A - Potions
Description
Problem Statement
Naohiro has a monster. The monster’s current health is
H
H
H.
He also has
N
N
N kinds of potions, numbered from
1
1
1 to
N
N
N in ascending order of effectiveness.
If you give the monster potion
n
n
n, its health will increase by
P
n
P_n
Pn. Here,
P
1
<
P
2
<
⋯
<
P
N
P_1 \lt P_2 \lt \dots \lt P_N
P1<P2<⋯<PN.
He wants to increase the monster’s health to
X
X
X or above by giving it one of the potions.
Print the number of the least effective potion that can achieve the purpose. (The constraints guarantee that such a potion exists.)
Constraints
2
≤
N
≤
100
2 \leq N \leq 100
2≤N≤100
1
≤
H
<
X
≤
999
1 \leq H \lt X \leq 999
1≤H<X≤999
1
≤
P
1
<
P
2
<
⋯
<
P
N
=
999
1 \leq P_1 \lt P_2 \lt \dots \lt P_N = 999
1≤P1<P2<⋯<PN=999
All input values are integers.
Input
The input is given from Standard Input in the following format:
N N N H H H X X X
P 1 P_1 P1 P 2 P_2 P2 … \dots … P N P_N PN
Output
Print the number of the least effective potion that can achieve the purpose.
Sample Input 1
3 100 200
50 200 999
Sample Output 1
2
Below is the change in the monster’s health when one of the potions is given to the monster.
If potion
1
1
1 is given, the monster’s health becomes
100
+
50
=
150
100 + 50 = 150
100+50=150.
If potion
2
2
2 is given, the monster’s health becomes
100
+
200
=
300
100 + 200 = 300
100+200=300.
If potion
3
3
3 is given, the monster’s health becomes
100
+
999
=
1099
100 + 999 = 1099
100+999=1099.
The potions that increase the monster’s health to at least
X
=
200
X = 200
X=200 are potions
2
2
2 and
3
3
3.
The answer is the least effective of them, which is potion
2
2
2.
Sample Input 2
2 10 21
10 999
Sample Output 2
2
Sample Input 3
10 500 999
38 420 490 585 613 614 760 926 945 999
Sample Output 3
4
Solution
直接暴力找最小…
Code
#include <bits/stdc++.h>
#define int long long
using namespace std;
signed main()
{
cin.tie(0);
cout.tie(0);
ios::sync_with_stdio(0);
int N, K, X;
cin >> N >> K >> X;
std::vector<int> A(N + 1);
int mn = 1e18, pos;
for (int i = 1; i <= N; i ++)
{
cin >> A[i];
if (A[i] < mn && A[i] + K >= X)
{
mn = A[i];
pos = i;
}
}
cout << pos << endl;
return 0;
}
Time Complexity: O(N) \text{\color{blue}{O(N)}} O(N)
B - MissingNo.
Description
Problem Statement
Naohiro had
N
+
1
N+1
N+1 consecutive integers, one of each, but he lost one of them.
The remaining
N
N
N integers are given in arbitrary order as
A
1
,
…
,
A
N
A_1,\ldots,A_N
A1,…,AN. Find the lost integer.
The given input guarantees that the lost integer is uniquely determined.
Constraints
2
≤
N
≤
100
2 \leq N \leq 100
2≤N≤100
1
≤
A
i
≤
1000
1 \leq A_i \leq 1000
1≤Ai≤1000
All input values are integers.
The lost integer is uniquely determined.
Input
The input is given from Standard Input in the following format:
N N N
A 1 A_1 A1 A 2 A_2 A2 … \ldots … A N A_N AN
Output
Print the answer.
Sample Input 1
3
2 3 5
Sample Output 1
4
Naohiro originally had four integers,
2
,
3
,
4
,
5
2,3,4,5
2,3,4,5, then lost
4
4
4, and now has
2
,
3
,
5
2,3,5
2,3,5.
Print the lost integer,
4
4
4.
Sample Input 2
8
3 1 4 5 9 2 6 8
Sample Output 2
7
Sample Input 3
16
152 153 154 147 148 149 158 159 160 155 156 157 144 145 146 150
Sample Output 3
151
Solution
- 排序
- 找出相邻两个数差不为 1 1 1
- 输出这两个数中较小的数 + 1 +1 +1
Code
#include <bits/stdc++.h>
#define int long long
using namespace std;
signed main()
{
cin.tie(0);
cout.tie(0);
ios::sync_with_stdio(0);
int N;
cin >> N;
std::vector<int> A(N + 1);
for (int i = 1; i <= N; i ++)
cin >> A[i];
sort(A.begin() + 1, A.end());
for (int i = 2; i <= N; i ++)
if (A[i] != A[i - 1] + 1)
{
cout << A[i - 1] + 1 << endl;
return 0;
}
return 0;
}
Time Complexity: O ( N log 2 N ) \color{blue}{O(N\log_2N)} O(Nlog2N)
C - Remembering the Days
Description
Problem Statement
A region has
N
N
N towns numbered
1
1
1 to
N
N
N, and
M
M
M roads numbered
1
1
1 to
M
M
M.
The
i
i
i-th road connects town
A
i
A_i
Ai and town
B
i
B_i
Bi bidirectionally with length
C
i
C_i
Ci.
Find the maximum possible total length of the roads you traverse when starting from a town of your choice and getting to another town without passing through the same town more than once.
Constraints
2
≤
N
≤
10
2 \leq N \leq 10
2≤N≤10
1
≤
M
≤
N
(
N
−
1
)
2
1 \leq M \leq \frac{N(N-1)}{2}
1≤M≤2N(N−1)
KaTeX parse error: Expected 'EOF', got '&' at position 12: 1 \leq A_i &̲lt; B_i \leq N
The pairs
(
A
i
,
B
i
)
(A_i,B_i)
(Ai,Bi) are distinct.
1
≤
C
i
≤
1
0
8
1\leq C_i \leq 10^8
1≤Ci≤108
All input values are integers.
Input
The input is given from Standard Input in the following format:
N N N M M M
A 1 A_1 A1 B 1 B_1 B1 C 1 C_1 C1
⋮ \vdots ⋮
A M A_M AM B M B_M BM C M C_M CM
Output
Print the answer.
Sample Input 1
4 4
1 2 1
2 3 10
1 3 100
1 4 1000
Sample Output 1
1110
If you travel as 4 → 1 → 3 → 2 4\to 1\to 3\to 2 4→1→3→2, the total length of the roads you traverse is 1110 1110 1110.
Sample Input 2
10 1
5 9 1
Sample Output 2
1
There may be a town that is not connected to a road.
Sample Input 3
10 13
1 2 1
1 10 1
2 3 1
3 4 4
4 7 2
4 8 1
5 8 1
5 9 3
6 8 1
6 9 5
7 8 1
7 9 4
9 10 3
Sample Output 3
20
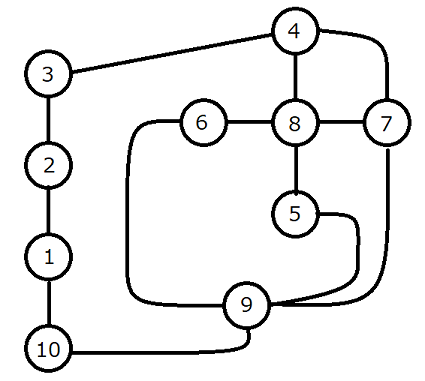
Solution
- 要想富,先读入
- 建图(邻接表)
- 对于每一个点,通过 DFS \text{DFS} DFS 算出最长距离(具体看代码)
Code
#include <bits/stdc++.h>
#define int long long
using namespace std;
const int SIZE = 1e2 + 10;
int N, M;
int h[SIZE], e[SIZE], ne[SIZE], w[SIZE], idx;
int st[SIZE], res = 0;
void add(int a, int b, int c)
{
e[idx] = b, ne[idx] = h[a], w[idx] = c, h[a] = idx ++;
}
void DFS(int u, int sum)
{
res = max(res, sum); //取最大
for (int i = h[u]; ~i; i = ne[i])
{
int j = e[i];
if (st[j]) continue; //说明走过
st[j] = 1;
DFS(j, sum + w[i]); //记录距离
st[j] = 0; //回溯
}
}
signed main()
{
cin.tie(0);
cout.tie(0);
ios::sync_with_stdio(0);
memset(h, -1, sizeof h);
cin >> N >> M;
int a, b, c;
for (int i = 1; i <= M; i ++)
cin >> a >> b >> c, add(a, b, c), add(b, a, c);
for (int i = 1; i <= N; i ++)
{
memset(st, 0, sizeof st); //清空
st[i] = 1;
DFS(i, 0);
}
cout << res << endl;
return 0;
}
Time Complexity: O ( N × N ! ) \color{blue}{O(N\times N!)} O(N×N!)
D - President
Description
Problem Statement
Takahashi and Aoki are competing in an election.
There are
N
N
N electoral districts. The
i
i
i-th district has
X
i
+
Y
i
X_i + Y_i
Xi+Yi voters, of which
X
i
X_i
Xi are for Takahashi and
Y
i
Y_i
Yi are for Aoki. (
X
i
+
Y
i
X_i + Y_i
Xi+Yi is always an odd number.)
In each district, the majority party wins all
Z
i
Z_i
Zi seats in that district. Then, whoever wins the majority of seats in the
N
N
N districts as a whole wins the election. (
∑
i
=
1
N
Z
i
\displaystyle \sum_{i=1}^N Z_i
i=1∑NZi is odd.)
At least how many voters must switch from Aoki to Takahashi for Takahashi to win the election?
Constraints
1
≤
N
≤
100
1 \leq N \leq 100
1≤N≤100
0
≤
X
i
,
Y
i
≤
1
0
9
0 \leq X_i, Y_i \leq 10^9
0≤Xi,Yi≤109
X
i
+
Y
i
X_i + Y_i
Xi+Yi is odd.
1
≤
Z
i
1 \leq Z_i
1≤Zi
∑
i
=
1
N
Z
i
≤
1
0
5
\displaystyle \sum_{i=1}^N Z_i \leq 10^5
i=1∑NZi≤105
∑
i
=
1
N
Z
i
\displaystyle \sum_{i=1}^N Z_i
i=1∑NZi is odd.
Input
The input is given from Standard Input in the following format:
N N N
X 1 X_1 X1 Y 1 Y_1 Y1 Z 1 Z_1 Z1
X 2 X_2 X2 Y 2 Y_2 Y2 Z 2 Z_2 Z2
⋮ \vdots ⋮
X N X_N XN Y N Y_N YN Z N Z_N ZN
Output
Print the answer.
Sample Input 1
1
3 8 1
Sample Output 1
3
Since there is only one district, whoever wins the seat in that district wins the election.
If three voters for Aoki in the district switch to Takahashi, there will be six voters for Takahashi and five for Aoki, and Takahashi will win the seat.
Sample Input 2
2
3 6 2
1 8 5
Sample Output 2
4
Since there are more seats in the second district than in the first district, Takahashi must win a majority in the second district to win the election.
If four voters for Aoki in the second district switch sides, Takahashi will win five seats. In this case, Aoki will win two seats, so Takahashi will win the election.
Sample Input 3
3
3 4 2
1 2 3
7 2 6
Sample Output 3
0
If Takahashi will win the election even if zero voters switch sides, the answer is 0 0 0.
Sample Input 4
10
1878 2089 16
1982 1769 13
2148 1601 14
2189 2362 15
2268 2279 16
2394 2841 18
2926 2971 20
3091 2146 20
3878 4685 38
4504 4617 29
Sample Output 4
86
Solution
这道题我们会发现,对于每一个选区,我们可以选择让 Takahashi \text{Takahashi} Takahashi 获胜或不让。
这,让我们想到了甚么? → 01 背包 \rightarrow 01背包 →01背包
背包首先要枚举物品 N N N,然后枚举价值也就是获胜之后的得分,即 ∑ i = 1 N Z i \displaystyle \sum_{i=1}^N Z_i i=1∑NZi。
这时候,我们看一眼数据范围: ∑ i = 1 N Z i ≤ 1 0 5 \displaystyle \sum_{i=1}^N Z_i \leq 10^5 i=1∑NZi≤105, 1 ≤ N ≤ 100 1 \leq N \leq 100 1≤N≤100
我们会发现相乘之后得到 1 0 7 10^7 107!正好在我们可接受的范围内,这更加坚定了我们用 01 背包 01背包 01背包 的决心。
01 背包 01背包 01背包
F i F_i Fi 表示物品价值为 i i i 时的最小花费
那么,我们为了让
Takahashi
\text{Takahashi}
Takahashi 获胜,即
Takahashi
\text{Takahashi}
Takahashi 得分必须大于
⌈
∑
i
=
1
N
Z
i
2
⌉
\left \lceil\frac{\displaystyle \sum_{i=1}^N Z_i}{2}\right \rceil
2i=1∑NZi
,所以最终答案就是
min
i
=
⌈
∑
i
=
1
N
Z
i
2
⌉
∑
i
=
1
N
Z
i
(
F
i
)
\min_{i=\left \lceil\frac{\sum_{i=1}^N Z_i}{2}\right \rceil}^{\sum_{i=1}^N Z_i}(F_i)
i=⌈2∑i=1NZi⌉min∑i=1NZi(Fi)
转移就和
01
背包
01背包
01背包 一模一样:
F
j
=
min
i
=
1
N
(
F
j
,
F
j
−
Z
i
+
B
i
−
A
i
+
1
2
)
F_j=\min_{i=1}^N(F_j, F_{j-Z_i}+\frac{B_i-A_i+1}{2})
Fj=i=1minN(Fj,Fj−Zi+2Bi−Ai+1)
注: A i A_i Ai, B i B_i Bi与题目中含义相同。
当然,有一个特殊情况:
B
i
<
A
i
B_i<A_i
Bi<Ai
此时,我们要特判,这时候本来就是
Takahashi
\text{Takahashi}
Takahashi 获胜,所以花费为
0
0
0。
最终转移方程为:
F
j
=
{
min
i
=
1
N
(
F
j
,
F
j
−
Z
i
)
B
i
<
A
i
min
i
=
1
N
(
F
j
,
F
j
−
Z
i
+
B
i
−
A
i
+
1
2
)
B
i
>
A
i
F_j=\begin{cases} & \min\limits_{i=1}^N(F_j, F_{j-Z_i}) &B_i<A_i\\ & \min\limits_{i=1}^N(F_j, F_{j-Z_i}+\frac{B_i-A_i+1}{2}) &B_i>A_i \end{cases}
Fj=⎩
⎨
⎧i=1minN(Fj,Fj−Zi)i=1minN(Fj,Fj−Zi+2Bi−Ai+1)Bi<AiBi>Ai
Code
#include <bits/stdc++.h>
#define int long long
using namespace std;
signed main()
{
cin.tie(0);
cout.tie(0);
ios::sync_with_stdio(0);
int N;
cin >> N;
std::vector<int> A(N + 1), B(N + 1), C(N + 1);
int sum = 0;
for (int i = 1; i <= N; i ++)
cin >> A[i] >> B[i] >> C[i], sum += C[i];
std::vector<int> F(sum + 1, 1e18);
F[0] = 0;
for (int i = 1; i <= N; i ++)
for (int j = sum; j >= C[i]; j --)
F[j] = min(F[j], F[j - C[i]] + (B[i] < A[i] ? 0 : (B[i] - A[i] + 1) / 2));
int res = 1e18;
for (int i = (sum + 1) / 2; i <= sum; i ++)
res = min(res, F[i]);
cout << res << endl;
return 0;
}
Time Complexity: O ( N × ∑ i = 1 N Z i ) \color{blue}{O(N\times\displaystyle \sum_{i=1}^N Z_i)} O(N×i=1∑NZi)
E - Avoid Eye Contact
Description
Problem Statement
There is a field divided into a grid of
H
H
H rows and
W
W
W columns.
The square at the
i
i
i-th row from the north (top) and the
j
j
j-th column from the west (left) is represented by the character
A
i
,
j
A_{i, j}
Ai,j. Each character represents the following.
.
: An empty square. Passable.
#
: An obstacle. Impassable.
>
, v
, <
, ^
: Squares with a person facing east, south, west, and north, respectively. Impassable. The person’s line of sight is one square wide and extends straight in the direction the person is facing, and is blocked by an obstacle or another person. (See also the description at Sample Input/Output
1
1
1.)
S
: The starting point. Passable. There is exactly one starting point. It is guaranteed not to be in a person’s line of sight.
G
: The goal. Passable. There is exactly one goal. It is guaranteed not to be in a person’s line of sight.
Naohiro is at the starting point and can move one square to the east, west, south, and north as many times as he wants. However, he cannot enter an impassable square or leave the field.
Determine if he can reach the goal without entering a person’s line of sight, and if so, find the minimum number of moves required to do so.
Constraints
2
≤
H
,
W
≤
2000
2 \leq H, W \leq 2000
2≤H,W≤2000
A
i
,
j
A_{i,j}
Ai,j is .
, #
, >
, v
, <
, ^
, S
, or G
.
Each of S
and G
occurs exactly once among
A
i
,
j
A_{i, j}
Ai,j.
Neither the starting point nor the goal is in a person’s line of sight.
Input
The input is given from Standard Input in the following format:
H H H W W W
A 1 , 1 A 1 , 2 … A 1 , W A_{1,1}A_{1,2}\dots A_{1,W} A1,1A1,2…A1,W
A 2 , 1 A 2 , 2 … A 2 , W A_{2,1}A_{2,2}\dots A_{2,W} A2,1A2,2…A2,W
⋮ \vdots ⋮
A H , 1 A H , 2 … A H , W A_{H,1}A_{H,2}\dots A_{H,W} AH,1AH,2…AH,W
Output
If Naohiro can reach the goal without entering a person’s line of sight, print the (minimum) number of moves required to do so. Otherwise, print -1
.
Sample Input 1
5 7
....Sv.
.>.....
.......
>..<.#<
^G....>
Sample Output 1
15
For Sample Input
1
1
1, the following figure shows the empty squares that are in the lines of sight of one or more people as !
.
Let us describe some of the squares. (Let
(
i
,
j
)
(i, j)
(i,j) denote the square in the
i
i
i-th row from the north and the
j
j
j-th column from the west.)
(
2
,
4
)
(2, 4)
(2,4) is a square in the line of sight of the east-facing person at
(
2
,
2
)
(2, 2)
(2,2).
(
2
,
6
)
(2, 6)
(2,6) is a square in the lines of sight of two people, one facing east at
(
2
,
2
)
(2, 2)
(2,2) and the other facing south at
(
1
,
6
)
(1, 6)
(1,6).
The square
(
4
,
5
)
(4, 5)
(4,5) is not in anyone’s line of sight. The line of sight of the west-facing person at
(
4
,
7
)
(4, 7)
(4,7) is blocked by the obstacle at
(
4
,
6
)
(4, 6)
(4,6), and the line of sight of the east-facing person at
(
4
,
1
)
(4, 1)
(4,1) is blocked by the person at
(
4
,
4
)
(4, 4)
(4,4).
Naohiro must reach the goal without passing through impassable squares or squares in a person’s line of sight.
Sample Input 2
4 3
S..
.<.
.>.
..G
Sample Output 2
-1
Print -1
if he cannot reach the goal.
Solution
操作方式
- 标记不能走的点(具体标记往下看)
- BFS \text{BFS} BFS 求最短路
标记不能走的点
首先,<,>,v,^,#
先都标记一下
然后,我们操作两遍:
-
第一遍:从 ( 1 , 1 ) (1,1) (1,1) 枚举到 ( H , W ) (H,W) (H,W),对于
>,v
进行处理- 如果当前遇到了
>
,那么我们将这一行标记为 1 1 1,这里由于已经枚举到了>
这个点,所以左边的是不会被标记的。 - 如果当前遇到了
v
,那么我们将这一列标记为 1 1 1,这里由于已经枚举到了这个点,所以上边的是不会被标记的。
- 因为有障碍物阻挡的话会停止标记,所以我们要判断:
- 如果当前是
>
,且这一列被标记为了 1 1 1,那么这个>
就会阻挡竖着的视线,所以这时候要标记会 0 0 0 - 如果当前是
v
,且这一行被标记为了 1 1 1,那么这个v
就会阻挡横着的视线,所以这时候要标记会 0 0 0 - 如果是其他情况,那么就会即阻挡行,也阻挡列,所以把当前行和当前列赋值为 0 0 0
- 如果当前是
- 如果当前遇到了
-
第二遍:从 ( H , W ) (H,W) (H,W) 枚举到 ( 1 , 1 ) (1,1) (1,1),对于
<,^
进行处理- 与上面类似,不写了(
其实是懒得写,qaq)
- 与上面类似,不写了(
为什么一个正着,一个倒着呢?
主要是对哪个方向的影响罢了~~~orz,orz
Code
#include <bits/stdc++.h>
#define int long long
#define x first
#define y second
using namespace std;
signed main()
{
cin.tie(0);
cout.tie(0);
ios::sync_with_stdio(0);
int H, W;
cin >> H >> W;
std::vector<vector<int>> st(H + 1, vector<int>(W + 1));
std::vector<vector<char>> graph(H + 1, vector<char>(W + 1));
int sx, sy, fx, fy;
for (int i = 1; i <= H; i ++)
for (int j = 1; j <= W; j ++)
{
cin >> graph[i][j], st[i][j] = (graph[i][j] != '.' && graph[i][j] != 'S' && graph[i][j] != 'G');
if (graph[i][j] == 'S') sx = i, sy = j;
if (graph[i][j] == 'G') fx = i, fy = j;
}
vector<int> col(W + 1), lin(H + 1);
for (int i = 1; i <= H; i ++) //正着处理
for (int j = 1; j <= W; j ++)
{
if (graph[i][j] == '>')
lin[i] = 1;
if (graph[i][j] == 'v')
col[j] = 1;
if (graph[i][j] == '.')
{
st[i][j] = col[j] | lin[i];
continue;
}
else if (graph[i][j] == '>')
col[j] = 0;
else if (graph[i][j] == 'v')
lin[i] = 0;
else
col[j] = lin[i] = 0;
}
for (int i = 1; i <=H; i ++)
lin[i] = 0;
for (int i = 1; i <= W; i ++)
col[i] = 0;
for (int i = H; i >= 1; i --) //倒着处理
for (int j = W; j >= 1; j --)
{
if (graph[i][j] == '<')
lin[i] = 1;
if (graph[i][j] == '^')
col[j] = 1;
if (graph[i][j] == '.')
{
st[i][j] |= col[j] | lin[i];
continue;
}
else if (graph[i][j] == '<')
col[j] = 0;
else if (graph[i][j] == '^')
lin[i] = 0;
else
col[j] = lin[i] = 0;
}
std::vector<vector<int>> dis(H + 1, vector<int>(W + 1, 2e9));
std::vector<vector<int>> st2(H + 1, vector<int>(W + 1));
int dx[4] = {1, 0, -1, 0}, dy[4] = {0, 1, 0, -1};
auto bfs = [&]() //搜索
{
queue<pair<int, int>> q;
q.push({sx, sy});
dis[sx][sy] = 0;
while (q.size())
{
auto t = q.front();
q.pop();
if (st2[t.x][t.y]) continue;
st2[t.x][t.y] = 1;
for (int i = 0; i < 4; i ++)
{
int xx = t.x + dx[i], yy = t.y + dy[i];
if (xx < 1 || yy < 1 || xx > H || yy > W || st[xx][yy]) continue;
dis[xx][yy] = min(dis[xx][yy], dis[t.x][t.y] + 1);
q.push({xx, yy});
}
}
};
bfs();
if (dis[fx][fy] == 2e9) puts("-1");
else cout << dis[fx][fy] << endl;
return 0;
}
Time Complexity: O ( H × W ) \color{blue}{O(H\times W)} O(H×W)
祝贺一下,
Atcoder
\colorbox{222222}{\color{FEFEFE}{Atcoder}}
Atcoder 登临
900
分
\bold{\color{337F00}{900分}}
900分!
祝大家也早日
AC
\colorbox{6EC40F}{\color{white}{AC}}
AC!