8563
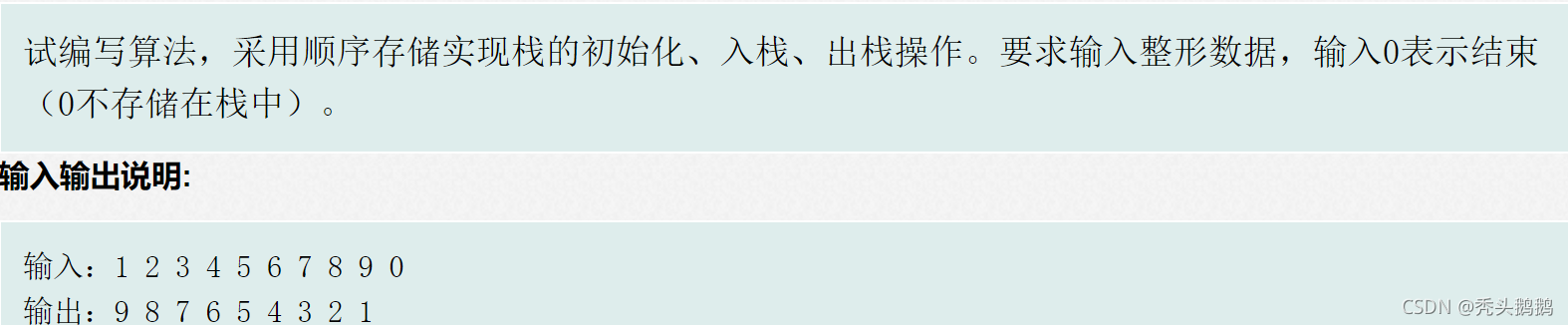
#include <bits/stdc++.h>
using namespace std;
#define Maxsize 100
#define SElemtype int
#define OK 1
typedef struct {
SElemtype *base;
SElemtype *top;
int stacksize;
}sqstack;
void init(sqstack &s)
{
s.base=new SElemtype[Maxsize];
if(!s.base)
exit(OVERFLOW);
s.top=s.base;
s.stacksize=Maxsize;
}
void in(sqstack &s,SElemtype e)
{
*s.top++=e;
}
void out(sqstack &s,SElemtype &e)
{
while(s.top!=s.base)
{e=*--s.top;
cout<<e;}
}
int main()
{ sqstack s;
init(s);
int n,x,e;
cin>>x>>n;
printf("%d(%d)=",x,10);
while(x/n!=0){
e=x%n;
x=x/n;
in(s,e);
}
in(s,x);
out(s,e);
printf("(%d)",n);
return 0;
}
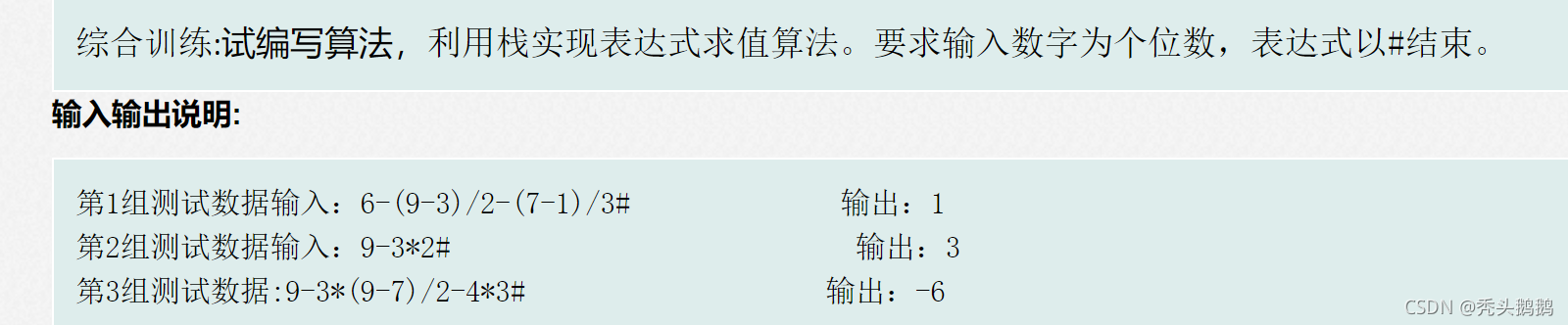
#include<iostream>
#include<stack>
using namespace std;
int Calculate_Func(int n1, char opt, int n2);
int main() {
stack<char> optstack;
stack<int> numstack;
optstack.push('#');
char ch;
ch = getchar();
char ctemp;
char ntemp1, ntemp2;
while (ch != '#') {
if (ch <= '9' && ch >= '0') {
numstack.push(ch-'0');
}
if (ch == '(' || ch == ')' || ch == '[' || ch == ']' || ch == '*' || ch == '/' || ch == '+' || ch == '-' || ch == '{' || ch == '}') {
switch (ch) {
case '{':
optstack.push(ch);
break;
case '[':
optstack.push(ch);
break;
case '(':
optstack.push(ch);
break;
case '}':
ctemp = optstack.top();
optstack.pop();
while (ctemp != '{') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
ctemp = optstack.top();
optstack.pop();
}
if (ctemp == '[' || ctemp == '(') {
cout << 0;
return 0;
}
break;
case ']':
ctemp = optstack.top();
optstack.pop();
while (ctemp != '[') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
ctemp = optstack.top();
optstack.pop();
}
if (ctemp == '{' || ctemp == '(') {
cout << 0;
return 0;
}
break;
case ')':
ctemp = optstack.top();
optstack.pop();
while (ctemp != '(') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
ctemp = optstack.top();
optstack.pop();
}
if (ctemp == '{' || ctemp == '[') {
cout << 0;
return 0;
}
break;
case '+':
ctemp = optstack.top();
optstack.pop();
if (ctemp == '(' || ctemp == '{' || ctemp == '[' || ctemp == '+' || ctemp == '-') {
optstack.push(ctemp);
optstack.push(ch);
}
else {
while (ctemp == '*' || ctemp == '/') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
ctemp = optstack.top();
optstack.pop();
}
optstack.push(ctemp);
optstack.push(ch);
}
break;
case '-':
ctemp = optstack.top();
optstack.pop();
if (ctemp == '(' || ctemp == '{' || ctemp == '[' || ctemp == '+' || ctemp == '-') {
optstack.push(ctemp);
optstack.push(ch);
}
else {
while (ctemp == '*' || ctemp == '/') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
ctemp = optstack.top();
optstack.pop();
}
if (ctemp == ch) {
optstack.push(ctemp);
optstack.push('+');
break;
}
optstack.push(ctemp);
optstack.push(ch);
}
break;
case '*':
optstack.push(ch);
break;
case '/':
optstack.push(ch);
break;
}
}
ch = getchar();
}
while (optstack.top() != '#') {
ntemp1 = numstack.top();
numstack.pop();
ntemp2 = numstack.top();
numstack.pop();
ctemp = optstack.top();
optstack.pop();
numstack.push(Calculate_Func(ntemp1, ctemp, ntemp2));
}
cout << numstack.top() << endl;
return 0;
}
int Calculate_Func(int n1, char opt, int n2) {
switch (opt) {
case '+':
return n1 + n2;
case '-':
return n2 - n1;
case '*':
return n1 * n2;
case '/':
return n2 / n1;
}
}
8564
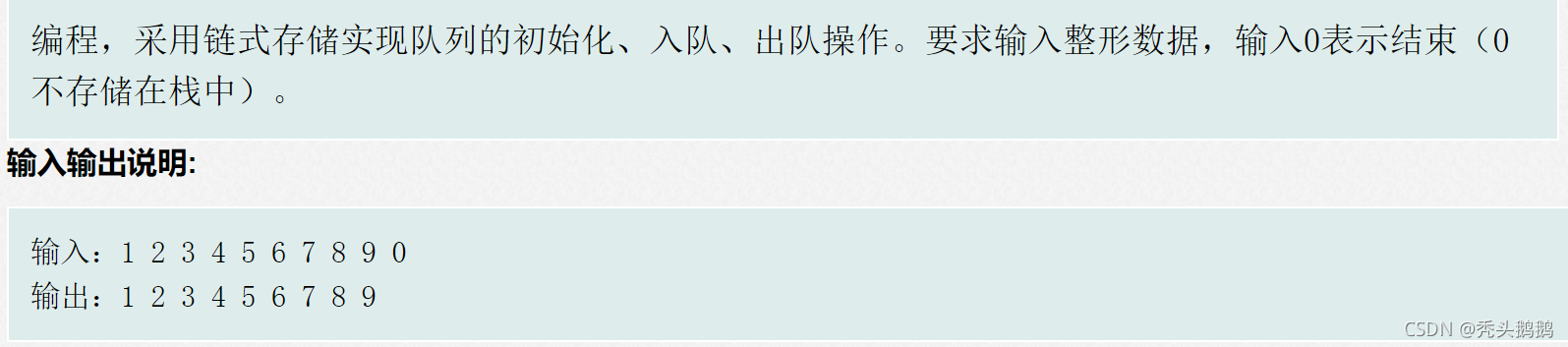
#include <bits/stdc++.h>
using namespace std;
#define Maxsize 100
#define Elemtype int
typedef struct node {
int data;
node* prior;
node* next;
}node;
typedef struct queue {
node* front;
node* rear;
}linkqueue;
void in(linkqueue &q,Elemtype e)
{
node *p=new node;
p->data=e;
p->next=NULL;
q.rear->next=p;
q.rear=p;
}
void out(linkqueue &q)
{
while(q.front->next!=q.rear)
{ node *p=new node;
p=q.front->next;
int n=p->data;
q.front->next=q.front->next->next;
cout<<n<<' ';
}
cout<<q.rear->data;
}
int main()
{
linkqueue q;
q.front=q.rear=new node;
q.front->next=NULL;
int n;
cin>>n;
while(n)
{
in(q,n);
cin>>n;
}
out(q);
return 0;
}
8565
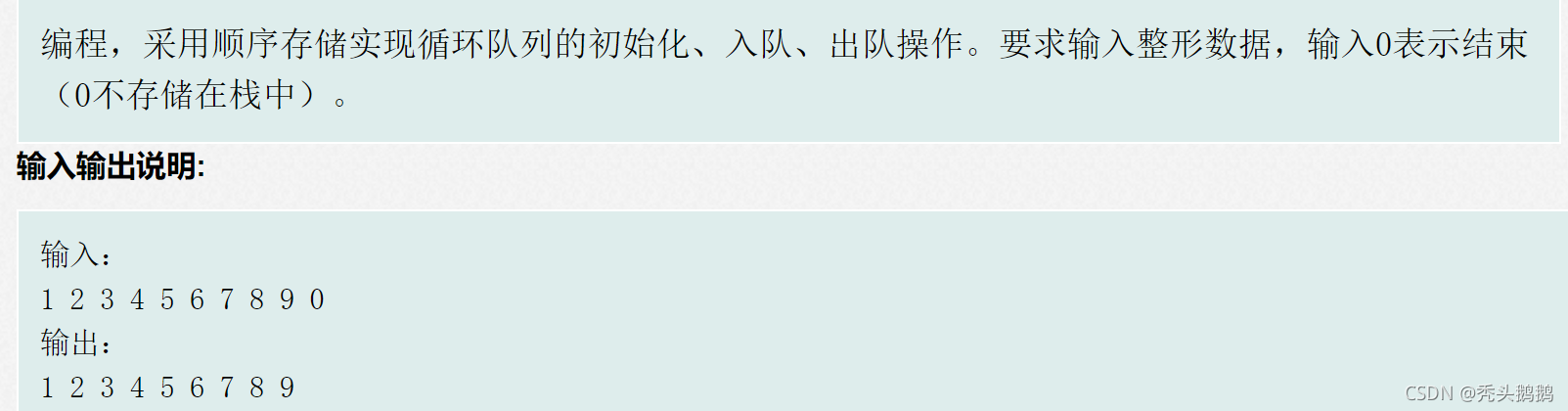
#include <bits/stdc++.h>
using namespace std;
#define Maxsize 100
typedef struct
{
int base[100];
int front,rear;
}squeue;
void in(squeue &q,int n)
{
q.rear=0;
while(n)
{q.base[q.rear]=n;
q.rear++;
cin>>n;
}
}
void out (squeue &q)
{int e;
while((q.rear-q.front+100)%100>0)
{
e=q.base[q.front];
q.front++;
cout<<e<<' ';
}
}
int main()
{ squeue q;
q.front=q.rear=0;
int n;cin>>n;
in(q,n);
out(q);
return 0;
}