1.简介
SpringBoot是为了简化Spring 应用的搭建和开发过程
SpringBoot 可以自动扫描到要加载的信息并启动相应的默认程序
2.特点
- 为所有的Spring开发提供从根本上更快且可广泛访问的入门体验
- 可以创建独立的Spring 应用程序,并且基于其Maven或Gradle插件,可以创建可执行的jat包和war包
- 开箱即用特性,提供自动配置的“Starter”项目对象模型(POMS)以达到简化Maven配置的作用
- 内嵌Tomcat或者Jetty等Servlet容器
- 尽可能自动配置Spring容器
- 提供一系列的大型项目的通用的非功能特征。
- 绝对没有代码生成,不需要XML配置
SpringBoot项目创建方法
方式一,创建一个maven项目
创建maven工程
修改pom.xml配置
<parent><groupId> org.springframework.boot </groupId><artifactId> spring-boot-starterparent </artifactId><version> 2.6.6 </version></parent>
添加依赖
<dependencies><dependency><groupId> org.springframework.boot </groupId><artifactId> spring-boot-starter-web </artifactId></dependency></dependencies>
编写代码
@SpringBootApplication@RestControllerpublic class DemoApplication {@RequestMapping ( "/hello" )public String hello (){return "hello world!" ;}public static void main ( String [] args ) {SpringApplication . run ( DemoApplication . class ,args );}
方式二 , 官网向导创建
打开官网向导
打开浏览器,输入网址
http://start.spring.io/
,如图 所示
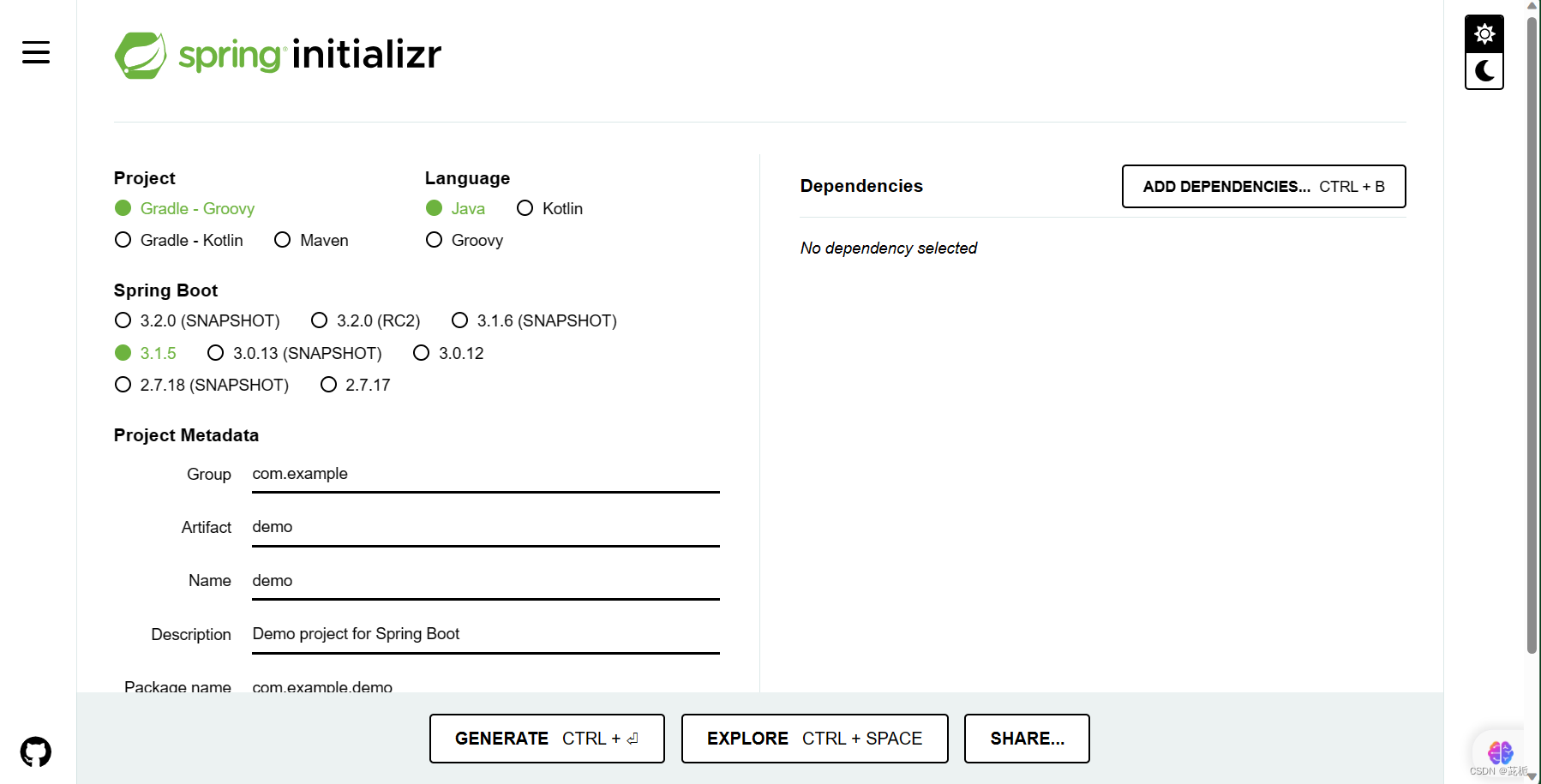
创建一个最简单的
SpringBoot
应用,填写项目信息。
GENERATE :
直接生成并下载项目
EXPLORE :
查看项目
SHARE
: 分享项目
导入IDEA
main方法
程序的最后一部分是main方法。这是遵循java约定的应用程序入口点的标准方法,main方法SpringApplication通过调用委托给Spring Boot 的类run。SpringApplication引用应用程序,启用Spring,这反过来又起来了自动配置的Tomcat Web服务器。
@SpringBootApplication@RestControllerpublic class DemoApplication {@RequestMapping ( "/hello" )public String hello (){return "hello world!" ;}public static void main ( String [] args ) {SpringApplication . run ( DemoApplication . class ,args );}}
方式三 ,IDEA向导创建
初识Spring Boot Starter
传统的Spring与Spring Boot Starter相比
1.传统的Spring还要配置繁琐的配置文件(不仅要导入各种依赖,配置XML文件)
2.相比之下Spring Boot 项目在创建完成后,即便不写代码,不进行任何配置也可以运行,这都归功于Spring Boot的Starter机制
什么叫starter
1,starter是Spring Boot 将日常企业应用研发中的各种场景都抽了出来,这成了一个个的启动器(starter)。
2.starter中整合了该场景下的各种可能用的到的依赖,用户只需要在Maven 中引入starter依赖,SpringBoot 就可以自动扫描要加载的相应的默认配置并启动
3.starter提供了大量的自动配置,让用户摆脱了各种依赖和配置的烦恼
总结:所有的这些都遵循starter的约定俗成的默认配置,并且允许用户调整这些配置,即遵循“约定大于配置”的原则。
pom,xml中引入spring-boot-starter-web
<?xml version="1.0" encoding="UTF-8"?><projectxmlns = "http://maven.apache.org/POM/4.0.0"xmlns:xsi = "http://www.w3.org/2001/XMLSchemainstance"xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd" ><modelVersion> 4.0.0 </modelVersion><!--SpringBoot 父项目依赖管理 --><parent><groupId> org.springframework.boot </groupId><artifactId> spring-boot-starterparent </artifactId><version> 2.6.6 </version><relativePath/> <!-- lookup parentfrom repository --></parent><groupId> com.by </groupId><artifactId> sdemo </artifactId><version> 0.0.1-SNAPSHOT </version><name> sdemo </name><description> sdemo </description><properties> <java.version> 1.8 </java.version></properties><dependencies><!-- 导入 spring-boot-starter-web--><dependency><groupId> org.springframework.boot </groupId><artifactId> spring-boot-starterweb </artifactId></dependency><dependency><groupId> org.springframework.boot </groupId><artifactId> spring-boot-startertest </artifactId><scope> test </scope></dependency></dependencies><build><plugins><plugin><groupId> org.springframework.boot </groupId><artifactId> spring-bootmaven-plugin </artifactId></plugin> </plugins></build></project>
- 我们在Spring中导入了springftamework,logging,jackson以及Tomcat等依赖,这正是五年在开发web项目时所需要的。
- 在以上配置中我们没有表明spring-boot-stater-web 依赖的版本(version),但是在依赖树中的依赖都有版本信息,那么这些版本是在那里控制的呢?是由Spring-boot-starter-parent(版本仲裁中心) 统一控制的。
Spring-boot-starter-parent
Spring Boot 项目可以通过继承 spring-boot-starterparent 来获得一些合理的默认配置默认 JDK 版本( Java 8 )默认字符集( UTF-8 )依赖管理功能<!--SpringBoot 父项目依赖管理 --><parent><groupId> org.springframework.boot </groupId><artifactId> spring-boot-starterparent </artifactId><version> 2.6.6 </version><relativePath/> <!-- lookup parent fromrepository --></parent> 资源过滤默认插件配置识别 application.properties 和 application.yml 类型的配置文件查看 spring-boot-starter- parent 的 pom 文件可以发现其有一个父级依赖 spring-boot-dependenciesspring-boot-starter-parent-2.6.6.pomspring-boot-dependencies-2.6.6.pom<?xml version="1.0" encoding="UTF-8"?><projectxmlns = "http://maven.apache.org/POM/4.0.0"xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0http://maven.apache.org/xsd/maven-4.0.0.xsd"xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" ><modelVersion> 4.0.0 </modelVersion><groupId> org.springframework.boot </groupId> <artifactId> spring-bootdependencies </artifactId><version> 2.6.6 </version><packaging> pom </packaging><name> spring-boot-dependencies </name>.....<properties><activemq.version> 5.16.4 </activemq.version>...<freemarker.version> 2.3.31 </freemarker.version>....<hibernate.version> 5.6.7.Final </hibernate.version><hibernatevalidator.version> 6.2.3.Final </hibernatevalidator.version>....<jakarta-json.version> 1.1.6 </jakartajson.version><jakarta-json-bind.version> 1.0.2 </jakartajson-bind.version>....<mysql.version> 8.0.28 </mysql.version></properties><dependencyManagement> ....</dependencyManagement><build><pluginManagement>....</pluginManagement></build></project>以上配置中,部分元素说明如下:dependencyManagement :负责管理依赖;pluginManagement :负责管理插件;properties :负责定义依赖或插件的版本号。spring-boot-dependencies 通过dependencyManagement 、 pluginManagement 和properties 等元素对一些常用技术框架的依赖或插件进行了统一版本管理,例如 Activemq 、 Spring 、 Tomcat 等。