closing{border-left:1px solid #c8c8c8;width:103px;text-align:center;color:#666;font-weight:bold;cursor:pointer;background-image:linear-gradient(RGB(241,241,241),RGB(226,226,226));}
.foot .closing:hover{background-image:linear-gradient(RGB(226,226,226),RGB(241,241,241));color:#333;}
.foot .total{margin:0 20px;cursor:pointer;}
.foot #priceTotal, .foot #selectedTotal{color:red;font-family:"Microsoft Yahei";font-weight:bold;}
.foot .selected{cursor:pointer;}
.foot .selected .arrow{position:relative;top:-3px;margin-left:3px;}
.foot .selected .down{position:relative;top:3px;display:none;}
.show .selected .down{display:inline;}
.show .selected .up{display:none;}
.foot .selected:hover .arrow{color:red;}
.foot .selected-view{width:938px;border:1px solid #c8c8c8;position:absolute;height:auto;background:#ffffff;z-index:9;bottom:48px;left:-1px;display:none;}
.show .selected-view{display:block;}
.foot .selected-view div{height:auto;}
.foot .selected-view .arrow{font-size:16px;line-height:100%;color:#c8c8c8;position:absolute;right:330px;bottom:-9px;}
.foot .selected-view .arrow span{color:#ffffff;position:absolute;left:0px;bottom:1px;}
#selectedViewList{padding:10px 20px 10px 20px;}
#selectedViewList div{display:inline-block;position:relative;width:100px;height:80px;border:1px solid #ccc;margin:10px;float:left;}
#selectedViewList div img{width:100px;height:80px;margin-right:10px;float:left;}
#selectedViewList div span{display:none;color:#ffffff;font-size:12px;position:absolute;top:0px;right:0px;width:60px;height:18px;line-height:18px;text-align:center;background:#000;cursor:pointer;}
#selectedViewList div:hover span{display:block;}