1.mustache动态插值
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<script src="./lib/vue.js"></script>
<script>
const app = Vue.createApp({
// 插值语法: {{title}}
template: `<h2>{{message}}</h2>`,
data: function() {
return {
title: "Hello World",
message: "你好啊, Vue3"
}
}
})
app.mount("#app")
</script>
</body>
</html>
2.v-for遍历列表
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<script src="./lib/vue.js"></script>
<script>
const app = Vue.createApp({
template: `
<h2>电影列表</h2>
<ul>
<li v-for="item in movies">{{item}}</li>
</ul>
`,
data: function() {
return {
message: "你好啊, 李银河",
movies: [ "大话西游", "星际穿越", "盗梦空间", "少年派", "飞驰人生" ]
}
}
})
app.mount("#app")
</script>
</body>
</html>
3.@click事件监听
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<script src="./lib/vue.js"></script>
<script>
const app = Vue.createApp({
template: `
<h2>当前计数: {{counter}}</h2>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
`,
data: function() {
return {
counter: 0
}
},
methods: {
increment: function() {
this.counter++
},
decrement: function() {
this.counter--
}
}
})
app.mount("#app")
</script>
</body>
</html>
template如果里面有内容,会覆盖掉app里面的所有内容,如果无内容,默认渲染app内的内容
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>当前计数: {{counter}}</h2>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
</div>
<script src="./lib/vue.js"></script>
<script>
const app = Vue.createApp({
data: function() {
return {
counter: 0
}
},
methods: {
increment: function() {
this.counter++
},
decrement: function() {
this.counter--
}
}
})
app.mount("#app")
</script>
</body>
</html>
4.js原生实现计数器
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h2>当前计数: <span class="counter"></span></h2>
<button class="add">+1</button>
<button class="sub">-1</button>
<script>
// 1.获取dom
const h2El = document.querySelector("h2")
const counterEl = document.querySelector(".counter")
const addBtnEl = document.querySelector(".add")
const subBtnEl = document.querySelector(".sub")
// 2.定义一个变量记录数据
let counter = 100
counterEl.textContent = counter
// 2.监听按钮的点击
addBtnEl.onclick = function() {
counter++
counterEl.textContent = counter
}
subBtnEl.onclick = function() {
counter--
counterEl.textContent = counter
}
</script>
</body>
</html>
5.命令式与响应式编程
js原生是命令式编程,vue是响应式编程
原生开发和 Vue 开发的模式和特点,是完全不同的,这里其实涉及到两种不同的编程范式:
命令式编程关注的是 “ how to do” 自己完成整个 how 的过程;
声明式编程关注的是 “ what to do” ,由框架 ( 机器 ) 完成 “ how” 的过程;
在js原生的实现过程中,我们是如何操作的呢?
我们每完成一个操作,都需要通过 JavaScript 编写一条代码,来给浏览器一个指令;
这样的编写代码的过程,我们称之为命令式编程;
在早期的原生 JavaScript 和 jQuery 开发的过程中,我们都是通过这种命令式的方式在编写代码的;
在 Vue 的实现过程中,我们是如何操作的呢?
我们会在 createApp 传入的对象中声明需要的内容,模板 template 、数据 data 、方法 methods ;
这样的编写代码的过程,我们称之为是声明式编程;
目前 Vue 、 React 、 Angular 、小程序的编程模式,我们称之为声明式编程;
6.data属性
data: function() {
return {
message: "Hello Data"
}
},
data 属性是传入一个函数,并且该函数需要返回一个对象:
在 Vue2.x 的时候,也可以传入一个对象(虽然官方推荐是一个函数);
在 Vue3.x 的时候,必须传入一个函数,否则就会直接在浏览器中报错;
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>{{message}}</h2>
<button @click="changeMessage">改变message</button>
</div>
<script src="./lib/vue.js"></script>
<script>
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Data"
}
},
// methods: option api
methods: {
changeMessage: function() {
this.message = "你好, 师姐!"
}
}
})
app.mount("#app")
</script>
</body>
</html>
7.methods 属性
methods 属性是一个对象,通常我们会在这个对象中定义很多的方法:
这些方法可以被绑定到 模板中;
在该方法中,我们可以使用 this 关键字来直接访问到 data 中返回的对象的属性;
对于有经验的同学,在这里我提一个问题,官方文档有这么一段描述:
问题一:为什么不能使用箭头函数(官方文档有给出解释)?
问题二:不使用箭头函数的情况下, this 到底指向的是什么?(可以作为一道面试题)
8.代码片段
9.模板:mustache插值语法
在模板中,允许开发者以声明式的方式将 DOM 和底层组件实例的数据绑定在一起;
在底层的实现中, Vue 将模板编译成虚拟 DOM 渲染函数
如果我们希望把数据显示到模板( template )中,使用最多的语法是 “ Mustache” 语法 ( 双大括号 ) 的文本插值。
并且我们前端提到过, data 返回的对象是有添加到 Vue 的响应式系统中;
当 data 中的数据发生改变时,对应的内容也会发生更新。
当然, Mustache 中不仅仅可以是 data 中的属性,也可以是一个 JavaScript 的表达式。
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<!-- 1.基本使用 -->
<h2>{{ message }}</h2>
<h2>当前计数: {{ counter }} </h2>
<!-- 2.表达式 -->
<h2>计数双倍: {{ counter * 2 }}</h2>
<h2>展示的信息: {{ info.split(" ") }}</h2>
<!-- 3.三元运算符 -->
<h2>{{ age >= 18? "成年人": "未成年人" }}</h2>
<!-- 4.调用methods中函数 -->
<h2>{{ formatDate(time) }}</h2>
<!-- 5.注意: 这里不能定义语句 -->
<!-- <h2>{{ const name = "why" }}</h2> -->
</div>
<script src="../lib/vue.js"></script>
<script>
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Vue",
counter: 100,
info: "my name is why",
age: 22,
time: 123
}
},
methods: {
formatDate: function(date) {
return "2022-10-10-" + date
}
}
})
// 2.挂载app
app.mount("#app")
</script>
</body>
</html>
10.v-once:只渲染一次
只渲染一次,比如counter,每次点击数值+10,控制台此处每次输出counter值,每次+10,但是页面上counter数值不变
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<!-- 指令: v-once -->
<h2 v-once>
{{ message }}
<span>数字: {{counter}}</span>
</h2>
<h1>{{message}}</h1>
<button @click="changeMessage">改变message</button>
</div>
<script src="../lib/vue.js"></script>
<script>
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Vue",
counter: 100
}
},
methods: {
changeMessage: function() {
this.message = "你好啊, 李银河"
this.counter += 100
console.log(this.message, this.counter)
}
}
})
// 2.挂载app
app.mount("#app")
</script>
</body>
</html>
11.v-text 指令:更新元素内容
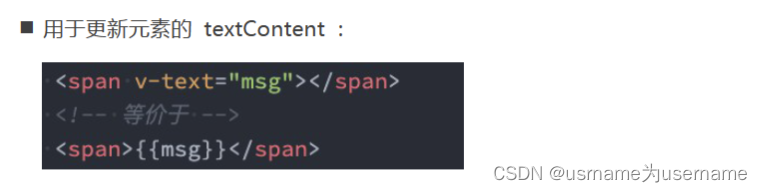
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2> aa {{message}} bbb</h2>
<h2 v-text="message">aaa</h2>
</div>
<script src="../lib/vue.js"></script>
<script>
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Vue"
}
},
})
// 2.挂载app
app.mount("#app")
</script>
</body>
</html>
12.v-html:解析html语言
默认情况下,如果我们展示的内容本身是 html 的,那么 vue 并不会对其进行特殊的解析。
如果我们希望这个内容被 Vue 可以解析出来,那么可以使用 v-html 来展示;
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h2>{{ content }}</h2>
<h2 v-html="content"></h2>
</div>
<script src="../lib/vue.js"></script>
<script>
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
content: `<span style="color: red; font-size: 30px;">哈哈哈</span>`
}
},
})
// 2.挂载app
app.mount("#app")
</script>
</body>
</html>
13.v-pre:显示原始的 Mustache 标签
v-pre 用于跳过元素和它的子元素的编译过程,显示原始的 Mustache 标签:
跳过不需要编译的节点,加快编译的速度;
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<div v-pre>
<h2>{{ message }}</h2>
<p>当前计数: {{ counter }}</p>
<p>{{}}</p>
</div>
</div>
<script src="../lib/vue.js"></script>
<script>
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Vue",
counter: 0
}
},
})
// 2.挂载app
app.mount("#app")
</script>
</body>
</html>
14.v-cloak:常用于等元素渲染完毕再展示
这个指令保持在元素上直到关联组件实例结束编译。
和 CSS 规则如 [v-cloak] { display: none } 一起用时,这个指令可以隐藏未编译的 Mustache 标签直到组件实例准备完毕。 <div> 不会显示,直到编译结束。
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
[v-cloak] {
display: none;
}
</style>
</head>
<body>
<div id="app">
<h2 v-cloak>{{message}}</h2>
</div>
<script src="../lib/vue.js"></script>
<script>
setTimeout(() => {
// 1.创建app
const app = Vue.createApp({
// data: option api
data: function() {
return {
message: "Hello Vue"
}
},
})
// 2.挂载app
app.mount("#app")
}, 3000)
</script>
</body>
</html>
15.v-memo???????????????
16.v-bind(重点)
v-bind复习_usrname为username的博客-CSDN博客
17.v-on
v-on绑定事件_usrname为username的博客-CSDN博客
18.条件渲染
vue条件渲染复习_usrname为username的博客-CSDN博客
19.