1. 分支结构:if ifelse elseif switch
2. 循环结构:while dowhile for
一、分支
if分支
顺序结构
:if可以单点存在
package com.myif;
import java.util.Scanner;
/**
* @version 1.0
* @author:张先生
* @description:
* @date 2022/8/12 19:33
*/
public class TestIf {
public static void main(String[] args) {
System.out.println("上课");
//固定格式写--
Scanner luru = new Scanner(System.in);
//登录控制台录入--录入内容赋值给re
String re = luru.next();
if (re.equals("饿了")){
//equals 判断内容是否相等
System.out.println("吃饭");
}
System.out.println("写作业");
}
}
if else 双分支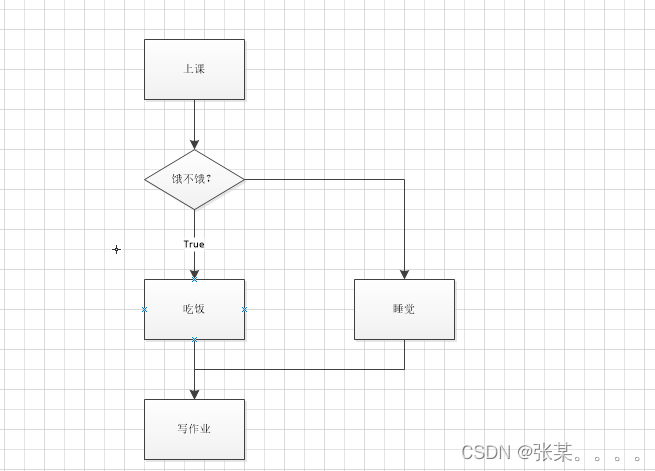
package com.myif;
import java.util.Scanner;
/**
* @version 1.0
* @author:张先生
* @description:
* @date 2022/8/12 19:33
*/
public class TestIf {
public static void main(String[] args) {
System.out.println("上课");
//固定格式写--
Scanner luru = new Scanner(System.in);
//登录控制台录入--录入内容赋值给re
String re = luru.next();
if (re.equals("饿了")){
//equals 判断内容是否相等
System.out.println("吃饭");
}else {
System.out.println("睡觉");
}
System.out.println("写作业");
}
}
--if可以独立存在——单分支
--else:如果if不成立将会执行else中的代码。如果语句块只有一句话:{}可以不写
public static void main(String[] args) {
System.out.println("上课");
Scanner luru=new Scanner(System.in);//固定格式写--
String re=luru.next();//登录控制台录入——录入内容赋值给re
if(re.equals("饿了")) //equals判断内容是否相等
System.out.println("吃饭");
else
System.out.println("睡觉");
System.out.println("写作业");
}
-- if可以嵌套:如果存在嵌套并且{}没写,else会匹配紧邻着的if
public class TestIf {
public static void main(String[] args) {
System.out.println("上课");
//固定格式写--
Scanner luru = new Scanner(System.in);
//登录控制台录入--录入内容赋值给re
String re = luru.next();
if (re.equals("饿了")){
//equals 判断内容是否相等
//System.out.println("吃饭");
String food = luru.next();
if (food.equals("炸酱面")){
System.out.println("去吃炸酱面");
}else {
System.out.println("吃方便面");
}
}else {
System.out.println("睡觉");
}
System.out.println("写作业");
}
}
else if多重分支
第一个条件成立:执行第一个后边的代码块
第一个不成立才会进行第二个判断:以此类推。
后边的每一个条件都是由前提:前边的所有条件都不成立。
最后都不成立执行的:else——可要可不要
public static void main(String[] args) {
System.out.println("起床");
Scanner scanner=new Scanner(System.in);
String xuanze=scanner.next();
if(xuanze.equals("开车")){
System.out.println("开车去");
}else if(xuanze.equals("公交")){
System.out.println("坐公交去");
}else if(xuanze.equals("打车")){//前边的条件不成立
System.out.println("打车去");
}else if(xuanze.equals("骑车")){
System.out.println("骑车去");
}else{
System.out.println("送我去");
}
System.out.println("办理业务");
}
:
public static void main(String[] args) {
//如果=100:放假 >=90:吃顿火锅 >=80:吃个鸡腿 >=60:教育 不及格:打一顿
Scanner sca=new Scanner(System.in);
int score=sca.nextInt();//next是录入字符串 nextInt是录入数字——必须是数字如果不是直接报错
//依赖默认前提的时候是不能乱序的——
if(score==100){
System.out.println("放假");
}else if(score>=90){//score>=90 && score<100
System.out.println("吃顿火锅");
}else if(score>=80){//score>=80 && score<90
System.out.println("吃个鸡腿");
}else if(score>=60){//score>=60 && score<80
System.out.println("教育");
}else{
System.out.println("打一顿");
}
System.out.println("--------------");
}
:
public static void main(String[] args) {
//输入年份 输入月份:输出:天数
Scanner sca=new Scanner(System.in);
int year=sca.nextInt();
int month=sca.nextInt();
if(month==1){
System.out.println("31");
}else if(month==3){
System.out.println("31");
}else if(month==5){
System.out.println("31");
}else if(month==7){
System.out.println("31");
}else if(month==8){
System.out.println("31");
}else if(month==10){
System.out.println("31");
}else if(month==12){
System.out.println("31");
}else if(month==2){
if((year%4==0&&year%100!=0)||year%400==0){
System.out.println("29");
}else{
System.out.println("28");
}
}else if(month==4){
System.out.println("30");
}else if(month==6){
System.out.println("30");
}else if(month==9){
System.out.println("30");
}else if(month==11){
System.out.println("30");
}else{
System.out.println("录入错误");
}
}
switch多重分支
switch从分支理解上来看:和elseif没有区别:判断第一个,不成立判断第二个不成立判断第三个,都不成立执行default——else
每一个判断都是等值判定——语法上case 值,然后相当于表达式==值,成立执行对应的代码块,不成立往后继续。
并且值有限定:类型:String 整型数字 char——8以前字符串都不行
swtich(表达式){
case 1:
case 2:
....
default:--可选
}
//输入年份 输入月份:输出:天数
Scanner sca=new Scanner(System.in);
int year=sca.nextInt();
int month=sca.nextInt();
switch(month){
case 1://case后边的代码块:所有case后的代码一直到swtich结束这些代码都属于他们的代码块
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
System.out.println("31");break;//中止switch
case 4:
case 6:
case 9:
case 11:
System.out.println("30");break;//中止switch
case 2:
if((year%4==0&&year%100!=0)||year%400==0){
System.out.println("29");break;//中止switch
}else{
System.out.println("28");break;//中止switch
}
default://不管default放到那个位置都是最后执行的:
System.out.println("输入错误");break;//中止switch
}
elseif做多分支:功能很强大,都可以做
switch做多分支:只能做等值,但是语法更简洁,效率更快
二、循环结构
循环的:意义就是想让一段代码重复执行。
想重复执行的那一段代码——循环体。
需要有中止循环的条件——循环条件(如果没有——这就是一个死循环)——条件表达式:1>1 1>=0--结果是固定的,不行--要不死循环 要不进不去。
循环条件中:一定有循环变量的:a>=1 b<=1这种写法,a循环变量。
循环变量需要初始化:变量不初始化不可以使用 如果a初始化为2,如果a的值不在改变,那么恒true。
循环变量在每一次的循环中需要更替。——改变——朝着可以将循环中止的方向改变。
循环:四个要求:
循环体 循环条件 循环变量更替 循环变量初始化
1 While循环
循环变量初始化;1
while(循环条件){2
循环体;3
循环变量更替4
}
1 - 2 - 3- 4- 2- 3 - 4- 2....一直到2的结果为false退出循环
int n=1;//初始值为46:while一次也进不去
while(n<=45){
System.out.print("点名"+n+"\t");
n=n+1;
}
do-while循环
循环变量初始化;1
do{
循环体;3
循环变量更替;4
}while(循环条件);2
1--3--4--2--3--4--2--3--4--2----一直到2为false、
先执行后判断:保证了do-while至少执行一次.
int n=1;//初始值为46:do——while最少执行一次
do{
System.out.print("点名"+n+"\t");
n=n+1;
}while(n<=45);
for循环
执行过程与while一样:区别就是语法不同
for(循环变量初始化1;循环条件2;循环变量更替4){
循环体3
};
1-2-3-4-2-3-4-2-3-4......
for(int tian=1;tian<=30;tian++){
//点名:每次点名都是45次
for(int j=1;j<=45;j++){
System.out.print("点名"+j+"\t");
}
System.out.println();
System.out.println("第"+tian+"点名完毕");
}
循环嵌套
循环嵌套:9*9乘法表
class 乘法表{
public static void main(String[] args) {
for(int i=1;i<=9;i++){
for(int j=1;j<=i;j++){
System.out.print(j+"*"+i+"="+i*j+"\t");
}
System.out.println();
}
}
}
break和continue
break:
break退出循环,如果代码走到break,循环终止
for(int i=1;i<150;i++ ){
System.out.print("点名"+i+"\t");
if(i>45){
break;//退出循环的--代码会回到所属循环的外部执行后边的代码
}
}
contine:终止本次:继续下一次
while:执行contiue避开了当前这一次的后续代码——后续代码有可能有变量所以有可能出问题,需要自己手动调整自己的代码
for(int j=1;j<=45;j++){
//20号请假,不点
if(j==20){
continue;
}
System.out.print("点名"+j+"\t");//代码块3:
}//