ajax的异步调用
jQuery实现数据获取
业务说明
1).用户通过http://localhost:8090/userAjax 要求跳转到userAjax.html中
2).通过ajax请求向后端服务器获取数据,并且在页面中展现数据.
jQuery下载(官方网站):https://jquery.com/
Ajax介绍
Ajax特点
局部刷新-异步访问
同步: 当用户发起请求时,如果这时服务器正忙,那么用户处于等待的状态,同时不可以做其他的操作.
异步: 当用户发起请求时,如果遇到服务器正忙,这时用户可以继续执行后续的操作.同时通过**回调函数(钩子函数)**与服务器沟通.
为什么ajax可以一步调用
Ajax异步原理
1).常规同步的调用方式
Ajax异步调用
说明: Ajax通过Ajax引擎实现异步的调用.当后台的服务器响应数据之后,通过预先设置好的回调函数进行数据的展现.
编辑ajaxList.html页面
官网下载jquery文件
<!DOCTYPE html>
<!--导入模板标签!!!!!-->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>用户列表数据</title>
</head>
<body>
<!--准备一个表格-->
<table border="1px" align="center" width="800px">
<tr align="center">
<td colspan="4"><h3>用户列表</h3></td>
</tr>
<tr align="center">
<td>ID</td>
<td>名称</td>
<td>年龄</td>
<td>住址</td>
</tr>
</table>
</body>
</html>
编辑controller代码
package com.jt.controller;
import com.jt.pojo.User;
import com.jt.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
@Controller
public class UserController {
@Autowired
private UserService userService;
/**
* 要求跳转到userAjax页面中
* @return
*/
@RequestMapping("/userAjax")
public String userAjax(){
return "userAjax";
}
/**
* 接收ajax请求 /findAjaxUser 查询所有的用户信息
* 返回值结果: JSON字符串
* @return
*/
@RequestMapping("/findAjaxUser")
@ResponseBody
public List<User> findAjaxUser(){
return userService.findAll();
}
关于JS 循环遍历的写法
1.常规for循环
for(var i=0;i<result.length;i++){
var user = result[i];
console.log(user)
}
2.使用in的关键字
//关键字 in index 代表遍历的下标
for(index in result){
var user = result[index]
console.log(user)
}
3.使用of关键字
for(user of result){
console.log(user)
}
编辑整体的html代码(通过使用js编写的)
<!DOCTYPE html>
<!--导入模板标签!!!!!-->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>用户列表数据</title>
<!--
1.引入函数类库
2.使用模板工具类中的静态资源文件默认的路径都在static目录下
-->
<script src="/jquery-3.6.0.min.js"></script>
<script>
//1.jQuery中有一种编程思想 函数式编程
$(function(){
//$此处的$表示jquery
//让整个页面加载完成之后再执行. 以后所有的操作都应该在函数内完成!!!!
//异步提交多数都是json串
/**
* 常见Ajax用法,
* 1.$.get(url地址,提交数据,回调函数,返回值类型)
* function(result){ }回调函数
* 如果不需要相应的参数则可以不写
* 2.$.post(...)
*
* for循环的写法
1.常规for循环
for(var i=0;i<result.length;i++){
var user = result[i];
console.log(user)
}
2.使用in的关键字
//关键字 in index 代表遍历的下标
for(index in result){
var user = result[index]
console.log(user)
}
*
3.使用of关键字
for(user of result){
console.log(user)
}
* 业务需求:
* 要求向后端服务器发起请求 /findAjaxUser,之后将返回值结果 进行页面解析
*/
$.get("/findAjaxUser",function(result){
//1.result是服务器返回的结果 [{},{},{}....}]
//2.将返回值结果进行循环遍历
for(user of result){
//3.获取对象的属性值
var id = user.id
var name = user.name
var age = user.age
var sex = user.sex
//将结果一行一行的展现在页面中的form表单中
var tr = "<tr align='center'><td>"+ id +"</td><td>"+name+"</td><td>"+age+"</td><td>"+sex+"</td></tr>"
//4.选中id=userTable的元素
//5.之后追加数据append(追加的内容)
$("#userTable").append(tr)
}
})
/**
* 参数说明:
* 1.字符串拼接
* 语法:key=value&key2=value2....
* 例如: id=1&name=tom
*
* 2.js对象的写法
* 语法: {key:value,key2:value2.....}
* 例如: {id:1,name='tom'}
*/
/* 带参数的ajax请求
$.get("/findAjaxUser",'id=1&name=tom',function(result){
console.log("ajax请求成功!!!")
}) */
console.log("对象结构的语法")
$.get("/findAjaxUser",{id:1,name:'tomcat'},function(result){
console.log("ajax Get请求成功!!!")
})
$.post('findAjaxUser',{id:1,name:'tomcat'},function(result){
console.log("post请求执行成功")
})
//要求返回值都是json字符串.
$.getJSON('findAjaxUser',{id:1,name:'tomcat'},function(resuslt){
console.log("getJSON的请求数据!!!")
})
//最为基本的ajax
$.ajax({
type: "put", //请求的类型
url: 'findAjaxUser', //请求的路径
data: {id:1,name:'tomcat猫'}, //请求的参数
//成功之后的回调函数
success: function(result){ //成功回调
console.log("ajax请求成功!!!!")
},
error: function(result){ //失败回调
console.log("ajax请求失败")
}
})
})
</script>
</head>
<body>
<h1>修改数据</h1>
<div>
<p>编号:</p>
<p>姓名:</p>
<p>年龄:</p>
<p>性别:</p>
<button>更新</button>
</div>
<hr />
<!-- -->
<table id="userTable" border="1px" align="center" width="800px">
<tr align="center">
<td colspan="4"><h3>用户列表</h3></td>
</tr>
<tr align="center">
<td>ID</td>
<td>名称</td>
<td>年龄</td>
<td>性别</td>
<td>操作</td>
</tr>
<tr align="center">
<td>1</td>
<td>测试</td>
<td>18</td>
<td>男</td>
<td>
<button>修改</button>
<button>删除</button>
</td>
</tr>
</table>
</body>
</html>
页面效果调用
关于常见Ajax种类介绍
带参数的请求
1).字符串拼接
/**
* 参数说明:
* 1.key=value&key2=value2....
* 例如: id=1&name=tom
*
*/
$.get("/findAjaxUser",'id=1&name=tom',function(result){
console.log("ajax请求成功!!!")
})
在ajax里面没有put,delete请求
.js对象的写法
语法: {key:value,key2:value2.....}
例如: {id:1,name='tom'}
$.get('findAjaxUser',{id:1,name:'tomcat'},function(resuslt){
console.log("getJSON的请求数据!!!")
})
当参数有很多个的时候可以使用下面进行封装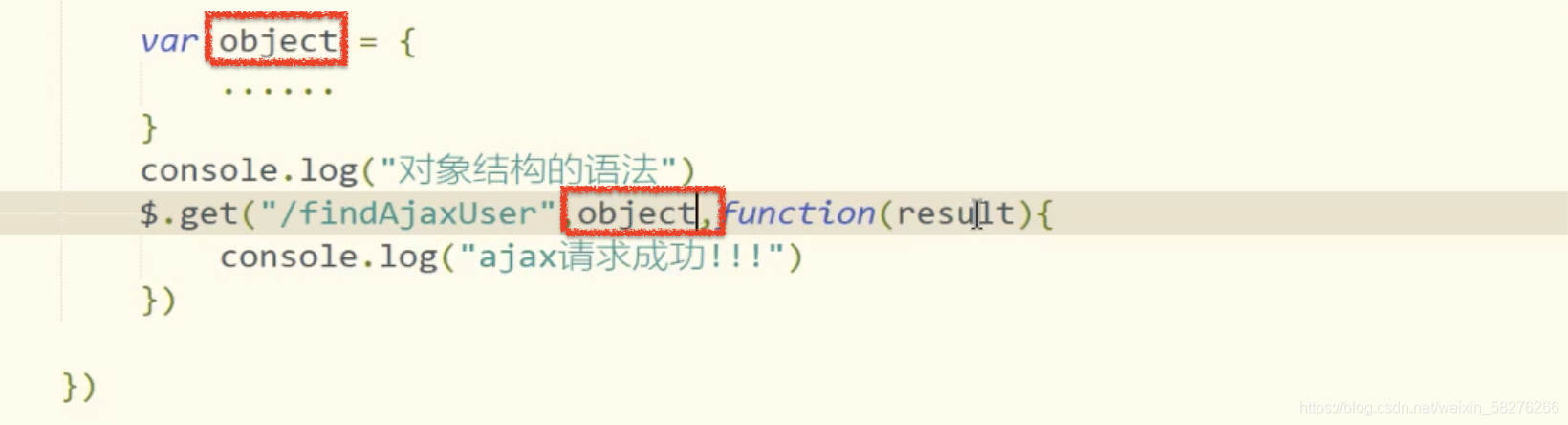
post请求结构
$.post('findAjaxUser',{id:1,name:'tomcat'},function(result){
console.log("post请求执行成功")
})
getJSON类型
//要求返回值都是json字符串.
$.getJSON('findAjaxUser',{id:1,name:'tomcat'},function(resuslt){
console.log("getJSON的请求数据!!!")
})
. g e t J S O N 和 .getJSON和 .getJSON和.get的区别
$.getJSON返回的请求类型只能是string类型
$.get返回的请求类型可以是任何一种
$.ajax类型
//最为基本的ajax
$.ajax({
type: "put", //请求的类型 get post put delete
url: 'findAjaxUser', //请求的路径
data: {id:1,name:'tomcat猫'}, //请求的参数
//成功之后的回调函数
success: function(result){ //成功回调
console.log("ajax请求成功!!!!")
},
error: function(result){ //失败回调
console.log("ajax请求失败")
}
})
})
前端的知识架构
js jquery ajax vue
web >=前端。 前端只是一个静态页面,而web不仅有静态页面海包括一个服务器;
html是一个静态页面 , js是一个动态的页面,css是一个样式(美化页面);
请求类型说明
get请求
1.会将参数通过?号的形式进行拼接. http://localhost:8090/findUser?id=1&password=123456
2.get请求会将所有的参数动态的拼接到URL中,相对不安全.
3.Get请求不适用于大量的数据提交 各大的浏览器对于Get请求一般都是有上限的.
总结:
1.查询数据时.
2.获取简单数据时使用(页面/JS/CSS…)
3.一般请求中get请求居多.
POST
1.POST请求将所有的参数都会进行form的封装.
2.如果需要传递海量的数据,则首选POST
3.POST的请求使用form进行封装,相对于GET请求更加的安全.
总结:
1.提交海量的数据时使用.
2.一般提交文件(视频,音频等文件)时使用
3.提交
VUE
2.1 VUE介绍
Vue (读音 /vjuː/,类似于 view) 是一套用于构建用户界面的渐进式(灵活)框架。与其它大型框架不同的是,Vue 被设计为可以自底向上逐层应用。Vue 的核心库只关注视图层,不仅易于上手,还便于与第三方库或既有项目整合。另一方面,当与现代化的工具链以及各种支持类库结合使用时,Vue 也完全能够为复杂的单页应用提供驱动。
2.2 VUE入门案例
2.2.1 导入JS
说明: 将课前资料中的js文件导入IDEA中 如图所示
VUE入门案例
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>VUE入门案例</title>
</head>
<body>
<div id="app">
<!-- 插值表达式 内容 data中对象的属性 -->
<h1>{{hello}}</h1>
</div>
<!-- 1.导入vue.js的类库 -->
<script src="../js/vue.js"></script>
<!-- 2.编辑VUE js -->
<script>
//新规定: 1.结尾的;号可以省略 2.字符一般使用'单引号'
//补充知识: var: js中声明变量的修饰符 没有作用域的概念
// 1.const 定义常量的
// 2.let 有作用域的变量声明.
//1.实例化VUE对象 函数式编程
const app = new Vue({
//1.定义el元素 要在哪个位置使用vue进行渲染
el: "#app",
//2.定义数据对象
data: {
hello: 'VUE入门案例!!!!'
}
})
</script>
</body>
</html>
编辑axiosusercontroller实现用户的删除
package com.jt.controller;
import com.jt.pojo.User;
import com.jt.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
//使用VUE的方式 实现用户的CURD操作
@RestController
@CrossOrigin //默认值为*号 允许所有的网站访问
//@CrossOrigin("http://www.jt.com")
public class AxiosUserController {
@Autowired
private UserService userService;
/**
* Axios 案例
* 1.查询数据库用户列表数据
* 2.url: /axiosUser/findAll
* 3.返回值结果: List<User>
*/
@GetMapping("/axiosUser/findAll")
public List<User> findAll(){
return userService.findAll();
}
/**
* 实现根据更新操作
* URL地址: http://localhost:8090/axiosUser/updateUser
* 参数: user对象的JSON串
* 返回值: 不要求 void
*/
@PutMapping("/axiosUser/updateUser")
public void updateUser(@RequestBody User user){
userService.updateUser(user);
}
/**
* 业务需求: 删除用户信息
* URL: http://localhost:8090/axiosUser/deleteUser?id=1
* 参数: id=1
* 返回值: void
*/
@DeleteMapping("/axiosUser/deleteUser")
public void deleteUser(Integer id){
userService.deleteUserById(id);
}
}
编辑axioscontroller(axios的post form对象的写法)
package com.jt.controller;
import com.jt.pojo.AxiosPOJO;
import com.sun.media.sound.AiffFileReader;
import org.springframework.web.bind.annotation.*;
//在后端接收前端axios发来的请求
@RestController
@CrossOrigin //允许当前类中的所有方法执行跨域操作!!!
public class AxiosController {
/**
* Axios入门案例
* url: http://localhost:8090/hello
*/
@GetMapping("/hello")
public String hello(){
return "VUE的ajax异步调用";
}
/**
* 1.接收get请求的Id参数
* http://localhost:8090/axios?id=100
*/
@GetMapping("/axios")
public String getAxios(Integer id){
return "动态获取数据:"+id;
}
/**
* restFul接收参数
* http://localhost:8090/axios/100
*/
@GetMapping("/axios/{id}")
public String axiosRestFul(@PathVariable Integer id){
return "restFul接收参数:"+id;
}
/**
* 测试params对象数据传参
* /axiosParams
*/
@GetMapping("/axiosParams")
public String params(Integer id){
return "params获取参数成功!!!";
}
/**
* 动态接收post请求 并且接收json参数
* url地址: /addAxios
* 语法:
* 如果前端传递的是一个JSON的字符串.
* 则使用注解 @RequestBody 将json串转化为对象
*/
@PostMapping("/addAxios")
public AxiosPOJO addAxios(@RequestBody AxiosPOJO axiosPOJO){
return axiosPOJO;
}
//利用Form表单接收数据
//id: 100 name: tomcat猫 可以使用对象接收参数
@PostMapping("/addAxiosForm")
public AxiosPOJO addAxiosForm(AxiosPOJO axiosPOJO){
return axiosPOJO;
}
}