本篇是小编的第一篇文章,如有不足之处,还望指正!!!
在校大三学生,望各位前辈多指点,给予宝贵的建议!!!
废话不多说,闲话不多讲
接下来由由小编来讲述IO输入输出流的详细介绍,下图附上代码目录
一、文件的概念
要学会输入输出流首先要明白什么是文件,通俗的讲,文件就是我们保存数据的地方,比如我们用到的word文档,txt文件以及excel文件...都是文件,它既可以保存图片,也可以保存视频、声音...
知道了文件的定义,接下来我们要学一个词叫文件流,文件再程序中是以流的形式来操作的
二、流是什么?
数据再数据源(文件)和程序(内存)之间经历的路径就叫流
从图片中得知,流还分为输入流和输出流
1.输入流:数据从数据源(文件)到程序(内存)的路径。
2.输出流:数据从程序(内存)到数据源(文件)的路径。
三、创建文件
弄懂了文件的概念,接下来我们要学会创建文件,那么通常我们创建文件都是鼠标右键点新建,那么今天我们来学如果在程序中创建文件。
创建文件对象相关构造器和方法:
createNewFile()方法是创建新的文件
由图中看出相关方法和实现接口:
方式一:new File(String pathname) //根据路径构建一个File对象
方式二:new FIle(File parent ,String child) //根据父目录文件+子路径构建
方式三:new File(String parent,String child) //根据父目录+子路径创建
四、获取文件信息
学会了创建文件我们还要掌握如何获取文件信息
由图中我们可以看到File的方法,那么这些方法都是如何用的呢?
.我们需要掌握这几个方法getName、getAbslutePath、getParent、length、exists、isFile、isDirectory
1.getName:顾名思义就是获取文件的名字
2.getAbslutePath:获取文件的绝对路径
3.getParent:获取文件父级目录
4.length:获取文件大小(字节)
5.exists:文件是否存在
6.isFile:是不是一个文件
7.isDirectory:是不是一个目录
当我们掌握了创建文件和获取文件信息之后我们还需要掌握删除文件
五、删除文件
接下来我们来讲目录的操作和文件的删除
mkdir创建一级目录、mkdirs创建多级目录、delete删除空目录或文件
1.删除文件
2.删除多级目录
六、IO流的原理及流的分类
当我们掌握了这些我们就开始下一个阶段的学习IO流的原理及流的分类
1.Java IO流的原理:
(1)I/O是Input/Output的缩写,I/O技术是非常实用的技术,用于处理数据传输。如读/写文件,网络通讯等。
(2)Java程序中,对于数据的输入/输操作以流(stream)的方式进行。
(3)java.io包下提供了个各种“流”类和接口,用以获取不同种类的数据并通过方法输入或输出数据
(4)输入input:读取外部数据(磁盘、光盘等存储设备的数据)到程序(内存)中。
(5)输出output:将程序(内存)数据输出到磁盘、光盘等存储设备中。
2.流的分类:
(1)按操作数据单位不同分为:字节流(8 bit)二级制文件,字符流(按字符)文本文件
(2)按数据流的流向不同分为:输入流和输出流
(3)按流的角色的不同分为:节点流和处理流/包装流
该图的四个类都是抽象类
IO流体系图-常用的类
七、IO的常用类
下面开始细讲IO常用的类
1.InputStream:字节输入流
(1)InputStream抽象类是所有类字节输入流的超类
(2)InputStream常用类的子类
①FileInputStream:文件输入流
②BufferedInputStream:缓冲字节输入流
③ObjectInputStream:对象字节输入流
FileInputStream的基本使用:
演示FileInputStream的使用(字节输入流 文件 --> 程序)
(1).单个字节读取(效率较慢)
@Test
public void readFile01() throws IOException {
String filePath = "e:\\hello.txt"; //给出读取出路径
int readData = 0; //接收读到的内容
FileInputStream fileInputStream = null; //创建对象
try {
//创建了FileInputStream 对象 ,用于读取文件e:\hello.txt
fileInputStream = new FileInputStream(filePath);
//从该输入流读取一个字节的数据,如果没有输入可用,此方法将阻止
//如果返回-1,表示读取完毕
while((readData = fileInputStream.read()) != -1){
System.out.print((char)readData); //转成char显示
}
} catch (IOException e) {
e.printStackTrace();
}finally {
//关闭文件流,释放资源
fileInputStream.close();
}
}
注意:一定要关闭文件,释放资源,否则读取不成功
(2).使用 read(byte[] b) 读取文件,提高效率
@Test
public void readFile02() throws IOException {
String filePath = "e:\\hello.txt";
byte[] buf = new byte[8]; //一次读取八个字节
int readLen = 0;
FileInputStream fileInputStream = null;
try {
//创建了FileInputStream 对象 ,用于读取文件e:\hello.txt
fileInputStream = new FileInputStream(filePath);
//从该输入流读取读取最多b.length字节的数据到数组,此方法将阻止
//如果返回-1,表示读取完毕
//如果读取正常,返回实际读取的字节数
while((readLen = fileInputStream.read(buf)) != -1){
System.out.print(new String(buf,0, readLen)); //转成char显示
}
} catch (IOException e) {
e.printStackTrace();
}finally {
//关闭文件流,释放资源
fileInputStream.close();
}
}
2.FileOutputStream:字节输出流
构造方法与方法摘要:
FileOutputStream的类图
演示使用FileOutputStream 将数据写到文件中,如果该文件不存在,则创建文件
public class FileOutputStream01 {
public static void main(String[] args) {
}
/*
演示使用FileOutputStream 将数据写到文件中,
如果该文件不存在,则创建文件
*/
@Test
public void writeFile() throws IOException {
//创建 FileOutputStream对象
String filePath = "e:\\a.txt"; //路径
FileOutputStream fileOutputStream = null;
try {
//得到一个FileOutputStream 对象
//fileOutputStream = new FileOutputStream(filePath);
//创建方式,当写入内容时,时追加到文件后面
//fileOutputStream = new FileOutputStream(filePath,trur);
//创建方式,当写入内容时,是覆盖文件的内容
fileOutputStream = new FileOutputStream(filePath);
//一个字节
//fileOutputStream.write('H');
//写入一个字符串
String str = "hello,shr!";
fileOutputStream.write(str.getBytes());
//write(byte[] b,int off, int len)
//将len字节从位于偏移量 off的指定字节数组写入此文件输入流
//fileOutputStream.write(str.getBytes(),0,str.length());
} catch(IOException e) {
e.printStackTrace();
}finally {
fileOutputStream.close();
}
}
}
(1)综合使用FileInputSream和FileOutputStream,完成文件的拷贝
要求:编程完成图片/音乐的拷贝
代码演示:
public class FileCopy {
public static void main(String[] args) throws IOException {
//完成图片拷贝 将e:\\截图.jpg 拷贝到e:\\
/*
思路:
1.创建文件的输入流,将文件读取到程序
2.创建文件的输出流,将读取的文件数据,写入到指定文件
*/
String srcFilePath = "e:\\截图.jpg"; //原文件
String desFilePath = "e:\\截图3.jpg"; //目的文件
FileInputStream fileInputStream = null; //创建FileInputStream 输入对象
FileOutputStream fileOutputStream = null; //创建 FileOutputStream 输出对象
try {
fileInputStream = new FileInputStream(srcFilePath);
fileOutputStream = new FileOutputStream(desFilePath);
//定义一个字节数组 提高拷贝效率
byte[] buf = new byte[1024];
int readLen = 0;
while((readLen = fileInputStream.read(buf)) != -1){
//读取到后,就写入文件,通过fileOutputStream
//即,是一边读,一边写
,
//如果使用fileOutputStream.write(buf); 可能造成文件损失,
//因为使用了字节数组,每次都是读取1024个字节,
//如果最后一次读取不足1024个字节就会导致文件损失
fileOutputStream.write(buf,0,readLen);
}
System.out.println("拷贝完成~");
}catch (IOException e) {
e.printStackTrace();
} finally {
//关闭流
if (fileInputStream != null) {
fileInputStream.close();
}
//关闭流
if (fileOutputStream == null) {
fileOutputStream.close();
}
}
}
}
FileReader和FileWriter是字符流,即按照字符来操作io
3.FileReader:字符输入流
FileReader类图:
FileReader相关方法:
(1)new FileReader(File/String)
(2)read:每次读取单个字节,返回该字符,如果文件末尾返回-1
(3)read(char[]):批量读取多个字符到数组,返回读取到的字符数,如果带文件末尾返回-1
相关API:
(1)new String(char[]):将char[]转换成String
(2)new String(char[],off,len):将char[]的指定部分转换成String
FileReader实例:使用FileReader从story.txt读取内容
第一种方法:单个字符读取
//单个字符读取
public static void main(String[] args) {
String filePath = "e:\\story.txt"; //读取路径
//创建FlieReader对象
FileReader fileReader = null;
int date = 0; //接收读取的返回值
try {
fileReader = new FileReader(filePath);
//循环读取,使用read()
while ((date = fileReader.read()) != -1) {
System.out.print((char) date);
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
if (fileReader == null) {
try {
fileReader.close(); //关闭流
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
方法二:字符数组读取
public void readFile01(){
String filePath = "e:\\story.txt"; //路径
FileReader fileReader = null;//创建对象
int readLen = 0; //接收返回值
char[] buf = new char[8]; //字符数组
try {
fileReader = new FileReader(filePath);
//循环读取,使用read(buf) ,返回的结果是实际读取带的字符数
while ((readLen = fileReader.read(buf)) != -1) {
System.out.print(new String(buf, 0, readLen));
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
if (fileReader == null) {
try {
fileReader.close(); //关闭流
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
4.FileWriter:字符输出流
FileWriter类图
FileWriter常用方法:
(1)new FileWriter(File/String):覆盖模式,相当于流的指针再首段
(2) new FileWriter(File/String,true):追加模式,相当于流的指针再末端
(3)writr(int):写入单个字符
(4)writer(char[]):写入指定数组
(5)writer(char[],off,len):写入字符串的指定部分
(6)writer(string):写入整个字符串
(7)writer(String,off,len):写入字符串的指定部分
相关API:
String类:toCharArray:将String转换成char[]
注意:
FileWriter使用后,必须要关闭(close)或刷新(flush),否则写入不到指定的文件!
FileWriter实例:使用FileWriter将"风雨之后,定见彩虹"写入到note.txt文件中,注意细节
public class FileWriter_ {
public static void main(String[] args) {
//路径
String filePath = "e:\\note.txt";
//创建FileWriter对象
FileWriter fileWriter = null;
char[] chars = {'a','b','c'};
String str = "孙浩然";
try {
fileWriter = new FileWriter(filePath);
//writer(int) :写入单个字符
fileWriter.write('H');
//write(char[]):写入字符数组
fileWriter.write(chars);
//writer(char[],off,len): 写入指定数组的指定部分
fileWriter.write(chars, 0, chars.length);
//writer(String): 写入整个字符串
fileWriter.write(str);
fileWriter.write(" 风雨之后,必见彩虹");
//writer(String,off,len): 写入字符串的指定部分
fileWriter.write(str, 1, str.length() - 1);
//在数据量大的情况下,也可以循环添加
System.out.println("程序结束~~");
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
//对于我们的FileWriter,一定要关闭流,
// 或者flush才能真正把数据写入文件
try {
//fileWriter.flush(); //刷新
fileWriter.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
八、节点流和处理流
(1)节点流(低级流)可以从一个特定的数据源读写数据,如FileReader、FileWriter
(2)处理流/包装流是”连接“再已存在的流(节点流或处理流)之上,为程序提供更为强大的读写功能也更灵活,如BufferedReader和BufferedWriter(处理字符 文本文件)BufferedInputStream和BufferedOutputStream(处理字节 二进制文件)
BufferedReader类的属性有一个Reader,即可以封装一个节点流,该节点流可以是任意的(只要是Reader的子类)
BufferedWriter类的属性有一个Writer,即可以封装一个节点流,该节点流可以是任意的(只要是Writer的子类)
节点流和处理流的区别和联系
(1)节点流是底层流/低级流,直接跟数据源相连
(2)处理流包装节点流,既可以显出不同节点流实现的差异,也可以提供更方便的方法来完成输入输出。
(3)处理流/包装流对节点流进行包装,使用了修饰器设计模式,不会直接与数据源相连
处理流的功能主要体现再以下两个方面:
(1)性能的提高:主要以增加缓冲的方式来提高输入输出的效率。
(2)操作的便捷:处理流可能提供了一系列便捷的方法来一次输入输出大批量数据,使用更加灵活方便
1.BufferedReader类图:
BufferedReader实例:使用BufferedReader读取文本,并显示再控制台
public class BufferedReader_ {
public static void main(String[] args) throws IOException {
String filePath = "e:\\ok.txt";//路径
//创建缓冲流对象,套接在指定的节点流基础上
BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath));
//读取
String line = null;
while ((line = bufferedReader.readLine()) != null) { //读取一行
System.out.println(line);
}
//关闭,只需要关闭外层流即可
bufferedReader.close();
}
}
2.BufferedWriter类图:
BufferedWriter实例:将”Hello,shr“,写入到文件中
public class BufferedWriter_ {
public static void main(String[] args) throws IOException {
String filePath = "e:\\ok.txt"; //路径
//创建BufferedWriter
//1.new FileWriter(filePath),表示以覆盖的方式写入
//2.new FileWriter(filePath,true),表示以追加的方式写入
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(filePath));
bufferedWriter.write("hello1,shr");
bufferedWriter.newLine(); //插入一个和系统相关的换行
bufferedWriter.write("hello2,shr");
bufferedWriter.newLine();
bufferedWriter.write("hello3,shr");
//说明:关闭外层六即可,传入的new FileWriter(filePath)
//会在底层关闭
bufferedWriter.close();
}
}
3.应用实例:综合使用BufferedReader和BufferedWriter完成文本文件拷贝
public class BufferedCopy {
public static void main(String[] args) throws IOException {
//因为BufferedReader和BufferedWriter 是按照字符操作
//不要去操作二进制文件[声音,视频,doc,pdf],才做了可能会造成文件损坏
String srcFilePath = "e:\\ok.txt"; //原路径
String destFilePath = "e:\\note.txt"; //目的路径
BufferedReader br = null;//创建读对象
BufferedWriter bw = null; //创建写对象
try {
br = new BufferedReader(new FileReader(srcFilePath));
bw = new BufferedWriter(new FileWriter(destFilePath));
String line = null;
while ((line = br.readLine()) != null) {
//每读取一行就写入
bw.write(line);
//插入换行符
bw.newLine();
}
System.out.println("拷贝完毕~~");
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
//关闭流
if(br != null) {
br.close();
}
if(bw != null) {
bw.close();
}
}
}
}
BufferedInputStream和BufferedOutputStream是字节流,可以处理二级制文件
4.BufferedInputStream类图:
在创建BufferedInputStream时,会创建一个内部缓冲区数组
5.BufferedOutputStream类图:
BufferedOutputStream,实现缓冲的输出流,可以将多个字节写入底层输出流中,而不必每次字节写入调用底层系统
6.应用实例:编程完成图片/音乐的拷贝
public class BufferedCopy02 {
public static void main(String[] args) throws IOException {
String srcPath = "e:\\截图.jpg"; //原路径
String destPath = "e:\\截图3.jpg"; //目的路径
BufferedInputStream bufferedInputStream = null; //创建处理输入流对象
BufferedOutputStream bufferedOutputStream = null; //创建处理输出流对象
try {
bufferedInputStream = new BufferedInputStream(Files.newInputStream(Paths.get(srcPath)));
bufferedOutputStream = new BufferedOutputStream(Files.newOutputStream(Paths.get(destPath)));
//循环的读取文件,并写入到 destPath中
byte[] buffer = new byte[1024]; //字节数组
int length;//接收返回值
//当返回-1时,就表示读取完毕
while ((length = bufferedInputStream.read(buffer)) != -1) {
bufferedOutputStream.write(buffer, 0, length);
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
//关闭流,关闭外层流即可,底层会去关闭节点流
if (bufferedOutputStream != null) {
bufferedInputStream.close();
}
if (bufferedOutputStream != null) {
bufferedOutputStream.close();
}
}
}
}
九、对象流
下面是对象流的讲述:
对象流分为ObjectInputStream和ObjectOutputStream;
我们来看一个需求:
将int num=100 这个int数据保存到文件中,注意不是100这个数字,而是 int 100,并且能够从文件中直接恢复 int 100;
将Dog dog = new Dog("小黄",3),这个dog对象保存到文件中,并且能够从文件恢复;
上面的要求,就是能够将基本数据类型或者对象进行序列化和反序列化操作
序列化和反序列化:
(1)序列化就是在保存数据时,保存数据的值和数据类型
(2)反序列化就是在恢复数据时,恢复数据的值和数据类型
(3)需要让某个对象支持序列化机制,则必须让其类是可序列化的,为了让某个类是可序列化的,该类必须事项如下两个接口之一:
Serializable //这是一个标记接口
Externalizable
1.ObjectInputStream 提供反序列化功能
类图:
应用实例:使用ObjectInputStream读取data.dat并反序列化恢复数据
public class ObjectInputStream_ {
public static void main(String[] args) throws IOException, ClassNotFoundException {
String filePath = "e:\\data.txt";
ObjectInputStream ois = new ObjectInputStream(Files.newInputStream(Paths.get(filePath)));
//读取(反序列化)的顺序需要和你保存的数据(序列化)的顺序一致
System.out.println(ois.readInt());
System.out.println(ois.readBoolean());
System.out.println(ois.readDouble());
System.out.println(ois.readChar());
System.out.println(ois.readUTF());
Object object = ois.readObject();
System.out.println("运行类型为:" + object.getClass());
System.out.println("dog的信息为:" + object);
ois.close();//关闭流
}
}
2.ObjectOutputStream提供序列化功能
类图:
应用实例:使用ObjectOutputStream序列化基本数据类型和一个Dog对象(name,age),并保存到data.dat文件中
public class ObjectOutputStream_ {
public static void main(String[] args) throws IOException {
//序列化后,保存的文件格式,不是存文本,而是按照他的格式来保存
String filePath = "e:\\data.txt";
ObjectOutputStream oos =
new ObjectOutputStream(Files.newOutputStream(Paths.get(filePath)));
oos.writeInt(100); //int -> Integer实现了 Serializable)
oos.writeBoolean(true); //boolean -> Boolean(实现了 Serializable)
oos.writeDouble(3.14); //double -> Double(实现了 Serializable)
oos.writeChar('a'); // char -> Character(实现了 Serializable)
oos.writeUTF("shr"); //String -> String(实现了 Serializable)
//保存一个dog对象
oos.writeObject(new Dog("小黄",10));
oos.close();
System.out.println("数据保存成功(序列化形式)");
}
}
class Dog implements Serializable {
private String name;
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "name = " + name + " age = " + age;
}
}
从上图中我们可以看出这是一些乱码,但是我们依然能够从中读取带数据类型。
对象处理流的注意细节:
(1)读写顺序要一致
(2)要求实现序列化或反序列化对象,需要实现Serializable
(3)序列化的类中建议添加SeriaIVersionUID,为了提高版本的兼容性
(4)序列化对象时,默认将里面所有属性都进行序列化,但除了static或transient修饰的成员
(5)序列化对象时,要求里面属性的类型也需要实现序列化接口
(6)序列化具备可继承性,也就是如果某个类已经实现了序列化,则他的子类也已经默认实现了序列化
十、标准输入输出流
类型 | 默认设备 | |
System.in 标准输入 | InputStream | 键盘 |
System.out 标准输出 | PrintStream | 显示器 |
public class InputAndOutput {
public static void main(String[] args) {
//System 类的 public final static InputStream in = null;
//System.in 的编译类型 InputStream
//System.in 的运行类型 BufferedInputStream
//标准输入 键盘
System.out.println(System.in.getClass());
//System 类的 public final static PrintStream out = null;
//System.out 的编译类型是 PrintStream
//System.out 的运行类型是 PrintStream
//标准输出,显示器
System.out.println(System.out.getClass());
System.out.println("hello,shr");
Scanner scanner = new Scanner(System.in);
String next = scanner.next();
System.out.println("next = " + next);
}
}
十一、转换流
:InputStreamReader和OutputStreamWriter
先看一个乱码问题,引出学习转换流的必要性:
此时我们看到运行结果是是正常的,那么如果我们把编码改成ANSI呢,运行结果还是正常的吗?
我们来看运行结果:
这个时候我们发现,乱码出现了,那么这是为什么呢?为什么我们修改了编码就会出现乱码呢?
小猿来解答:出现乱码的根本原因是我们没有指定读取文件的编码方式,如果我们可以指定文件的编码方式,我i们就能够解决这个问题
转换流介绍:
(1)InputStreamReader:Reader的子类,可以将InputStream(字节流)包装成Reader(字符流)
(2)OuputStreamWtier:Writer的子类,实现将OutputStream(字节流)包装成Writer(字符流)
(3)当处理纯文本数据时,如果使用字符流酰氯更高,并且可以有效解决中文问题,所以建议将字节流转换成字符流
(4)可以在使用时指定编码方式(比如UTF-8、gbk、gb2312、iso8859-1等)
1.InputStreamReader类图:
下面来解决乱码问题:
public class InputStreamReader_ {
public static void main(String[] args) throws IOException {
String filePath = "e:\\a.txt";
//1.把 FileInputStream 转成InputStreamReader
//2.指定编码
//InputStreamReader isr = new InputStreamReader
//(new FileInputStream(filePath),"gbk");
//3.把InputStreamReader 传入 BufferedReader
//BufferedReader br = new BufferedReader(isr);
//可以把2,3合在一起
BufferedReader br = new BufferedReader(
new InputStreamReader(
new FileInputStream(filePath),"gbk"));
String string = br.readLine();
System.out.println("读取到的内容 = " + string);
br.close();
}
}
2.OutputStreamWriter类图: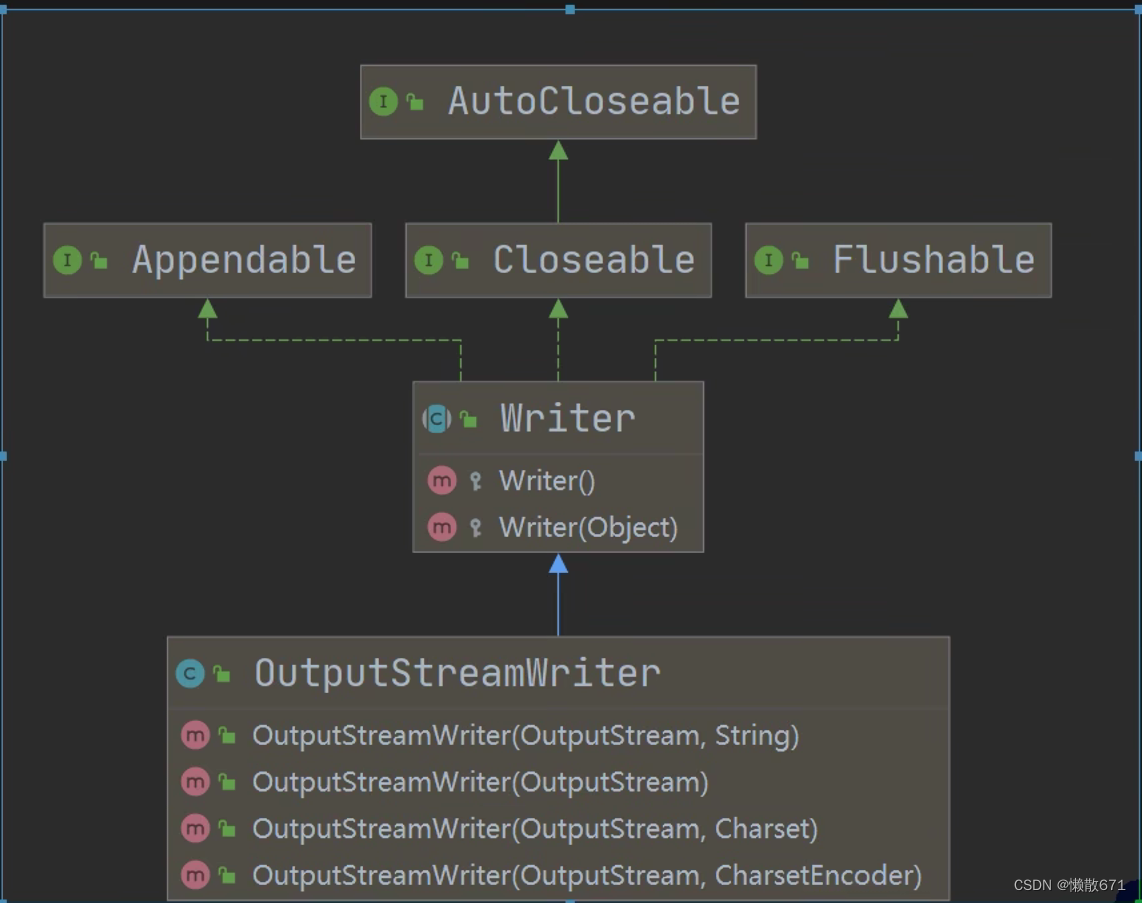
应用实例:将字节流FileOutputStream包装成(转换成)字符流OutputStreamWriter对文进行写入(按照gbk格式,可以指定其他)
public class OutputStreamReader_ {
public static void main(String[] args) throws IOException {
String filePath = "e:\\shr.txt";
OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream(filePath), "gbk");
osw.write("hi,孙浩然");
osw.close();
System.out.println("按照gbk保存文件成功~");
}
}
十二、打印流
:PrintStream和PrintWriter
只有输出流没有输入流
1.PrintStream类图:
演示PrintStream(字节打印流)
public class PrintStream_ {
public static void main(String[] args) throws IOException {
PrintStream out = System.out;
//在默认情况下,PrintStream 输出的位置是标准输出,即显示器
/*
public void print(String s) {
if (s == null) {
s = "null";
}
write(s);
}
*/
out.println("Hello,shr");
//因为print底层使用的是writer,所以我们可以直接调用writer进行打印
out.write("Hello,shr".getBytes());
//我们也可以改变打印流输出的位置
//修改位置到"e:\\a.txt"
System.setOut(new PrintStream("e:\\a.txt"));
System.out.println("hello,shr");
out.close();
}
}
2.PrintWriter类图:
演示PrintWriter(字符打印流)
public class PrintWriter_ {
public static void main(String[] args) throws IOException {
PrintWriter pw = new PrintWriter(System.out);
pw.println("Hello,shr");
pw.write("Hello,shr");
PrintWriter printWriter = new PrintWriter(new FileWriter("e:\\hello.txt"));
printWriter.write("hello,shr");
pw.close();
printWriter.close();
}
}
十三、Properties类
看一个需求:
如下配置文件 mysql.properties
ip=192.168.0.13 user=root pwd=12345
请问编程读取 ip、user、pwd的值是多少?
1.传统方法:
public class Properties01 {
public static void main(String[] args) throws IOException {
//读取mysql.properties 文件,得到ip,user,pwd
BufferedReader br = new BufferedReader(
new FileReader("src\\mysql.properties"));
String line = "";
while((line = br.readLine()) != null){ //循环读取
//System.out.println(line);
//把得到的字符串按照"="切割,变成字符数组
String[] split = line.split("=");
if(split.length == 2){
System.out.println(split[0] + "值是" + split[1]);
}
}
br.close();
}
}
2.Properties:
(1)专门用于读写配置文件的集合类
配置文件的格式:
键=值
键=值
(2)注意:键值对不需要空格,值不需要用引号包起来。默认类型是String
使用Properties类可以方便实现:
常用方法:
load:加载配置文件的键值对到Properties对象
list:将数据显示到指定设备
getProperty(key):根据键获取值
setProperty(key,value):设置键值对到Properties对象
store:将Properties中的键值对存储到配置文件,在idea中,保存信息到配置文件,如果含有中文,会存储为unicode码
3.应用案例:
(1)使用Properties类完成对mysql.properties的读取,代码演示:
public class Properties02 {
public static void main(String[] args) throws IOException {
//1.创建
Properties properties = new Properties();
//2.加载
properties.load(new FileReader("src\\mysql.properties"));
//3.把k-v显示控制台
properties.list(System.out);
//4.根据key获取对应的值
System.out.println(properties.getProperty("user"));
}
}
(2)使用Properties类来创建配置文件,修改配置文件的内容,代码演示:
public class Properties03 {
public static void main(String[] args) throws IOException {
Properties properties = new Properties();
//1.如果该文件没有key就是创建
//2.如果该文件有key就是修改
/*
properties 父类就是Hashtable 底层就是Hashtable核心方法
//源码:
public synchronized V put(K key, V value) {
// Make sure the value is not null
if (value == null) {
throw new NullPointerException();
}
// Makes sure the key is not already in the hashtable.
Entry<?,?> tab[] = table;
int hash = key.hashCode();
int index = (hash & 0x7FFFFFFF) % tab.length;
@SuppressWarnings("unchecked")
Entry<K,V> entry = (Entry<K,V>)tab[index];
for(; entry != null ; entry = entry.next) {
if ((entry.hash == hash) && entry.key.equals(key)) {
V old = entry.value;
entry.value = value; //如果存在就是替换
return old;
}
}
addEntry(hash, key, value, index); //如果不存在就创建
return null;
}
*/
properties.setProperty("charset", "utf-8");
properties.setProperty("user", "汤姆"); //注意保存时,是中文的unicode码值
properties.setProperty("pwd", "abc");
properties.setProperty("user","tom");
//将k-v存储到文件中
properties.store(Files.newOutputStream(Paths.get("src\\mysql.properties")),null);
System.out.println("保存配置文件成功");
}
}
到这里IO输入输出流就已经讲解结束,希望同学们有所收获,同时多交流,交换经验,如有不足之处,还望指出,小编也能及时更正。