思维导图
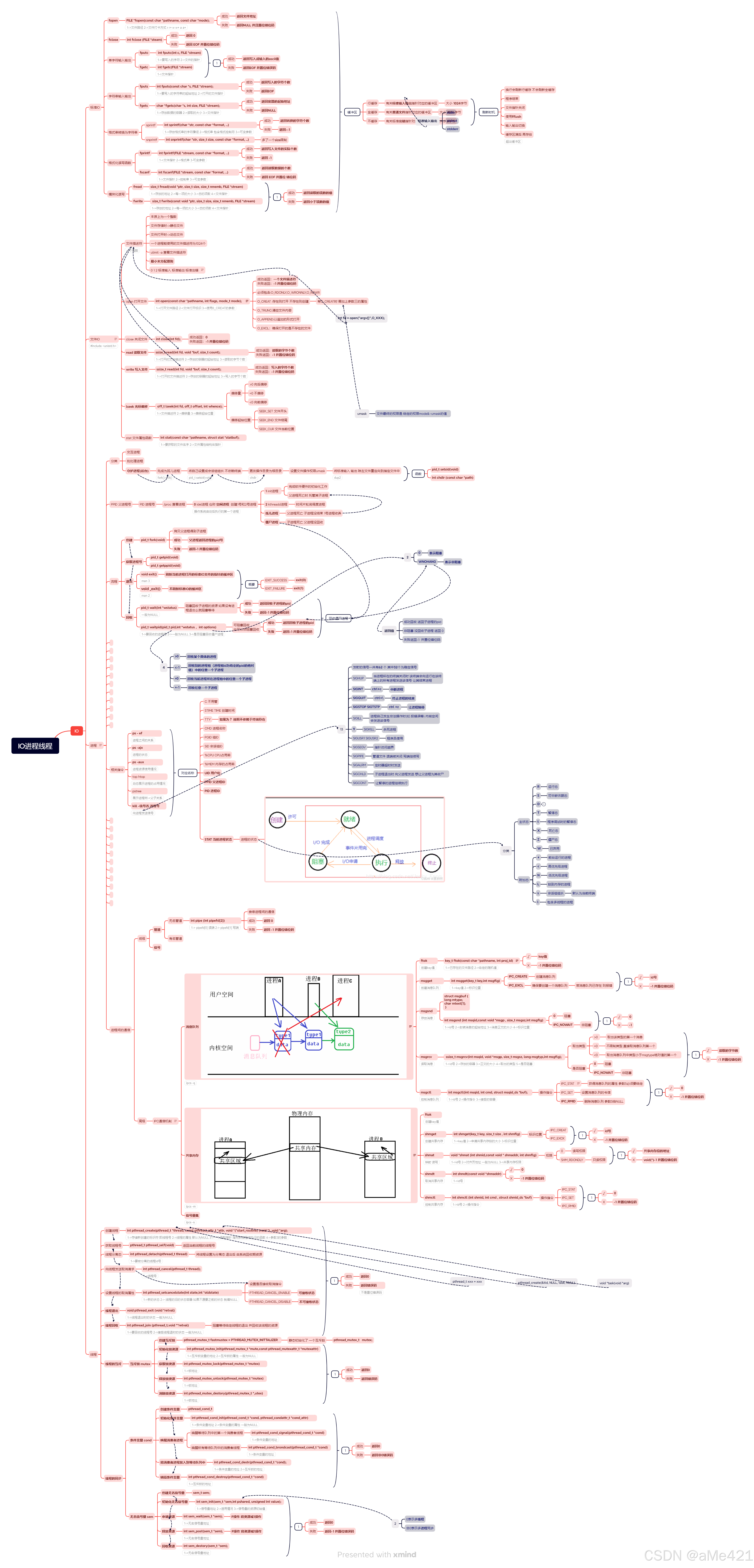
作业
// 使用消息队列完成两个进程间的互相通信
#include <myhead.h>
struct msbuf
{
long mtype;
char mtext[1024];
};
int main(int argc, char const *argv[])
{
// 创建子进程
pid_t pid = fork();
if (pid == -1)
{
perror("pid1 fork error");
return -1;
}
// 父进程
if (pid > 0)
{
// key
key_t key1 = ftok("/", 'k');
// 消息队列
int id = msgget(key1, IPC_CREAT | 0664);
if (id == -1)
{
perror("msgget error");
return -1;
}
// 写入
struct msbuf buf;
while (1)
{
printf("请输入消息的类型:");
scanf("%ld", &buf.mtype);
getchar();
printf("请输入要发送的消息:");
fgets(buf.mtext, sizeof(buf.mtext), stdin);
buf.mtext[strlen(buf.mtext) - 1] = 0;
msgsnd(id, &buf, sizeof(buf.mtext), 0);
}
sleep(1);
// 删除
if (msgctl(id, IPC_RMID, NULL) == -1)
{
perror("msgctl error");
}
}
else if (pid == 0)
{
// key
key_t key1 = ftok("/", 'a');
// 消息队列
int id = msgget(key1, IPC_CREAT | 0664);
if (id == -1)
{
perror("msgget error");
return -1;
}
// 读取
struct msbuf buf;
while (1)
{
msgrcv(id, &buf, sizeof(buf.mtext), 0, 0);
printf("\n");
printf("收到的消息为:%s \n", buf.mtext);
}
// 删除
if (msgctl(id, IPC_RMID, NULL) == -1)
{
perror("msgctl error");
}
}
return 0;
}
// 使用消息队列完成两个进程间的互相通信
#include <myhead.h>
struct msbuf
{
long mtype;
char mtext[1024];
};
int main(int argc, char const *argv[])
{
// 创建子进程
pid_t pid = fork();
if (pid == -1)
{
perror("pid1 fork error");
return -1;
}
if (pid == 0)
{
// key
key_t key1 = ftok("/", 'a');
// 消息队列
int id = msgget(key1, IPC_CREAT | 0664);
if (id == -1)
{
perror("msgget error");
return -1;
}
// 写入
struct msbuf buf;
while (1)
{
printf("请输入消息的类型:");
scanf("%ld", &buf.mtype);
getchar();
printf("请输入要发送的消息:");
fgets(buf.mtext, sizeof(buf.mtext), stdin);
buf.mtext[strlen(buf.mtext) - 1] = 0;
msgsnd(id, &buf, sizeof(buf.mtext), 0);
}
// 删除
if (msgctl(id, IPC_RMID, NULL) == -1)
{
perror("msgctl error");
}
}
// 父进程
else if (pid > 0)
{
// key
key_t key1 = ftok("/", 'k');
// 消息队列
int id = msgget(key1, IPC_CREAT | 0664);
if (id == -1)
{
perror("msgget error");
return -1;
}
// 读取
struct msbuf buf;
while (1)
{
msgrcv(id, &buf, sizeof(buf.mtext), 0, 0);
printf("\n");
printf("收到的消息为:%s \n", buf.mtext);
}
sleep(1);
// 删除
if (msgctl(id, IPC_RMID, NULL) == -1)
{
perror("msgctl error");
}
}
return 0;
}