1.所需关键依赖
<!--pdf导出工具依赖-->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
<!--解决中文字体不显示问题-->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itext-asian</artifactId>
<version>5.2.0</version>
</dependency>
2.方法参数如下
//file1和file2为照片
public void exportPdf(MultipartFile file1, MultipartFile file2, HttpServletResponse response)
3.开始画文档
step1.创建一个pdf文档,关联一个OutputStream,打开文档
//新建一个A4大小的文档
Document document = new Document(PageSize.A4);
//创建一个ByteArrayOutputStream绑定document,后续只需要将数据流写入baos即可
ByteArrayOutputStream baos = new ByteArrayOutputStream();
PdfWriter.getInstance(document, baos);
//打开文档
document.open();
step2.解决中文字体不显示问题
// 设置中文字体
BaseFont bfChinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
//文档标题字体样式
Font titleFontChinese = new Font(bfChinese, 15, Font.BOLD);
//小标题字体样式
Font smallTitleFontChinese = new Font(bfChinese, 8, Font.BOLD);
//表头字体样式
Font headFontChinese = new Font(bfChinese, 8, Font.BOLD);
//内容字体样式
Font contentFontChinese = new Font(bfChinese, 9, Font.NORMAL);
step3.设置文档标题
代码:
//这一步将step2中的titleFontChinese设置进来即可解决中文字体不显示问题
Paragraph title = new Paragraph("文档标题", titleFontChinese);
//标题居中
title.setAlignment(Element.ALIGN_CENTER);
//将标题添加到文档中
document.add(title);
效果:

step4.插入第一张图片
代码:
//保持美观,空一行
document.add(new Paragraph(" "));
//设置小标题
document.add(new Paragraph("图片一", smallTitleFontChinese));
// 添加第一张图片到PDF
Image img1 = Image.getInstance(file1.getBytes());
//重新设置图片大小,适配PDF
img1.scaleToFit(525, img1.getHeight());
//将图片添加进PDF
document.add(img1);
效果:
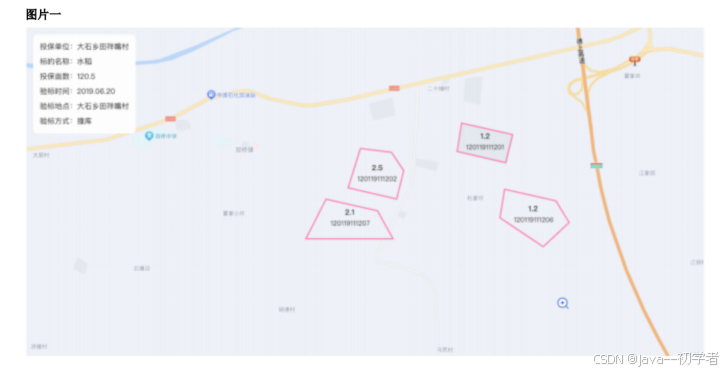
step5.插入第二张图片并在右侧插入一个表格
代码:
//这个地方的基础思路还是利用表格实现
//添加一行间隔
document.add(new Paragraph(" "));
//创建一个包含两列的表格,这个表格里面左列是图片,右列是表格
PdfPTable table = new PdfPTable(2);
//设置表格宽度
table.setWidthPercentage(100);
//希望最终图片的大小小于右边的表格,需要划分两列比例
table.setWidths(new int[]{1, 2});
//第一列添加图片
PdfPCell imageCell = new PdfPCell();
Image img2 = Image.getInstance(file2.getBytes());
//设置内部表格宽度为100%
img2.setWidthPercentage(100);
imageCell.addElement(img2);
//设置图片水平居中
imageCell.setHorizontalAlignment(Element.ALIGN_CENTER);
//设置图片垂直居中
imageCell.setVerticalAlignment(Element.ALIGN_MIDDLE);
//设置单元格无边框
imageCell.setBorder(Rectangle.NO_BORDER);
//将带图片的单元格设置进入表格中
table.addCell(imageCell);
//第二列添加表格
//内部表格,包含四列
PdfPTable innerTable = new PdfPTable(4);
//设置内部表格宽度为100%
innerTable.setWidthPercentage(100);
//划分表格每一列比例
innerTable.setWidths(new float[]{1f, 1.5f, 1.5f, 6f});
// 添加表头
PdfPCell cell;
//表头单元格背景色
BaseColor lightGray = new BaseColor(208, 223, 230);
//设置文本
cell = new PdfPCell(new Phrase("姓名", headFontChinese));
//设置背景色
cell.setBackgroundColor(lightGray);
//水平居中
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
//单元格最小高度
cell.setMinimumHeight(30);
//垂直居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
innerTable.addCell(cell);
cell = new PdfPCell(new Phrase("性别", headFontChinese));
cell.setBackgroundColor(lightGray);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
cell.setMinimumHeight(30);
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
innerTable.addCell(cell);
cell = new PdfPCell(new Phrase("年龄", headFontChinese));
cell.setBackgroundColor(lightGray);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
cell.setMinimumHeight(30);
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
innerTable.addCell(cell);
cell = new PdfPCell(new Phrase("学号", headFontChinese));
cell.setBackgroundColor(lightGray);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
cell.setMinimumHeight(30);
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
innerTable.addCell(cell);
// 添加数据就和添加表头差不多,只不过headFontChinese改为contentFontChinese,并且不要设置背景色,可以继续复用cell对象,并使用innerTable.addCell(cell);继续往后面添加单元格即可
// 将内部表格添加到外部表格的单元格中
PdfPCell tableCell = new PdfPCell(innerTable);
// 设置单元格无边框,否则内部表格会有两个边框
tableCell.setBorder(Rectangle.NO_BORDER);
//将含有内部表格的单元格设置进入外部表格中
table.addCell(tableCell);
//将外部表格添加到文档中
document.add(table);
效果:
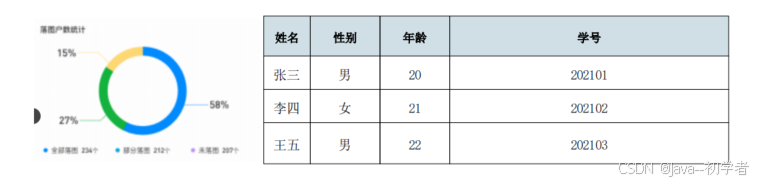
step6.插入最后两个表格
代码:
//添加间隔
document.add(new Paragraph(" "));
//设置小标题
Paragraph paragraph1 = new Paragraph("表格", smallTitleFontChinese);
//防止小标题和表格挨得太近,在小标题之后设置一些间距
paragraph1.setSpacingAfter(8f);
document.add(paragraph1);
//创建一个包含五列的表格
PdfPTable newTable = new PdfPTable(5);
//设置表格宽度为100%
newTable.setWidthPercentage(100);
//设置列宽比例
newTable.setWidths(new float[]{0.8f, 1f, 1f, 1f, 6.2f});
// 添加表头和添加内容的基本代码逻辑与innertable一致,不做赘述
// 将新表格添加到文档中
document.add(newTable);
//第二张表格和第一张表格代码一致,不做赘述
效果:
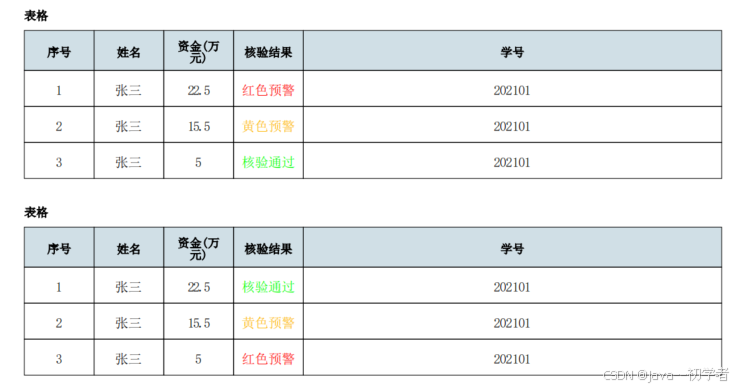
step7.将PDF数据流导出
//设置响应头和内容格式
response.setContentType("application/pdf");
response.setHeader(HttpHeaders.CONTENT_DISPOSITION, "attachment;filename=export.pdf");
//关闭文档
document.close();
//将PDF内容写入响应输出流并关闭
ServletOutputStream outputStream = response.getOutputStream();
outputStream.write(baos.toByteArray());
outputStream.flush();
outputStream.close();
tips.如何设置表格中字体颜色
//首先预设置几种颜色样式,并保持除颜色外,其它样式和其它内容一致
//红色
Font redFont = new Font(bfChinese, contentFontChinese.getSize(), contentFontChinese.getStyle(), BaseColor.RED);
//绿色
Font greenFont = new Font(bfChinese, contentFontChinese.getSize(), contentFontChinese.getStyle(), BaseColor.GREEN);
//黄色
Font yellowFont = new Font(bfChinese, contentFontChinese.getSize(), contentFontChinese.getStyle(), new BaseColor(254,186,7));
//根据实际情况将cell对象重新赋值
if(Condition 1){
cell = new PdfPCell(new Phrase("原文本", greenFont));
}
if(Condition 2){
cell = new PdfPCell(new Phrase("原文本", redFont ));
}
if(Condition 3){
cell = new PdfPCell(new Phrase("原文本", yellowFont));
}
//最后还是将cell设置进入table即可
table.addCell(cell);
4.最终效果
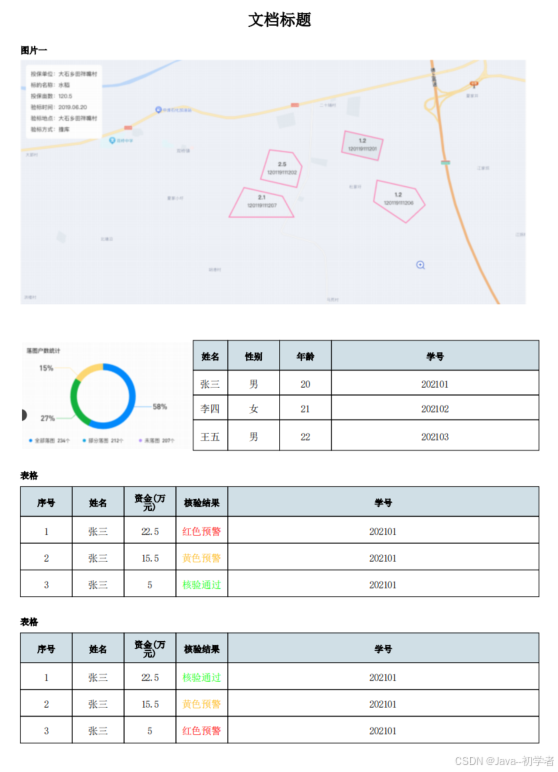