#include<iostream>
using namespace std;
#include<string>
#include<sstream>
class Solution
{
public:
int reverse(int x)
{
long result; //利用long避免溢出
if (x == INT_MIN)
{
return 0;
}
if (x < 0)
{
return -reverse(-x);
}
//主要用来进行数据类型转换,由于 使用 string 对象来代替字符数组(snprintf方式),
//就避免缓冲区溢出的危险;而且,因为传入参数和目标对象的类型会被自动推导出来,
//所以不存在错误的格式化符的问题。简单说,相比c库的数据类型转换而言, 更加安全、自动和直接。
stringstream ss;
ss << x;
string tmpStr;
ss >> tmpStr;
// 数字转换为字符串
int tmpStrSize = int(tmpStr.size());
string resultStr;
for (int index = tmpStrSize - 1; index >= 0; index--)
{
resultStr.push_back(tmpStr[index]);
}
ss.clear();
ss << resultStr;
ss >> result;
if (result > INT_MAX)
{
return 0;
}
return int(result);
}
};
int main()
{
Solution aaa;
int b = -1564;
aaa.reverse(b);
system("pause");
return 0;
}
//#include<iostream>
//using namespace std;
//#include<string>
//#include<sstream>
//
//class Solution
//{
//public:
// int reverse(int x)
// {
// long result(0);
// if (x == INT_MIN)
// {
// return 0;
// }
// if (x < 0)
// {
// return -reverse(-x);
// }
//
// int digit(0);
// while (x > 0)
// {
// digit = x % 10;
// x /= 10;
// result = result * 10 + digit;
// }
// if (result > INT_MAX)
// {
// return 0;
// }
// return int(result);
// }
//};
//
//int main()
//{
// Solution aaa;
// int b = -1564;
// aaa.reverse(b);
//
// system("pause");
// return 0;
//}
07 整数反转
最新推荐文章于 2024-10-31 16:16:13 发布
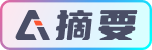