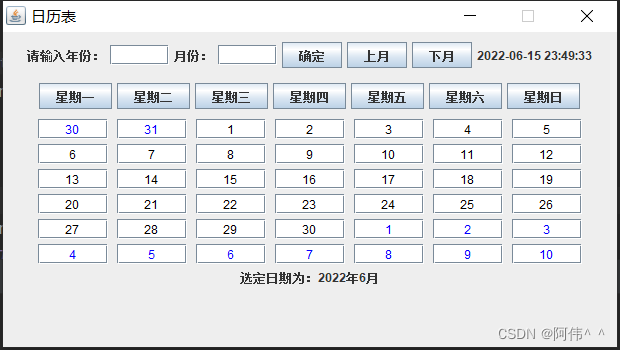
这次制作了一个可以实时显示日期的日历,其中包括搜索日期,实时显示当前日期,同时显示上下月的日期
package Test1; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; public class Gui2 extends JFrame implements ActionListener { JFrame jf = new JFrame("日历表"); JButton define = new JButton("确定"); JButton preMonth = new JButton("上月"); JButton nextMonth = new JButton("下月"); JLabel getYear = new JLabel("请输入年份:"); JLabel getMonth = new JLabel("月份:"); JTextField year = new JTextField(5); JTextField month = new JTextField(5); static JLabel Time = new JLabel(); JLabel Dep = new JLabel(); JPanel jp2 = new JPanel(); Container maxBox = jf.getContentPane(); Gui2(){ JButton j1 = new JButton("星期一"); JButton j2 = new JButton("星期二"); JButton j3 = new JButton("星期三"); JButton j4 = new JButton("星期四"); JButton j5 = new JButton("星期五"); JButton j6 = new JButton("星期六"); JButton j7 = new JButton("星期日"); jf.setLayout(new FlowLayout()); JPanel jp = new JPanel(); jp.add(getYear); jp.add(year); jp.add(getMonth); jp.add(month); jp.add(define); define.addActionListener(this); jp.add(preMonth); preMonth.addActionListener(this); jp.add(nextMonth); nextMonth.addActionListener(this); jp.add(Time); maxBox.add(jp); JPanel jp3 = new JPanel(); jp3.setLayout(new FlowLayout()); jp3.add(j1); jp3.add(j2); jp3.add(j3); jp3.add(j4); jp3.add(j5); jp3.add(j6); jp3.add(j7); showCalendar(); jp2.setLayout(null); jp2.setLayout(new GridLayout(6,7,9,5)); maxBox.add(jp3); maxBox.add(jp2); maxBox.add(Dep); jf.setResizable(false); jf.setVisible(true); jf.setSize(620,350); jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } //显示日历界面 public void showCalendar(){ Start2.nowTime.set(Calendar.DATE,1); Start2.nowDay = Start2.nowTime.get(Calendar.DATE); int count = 1; int weekDay = Start2.getFirstWeekDay(Start2.nowYear,Start2.nowMonth,Start2.nowDay); int monthDay = Start2.getMonthDays(Start2.nowYear, Start2.nowMonth); ; int preMonthDay = Start2.getMonthDays(Start2.nowYear, Start2.nowMonth -1); int preDay = preMonthDay-weekDay+2; for(int i =1;i<=42;i++) { JTextField j = new JTextField(6); j.setHorizontalAlignment(SwingConstants.CENTER); if (i >=weekDay && (i-weekDay+1)<=monthDay){ j.setForeground(Color.black); j.setText(""+(i-weekDay+1)); } else if (i <weekDay) { j.setText(""+preDay); j.setForeground(Color.blue); preDay++; }else { j.setText(String.valueOf(count)); j.setForeground(Color.blue); count++; } jp2.add(j); } Dep.setText("选定日期为:"+ Start2.nowYear +"年"+ Start2.nowMonth +"月"); } //显示当前时间 public static void showNowTime() { try { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Time.setText(sdf.format(new Date())); Thread.sleep(1000); }catch (InterruptedException exception){ System.out.println("error"); } } //监听按钮 @Override public void actionPerformed(ActionEvent e) throws RuntimeException{ if (e.getSource()==preMonth){ if (Start2.nowMonth ==0) { Start2.nowMonth = 12; Start2.nowYear -=1; } Start2.nowMonth -=1; jp2.removeAll(); jp2.repaint(); showCalendar(); } if (e.getSource() ==nextMonth){ if (Start2.nowMonth ==12) { Start2.nowMonth = 0; Start2.nowYear +=1; } Start2.nowMonth +=1; jp2.removeAll(); jp2.repaint(); showCalendar(); } try{ if (e.getSource() ==define){ int Y = Integer.parseInt(year.getText()); int M = Integer.parseInt(month.getText()); Start2.nowMonth = M; Start2.nowYear = Y; jp2.removeAll(); jp2.repaint(); showCalendar(); } }catch (NumberFormatException exception){ JOptionPane.showMessageDialog(this.getContentPane(), "暂无查询对象"); } } } 另起一个类
package Test1; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; public class Start2 extends Thread{ static Calendar nowTime = Calendar.getInstance(); static int nowYear = nowTime.get(Calendar.YEAR); static int nowMonth = nowTime.get(Calendar.MONTH)+1; static int nowDay = nowTime.get(Calendar.DATE); //判断所选月份的天数,并返回 public static int getMonthDays(int year, int month){ int monthDays =0; if (month ==4 || month ==6 || month ==9 || month ==11){ monthDays =30; }else if (month ==2){ if (year%4==0 && year%100!=0 || year%400==0){ monthDays =29; }else monthDays =28; }else monthDays =31; return monthDays; } //返回这个月第一天是星期几 public static int getFirstWeekDay(int year, int month, int day){ nowTime.set(Calendar.YEAR,year); nowTime.set(Calendar.MONTH,month); nowTime.set(Calendar.DATE,day); String date = nowYear+"-"+nowMonth+"-"+nowDay; SimpleDateFormat dFormat = new SimpleDateFormat("yyyy-MM-dd"); Date parse; int c=0; try { parse = dFormat.parse(date); Calendar calendar = new GregorianCalendar(); calendar.setTime(parse);// 把格式化好的日期对象放进Calendar c = calendar.get(Calendar.DAY_OF_WEEK); } catch (ParseException e) { e.printStackTrace(); } if (c-1 ==0) return 7; return c-1; } //线程调用,做到实时显示时间 public void run(){ while (true) Gui2.showNowTime(); } public static void main(String[] args) { new Start2().start(); new Gui2(); } }