链表理论基础总结:
什么是链表,链表是一种通过指针串联在一起的数据结构,每一个节点由两部分组成,一个是数据域一个是指针域(存放指向下一个节点的指针),最后一个节点的指针域指向null(空指针)
链表的入口节点称为链表的头节点,也就是head。
链表的类型
单链表: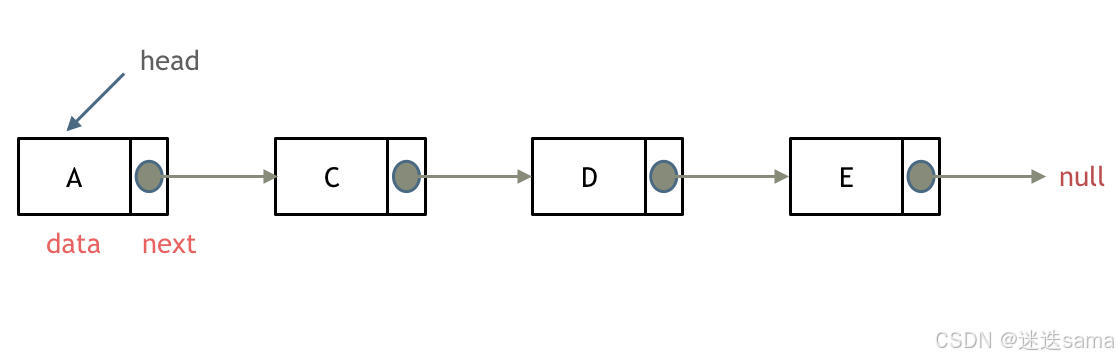
双链表:
单链表中的指针域只能指向节点的下一个节点。
双链表每一个节点有两个指针域,一个指向下一个节点,一个指向上一个节点。
循环链表:
循环链表,顾名思义,就是链表首尾相连。
链表的存储方式
数组在内存中是连续分布的,但是链表在内存中可不是连续分布的。
链表是通过指针域的指针链接在内存中各个节点。
所以链表中的节点在内存中不是连续分布的,而是散乱分布在内存中的某地上,分配机制取决于操作系统的内存管理。
链表的定义
// 单链表
struct ListNode {
int val; // 节点上存储的元素
ListNode *next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(NULL) {} // 节点的构造函数
};
链表的经典题目 :
/*
这里涉及如下链表操作的两种方式:
1.直接使用原来的链表来进行删除操作
2.设置一个虚拟头节点再进行删除操作
*/
1.直接使用原来的链表来进行移除节点操作:
class Solution {
public:
ListNode* removeElements(ListNode* head, int val) {
// 删除头结点
while (head != NULL && head->val == val) { // 注意这里不是if
ListNode* tmp = head;
head = head->next;
delete tmp;
}
// 删除非头结点
ListNode* cur = head;
while (cur != NULL && cur->next!= NULL) {
if (cur->next->val == val) {
ListNode* tmp = cur->next;
cur->next = cur->next->next;
delete tmp;
} else {
cur = cur->next;
}
}
return head;
}
};
2.设置一个虚拟头节点再进行移除节点操作:
class Solution {
public:
ListNode* removeElements(ListNode* head, int val) {
ListNode* dummyHead = new ListNode(0); // 设置一个虚拟头结点
dummyHead->next = head; // 将虚拟头结点指向head,这样方便后面做删除操作
ListNode* cur = dummyHead;
while (cur->next != NULL) {
if(cur->next->val == val) {
ListNode* tmp = cur->next;
cur->next = cur->next->next;
delete tmp;
} else {
cur = cur->next;
}
}
head = dummyHead->next;
delete dummyHead;
return head;
}
};
class MyLinkedList {
public:
// 定义链表节点结构体
struct LinkedNode {
int val;
LinkedNode *next;
LinkedNode(int val) : val(val), next(nullptr) {}
};
// 初始化链表
MyLinkedList() {
m_size = 0;
m_dummyHead = new LinkedNode(0);
}
int get(int index) {
if(index > (m_size - 1) || index < 0) {
return -1;
}
LinkedNode *cur = m_dummyHead->next;
while(index--) {
cur = cur->next;
}
return cur->val;
}
void addAtHead(int val) {
LinkedNode *newHead = new LinkedNode(val);
newHead->next = m_dummyHead->next;
m_dummyHead->next = newHead;
m_size++;
}
void addAtTail(int val) {
LinkedNode *newNode = new LinkedNode(val);
LinkedNode *cur = m_dummyHead;
while(cur->next != nullptr) {
cur = cur->next;
}
cur->next = newNode;
m_size++;
}
void addAtIndex(int index, int val) {
if(index > m_size) return;
if(index < 0) index = 0;
LinkedNode *newNode = new LinkedNode(val);
LinkedNode *cur = m_dummyHead;
while(index--) {
cur = cur->next;
}
newNode->next = cur->next;
cur->next = newNode;
m_size++;
}
void deleteAtIndex(int index) {
if(index >= m_size || index < 0) {
return;
}
LinkedNode *cur = m_dummyHead;
while(index--) {
cur = cur->next;
}
LinkedNode* temp = cur->next;
cur->next = cur->next->next;
delete temp;
temp = nullptr;
m_size--;
}
private:
int m_size;
LinkedNode *m_dummyHead;
};
/* 双指针法 */
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode *preNode = nullptr;
ListNode *curNode = head;
ListNode *tmp; // 保存cur的下一个节点
while(curNode) {
tmp = curNode->next;
curNode->next = preNode;
// 更新 preNode 和 curNode
preNode = curNode;
curNode = tmp;
}
return preNode;
}
};
/* 递归法 */
class Solution {
public:
ListNode* reverse(ListNode* pre,ListNode* cur){
if(cur == NULL) return pre;
ListNode* temp = cur->next;
cur->next = pre;
// 可以和双指针法的代码进行对比,如下递归的写法,其实就是做了这两步
// pre = cur;
// cur = temp;
return reverse(cur,temp);
}
ListNode* reverseList(ListNode* head) {
// 和双指针法初始化是一样的逻辑
// ListNode* cur = head;
// ListNode* pre = NULL;
return reverse(NULL, head);
}
};
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
ListNode *dummyNode = new ListNode(0);
dummyNode->next = head;
ListNode *currentNode = dummyNode;
while(currentNode->next != nullptr && currentNode->next->next != nullptr) {
ListNode *tmp1 = currentNode->next;
ListNode *tmp2 = currentNode->next->next->next;
currentNode->next = currentNode->next->next; // 步骤一
currentNode->next->next = tmp1; // 步骤二
currentNode->next->next->next = tmp2; // 步骤三
currentNode = currentNode->next->next; // 后移两位,准备下一轮交换
}
ListNode *res = dummyNode->next;
delete dummyNode;
return res;
}
};
/*
基本方法:1.遍历链表一次,计算长度
2.再次遍历链表,定位目标节点
时间复杂度是O(2n) = O(n)
*/
/*
双指针法:1.初始化两个指针'fast'和'slow',都指向链表的头节点
2.先让'fast'指针向前移动N步
3.然后同时移动'fast'和'slow'指针,直到'fast'指针指向链表的末尾,此时'slow'指针刚好指向倒数第N个节点的前一个节点
4.删除目标节点
时间复杂度是O(n)
*/
class Solution {
public:
ListNode* removeNthFromEnd(ListNode* head, int n) {
ListNode* dummyHead = new ListNode(0);
dummyHead->next = head;
ListNode* slow = dummyHead;
ListNode* fast = dummyHead;
while(n-- && fast != NULL) {
fast = fast->next;
}
fast = fast->next; // fast再提前走一步,因为需要让slow指向删除节点的上一个节点
while (fast != NULL) {
fast = fast->next;
slow = slow->next;
}
slow->next = slow->next->next;
// ListNode *tmp = slow->next; C++释放内存的逻辑
// slow->next = tmp->next;
// delete tmp;
return dummyHead->next;
}
};
/*
思路分析:1.计算链表长度,'lenA'和'lenB'
2.对齐两个链表的起点,假设'lenA > lenB',那么让链表A的指针向前移动'lenA - lenB'步,这样两个链表的剩余部分的长度相同
3.同时遍历两条链表,逐个比较它们的节点是否相同,如果找到一个节点相同(即'nodeA == nodeB'),那么这个节点就是它们的相交点,如果遍历到链表末尾都没有找到相同的节点,说明两个链表不相交
*/
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
ListNode* curA = headA;
ListNode* curB = headB;
int lenA = 0, lenB = 0;
while (curA != NULL) { // 求链表A的长度
lenA++;
curA = curA->next;
}
while (curB != NULL) { // 求链表B的长度
lenB++;
curB = curB->next;
}
curA = headA;
curB = headB;
// 让curA为最长链表的头,lenA为其长度
if (lenB > lenA) {
swap (lenA, lenB);
swap (curA, curB);
}
// 求长度差
int gap = lenA - lenB;
// 让curA和curB在同一起点上(末尾位置对齐)
while (gap--) {
curA = curA->next;
}
// 遍历curA 和 curB,遇到相同则直接返回
while (curA != NULL) {
if (curA == curB) {
return curA;
}
curA = curA->next;
curB = curB->next;
}
return NULL;
}
};
/*
双指针法:1.初始化两个指针'curA'和'curB',分别指向链表A和链表B的头节点
2.在每次迭代中,如果'curA'到达链表A的末尾,则将其重置为链表B的头节点;如果'curB'到达链表B的末尾,则将其重置为链表A的头节点
3.继续遍历,直到'curA'和'curB'相遇,即'curA == curB',这时这个节点就是两个链表的相交点
4.如果两个指针最终都遍历到了末尾且没有相交点,两个指针都会变成'nullptr',循环结束
*/
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
if (!headA || !headB) return nullptr;
ListNode *curA = headA;
ListNode *curB = headB;
while (curA != curB) {
curA = curA ? curA->next : headB;
curB = curB ? curB->next : headA;
}
return curA;
}
};
/*
思路分析:1.判断链表是否有环,使用快慢指针法:定义'fast'和'slow'指针,从头节点出发,'fast'指针每次移动两个节点,'slow'指针每次移动一个节点,如果'fast'和'slow'指针在途中相遇,说明这个链表有环
2.如果有环,如何找到这个环的入口:假设从头节点到环形入口节点的节点数为'x',环形入口节点到'fast'指针与'slow'指针相遇节点节点数为'y',从相遇节点再到环形入口节点节点数为'z'。那么相遇时,'slow'指针走过的节点数为 'x + y','fast'指针走过的节点数为'x + y + n(y+z)','n'为'fast'指针在环内走了'n'圈才遇到'slow'指针,可得 '(x+y)*2 = x+y+n(y+z)' 化简得到 'x = n(y+z)+z',这个公式告诉我们,无论'n'是多少,'fast'和'slow'两个指针的行走路径最终会汇聚到同一个位置--环的入口。这就意味着,从头节点出发一个指针,从相遇节点也出发一个指针,这两个指针每次只走一个节点,那么当这两个指针相遇的时候就是环形入口的节点。
*/
class Solution {
public:
ListNode *detectCycle(ListNode *head) {
ListNode* fast = head;
ListNode* slow = head;
while(fast != NULL && fast->next != NULL) {
slow = slow->next;
fast = fast->next->next;
// 快慢指针相遇,此时从head 和 相遇点,同时查找直至相遇
if (slow == fast) {
ListNode* index1 = fast;
ListNode* index2 = head;
while (index1 != index2) {
index1 = index1->next;
index2 = index2->next;
}
return index2; // 返回环的入口
}
}
return NULL;
}
};