无名管道概述
管道(pipe)又称无名管道。无名管道是一种特殊类型的文件,在应用层体现为两个打开的文件描述符。
任何一个进程在创建的时候,系统都会给他分配4G的虚拟内存,分为3G的用户空间和1G的内核空间,内核空间是所有进程公有的,无名管道就是创建在内核空间的,多个进程知道同一个无名管道的空间,就可以利用他来进行通信
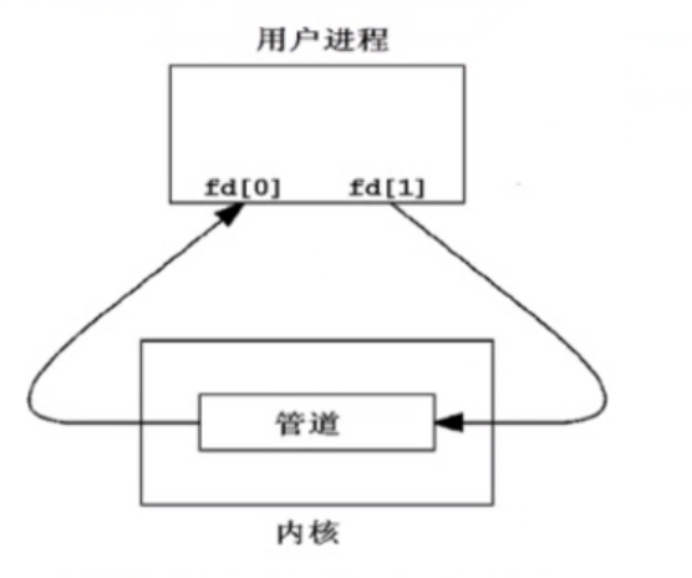
管道是最古老的UNIX IPC方式,其特点是:
1、半双工,数据在同一时刻只能在一个方向上流动。
2、数据只能从管道的一端写入,从另一端读出。
3、写入管道中的数据遵循先入先出的规则。
4、管道所传送的数据是无格式的,这要求管道的读出方与写入方必须事先约定好数据的格式,如多少字节算一个消息等。
5、管道不是普通的文件,不属于某个文件系统,其只存在于内存中。
6、管道在内存中对应一个缓冲区。不同的系统其大小不一定相同。
7、从管道读数据是一次性操作,数据一旦被读走,它就从管道中被抛弃,释放空间以便写更多的数据。
8、管道没有名字,只能在具有公共祖先的进程之间使用
无名管道的创建-- pipe函数
#include <unistd.h>
int pipe(int pipefd[2]);
功能:创建一个无名管道,返回两个文件描述符负责对管道进行读写操作
参数:
pipefd: int型数组的首地址,里面有两个元素
pipefd[0]负责对管道执行读操作
pipefd[1]负责对管道执行写操作
返回值:
成功:0
失败:-1
案例1:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
printf( "fd_pipe[0] = %d\n", fd_pipe[0]);
printf( "fd_pipe[1] = %d\n", fd_pipe[1]);
//由于无名管道给当前用户进程两个文件描述符,所以只要操作这两个文件
//描述符就可以操作无名管道,所以通过文件IO中的read和write函数对无名管道进行操作
//通过write函数向无名管道中写入数据
//fd_pipe[1]负责执行写操作
if(write(fd_pipe[1],"hello world",12)==-1)
{
perror("fail to write");
exit(1);
}
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
if(read(fd_pipe[0],buf,sizeof(buf))==-1)
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
return 0;
}
结果如下

案例2
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
printf( "fd_pipe[0] = %d\n", fd_pipe[0]);
printf( "fd_pipe[1] = %d\n", fd_pipe[1]);
//由于无名管道给当前用户进程两个文件描述符,所以只要操作这两个文件
//描述符就可以操作无名管道,所以通过文件IO中的read和write函数对无名管道进行操作
//通过write函数向无名管道中写入数据
//fd_pipe[1]负责执行写操作
if(write(fd_pipe[1],"hello world",12)==-1)
{
perror("fail to write");
exit(1);
}
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
ssize_t bytes;
if((bytes=read(fd_pipe[0],buf,sizeof(buf)))==-1)
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
return 0;
}
获取返回值

测试1:在写入数据后重新写入数据,会不会将之前的数据覆盖掉
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
printf( "fd_pipe[0] = %d\n", fd_pipe[0]);
printf( "fd_pipe[1] = %d\n", fd_pipe[1]);
//由于无名管道给当前用户进程两个文件描述符,所以只要操作这两个文件
//描述符就可以操作无名管道,所以通过文件IO中的read和write函数对无名管道进行操作
//通过write函数向无名管道中写入数据
//fd_pipe[1]负责执行写操作
if(write(fd_pipe[1],"hello world",11)==-1)
{
perror("fail to write");
exit(1);
}
write(fd_pipe[1],"nihao beijing",strlen("nihao beijing")+1);
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
ssize_t bytes;
if((bytes=read(fd_pipe[0],buf,sizeof(buf)))==-1)
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
return 0;
}
结果如下:并不会被覆盖掉

测试2:未读完的数据,不会消失,可继续打印出来
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
printf( "fd_pipe[0] = %d\n", fd_pipe[0]);
printf( "fd_pipe[1] = %d\n", fd_pipe[1]);
//由于无名管道给当前用户进程两个文件描述符,所以只要操作这两个文件
//描述符就可以操作无名管道,所以通过文件IO中的read和write函数对无名管道进行操作
//通过write函数向无名管道中写入数据
//fd_pipe[1]负责执行写操作
if(write(fd_pipe[1],"hello world",11)==-1)
{
perror("fail to write");
exit(1);
}
write(fd_pipe[1],"nihao beijing",strlen("nihao beijing")+1);
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
ssize_t bytes;
if((bytes=read(fd_pipe[0],buf,20))==-1)//只读取20个字节
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
bytes=read(fd_pipe[0],buf,sizeof(buf));
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
return 0;
}
结果如下:
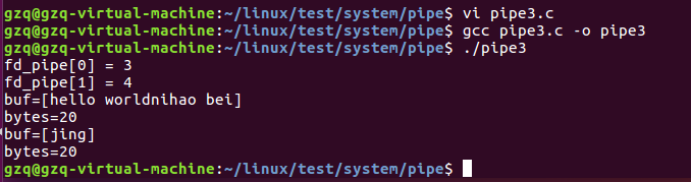
测试3:当管道内所有数据已读完,管道就会阻塞
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
printf( "fd_pipe[0] = %d\n", fd_pipe[0]);
printf( "fd_pipe[1] = %d\n", fd_pipe[1]);
//由于无名管道给当前用户进程两个文件描述符,所以只要操作这两个文件
//描述符就可以操作无名管道,所以通过文件IO中的read和write函数对无名管道进行操作
//通过write函数向无名管道中写入数据
//fd_pipe[1]负责执行写操作
if(write(fd_pipe[1],"hello world",11)==-1)
{
perror("fail to write");
exit(1);
}
write(fd_pipe[1],"nihao beijing",strlen("nihao beijing")+1);
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
ssize_t bytes;
if((bytes=read(fd_pipe[0],buf,20))==-1)//只读取20个字节
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
bytes=read(fd_pipe[0],buf,sizeof(buf));
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
bytes=read(fd_pipe[0],buf,sizeof(buf));
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
return 0;
}
结果如下:当管道中没有数据可读,就会进入阻塞
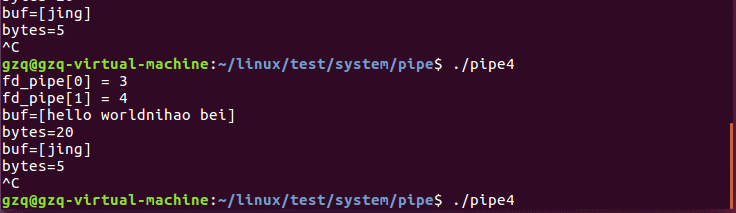
无名管道实现进程间通信
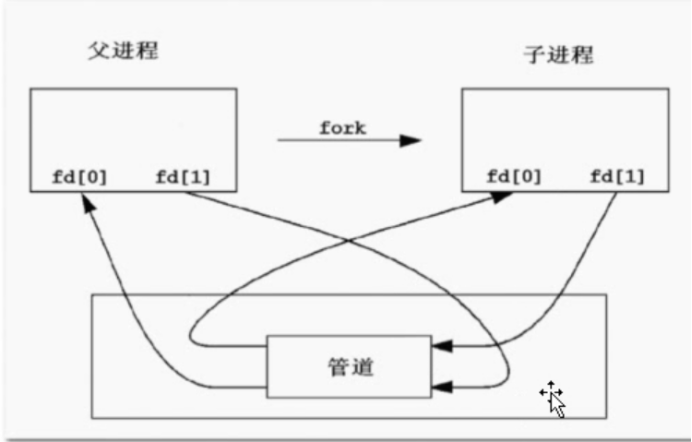
注意:
利用无名管道实现进程间的通信,都是父进程创建无名管道,然后再创建子进程,子进程继承父进程的无名管道的文件描述符,然后父子进程通过读写无名管道实现通信
适用范围小,用于亲缘关系的进程
案例:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
//使用无名管道实现父子进程间的通信
int main(int argc, char const *argv[])
{
int pipefd[2];
if(pipe(pipefd) == -1)
{
perror( "fail to pipe");
exit(1);
}
//使用fork函数创建子进程
pid_t pid;
if((pid = fork())<0)
{
perror( "fail to fork" );
exit(1);
}
else if(pid >0 )//父进程
{
//父进程负责给子进程发送数据
char buf[128] = {};
while(1)
{
fgets(buf,sizeof(buf),stdin);
buf[strlen(buf) - 1] = '\0';
if(write(pipefd[1], buf, sizeof(buf)) == -1){
perror("fail to write");
exit(1);
}
}
}
else //子进程
{
//子进程接收父进程的数据
char buf[128] = "";
while(1)
{
if(read(pipefd[0],buf, sizeof(buf)) == -1){
perror( "fail to read" );
exit(1);
}
printf( "from parent: %s \n", buf);
}
}
return 0;
}
注意:创建无名管道一定要放在fork之前,如果放在fork之后,父进程里边创建了无名管道,子进程是获取不到的,如果父子进程都创建了无名管道,他两是没有任何关系的。放在fork之前可以获取父进程fork之前所有的代码,所以可以保证父子进程所获取的无名管道的文件描述符对应在内核空间所开辟的空间是同一个,所以才能进行通信。
运行结果如下:
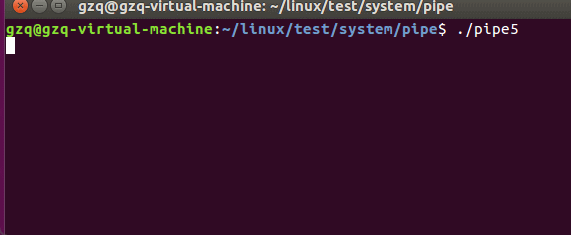
总结:
由于无名管道创建之后给当前进程两个文件描述符,所以如果是完全不相关的进程
无法获取同一个无名管道的文件描述符,所以无名管道只能在具有亲缘关系的进程间通信
从管道中读数据的特点
1、默认用read函数从管道中读数据是阻塞的。
2、调用write函数向管道里写数据,当缓冲早已满时write也会阻塞。
3、通信过程中,读端口全部关闭后,写进程向管道内写数据时,写进程会(收到SIGPIPE信号)退出。
无名管道读写规律
1、读写端都存在,只读不写会出现什么情况
实验如下:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[])
{
int pipefd[2];
if(pipe(pipefd) == -1)
{
perror( "fail to pipe");
exit(1);
}
write(pipefd[1],"hello world",11);
char buf[128] = "";
if(read(pipefd[0],buf,sizeof(buf)) == -1){
perror( "fail to read" );
exit(1);
}
printf( "from parent: %s \n", buf);
if(read(pipefd[0],buf,sizeof(buf)) == -1){
perror( "fail to read" );
exit(1);
}
printf( "from parent: %s \n", buf);
return 0;
}
运行结果:
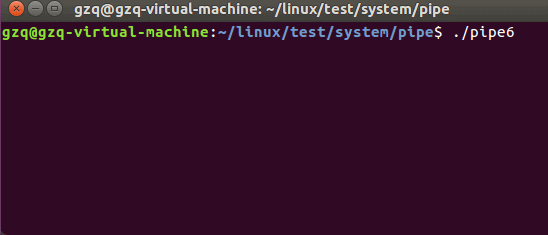
总结:
如果管道中有数据,会正常读取数据
如果管道中没有数据,则读操作会阻塞等待,直到有数据为止
2、读写端都存在,只写不读会出现什么情况
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[])
{
int pipefd[2];
//创建无名管道
if(pipe(pipefd) == -1)
{
perror( "fail to pipe");
exit(1);
}
int num = 0;
while(1){
//一次写1024个字节即1k
if(write(pipefd[1],"6666",1024)== -1){
perror("fail to write");
exit(1);
}
num++;
printf( "num = %d\n", num);
}
return0;
}
运行结果:
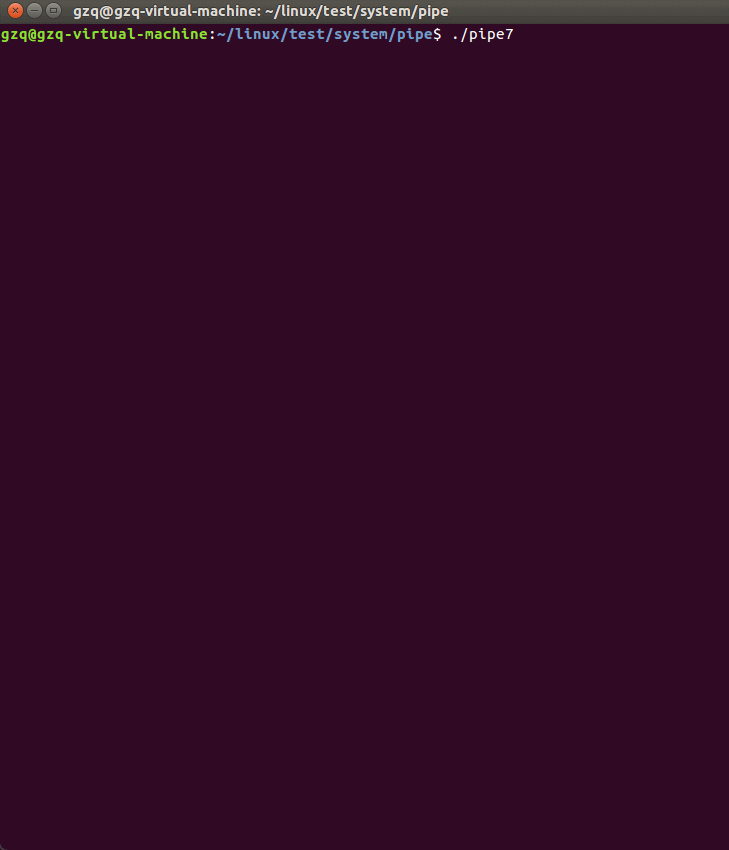
总结:
如果一直执行写操作,则无名管道对应的缓冲区会被写满,写满之后,write函数也会阻塞等待。
默认无名管道的缓冲区64K字节。
3、关闭写文件描述符,只有读端
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[]){
//使用pipe创建一个无名管道
int fd_pipe[2];
if(pipe(fd_pipe)== -1){
perror( "fail to pipe");
exit(1);
}
write(fd_pipe[1],"hello world",11);
close(fd_pipe[1]);
//通过read函数从无名管道中读取数据//fd_pipe[0]负责执行读操作
char buf[32]="";//创建buf变量,将从无名管道读到的数据存入到buf中
ssize_t bytes;
if((bytes=read(fd_pipe[0],buf,sizeof(buf)))==-1)//只读取20个字节
{
perror("fail to read");
exit(1);
}
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
memset(buf,0,sizeof(buf));
bytes=read(fd_pipe[0],buf,sizeof(buf));
printf("buf=[%s]\n",buf);
printf("bytes=%ld\n",bytes);
return 0;
}
运行结果:
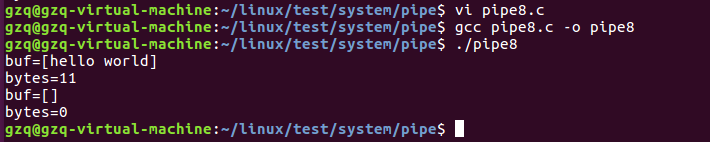
总结:
如果原本管道中有数据,则读操作正常读取数据
如果管道中没有数据,则read函数会返回0
4、关闭写操作文件描述符,只有写端
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char const *argv[])
{
int pipefd[2];
//创建无名管道
if(pipe(pipefd) == -1)
{
perror( "fail to pipe");
exit(1);
}
close(pipefd[0]);
int num = 0;
while(1){
//一次写1024个字节即1k
if(write(pipefd[1],"6666",1024)== -1){
perror("fail to write");
exit(1);
}
num++;
printf( "num = %d\n", num);
}
return0;
}
运行结果:什么都没发生

如果关闭读端,一旦执行写操作,就会产生一个信号SIGPIPE(管道破裂)
这个信号的默认处理方式是退出进程
通过自定义信号函数来验证管道破裂的现象
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <signal.h>
void handler(int sig)
{
printf("SIGPIPE信号产生了,管道破裂了");
}
int main(int argc, char const *argv[])
{
signal(SIGPIPE,handler);
int pipefd[2];
//创建无名管道
if(pipe(pipefd) == -1)
{
perror( "fail to pipe");
exit(1);
}
close(pipefd[0]);
int num = 0;
while(1){
//一次写1024个字节即1k
if(write(pipefd[1],"6666",1024)== -1){
perror("fail to write");
exit(1);
}
num++;
printf( "num = %d\n", num);
}
return 0;
}
运行结果:

编程时可通过fcntl函数设置文件的阻塞特性。如无名管道中读写端都存在,只读不写会出现阻塞的情况就可以通过fcntl函数来设置。
设置为阻塞:
fcntl(fd, F_SETFL,0);
设置为非阻塞:
fcntl(fd, F_SETFL,O_NONBLOCK);
案例:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/wait.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
int fd_pipe[2];
char buf[] = "hello world";
pid_t pid;
if (pipe(fd_pipe)<0){
perror( "fail to pipe");
exit(1);
}
pid = fork();
if (pid < 0)
{
perror( "fail to fork" );
exit(0);
}
if (pid == 0){
while(1){
sleep(5);
write(fd_pipe[1],buf,strlen(buf));
}
}
else{
while(1)
{
memset(buf,0,sizeof(buf));
read(fd_pipe[0],buf,sizeof(buf));
printf( "buf=[%s]\n", buf);
sleep(1);
}
}
return 0;
}
运行结果:每隔五秒写入一次
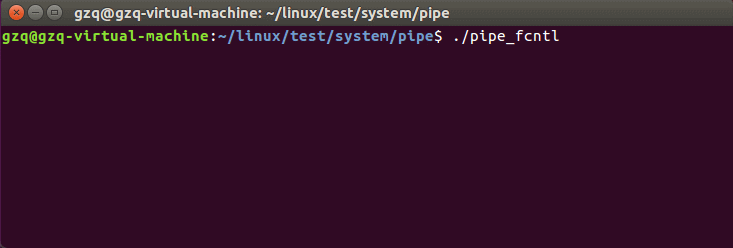
案例2:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/wait.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
int fd_pipe[2];
char buf[] = "hello world";
pid_t pid;
if (pipe(fd_pipe)<0){
perror( "fail to pipe");
exit(1);
}
pid = fork();
if (pid < 0)
{
perror( "fail to fork" );
exit(0);
}
if (pid == 0){
while(1){
sleep(5);
write(fd_pipe[1],buf,strlen(buf));
}
}
else{
//将fd_pipe[0]设置为非阻塞
fcntl(fd_pipe[0],F_SETFL,O_NONBLOCK);
while(1)
{
memset(buf,0,sizeof(buf));
read(fd_pipe[0],buf,sizeof(buf));
printf( "buf=[%s]\n", buf);
sleep(1);
}
}
return 0;
}
运行结果:
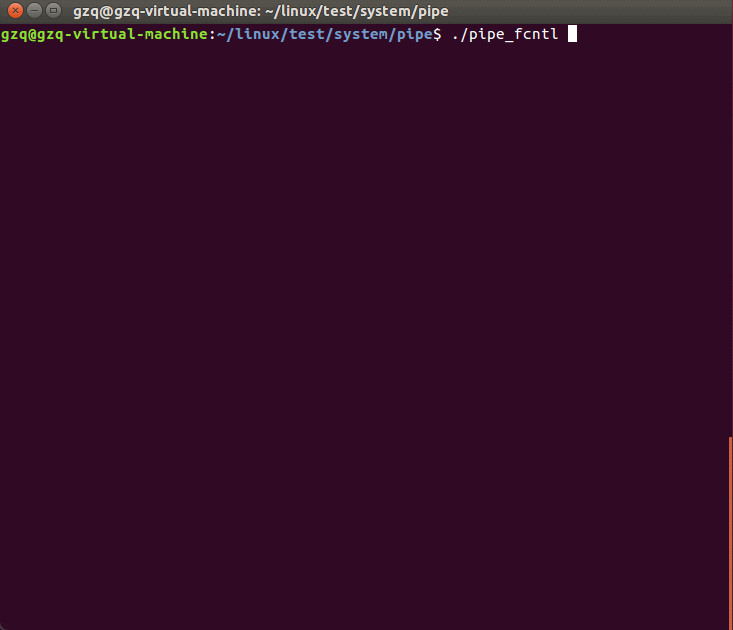
总结:
如果是阻塞,管道中没有数据,read会一直等待,直到有数据才会继续运行,否则一直等待
如果是非阻塞,read函数运行时,会先看一下管道中是否有数据,如果有数据,则正常运行读取数据,如果管道中没有数据,则read函数会立即返回,继续下面的代码运行