一、类的6个默认成员函数:
class Date {};
二.构造函数:
2.1 概念:
class Date
{
public:
void Init(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "-" << _month << "-" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
d1.Init(2022, 7, 5);
d1.Print();
Date d2;
d2.Init(2022, 7, 6);
d2.Print();
return 0;
}
构造函数 是一个 特殊的成员函数,名字与类名相同 , 创建类类型对象时由编译器自动调用 ,以保证每个数据成员都有 一个合适的初始值,并且在对象整个生命周期内只调用一次 。
2.2 特性:
其特征如下:
1. 函数名与类名相同。2. 无返回值。3. 对象实例化时编译器 自动调用 对应的构造函数。4. 构造函数可以重载。class Data { public: //构造函数 //Data() //{ // _year = 2023; // _month = 5; // _day = 7; //} Data(int year = 2023, int month = 5, int day = 7) { _year = year; _month = month; _day = day; } void Printf() { cout << _year << "年" << _month << "月" << _day << "日" << endl; } private: int _year; int _month; int _day; }; int main() { Data d1; Data d2(2023, 5, 1); d1.Printf(); d2.Printf(); return 0; }
5. 如果类中没有显式定义构造函数,则 C++ 编译器会自动生成一个无参的默认构造函数,一旦用户显式定义编译器将不再生成。class Data { public: //构造函数 //Data() //{ // _year = 2023; // _month = 5; // _day = 7; //} //Data(int year = 2023, int month = 5, int day = 7) //{ // _year = year; // _month = month; // _day = day; //} void Printf() { cout << _year << "年" << _month << "月" << _day << "日" << endl; } private: int _year; int _month; int _day; }; int main() { Data d1; d1.Printf(); return 0; }
6. 关于编译器生成的默认成员函数,很多童鞋会有疑惑:不实现构造函数的情况下,编译器会 生成默认的构造函数。但是看起来默认构造函数又没什么用?d 对象调用了编译器生成的默 认构造函数,但是d 对象 _year/_month/_day ,依旧是随机值。也就说在这里 编译器生成的默认构造函数并没有什么用??解答: C++ 把类型分成内置类型 ( 基本类型 ) 和自定义类型。内置类型就是语言提供的数据类型,如: int/char... ,自定义类型就是我们使用 class/struct/union 等自己定义的类型,看看下面的程序,就会发现编译器生成默认的构造函数会对自定类型成员 _t 调用的它的默认成员函数。7. 无参的构造函数和全缺省的构造函数都称为默认构造函数,并且默认构造函数只能有一个。 注意:无参构造函数、全缺省构造函数、我们没写编译器默认生成的构造函数,都可以认为 是默认构造函数。
如上图类中有两个构造函数,分别是无参构造和全缺省构造,可以看到报错"类中包含多个默认构造函数",默认的构造函数只能有一个。
看以上这种情况,我们知道,当我们不手动实现构造函数时,编译器会默认生成构造函数来帮助我们初始化,但观察以上的代码,经过初始化后居然是随机值,为什么是这种情况??
其实,这是C++在设计初的一个缺陷。
C++默认生成的构造函数,对自定义类型成员调用它的默认构造函数,而对内置类型成员不作处理。因此在初始化内置类型成员时的构造函数需要我们自己写。看如下代码。
class A
{
public:
A()
{
_a = 0;
cout << "A()构造函数" << endl;
}
private:
int _a;
};
class Date
{
public:
//Date(int year = 1, int month = 1, int day = 1)
//{
// _year = year;
// _month = month;
// _day = day;
//}
void Print()
{
cout << _year << "--" << _month << "--" << _day << endl;
}
private:
//内置类型
int _year;
int _month;
int _day;
//自定义类型
A _aa;
};
int main()
{
Date d1;
d1.Print();
return 0;
}
可以看出编译器对自定义类型成员_a初始化为0,而对内置类型成员没做处理。
那么如果我的自定义类型成员中存在内置类型的成员,该如何初始化?
class Stack
{
public:
Stack(int capacity = 4)
{
cout << "构造函数" << endl;
_a = (int*)malloc(sizeof(int) * capacity);
if (_a == nullptr)
{
perror("malloc fail");
exit(-1);
}
_top = 0;
_capacity = capacity;
}
~Stack()
{
cout << "析构函数" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
int* _a;
int _capacity;
int _top;
};
class MyQueue
{
public:
void Push()
{
//
}
Stack _pushST;
Stack _popSt;
int _size = 0;
};
int main()
{
MyQueue q;
return 0;
}
在后续的使用中,C++大佬们发现这个缺陷并加补丁进行了完善。
- C++11 中针对内置类型成员不初始化的缺陷,又打了补丁,即:内置类型成员变量在类中声明时可以给默认值。
如以上代码,在类MyQueue中的_size在声明时,并不是赋值,而是给的缺省值,这样就解决了编译器对内置类型成员不做处理的这一缺陷。
我们深入看一下初始化前后的变化:
未初始化:
初始化:
三.析构函数:
3.1 概念:
通过前面构造函数的学习,我们知道一个对象是怎么来的,那一个对象又是怎么没呢的?析构函数:与构造函数功能相反,析构函数不是完成对对象本身的销毁,局部对象销毁工作是由 编译器完成的。而对象在销毁时会自动调用析构函数,完成对象中资源的清理工作 。
3.2 特性:
1. 析构函数名是在类名前加上字符 ~ 。2. 无参数无返回值类型。3. 一个类只能有一个析构函数。若未显式定义,系统会自动生成默认的析构函数。注意:析构函数不能重载4. 对象生命周期结束时, C++ 编译系统系统自动调用析构函数5. 关于编译器自动生成的析构函数,是否会完成一些事情呢?下面的程序我们会看到,编译器生成的默认析构函数,对自定类型成员调用它的析构函数。6. 如果类中没有申请资源时,析构函数可以不写,直接使用编译器生成的默认析构函数,比如 Date类;有资源申请时,一定要写,否则会造成资源泄漏,比如 Stack 类。
3.3 实例:
class Stack
{
public:
Stack(int capacity = 4)
{
cout << "构造函数" << endl;
_a = (int*)malloc(sizeof(int) * capacity);
if (_a == nullptr)
{
perror("malloc fail");
exit(-1);
}
_top = 0;
_capacity = capacity;
}
~Stack()
{
cout << "析构函数" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
int* _a;
int _capacity;
int _top;
};
int main()
{
Stack st;
return 0;
}
对以上代码进行调试,我们可以感受到析构函数,当程序调试到return,我们按F11,可以发现程序跳到~Stack执行析构函数。
与构造函数一样,我们不自己写的话,编译器也会默认生成,其对自定义类型的成员会自动调用其析构函数释放空间,但对内置类型成员不做处理,也就是上述的_a需要我们自己写析构函数来释放其空间。
class Time
{
public:
~Time()
{
cout << "~Time()" << endl;
}
private:
int _hour;
int _minute;
int _second;
};
class Date
{
private:
// 基本类型(内置类型)
int _year = 1970;
int _month = 1;
int _day = 1;
// 自定义类型
Time _t;
};
int main()
{
Date d;
return 0;
}
在main方法中根本没有直接创建Time类的对象,为什么最后会调用Time类的析构函数?
因为:main方法中创建了Date对象d,而d中包含4个成员变量,其中_year, _month,_day三个是内置类型成员,销毁时不需要资源清理,最后系统直接将其内存回收即可;而_t是Time类对象,所以在 d销毁时,要将其内部包含的Time类的_t对象销毁,所以要调用Time类的析构函数。但是:main函数中不能直接调用Time类的析构函数,实际要释放的是Date类对象,所以编译器会调用Date类的析构函数,而Date没有显式提供,则编译器会给Date类生成一个默认的析构函数,目的是在其内部调用Time类的析构函数,即当Date对象销毁时,要保证其内销部每个自定义对象都可以正确毁main函数中并没有直接调用Time类析构函数,而是显式调用编译器为Date类生成的默认析构函数。
注意:创建哪个类的对象则调用该类的析构函数,销毁那个类的对象则调用该类的析构函数。
总结来说:如果类中没有申请资源时,析构函数可以不写,直接使用编译器生成的默认析构函数,比如Date类;有资源申请时,一定要写,否则会造成资源泄漏,比如Stack类。
四.拷贝构造函数:
4.1 概念:
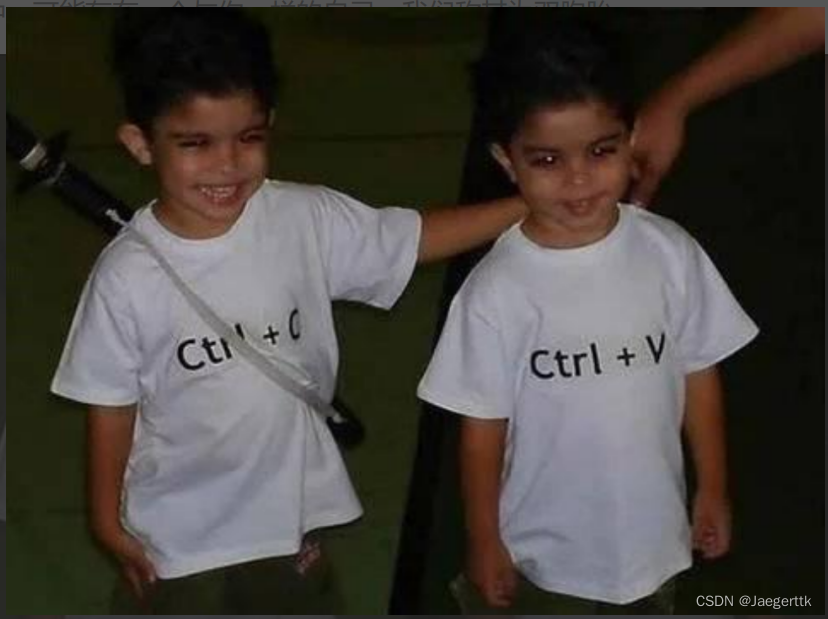
class Data
{
public:
//构造函数
//Data()
//{
// _year = 2023;
// _month = 5;
// _day = 7;
//}
Data(int year = 2023, int month = 5, int day = 7)
{
_year = year;
_month = month;
_day = day;
}
//拷贝构造
Data(Data& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
void Printf()
{
cout << _year << "年" << _month << "月" << _day << "日" << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Data d1;
Data d2(d1);
d1.Printf();
d2.Printf();
return 0;
}
4.2 特征:
1. 拷贝构造函数 是构造函数的一个重载形式 。2. 拷贝构造函数的 参数只有一个 且 必须是类类型对象的引用 ,使用 传值方式编译器直接报错, 因为会引发无穷递归调用
那么为什么会发生无穷的递归?我们看一下。
首先我们先来了解一下传值传参与传引用传参:
//传值传参
void func1(Data d)
{
cout << "func1" << endl;
}
//传引用传参
void func2(Data& d)
{
cout << "func2" << endl;
}
int main()
{
Data d1;
func1(d1);
func2(d1);
return 0;
}
通过运行结果可以看到传值传参func1调用了拷贝构造,func1在传参时调用了拷贝构造,我们知道传参时我们传的参数实际上是变量的临时拷贝,当我们在main函数中调用func1时,需要传d1,进入函数func1时,d会对d1进行拷贝,这时就调用了拷贝构造,而func2是传引用传参,即传入函数中的是d1的别名,就不需要再进行拷贝,也不会调用拷贝构造了。
回到原问题,为什么拷贝构造不能使用传值传参?
这是因为,编译器规定在传参时,对内置类型编译器可以直接拷贝,而自定义类型则需要调用拷贝构造。也就是说我们在实现拷贝构造时,使用传值传参时,编译器会自动去调用拷贝构造,而调用拷贝构造又需要传值传参,传值传参时又要进行拷贝调用拷贝构造,进而形成无穷的递归。
既然传值传参不可以,那我们延续C语言中的传址传参呢?
答案显而易见是可以的,那传址传参传的是参数的地址,传入函数中不是也需要拷贝吗?那为什么这样不会出现无限递归?因为不管是什么类型的指针,对于编译器来说都是属于指针类型,属于内置类型,编译器会对其直接拷贝,不需要调用拷贝构造,也就不会出现无穷递归的情况。
3. 若未显式定义,编译器会生成默认的拷贝构造函数。 默认的拷贝构造函数对象按内存存储按字节序完成拷贝,这种拷贝叫做浅拷贝,或者值拷贝。
class Data
{
public:
//构造函数
//Data()
//{
// _year = 2023;
// _month = 5;
// _day = 7;
//}
Data(int year = 2023, int month = 5, int day = 7)
{
_year = year;
_month = month;
_day = day;
}
//拷贝构造 - 浅拷贝(直接按字节拷贝)
Data(Data& d)
{
cout << "拷贝构造" << endl;
_year = d._year;
_month = d._month;
_day = d._day;
}
void Printf()
{
cout << _year << "年" << _month << "月" << _day << "日" << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Data d1;
Data d2(d1);
d1.Printf();
d2.Printf();
return 0;
}
typedef int DataType;
class Stack
{
public:
Stack(size_t capacity = 10)
{
_array = (DataType*)malloc(capacity * sizeof(DataType));
if (nullptr == _array)
{
perror("malloc申请空间失败");
return;
}
_size = 0;
_capacity = capacity;
}
void Push(const DataType& data)
{
// CheckCapacity();
_array[_size] = data;
_size++;
}
~Stack()
{
if (_array)
{
free(_array);
_array = nullptr;
_capacity = 0;
_size = 0;
}
}
private:
DataType *_array;
size_t _size;
size_t _capacity;
};
int main()
{
Stack s1;
s1.Push(1);
s1.Push(2);
s1.Push(3);
s1.Push(4);
Stack s2(s1);
return 0;
}
运行上边代码,可以发现程序崩溃了,既然编译器可以给我们默认生成拷贝构造函数,那为什么程序崩溃了,其实在之前我们写的拷贝构造都属于浅拷贝,即使我们不写编译器也会自动生成,按字节直接拷贝,而这种拷贝方式对_a这种动态开辟的空间来说是行不通的,我们需要自己写拷贝构造,手动开辟空间拷贝过去,即深拷贝。
正确代码:
class Stack
{
public:
Stack(int capacity = 4)
{
cout << "构造函数" << endl;
_a = (int*)malloc(sizeof(int) * capacity);
if (_a == nullptr)
{
perror("malloc fail");
exit(-1);
}
_top = 0;
_capacity = capacity;
}
//拷贝构造 - 深拷贝
Stack(const Stack& st)
{
int* tmp = (int*)malloc(sizeof(int) * st._capacity);
if (tmp == nullptr)
{
perror("malloc fail");
exit(-1);
}
memcpy(tmp, st._a, sizeof(int) * st._top);
_capacity = st._capacity;
_top = st._top;
}
~Stack()
{
cout << "析构函数" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
int* _a;
int _capacity;
int _top;
};
int main()
{
Stack st1;
Stack st2(st1);
return 0;
}
注意:类中如果没有涉及资源申请时,拷贝构造函数是否写都可以;一旦涉及到资源申请时,则拷贝构造函数是一定要写的,否则就是浅拷贝。
5. 拷贝构造函数典型调用场景:使用已存在对象创建新对象函数参数类型为类类型对象函数返回值类型为类类型对象
需要写析构函数的类,都需要写深拷贝的拷贝构造 比如 Stack
不需要写析构函数的类,默认生成的浅拷贝的拷贝构造就可以用 Date/MyQueue
对于内置类型的拷贝就不需要写拷贝构造,因为不会调用析构函数。
五.赋值运算符重载:
5.1 运算符重载:
函数名字为:关键字 operator 后面接需要重载的运算符符号 。函数原型: 返回值类型 operator 操作符 ( 参数列表 )
注意:
1. 不能通过连接其他符号来创建新的操作符:比如 operator@2. 重载操作符必须有一个类类型参数3. 用于内置类型的运算符,其含义不能改变,例如:内置的整型 + ,不 能改变其含义4. 作为类成员函数重载时,其形参看起来比操作数数目少 1 ,因为成员函数的第一个参数为隐藏的this5. .* :: sizeof ?: . 注意以上 5 个运算符不能重载。这个经常在笔试选择题中出现。
总结来说,运算符重载就是将内置类型的 + - * / 等运算,或者 > < = 等比较运算符,通过运算符重载,让类也可以运用这些运算符直接操作,不用再在类中写一些操作方法了。
我们看一下一下代码:
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
// 基本类型(内置类型)
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2022, 9, 21);
Date d2(2022, 9, 22);
cout << (d1 == d2) << endl;
return 0;
}
运行过后会发现报错,不运用运算符重载的话,只有内置类型可以直接使用运算符操作,类是不能直接运算的。
下面我们看一下运算符重载如何实现:(这里我们演示封装好的)
class Date
{
friend ostream& operator<<(ostream& out, const Date& d);
friend istream& operator>>(istream& in, Date& d);
public:
int GetMonthDay(int year, int month);
Date(int year = 2023, int month = 5, int day = 1);
Date(const Date& d);
void Printf();
bool operator==(const Date& d);
bool operator!=(const Date& d);
bool operator>(const Date& d);
bool operator<(const Date& d);
bool operator>=(const Date& d);
bool operator<=(const Date& d);
Date& operator=(const Date& d);
Date& operator+=(int day);
Date operator+(int day);
Date& operator-=(int day);
Date operator-(int day);
int operator-(const Date& d);
Date& operator++();
Date operator++(int);
private:
int _year;
int _month;
int _day;
};
bool Date::operator==(const Date& d)
{
return _year == d._year &&
_month == d._month &&
_day == d._day;
}
bool Date::operator!=(const Date& d)
{
return !(*this == d);
}
bool Date::operator>(const Date& d)
{
if (_year > d._year)
{
return true;
}
else if (_year == d._year && _month > d._month)
{
return true;
}
else if (_year == d._year && _month == d._month && _day > d._day)
{
return true;
}
else
{
return false;
}
}
bool Date::operator<(const Date& d)
{
return !(*this == d) && !(*this > d);
}
bool Date::operator>=(const Date& d)
{
return !(*this < d);
}
bool Date::operator<=(const Date& d)
{
return !(*this > d);
}
在实现各个运算符的重载时,尽量以复用的形式来完成函数,可以提高效率。
5.2 赋值运算符重载:
以上的重载都属于一些比较运算,而赋值重载顾名思义,是类之间的计算赋值,你如两个类d1,d2,满足d2 = d1,即将d1的属性给到d2,即为赋值重载。
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
void TestDate2()
{
Date d1(2023, 5, 1);
Date d2(2023, 4, 30);
d2 = d1; //赋值重载
d1.Printf();
d2.Printf();
}
仔细观察代码,发现赋值重载似乎于拷贝构造的一种写法相似,即Date d2 = d1,但不同的是赋值重载要求两个类都已经初始化,然后进行赋值。
赋值重载我们实现赋值的功能就基本完成,那为什么函数的返回值要写成Date&?
我们知道对于内置类型,可以进行连续赋值,即链式赋值。
int i, j;
i = j = 10;//链式赋值,因此日期类也要满足这种赋值
这属于运算符=的特性,所以我们在实现赋值重载时也需要完成该功能。
下面我们实现一下其他的赋值重载:
Date& Date::operator+=(int day)
{
if (day < 0)
{
*this -= -day;
return *this;
}
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_year++;
_month = 1;
}
}
return *this;
}
Date Date::operator+(int day)
{
Date tmp = *this;
tmp += day;
return tmp;
}
Date& Date::operator-=(int day)
{
if (day < 0)
{
*this += -day;
return *this;
}
_day -= day;
while (_day <= 0)
{
_day += GetMonthDay(_year, _month);
_month--;
if (_month == 0)
{
_year--;
_month = 12;
}
}
return *this;
}
Date Date::operator-(int day)
{
Date tmp = *this;
tmp -= day;
return tmp;
}
int Date::operator-(const Date& d)
{
Date max = *this;
Date min = d;
int flag = 1;
if (*this < d)
{
max = d;
min = *this;
flag = -1;
}
int count = 0;
while (max != min)
{
++min;
++count;
}
return count * flag;
}
Date& Date::operator++()
{
*this += 1;
return *this;
}
Date Date::operator++(int)
{
Date tmp = *this;
*this += 1;
return tmp;
}
5.3 注意:
1.赋值运算符重载格式参数类型 : const T& ,传递引用可以提高传参效率返回值类型 : T& ,返回引用可以提高返回的效率,有返回值目的是为了支持连续赋值检测是否自己给自己赋值返回 *this :要复合连续赋值的含义2.赋值运算符只能重载成类的成员函数不能重载成全局函数原因:赋值运算符如果不显式实现,编译器会生成一个默认的。此时用户再在类外自己实现一个全局的赋值运算符重载,就和编译器在类中生成的默认赋值运算符重载冲突了,故赋值运算符重载只能是类的成员函数。3. 用户没有显式实现时,编译器会生成一个默认赋值运算符重载,以值的方式逐字节拷贝 。注 意:内置类型成员变量是直接赋值的,而自定义类型成员变量需要调用对应类的赋值运算符 重载完成赋值。![]()
对于6个默认成员函数都有一个共同的特性,即如果我们不在程序中写编译器会默认生成,而默认生成的成员函数我们在学习前几个函数时已经知道,它们对于数据的拷贝默认都为浅拷贝,即按字节将数据拷贝过去,但这样拷贝对于动态空间会出现错误, 赋值重载也不例外,假设两个栈st1和st2,将st1中的数据调用赋值重载赋值给st2,当st1给st2赋值时,st1中的数据,包括开辟数组的地址将会原封不动的拷贝到st2中,即st2中的_a会指向st1的_a,这样就会导致,st2中原空间丢失,可能导致空间泄露,除此之外在析构st1和st2时,会析构两次st1的_a指向的地址,因为st2中的_a也指向这个地址,进而导致程序崩溃。
正确的Stack类的赋值重载:
Stack& operator=(const Stack& st)
{
if (this != &st)
{
free(_a);
_a = (int*)malloc(sizeof(int) * st._capacity);
if (_a == nullptr)
{
perror("malloc fail");
exit(-1);
}
memcpy(_a, st._a, sizeof(int) * st._top);
_capacity = st._capacity;
return *this;
}
}
六.const成员:
将 const 修饰的 “ 成员函数 ” 称之为 const 成员函数 , const 修饰类成员函数,实际修饰该成员函数 隐含的 this 指针 ,表明在该成员函数中 不能对类的任何成员进行修改。
class A
{
public:
//const *this
//*this的类型变成const A*
void Printf() const
{
cout << _a << endl;
}
private:
int _a = 10;//ȱʡֵ
};
int main()
{
const A aa;//权限放大
aa.Printf();
cout << &aa << endl;
return 0;
}
七.取地址及const取地址操作符重载
class Date
{
public :
Date* operator&()
{
return this;
}
const Date* operator&()const
{
return this;
}
private :
int _year ; // 年
int _month ; // 月
int _day ; // 日
};
八.日期类:
Date.h
#pragma once
#include<iostream>
#include<assert.h>
using namespace std;
//类中的小函数,适合做内联的函数,直接在类中定义即可
class Date
{
//友元函数
friend ostream& operator<<(ostream& out, const Date& d);
friend istream& operator>>(istream& in, Date& d);
public:
//构造函数
Date(int year = 2023, int month = 1, int day = 1);
Date(const Date& d);
int GetMonthDay(int year, int month) const;
void Printf() const;
//运算符重载
bool operator==(const Date& d) const;
bool operator!=(const Date& d) const;
bool operator>(const Date& d) const;
bool operator<(const Date& d) const;
bool operator<=(const Date& d) const;
bool operator>=(const Date& d) const;
//赋值重载
Date& operator=(const Date& d);
Date& operator+=(int day);
Date operator+(int day) const;
Date& operator-=(int day);
Date operator-(int day) const;
int operator-(const Date& d) const;//俩日期相减
Date& operator++();//前置++
Date operator++(int);//后置++
private:
int _year;
int _month;
int _day;
};
//流插入
inline ostream& operator<<(ostream& out, const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日";
return out;
}
//流提取
inline istream& operator>>(istream& in, Date& d)
{
in >> d._year >> d._month >> d._day;
return in;
}
Date.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"Date.h"
int Date::GetMonthDay(int year, int month) const
{
assert(month > 0 && month < 13);
int Array[13] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
if (month == 2 && (year % 4 == 0 && year % 100 != 0) || (year % 400) == 0)
{
return 29;
}
else
{
return Array[month];
}
}
//构造函数
Date::Date(int year, int month, int day)
{
if (month > 0 && month < 13 && (day > 0 && day <= GetMonthDay(year, month)))
{
_year = year;
_month = month;
_day = day;
}
else
{
cout << "错误日期" << endl;
}
}
//拷贝构造 - 浅拷贝
Date::Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
void Date::Printf() const
{
cout << _year << "年" << _month << "月" << _day << "日" << endl;
}
bool Date::operator==(const Date& d) const
{
return _year == d._year &&
_month == d._month &&
_day == d._day;
}
bool Date::operator!=(const Date& d) const
{
return !(*this == d);
}
bool Date::operator>(const Date& d) const
{
if (_year > d._year)
{
return true;
}
else if (_year == d._year && _month > d._month)
{
return true;
}
else if (_year == d._year && _month == d._month && _day > d._day)
{
return true;
}
else
{
return false;
}
}
bool Date::operator<(const Date& d) const
{
return !(*this == d) && !(*this > d);
}
bool Date::operator<=(const Date& d) const
{
return (*this < d) || (*this == d);
}
bool Date::operator>=(const Date& d) const
{
return !(*this < d);
}
//赋值运算符重载
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
Date& Date::operator+=(int day)
{
if (day < 0)
{
*this -= -day;
return *this;
}
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_year++;
_month = 1;
}
}
return *this;
}
Date Date::operator+(int day) const
{
Date tmp = *this;
tmp += day;
return tmp;
}
Date& Date::operator-=(int day)
{
if (day < 0)
{
*this += -day;
return *this;
}
_day -= day;
while (_day <= 0)
{
_month--;
if (_month == 0)
{
_year--;
_month = 12;
}
_day += GetMonthDay(_year, _month);
}
return *this;
}
Date Date::operator-(int day) const
{
Date tmp = *this;
tmp -= day;
return tmp;
}
int Date::operator-(const Date& d) const
{
Date max = *this;
Date min = d;
int flag = 1;
if (*this < d)
{
max = d;
min = *this;
flag = -1;
}
int n = 0;
while (max != min)
{
++min;
++n;
}
return n*flag;
}
//前置++
Date& Date::operator++()
{
*this += 1;
return *this;
}
//后置++
//类型int为和前置++区分,占位
Date Date::operator++(int)
{
Date tmp = *this;
*this += 1;
return tmp;
}
//void Date::operator<<(ostream& out);
//{
// out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
//}
流插入
//ostream& operator<<(ostream& out, const Date& d)
//{
// out << d._year << "年" << d._month << "月" << d._day << "日";
// return out;
//}
流提取
//istream& operator>>(istream& in, Date& d)
//{
// in >> d._year >> d._month >> d._day;
// return in;
//}
Test.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"Date.h"
void TestDate1()
{
Date d1(2023, 5, 1);//构造函数
Date d2(2023, 4, 30);//拷贝构造
d1.Printf();
d2.Printf();
cout << (d1 == d2) << endl;
cout << (d1 != d2) << endl;
cout << (d1 > d2) << endl;
cout << (d1 < d2) << endl;
cout << (d1 >= d2) << endl;
cout << (d1 <= d2) << endl;
}
void TestDate2()
{
Date d1(2023, 5, 1);
Date d2(2023, 4, 30);
d2 = d1; //赋值重载
d1.Printf();
d2.Printf();
}
void TestDate3()
{
Date d1(2023, 5, 1);
Date d2 = d1 + 100;
d1.Printf();
d2.Printf();
//d2 -= 100;
//d2.Printf();
d1 = d2 - 100;
d1.Printf();
d2.Printf();
d2 += -100;
d2.Printf();
d2 -= -100;
d2.Printf();
}
void TestDate4()
{
Date d1(2002, 12, 21);
Date d2(2023, 5, 1);
d1.Printf();
d2.Printf();
int day = d2 - d1;
cout << day << endl;
}
void TestDate5()
{
Date d1(2023, 5, 2);
cout << d1 << endl;
Date d2;
cin >> d2;
cout << d2 << endl;
}
int main()
{
/*TestDate1();*/
/*TestDate2();*/
/*TestDate3();*/
/*TestDate4();*/
TestDate5();
return 0;
}